实现一个图形类(Shape),包含受保护成员属性:周长、面积,
公共成员函数:特殊成员函数书写
定义一个圆形类(Circle),继承自图形类,包含私有属性:半径
公共成员函数:特殊成员函数、以及获取周长、获取面积函数
定义一个矩形类(Rect),继承自图形类,包含私有属性:长度、宽度
公共成员函数:特殊成员函数、以及获取周长、获取面积函数
在主函数中,分别实例化圆形类对象以及矩形类对象,并测试相关的成员函数。
#include <iostream>
using namespace std;
class Shape
{
protected:
double c;
double s;
public:
//构造函数
Shape(){
cout<<"无参构造"<<endl;
}
Shape (double c,double s):c(c),s(s)
{
cout<<"有参构造"<<endl;
}
//析构函数
~Shape(){cout<<"析构函数"<<endl;}
//拷贝构造函数
Shape(const Shape &other)
{
c=other.c;
s=other.s;
cout<<"拷贝构造函数"<<endl;
}
//拷贝赋值函数
Shape &operator=(const Shape &other)
{
c=other.c;
s=other.s;
cout<<"拷贝赋值函数"<<endl;
return *this;
}
};
class Circle:public Shape
{
private:
double r;
public:
//构造函数
Circle(){
cout<<"圆的无参构造"<<endl;
}
Circle (double c, double s,double r):Shape(c,s),r(r)
{
cout<<"圆的有参构造"<<endl;
}
Circle(double r):r(r)
{
c=2*3.14*r;
s=3.14*r*r;
cout<<"圆的一个参数的有参构造"<<endl;
}
//析构函数
~Circle(){cout<<"圆的析构函数"<<endl;}
//拷贝构造函数
Circle(const Circle &other)
{
r=other.r;
c=other.c;
s=other.s;
cout<<"圆的拷贝构造函数"<<endl;
}
//拷贝赋值函数
Circle &operator=(const Circle &other)
{
r=other.r;
c=other.c;
s=other.s;
cout<<"圆的拷贝赋值函数"<<endl;
return *this;
}
//获取周长
double get_C()
{
cout<<"圆的周长="<<c<<endl;
return c;
}
//获取面积函数
double get_S()
{
cout<<"圆的面积="<<s<<endl;
return s;
}
};
class Rect:public Shape
{
private:
double l;
double w;
public:
//构造函数
Rect(){
cout<<"矩形的无参构造"<<endl;
}
Rect (double c, double s,double l,double w):Shape(c,s),l(l),w(w)
{
cout<<"矩形的有参构造"<<endl;
}
Rect(double l,double w):l(l),w(w)
{
c=2*(l+w);
s=l*w;
cout<<"矩形的长宽参数的有参构造"<<endl;
}
//析构函数
~Rect(){cout<<"矩形的析构函数"<<endl;}
//拷贝构造函数
Rect(const Rect &other)
{
l=other.l;
w=other.w;
c=other.c;
s=other.s;
cout<<"矩形的拷贝构造函数"<<endl;
}
//拷贝赋值函数
Rect &operator=(const Rect &other)
{
l=other.l;
w=other.w;
c=other.c;
s=other.s;
cout<<"矩形的拷贝赋值函数"<<endl;
return *this;
}
//获取周长
double get_C()
{
cout<<"矩形的周长="<<c<<endl;
return c;
}
//获取面积函数
double get_S()
{
cout<<"矩形面积="<<s<<endl;
return s;
}
};
int main()
{
Shape s1(12,9); //有参构造
Shape s2=s1; //拷贝构造函数
Shape s3; //无参构造
s3=s1; //拷贝赋值函数
Circle c1(6*3.14,9*3.14,3); //圆的有参构造
Circle c2=c1; //圆的拷贝构造函数
Circle c3; //圆的无参构造
c3=c1; //圆的拷贝赋值函数
c3.get_C(); //获取周长
Circle c4(4); //圆的一个参数的有参构造
c4.get_C(); //获取周长
c4.get_S(); //获取面积
Rect r1(3,4); //矩形的长宽两个参数的有参构造
Rect r2=r1; //矩形的拷贝构造函数
Rect r3; //矩形的无参构造
r3=r1; //矩形的拷贝赋值函数
r3.get_C(); //获取矩形的周长
r3.get_S(); //获取矩形的面积
return 0;
}
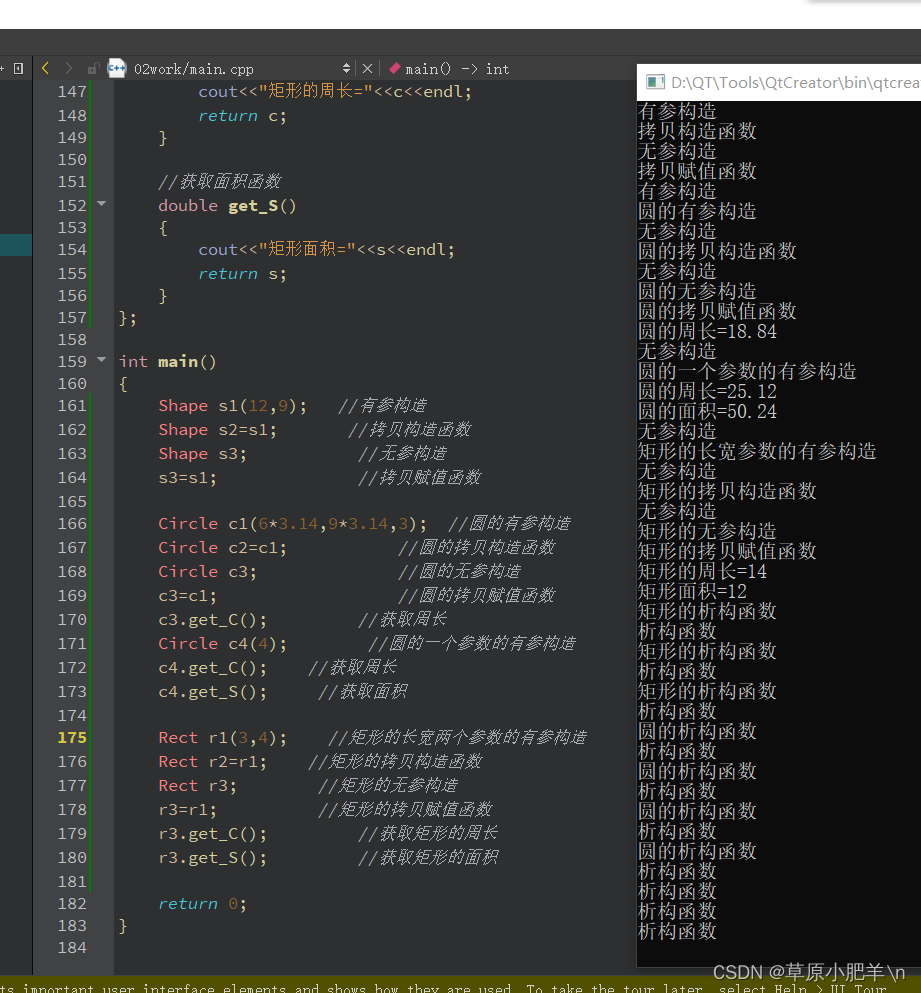
思维导图
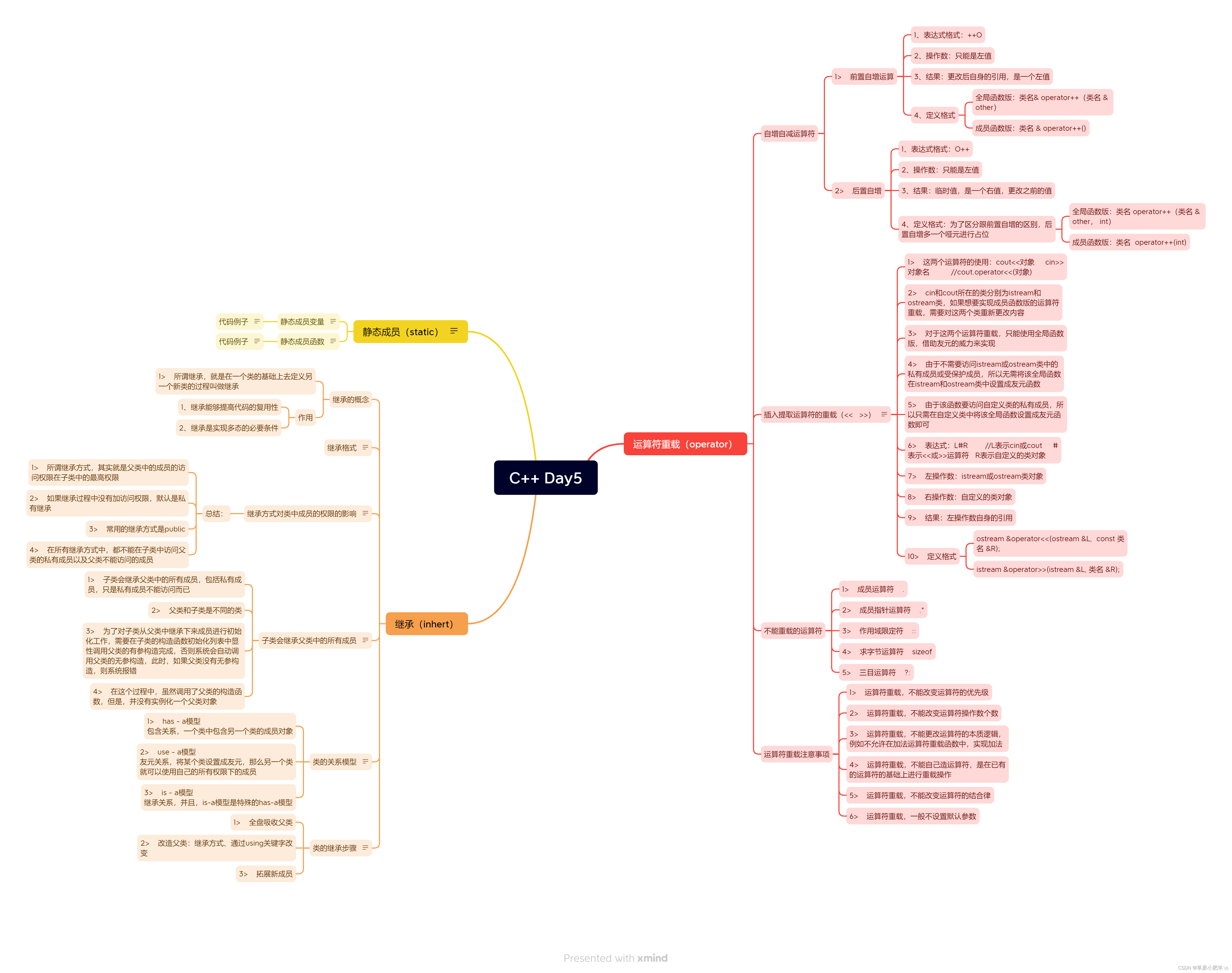