//尾删 (作业)
int list_delete_tail(LinkList L);
// 尾删
int list_delete_tail(linklist l)
{
list_delete_pos(l,l->len);
}
// 按位置删
int list_delete_pos(linklist l,int pos)
{
if(NULL==l || list_empty(l) || pos<1 || pos>l->len){
printf("删除失败\n");
return -1;
}
linklist p=list_find_node_pos(l,pos-1);
linklist q = p->next->next;
free(p->next);
p->next = q;
l->len--;
return 0;
}
// 按位查找返回结点
linklist list_find_node_pos(linklist l,int pos)
{
if(NULL==l || pos<0 || pos>l->len){
printf("查找失败\n");
return NULL;
}
while(pos){
l=l->next;
pos--;
}
return l;
}
//按值进行修改函数(作业)
int list_update_value(LinkList L, datatype old_e, datatype new_e);
// 按值修改
int list_update_value(linklist l,datatype old_e,datatype new_e){
if(NULL==l || list_empty(l)){
printf("修改失败");
return -1;
}
linklist p = list_search_value(l,old_e);
if(NULL==p){
printf("未找到值\n");
return -2;
}
while(p){
p->data = new_e;
p = list_search_value(l,old_e);
}
// printf("修改完成\n");
return 0;
}
// 按值查找 返回结点
linklist list_search_value(linklist l,datatype e)
{
if(NULL==l || list_empty(l)){
printf("查找失败\n");
return NULL;
}
int pos = 1;
l=l->next;
while(l){
if(l->data==e){
// printf("在第%d位\n",pos);
return l;
}
pos++;
l=l->next;
}
// printf("未找到值\n");
return NULL;
}
//链表翻转
void list_reverse(LinkList L);
// 链表翻转
void list_reverse_sub(linklist l)
{
if(l->next){
list_reverse_sub(l->next);
l->next->next=l;
}
}
void list_reverse(linklist l)
{
if(NULL==l || list_empty(l)){
printf("表不合理\n");
return;
}
linklist tail_node = list_find_node_pos(l,l->len);
if(NULL==tail_node){
printf("倒序失败\n");
return;
}
list_reverse_sub(l->next);
l->next->next=NULL;
l->next=tail_node;
}
//链表合并
void list_merge(LinkList L, LinkList H);
// 链表合并
void list_merge(linklist l1,linklist *l2)
{
if(NULL==l1 || NULL==*l2 || list_empty(l1) || list_empty(*l2)){
printf("合并失败");
return;
}
while(l1->next){
l1=l1->next;
}
l1->next=(*l2)->next;
free(*l2);
*l2=NULL;
printf("合并完成\n");
}
main.c
#include <stdio.h>
#include <stdlib.h>
#include "linklist.h"
int main(int argc, const char *argv[])
{
int a;
linklist l = list_create();
if(NULL == l){
return -1;
}
linklist l2 = list_create();
if(NULL == l2){
return -1;
}
list_insert_head(l,'Y');
list_insert_head(l,'h');
list_insert_head(l,'e');
list_insert_head(l,'l');
list_insert_head(l,'l');
list_insert_head(l,'o');
list_insert_head(l,'w');
printf("创建l1:");
list_show(l);
printf("删除l1尾结点:");
list_delete_tail(l);
list_show(l);
printf("将l1内的l修改为L:");
list_update_value(l,'l','L');
list_show(l);
printf("翻转l1:");
list_reverse(l);
list_show(l);
list_insert_head(l2,'w');
list_insert_head(l2,'o');
list_insert_head(l2,'r');
list_insert_head(l2,'l');
list_insert_head(l2,'d');
printf("创建l2:");
list_show(l2);
printf("&l2:%p\n",&l2);
printf("合并l1,l2\n");
list_merge(l,&l2);
list_show(l);
printf("&l2:%p\n",l2);
printf("&l1:%p\n",l);
list_free(&l);
printf("&l1:%p\n",l);
return 0;
}
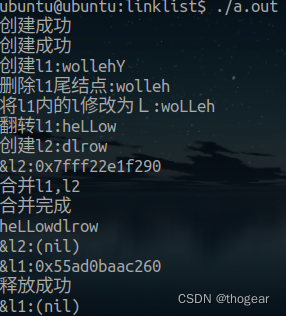