效果图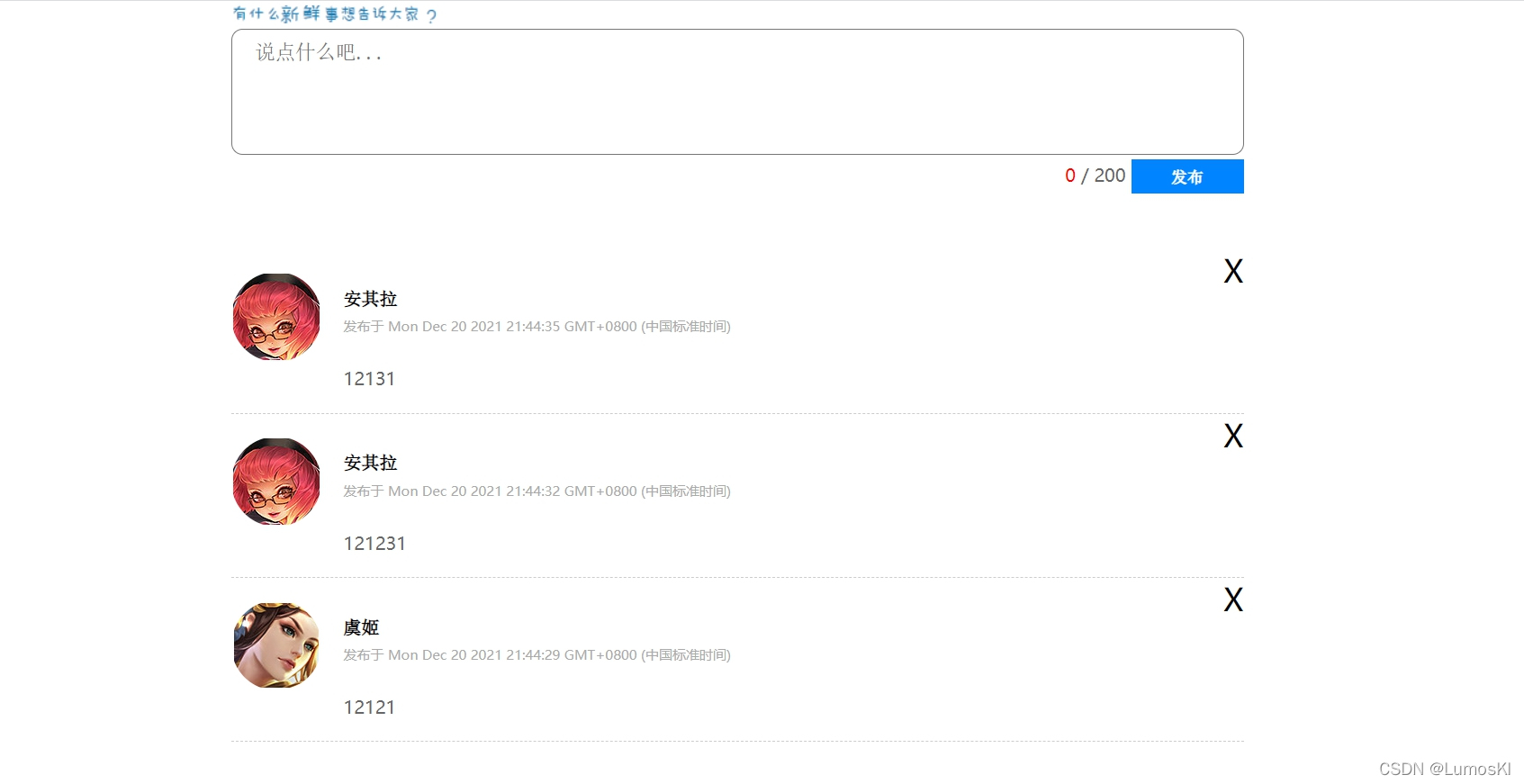
先整理一下思路:
1.文本域输入事件:获取文本域内容长度 赋值给 span标签文本
文本域文本:area.value
span标签文本:span.innerText
2.发布按钮点击事件:
2.1 非空判断:判断用户有没有输入 去除字符串
3.点击删除按钮:删除按钮所在的元素
CSS样式:
<style>
* {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
.w {
width: 900px;
margin: 0 auto;
}
.controls textarea {
width: 878px;
height: 100px;
resize: none;
border-radius: 10px;
outline: none;
padding-left: 20px;
padding-top: 10px;
font-size: 18px;
}
.controls {
overflow: hidden;
}
.controls div {
float: right;
}
.controls div span {
color: #666;
}
.controls div .useCount {
color: red;
}
.controls div button {
width: 100px;
outline: none;
border: none;
background: rgb(0, 132, 255);
height: 30px;
cursor: pointer;
color: #fff;
font: bold 14px '宋体';
transition: all 0.5s;
}
.controls div button:hover {
background: rgb(0, 225, 255);
}
.controls div button:disabled {
background: rgba(0, 225, 255, 0.5);
}
.contentList {
margin-top: 50px;
}
.contentList li {
padding: 20px 0;
border-bottom: 1px dashed #ccc;
position: relative;
}
.contentList li .info {
position: relative;
}
.contentList li .info span {
position: absolute;
top: 15px;
left: 100px;
font: bold 16px '宋体';
}
.contentList li .info p {
position: absolute;
top: 40px;
left: 100px;
color: #aaa;
font-size: 12px;
}
.contentList img {
width: 80px;
border-radius: 50%;
}
.contentList li .content {
padding-left: 100px;
color: #666;
word-break: break-all;
}
.contentList li .the_del {
position: absolute;
right: 0;
top: 0;
font-size: 28px;
cursor: pointer;
}
</style>
js样式:
<div class="w">
<!-- 操作的界面 -->
<div class="controls">
<img src="./images/9.6/tip.png" alt="" /><br />
<!-- maxlength 可以用来限制表单输入的内容长度 -->
<textarea placeholder="说点什么吧..." id="area" cols="30" rows="10" maxlength="200"></textarea>
<div>
<span class="useCount" id="useCount">0</span>
<span>/</span>
<span>200</span>
<button id="send">发布</button>
</div>
</div>
<!-- 微博内容列表 -->
<div class="contentList">
<ul id="list"></ul>
</div>
</div>
<!-- 添加了hidden属性元素会直接隐藏掉 -->
<li hidden>
<div class="info">
<img class="userpic" src="./images/9.6/03.jpg" />
<span class="username">死数据:百里守约</span>
<p class="send-time">死数据:发布于 2020年12月05日 00:07:54</p>
</div>
<div class="content">死数据:111</div>
<span class="the_del">X</span>
</li>
<script>
// maxlength 是一个表单属性, 作用是给表单设置一个最大长度
// 模拟数据
let dataArr = [{
uname: '司马懿',
imgSrc: './images/9.5/01.jpg'
}, {
uname: '女娲',
imgSrc: './images/9.5/02.jpg'
}, {
uname: '百里守约',
imgSrc: './images/9.5/03.jpg'
}, {
uname: '亚瑟',
imgSrc: './images/9.5/04.jpg'
}, {
uname: '虞姬',
imgSrc: './images/9.5/05.jpg'
}, {
uname: '张良',
imgSrc: './images/9.5/06.jpg'
}, {
uname: '安其拉',
imgSrc: './images/9.5/07.jpg'
}, {
uname: '李白',
imgSrc: './images/9.5/08.jpg'
}, {
uname: '阿珂',
imgSrc: './images/9.5/09.jpg'
}, {
uname: '墨子',
imgSrc: './images/9.5/10.jpg'
}, {
uname: '鲁班',
imgSrc: './images/9.5/11.jpg'
}, {
uname: '嬴政',
imgSrc: './images/9.5/12.jpg'
}, {
uname: '孙膑',
imgSrc: './images/9.5/13.jpg'
}, {
uname: '周瑜',
imgSrc: './images/9.5/14.jpg'
}, {
uname: '老夫子',
imgSrc: './images/9.5/15.jpg'
}, {
uname: '狄仁杰',
imgSrc: './images/9.5/16.jpg'
}, {
uname: '扁鹊',
imgSrc: './images/9.5/17.jpg'
}, {
uname: '马可波罗',
imgSrc: './images/9.5/18.jpg'
}, {
uname: '露娜',
imgSrc: './images/9.5/19.jpg'
}, {
uname: '孙悟空',
imgSrc: './images/9.5/20.jpg'
}, {
uname: '黄忠',
imgSrc: './images/9.5/21.jpg'
}, {
uname: '百里玄策',
imgSrc: './images/9.5/22.jpg'
}]
let useCount = document.querySelector('.useCount') //span
let send = document.querySelector('#send') //发布按钮
let list = document.querySelector('#list') //ul列表
//获取文本域内容长度 赋值给 span标签文本
area.oninput = function() {
useCount.innerText = area.value.length
}
//2.点击发布事件
send.onclick = function() {
//3.1非空判断
if (area.value.trim() == '') {
alert('请输入合法内容')
} else {
//3.2 新增元素
//(1).创建空li元素
let li = document.createElement('li')
//(2).设置li内容 生成数组随机下标
let index = parseInt(Math.random() * dataArr.length)
li.innerHTML = `
<div class="info">
<img class="userpic" src="${dataArr[index].imgSrc}" >
<span class="username">${dataArr[index].uname}</span>
<p class="send-time">发布于 ${Date().toLocaleString()}</p>
</div>
<div class="content">${area.value}</div>
<span class="the_del">X</span>`
//(3).添加到ul最前面
list.insertBefore(li, list.children[0])
//一定要有li,才可以获取删除按钮
li.querySelector('.the_del').onclick = function() {
list.removeChild(this.parentNode)
}
}
//4.清空表单文本和内容
area.value = ''
useCount.innerText = 0
}
</script>
大家可以把图片更换成自己喜欢的图片哦,仅供参考哈!