#include <iostream>
using namespace std;
class stu
{
private:
int num[20];
int top;
public:
//构造
stu(int n,int t)
{
num[top]=n;
top=t;
cout<<"stu::构造函数"<<endl;
}
//析构
~stu()
{
cout<<"stu::析构函数"<<this<<endl;
}
//拷贝
stu()
{
cout<<"拷贝构造函数"<<endl;
}
public:
//判空
bool stack_empty()
{
if(-1==top)
{
return 1;
}
return 0;
}
//判满
bool stack_full()
{
if(19==top)
{
return 1;
}
return 0;
}
//入栈
void stack_push(int n)
{
this->top++;
this->num[this->top]=n;
cout<<"入栈成功"<<endl;
}
//出栈
void stack_pop()
{
if(stack_empty())
{
cout<<"出栈失败"<<endl;
}
int n = this->num[this->top];
cout<<"出栈成功:"<<n<<endl;
this->top--;
}
//遍历栈
void stack_show()
{
if(stack_empty())
{
cout<<"遍历失败"<<endl;
}
cout<<"从栈顶到栈底分别为"<<endl;
for(int i=this->top;i>=0;i--)
{
cout<<this->num[i]<<" ";
}
cout<<endl;
}
//清空栈
void stack_null()
{
if(stack_empty())
{
cout<<"清空失败"<<endl;
}
for(int i=this->top;i>=0;i--)
{
this->num[i]=NULL;
}
cout<<"清空成功"<<endl;
}
//获取栈顶元素
void stack_top()
{
cout<<"栈顶元素"<<this->num[this->top]<<endl;
}
//求栈的大小
void stack_num()
{
cout<<"栈的大小:"<<this->top+1<<endl;
}
};
int main()
{
stu s1(0,-1);
s1.stack_push(6);
s1.stack_push(8);
s1.stack_push(9);
s1.stack_show();
s1.stack_top();
s1.stack_num();
s1.stack_null();
s1.stack_show();
return 0;
}
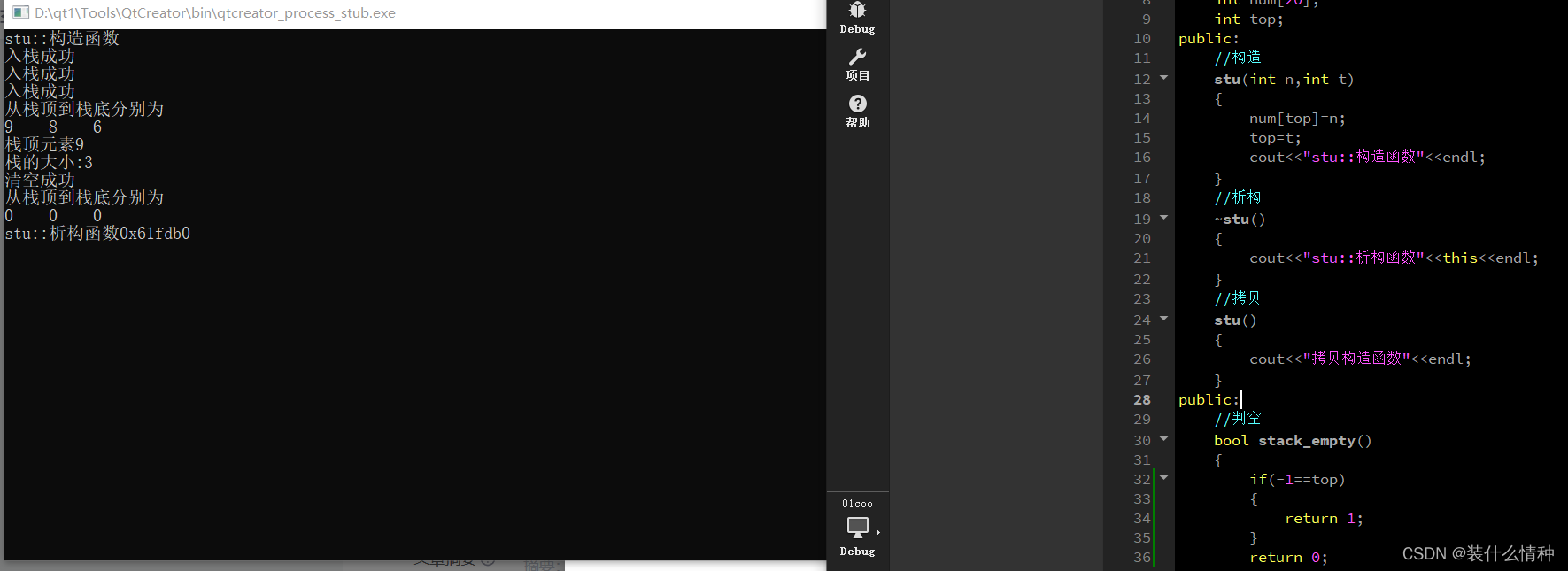
#include <iostream>
using namespace std;
class stu
{
private:
int num[20];
int head;
int low;
public:
stu(int h,int l)
{
head=h;
low=l;
cout<<"有参构造"<<endl;
}
~stu()
{
cout<<"析构函数"<<endl;
}
stu(const stu &other):head(other.head),low(other.low)
{
cout<<"拷贝构造函数"<<endl;
}
bool queue_empty()
{
if(this->low==this->head)
{
return 1;
}
return 0;
}
bool queue_full()
{
if((this->low+1)%20==head)
{
return 1;
}
return 0;
}
void queue_push(int n)
{
if(queue_full())
{
cout<<"队列已满"<<endl;
}
this->num[this->low]=n;
this->low=(this->low+1)%20;
cout<<"入队成功"<<endl;
}
void queue_pop()
{
if(queue_empty())
{
cout<<"出队失败"<<endl;
}
cout<<"出队成功"<<this->num[this->head]<<endl;
this->head=(this->head+1)%20;
}
void queue_null()
{
while(1)
{
cout<<this->num[this->head]<<endl;
this->head=(this->head+1)%20;
if(this->head==this->low)
{
cout<<"清空成功"<<endl;
break;
}
}
}
void queue_size()
{
cout<<"队列长度"<<(this->low-this->head+20)%20<<endl;
}
};
int main()
{
stu p1(0,0);
p1.queue_push(5);
p1.queue_push(8);
p1.queue_push(10);
p1.queue_pop();
p1.queue_size();
p1.queue_null();
p1.queue_size();
return 0;
}
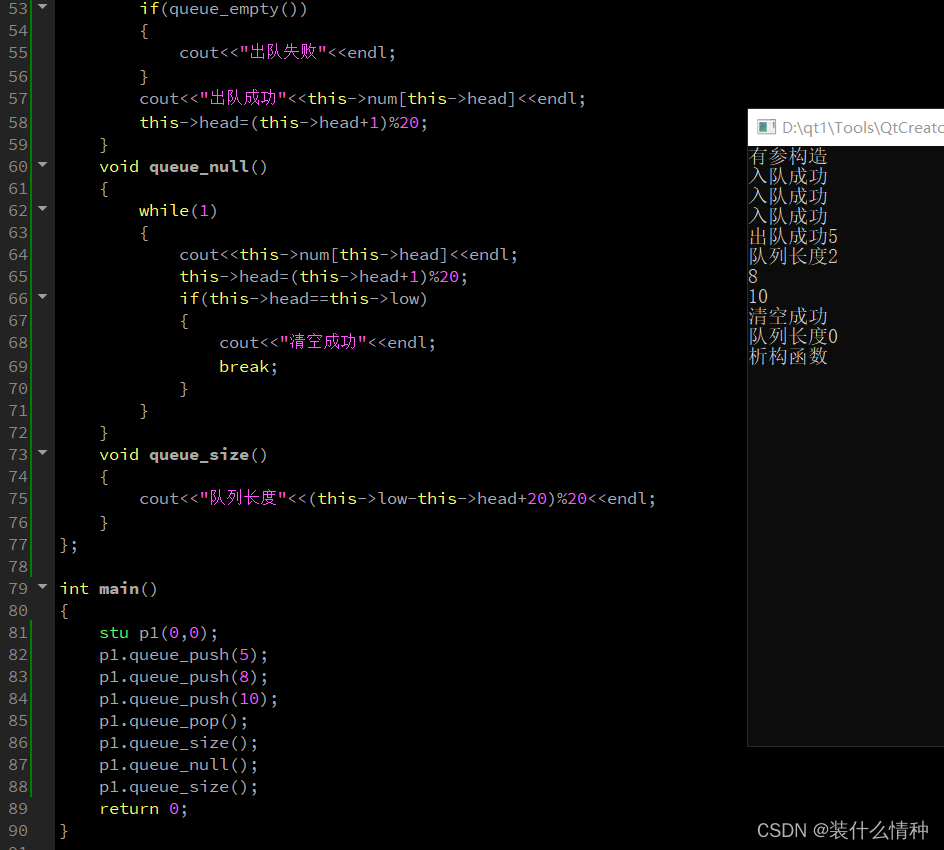