-
JavaScript 中的对象分为3种:自定义对象 、内置对象、 浏览器对象
-
内置对象就是指 JS 语言自带的一些对象,这些对象供开发者使用,并提供了一些常用的或是最基本而必要的功能
-
JavaScript 提供了多个内置对象:Math、 Date 、Array、String等
-
JS中常用的Date内置对象&处理Date内置对象的一些方法:http://t.csdn.cn/ge2w0
目录
2.Math.max()/Math.min()求最大值/最小值
e.g.1Math.max()求数字最大值【Max.min()同理】
e.g.2Math.max(...arr)/Math.min(...arr)求数组中数字最大值/最小值
4.Math.random() 返回一个随机小数,其取值范围是 [0,1),左闭右开 0 <= x < 1 【该方法不含参数】
e.g.2Math.random() 要想得到两个数之间【包含这两个数】的随机整数
(2)Math.random()让用户猜[1,50]之间一个数字,只有10次机会
3.Math.round() 四舍五入,其他数字都是四舍五入,.5往大取
Math对象
Math 对象不是构造函数,所以不需要用new来调用。
直接使用属性和方法。
跟数学相关的运算(求绝对值,取整、最大值等)可以使用 Math 中的成员。
以下方法必须有()
Math.PI // 圆周率
Math.floor() // 向下取整
Math.ceil() // 向上取整
Math.round() // 其他数四舍五入,.5往大里取 e.g. Math.round(-1.5) 结果是-1
Math.abs() // 绝对值
Math.max()/Math.min() // 求最大值/最小值
1.Math.PI圆周率
console.log(Math.PI);//圆周率3.141592653589793
2.Math.max()/Math.min()求最大值/最小值
e.g.1Math.max()求数字最大值【Max.min()同理】
console.log(Math.max(1, 99, 3));//99
//如果 有非数字参数
console.log(Math.max(1, 99, 'pink老师'));//NaN
//如果 无参数
console.log(Math.max());//-Infinity
e.g.2Math.max(...arr)/Math.min(...arr)求数组中数字最大值/最小值
var arr = [1, 33, 2, 88, 40];
console.log(Math.max(...arr));//88
console.log(Math.min(...arr));//1
利用对象封装自己的数学对象,里面有PI,最大值,最小值
var myMath = {
PI: 3.141592653,
max: function() {
var max = arguments[0];
for (var i = 1; i < arguments.length; i++) {
if (arguments[i] > max) {
max = arguments[i];
}
}
return max;
},
min: function() {
var min = arguments[0];
for (var i = 1; i < arguments.length; i++) {
if (arguments[i] < min) {
min = arguments[i];
}
}
return min;
}
}
console.log(myMath.PI);//圆周率3.141592653589793
console.log(myMath.max(1, 5, 9));//9
console.log(myMath.min(1, 5, 9));//1
3.Math.abs() 绝对值
console.log(Math.abs(1)); // 1
console.log(Math.abs(-1)); // 1
console.log(Math.abs('-1')); // 1 隐式转换,会把字符串-1 转换为数字型1
//如果参数是 非数字
console.log(Math.abs('pink'));//NaN
4.Math.random() 返回一个随机小数,其取值范围是 [0,1),左闭右开 0 <= x < 1 【该方法不含参数】
e.g.1Math.random() 返回一个随机小数
console.log(Math.random());//0.33675050626823744
e.g.2Math.random() 要想得到两个数之间【包含这两个数】的随机整数
//随机生成[1,10]之间的整数
function getRandom(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
console.log(getRandom(1, 10));//10
e.g.3Math.random()随机点名
function getRandom(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
var arr = ['张三', '李四', '王五', '赵六'];
console.log(arr[getRandom(0, arr.length - 1)]);//赵六
e.g.4Math.random()猜数字游戏
(1)让用户猜[1,10]之间的一个数字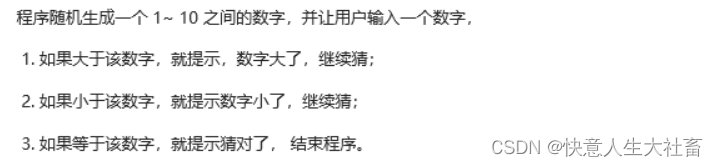
function getRandom(min,max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
var random = getRandom(1,10);
while(true) { //死循环 ,需要退出循环条件
var num = prompt('请输入1~10之间的一个整数:');
if(num > random) {
alert('你猜大了');
}else if (num < random) {
alert('你猜小了');
}else {
alert('你猜中了');
break; //退出整个循环
}
}
(2)Math.random()让用户猜[1,50]之间一个数字,只有10次机会
function getRandom(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
var random = getRandom(1, 50);
for (var i = 1; 1 <= 10; i++) {
var num = prompt('请输入1-50之间的一个数字');
if (num > random) {
alert('你猜大了');
} else if (num < random) {
alert('你猜小了');
} else if (num == 'exit') {
break;
} else {
alert('你猜对了');
break;
}
if (i == 10 && num != random) {
alert('10次机会已用完,游戏结束');
}
}
三个取整方法:
1.Math.floor() 向下取整,往小取
console.log(Math.floor(1.1)); // 1
console.log(Math.floor(1.9)); // 1
2.Math.cell() 向上取整,往大取
console.log(Math.ceil(1.1)); //2
console.log(Math.ceil(1.9)); //2
3.Math.round() 四舍五入,其他数字都是四舍五入,.5往大取
console.log(Math.round(1.1)); //1 四舍五入
console.log(Math.round(1.5)); //2
console.log(Math.round(1.9)); //2 四舍五入
console.log(Math.round(-1.1)); // -1 四舍五入
console.log(Math.round(-1.5)); // -1