项目场景:
发放一个总额为20万元的红包,拆分为2万个可抢的小红包。假设每个小红包都是10元,供网站用户抢夺,网站同时存在3万用户在线抢夺,典型的一个高并发场景。
目录
1.数据库表设计
分为两张表,一张表为红包表,一张表是用户抢红包表
create database red_packet;
# 红包表
create table T_RED_PACKET(
id INT(12) not null auto_increment, -- 红包编号
user_id int(12) not null, -- 发红包用户
amount decimal(16,2) not null, -- 红包金额
send_date datetime not null, -- 发红包时间
total int(12) not null, -- 小红包总数
unit_amount decimal(12) not null, -- 单个小红包金额
stock int(12) not null, -- 剩余小红包个数
version int(12) default 0 not null, -- 版本
note varchar(256) null, -- 备注
primary key clustered (id)
)
# 用户抢红包表
create table T_USER_RED_PACKET(
id int(12) not null auto_increment, # 编号
red_packet_id int(12) not null, # 红包编号
user_id int(12) not null, # 抢用户编号
amount decimal(16,2) not null, # 抢红包金额
grab_time datetime not null, # 抢红包时间
note varchar(256) null, # 备注
primary key clustered(id)
)
# 插入一个20万元金额,2万个小红包,每个10元的红包数据
insert into T_RED_PACKET(user_id,amount,send_date,total,unit_amount,stock,note)
values (1,200000.00, now(), 20000, 10.00, 20000,'20万元金额,2万个小红包,每个10元')
聚簇索引和非聚簇索引区别:
- 聚簇索引:将数据存储与索引放到了一块,找到索引也就找到了数据
- 非聚簇索引:将数据存储于索引分开结构,索引结构的叶子节点指向了数据的对应行,myisam通过key_buffer把索引先缓存到内存中,当需要访问数据时(通过索引访问数据),在内存中直接搜索索引,然后通过索引找到磁盘相应数据,这也就是为什么索引不在key buffer命中时,速度慢的原因
2.SSM开发环境搭建(XML)
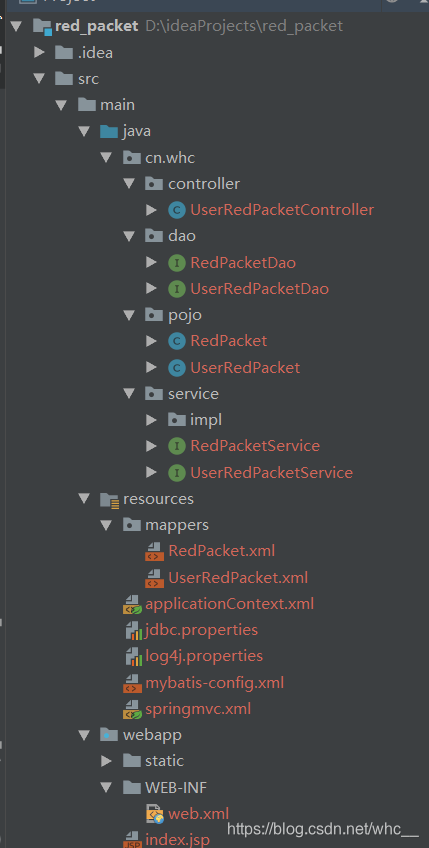
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.whc</groupId>
<artifactId>red_packet</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<spring.version>5.1.10.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<!--spring mvc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<!--spring mvc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<!--事务-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<!--mybatis-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.2</version>
</dependency>
<!--mybatis与spring整合-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.2</version>
</dependency>
<!--mysql驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<!--Druid-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.20</version>
</dependency>
<!--jdbc持久化相关jar包-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${spring.version}</version>
</dependency>
<!--mysql驱动包-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<!--支持json格式转换的相关jar包-->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.8</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.9.8</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.8</version>
</dependency>
</dependencies>
</project>
web.xml
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<!--配置Spring IoC配置文件路径-->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:applicationContext.xml</param-value>
</context-param>
<!--解决发送post请求乱码问题-->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--配置ContextLoaderListener用以初始化Spring IoC容器-->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!--配置DispatcherServlet-->
<servlet>
<servlet-name>dispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath*:springmvc.xml</param-value>
</init-param>
<!--使得Dispatcher在服务器启动的时候就初始化-->
<load-on-startup>2</load-on-startup>
</servlet>
<!--Servlet拦截配置-->
<servlet-mapping>
<servlet-name>dispatcherServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd">
<!--使用注解驱动-->
<context:annotation-config/>
<!--导入jdbc配置信息-->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!--druid数据库连接池-->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${db.driverClassName}"/>
<property name="url" value="${db.url}"/>
<property name="username" value="${db.username}"/>
<property name="password" value="${db.password}"/>
</bean>
<!--集成MyBatis-->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!--数据源-->
<property name="dataSource" ref="dataSource"/>
<!--MyBatis全局配置文件-->
<property name="configLocation" value="classpath:mybatis-config.xml"/>
<!--Mapper映射器配置路径-->
<property name="mapperLocations" value="classpath*:mappers/*.xml"/>
</bean>
<!--配置数据库事务管理器-->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<!--开启基于事务的注解-->
<tx:annotation-driven transaction-manager="transactionManager"/>
<!--采用自动扫描方式创建mapper bean-->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="cn.whc.dao"/>
<property name="annotationClass" value="org.springframework.stereotype.Repository"/>
</bean>
</beans>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!--定义扫描装载的包-->
<context:component-scan base-package="cn.whc"/>
<!-- 设置配置方案 -->
<mvc:annotation-driven>
<!-- 不使用默认的消息转换器 -->
<mvc:message-converters register-defaults="false">
<!--HTTP JSON转换器-->
<bean id="jsonConverter" class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter">
<!--http类型支持为JSON类型-->
<property name="supportedMediaTypes">
<list>
<value>application/json;charset=UTF-8</value>
</list>
</property>
</bean>
</mvc:message-converters>
</mvc:annotation-driven>
</beans>
mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--开启懒加载机制-->
<settings>
<!--全局的映射器启动缓存-->
<setting name="cacheEnabled" value="true"/>
<!--允许JDBC支持生成的键-->
<setting name="useGeneratedKeys" value="true"/>
<!--全局启动延迟加载-->
<setting name="lazyLoadingEnabled" value="true"/>
<!--关闭层级加载-->
<setting name="aggressiveLazyLoading" value="false"/>
<!--设置超时时间,它决定驱动等待一个数据库响应的时间-->
<setting name="defaultStatementTimeout" value="25000"/>
</settings>
</configuration>
jdbc.properties
db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost/red_packet?characterEncoding=utf-8
db.username=root
db.password=root