#include <iostream>
#include<stack>
#include<vector>
#include<string>
#include<stack>
using namespace std;
struct TreeNode
{
char data;
struct TreeNode* left;
struct TreeNode* right;
TreeNode(char x) :data(x), left(nullptr), right(nullptr){}
};
struct TreeNode* BuildTree(const string & datas,int& i)
{
if (i == datas.size() || datas[i] == '#')
{
i++;
return nullptr;
}
TreeNode* root = new TreeNode(datas[(i++)]);
root->left = BuildTree(datas,i);
root->right = BuildTree(datas,i);
return root;
}
string front(TreeNode* point)
{
string result;
struct TreeNode* root = point;
if (!root)
{
return result;
}
stack<TreeNode*>st;
st.push(root);
while ( !(st.empty()))
{
TreeNode* node = st.top();
st.pop();
result += node -> data;
result += ' ';
if (node->right) st.push(node->right);
if (node->left) st.push(node->left);
}
return result;
}
string mid(struct TreeNode* point)
{
string result;
stack<TreeNode*>st;
struct TreeNode* root = point;
while (root != nullptr ||! (st.empty()))
{
while (root != nullptr)
{
st.push(root);
root = root->left;
}
root = st.top();
st.pop();
result += root->data;
result += ' ';
root = root->right;
}
return result;
}
string back(struct TreeNode* root)
{
if (root == nullptr)
{
return "";
}
stack<TreeNode*>s1;
stack<TreeNode*> s2;
string result;
s1.push(root);
while (!s1.empty()) {
TreeNode* node = s1.top();
s1.pop();
s2.push(node);
if (node->left) {
s1.push(node->left);
}
if (node->right) {
s1.push(node->right);
}
}
while (!s2.empty()) {
TreeNode* node = s2.top();
s2.pop();
result += node->data;
result += ' ';
}
return result;
}
int main()
{
string good;
cin >> good;
int i = 0;
cout<<front(BuildTree(good,i));
cout << '\n';
i = 0;
cout << mid(BuildTree(good, i));
cout << '\n';
i = 0;
cout << back(BuildTree(good, i));
cout << '\n';
i = 0;
cout << front(BuildTree(good, i));
cout << '\n';
i = 0;
cout << mid(BuildTree(good, i));
cout << '\n';
i = 0;
cout << back(BuildTree(good, i));
}
二叉树的三种非递归遍历
最新推荐文章于 2024-11-10 21:43:58 发布
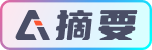