<cc1:MiniButton ID="btnZipDown" Width="35px" Height="25px" runat="server" Plain="true"
ToolTip="打包下载二维码" IconClass="Wexport" OnClick="btnZipDown_Click" Text="打包下载二维码" Visible="false" ConfirmMsg="打包下载二维码可能需要较长的时间才能完成,请耐心等待!" />
/// <summary>
/// 打包下载二维码按钮
/// </summary>
/// 根据当前勾选数据查询二维码照片是否已上传至文件服务器,如果已上传则获取对应二维码照片文件流,如果未上传则先上传至文件服务器后获取对应二维码照片文件流,最后批量压缩文件并弹框选择文件存放路径
/// <param name="sender"></param>
/// <param name="e"></param>
[GridPostBack(GridPostBackMode.CheckedRows, true, "dgList")]
protected void btnZipDown_Click(WuhanIns.Web.MiniUI.MiniButton sender, WuhanIns.Web.MiniUI.Core.AjaxActionEventArgs e)
{
HrStaffBaseItem[] detailItem = e.GetRequestParam<HrStaffBaseItem[]>("dgList");
if (detailItem.Count() <= 0)
{
JSAlert("请至少选择一条记录!");
return;
}
#region 验证数据状态
string strMsg = string.Empty;
string SubContractorName = string.Empty;
string projectid = e.GetRequestParam<string>("Project_Id");
List<SubcontractorBase> baseInfos = HrSubcontractorManager.Find(true);
foreach (HrStaffBaseItem BaseItem in detailItem)
{
if (baseInfos != null && baseInfos.Count() > 0)
{
IEnumerable<SubcontractorBase> entities = baseInfos.Where(x => x.Id == BaseItem.SubcontractorId && x.ProjectId == projectid);
if (entities != null && entities.Count() > 0)
{
SubContractorName = entities.First().SubcontractorName;
}
}
if (string.IsNullOrEmpty(BaseItem.CurState.ToString()))
{
strMsg += "分包商" + SubContractorName + ",人员" + BaseItem.Name + "当前状态为待进场, \n";
}
else if (BaseItem.CurState == EPC人员状态.待进场 || BaseItem.CurState == EPC人员状态.进场审批中 || BaseItem.CurState == EPC人员状态.已退场)
{
strMsg += "分包商" + SubContractorName + ",人员" + BaseItem.Name + "当前状态为" + BaseItem.CurState.ToString() + ", \n";
}
if (BaseItem.IsBlackList == 人员黑名单状态.拉入黑名单审批中)
{
strMsg += "分包商" + SubContractorName + ",人员" + BaseItem.Name + "当前状态为" + BaseItem.IsBlackList.ToString() + ", \n";
}
}
if (!string.IsNullOrEmpty(strMsg))
{
JSAlert(strMsg + "不能执行当前操作!");
return;
}
#endregion
string strAddress = Server.MapPath("../TempFiles/ZipFiles/");
if (Directory.Exists(strAddress)) //如果文件夹存在则删除,初始化
{
Directory.Delete(strAddress, true);//删除指定的目录并(如果指示)删除该目录中的所有子目录和文件。
}
string zipPath = Server.MapPath("../TempFiles/ZipFiles/") + "人员二维码打包下载" + DateTime.Now.ToString("yyyyMMddHHmmss");//压缩文件存放路径
string filePath = string.Empty;
List<string> files = new List<string>();//批量压缩文件路径
string FileType = "HrStaffBase人员二维码";
string FileId = string.Empty;//文件ID
string FileName = string.Empty;//文件名,带后缀
byte[] QRCodeByte = null;
string enCodeString = string.Empty;
StringBuilder strErr = new StringBuilder();
foreach (HrStaffBaseItem item in detailItem)
{
FileInfoListOutput file = client.ListFileInfo(item.Id, FileType).FirstOrDefault();//步骤1,根据ID与FileType获取指定文件
if (file != null)
{
#region 已上传文件服务器
FileId = file != null ? file.FileId : null;
FileName = file != null ? file.FileName : null;
if (!string.IsNullOrEmpty(FileId))
{
#region 步骤2,单个文件生成压缩文件
if (!Directory.Exists(zipPath)) //如果文件夹不存在则创建
{
Directory.CreateDirectory(zipPath);
}
filePath = zipPath + "\\" + FileName;
byte[] fileData = client.DownloadFile(file.FileId).Result;//根据FileId从文件服务器获取文件流
ZipHelper.BytesToFile(fileData, zipPath, filePath);//在临时路径中生成临时文件,用于压缩
#endregion
}
#endregion
}
else
{
#region 生成二维码图片并返回对应二维码图片字节流
enCodeString = item.Id + "&" + item.Name + "&" + item.IdentityCard + "&" + item.Position.ToString() + "&" + item.SubcontractorId + "&" + item.SupplyName;
FileName = item.Name + "&" + item.IdentityCard + ".jpg";
string FileDirPath = Server.MapPath("../TempFiles/QRCode/");
string QRCodefilePath = FileDirPath + FileName;//必须经过这一步操作才能变成有效路径
if (!Directory.Exists(FileDirPath))
{
Directory.CreateDirectory(FileDirPath);
}
QRCodeByte = client.CreateQRByte(enCodeString, ErrorCorrectionLevel.L, ImageFormat.Jpeg, 4, Color.Black, QRCodefilePath);
#endregion
#region 单个文件上传文件服务器,文件流上传
byte[] bytes = QRCodeByte;
string dept_id = UserContext.CurrentUser.AccCompanyId;
if (UserContext.CurrentUser.IsAccCompanyInner && !UserContext.CurrentUser.IsSystemAdmin)//内部单位
{
dept_id = UserContext.CurrentUser.DeptId;
}
Dictionary<string, string> metaData = new Dictionary<string, string>();
metaData.Add("ContentType", "application/octet-stream"); //mime类型
metaData.Add("From", "劳务人员管理");
metaData.Add("busiId", item.Id);
metaData.Add("FileType", FileType);
metaData.Add("ContractId", item.SubcontractorId);
metaData.Add("BusiTitle", item.Name + "&" + item.IdentityCard + "人员二维码");
metaData.Add("UploadDeptId", dept_id);
client.Upload(item.Id, "", FileType, FileName, bytes, metaData);
#endregion
#region 单个文件生成压缩文件
FileInfoListOutput fileInfo = client.ListFileInfo(item.Id, FileType).FirstOrDefault();//步骤1,根据ID与FileType获取指定文件
if (fileInfo != null)
{
if (!Directory.Exists(zipPath)) //如果文件夹不存在则创建
{
Directory.CreateDirectory(zipPath);
}
filePath = zipPath + "\\" + FileName;
byte[] fileData = client.DownloadFile(fileInfo.FileId).Result;//根据FileId从文件服务器获取文件流
ZipHelper.BytesToFile(fileData, zipPath, filePath);//在临时路径中生成临时文件,用于压缩
}
#endregion
}
}
#region 步骤3,打包压缩指定路径文件,并弹框选择下载路径
ICSharpCode.SharpZipLib.Checksum.Crc32 crc = new ICSharpCode.SharpZipLib.Checksum.Crc32();
string zipFilePath = (zipPath + ".zip");
ZipHelper.CreateZip(zipPath, zipFilePath);//根据临时文件夹路径生成打包压缩文件
if (Directory.Exists(zipPath))
Directory.Delete(zipPath, true);//删除临时文件夹及文件
UrlParams urlParam = new UrlParams();
urlParam.AddParameter("FILE_PATH", zipFilePath.Replace("\\", "|"));
string url = urlParam.GetUrl("../DownLoadFile.aspx");//弹框选择下载文件存放路径
this.JSShowWindow(url, true);
#endregion
}
using Gma.QrCodeNet.Encoding;
using System;
using System.Collections.Generic;
using System.Collections.Specialized;
using System.IO;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
using System.Web;
using WuhanIns.Infrastructure.Dtos;
using WuhanIns.Infrastructure.Utils;
namespace InsHR.Business.Util
{
public class FileClient
{
private const string FullTextSearchUrl = "api/file/search";
private const string RemoveByBusiUrl = "api/file/remove/{0}/{1}";
private const string RemoveUrl = "api/file/remove/{0}";
private const string FileInfoUrl = "api/file/fileinfo/{0}";
private const string FileInfoListUrl = "api/file/fileinfos/{0}/{1}";
private const string DownloadAssociatedFileUrl = "api/file/download/{0}/{1}";
private const string DownloadUrl = "api/file/download/{0}";
private const string UploadUrl = "api/file/upload";
private const string UploadChunkUrl = "api/file/UploadChunk";
private const string BeginUploadChunk = "api/file/BeginUploadChunk";
private const string EndUploadChunk = "api/file/EndUploadChunk";
private const string IndexFileUrl = "api/file/IndexFile";
private const string IsExistUrl = "api/Doc/IsExist/{0}/{1}";
private const string ForceCovertDocUrl = "api/Doc/ForceCovertDoc";
private readonly string _serverUrl;
private readonly int _chunkSize;
public FileClient(string serverUrl)
: this(serverUrl, 500 * 1000)
{
}
public FileClient(string serverUrl, int chunkSize)
{
this._chunkSize = chunkSize;
this._serverUrl = serverUrl;
}
public string Upload(string busiId, string sfileCode, string fileType, HttpPostedFile fileData, IDictionary<string, string> metaData)
{
using (HttpClient client = new HttpClient())
{
using (var content = new MultipartFormDataContent())//表明是通过multipart/form-data的方式上传数据
{
NameValueCollection namevals = new NameValueCollection();
namevals.Add("busiId", busiId);
namevals.Add("fileCode", sfileCode);
namevals.Add("fileType", fileType);
namevals.Add("metaData", JsonHelper.Serialize(metaData));
var formDatas = GetFormDataByteArrayContent(namevals);//获取键值集合对应的ByteArrayContent集合
HashSet<HttpPostedFile> fileset = new HashSet<HttpPostedFile>();
fileset.Add(fileData);
var files = GetFileByteArrayContent(fileset);//获取文件集合对应的ByteArrayContent集合
Action<List<ByteArrayContent>> act = (dataContents) =>
{//声明一个委托,该委托的作用就是将ByteArrayContent集合加入到MultipartFormDataContent中
foreach (var byteArrayContent in dataContents)
{
content.Add(byteArrayContent);
}
};
act(formDatas);//执行act
act(files);//执行act
try
{
return WebApiHelper.Post<List<string>>(this._serverUrl, UploadUrl, content).First();
}
catch (Exception ex)
{
throw ex;
}
}
}
}
private List<ByteArrayContent> GetFileByteArrayContent(HashSet<HttpPostedFile> files)
{
List<ByteArrayContent> list = new List<ByteArrayContent>();
foreach (var file in files)
{
byte[] by = new byte[file.ContentLength];
file.InputStream.Read(by, 0, by.Length);
file.InputStream.Close();
var fileContent = new ByteArrayContent(by);
fileContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment")
{
FileName = file.FileName,
};
list.Add(fileContent);
}
return list;
}
public string Upload(string busiId, string sfileCode, string fileType, string fileName, byte[] fileData, IDictionary<string, string> metaData)
{
using (HttpClient client = new HttpClient())
{
using (var content = new MultipartFormDataContent())//表明是通过multipart/form-data的方式上传数据
{
NameValueCollection namevals = new NameValueCollection();
namevals.Add("busiId", busiId);
namevals.Add("fileCode", sfileCode);
namevals.Add("fileType", fileType);
namevals.Add("metaData", JsonHelper.Serialize(metaData));
var formDatas = GetFormDataByteArrayContent(namevals);//获取键值集合对应的ByteArrayContent集合
var files = GetFileByteArrayContent(fileData, fileName);//获取文件集合对应的ByteArrayContent集合
Action<List<ByteArrayContent>> act = (dataContents) =>
{//声明一个委托,该委托的作用就是将ByteArrayContent集合加入到MultipartFormDataContent中
foreach (var byteArrayContent in dataContents)
{
content.Add(byteArrayContent);
}
};
act(formDatas);//执行act
act(files);//执行act
try
{
return WebApiHelper.Post<List<string>>(this._serverUrl, UploadUrl, content).First();
}
catch (Exception ex)
{
throw ex;
}
}
}
}
private List<ByteArrayContent> GetFileByteArrayContent(byte[] Data, string sFileName)
{
List<ByteArrayContent> list = new List<ByteArrayContent>();
var fileContent = new ByteArrayContent(Data);
fileContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment")
{
FileName = sFileName,
};
list.Add(fileContent);
return list;
}
/// <summary>
/// 获取键值集合对应的ByteArrayContent集合
/// </summary>
/// <param name="collection"></param>
/// <returns></returns>
private List<ByteArrayContent> GetFormDataByteArrayContent(NameValueCollection collection)
{
List<ByteArrayContent> list = new List<ByteArrayContent>();
foreach (var key in collection.AllKeys)
{
if (collection[key] != null)
{
var dataContent = new ByteArrayContent(Encoding.UTF8.GetBytes(collection[key]));
dataContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment")
{
Name = key
};
list.Add(dataContent);
}
}
return list;
}
public bool Remove(string fileId)
{
return WebApiHelper.Get<bool>(this._serverUrl, string.Format(RemoveUrl, fileId));
}
public async Task<byte[]> DownloadFile(string fileId)
{
return await WebApiHelper.GetByteArrayAsync(this._serverUrl, string.Format(DownloadUrl, fileId)).ConfigureAwait(false);
}
public async Task<byte[]> DownloadFileByFileType(string fileId, string associatedFileType)
{
return await WebApiHelper.GetByteArrayAsync(this._serverUrl, string.Format(DownloadAssociatedFileUrl, fileId, associatedFileType)).ConfigureAwait(false);
}
public List<FileInfoListOutput> ListFileInfo(string busiId, string sFileType)
{
return WebApiHelper.Get<List<FileInfoListOutput>>(this._serverUrl, string.Format(FileInfoListUrl, busiId, sFileType));
}
public FileInfoListOutput GetFileInfo(string sFileId)
{
return WebApiHelper.Get<FileInfoListOutput>(this._serverUrl, string.Format(FileInfoUrl, sFileId));
}
public FileFullTextSearchOutput FullTextSearch(FullTextSearchInput input)
{
return WebApiHelper.Post<FileFullTextSearchOutput>(this._serverUrl, FullTextSearchUrl, input);
}
public string Upload(string fileName, byte[] fileBytes, IDictionary<string, string> metadata, Action<int> reportProgress)
{
var fileHash = Md5Helper.Hash(fileBytes);
var chunkCount = fileBytes.Count() / this._chunkSize;
if (chunkCount * this._chunkSize < fileBytes.Count())
{
chunkCount++;
}
string fileId = string.Empty;
RetryHelper.Retry(
() =>
{
fileId = WebApiHelper.Post<string>(this._serverUrl, BeginUploadChunk, new { FileHash = fileHash, FileName = fileName, Metadata = metadata });
},
3,
typeof(Exception)
);
if (!string.IsNullOrWhiteSpace(fileId))
{
if (reportProgress != null)
{
reportProgress(100);
}
return fileId;
}
var oldPercent = 0;
for (var index = 0; index < chunkCount; index++)
{
RetryHelper.Retry(
() =>
{
var uploadResult = WebApiHelper.Post<string>(this._serverUrl, UploadChunkUrl, new { ChunkData = fileBytes.Skip(index * this._chunkSize).Take(this._chunkSize).ToArray(), FileHash = fileHash, Index = index });
if (!uploadResult.Contains("上传成功"))
{
throw new Exception(uploadResult);
}
},
3,
typeof(Exception)
);
if (reportProgress != null)
{
var newPercent = ((index * 100) / chunkCount);
if (newPercent > oldPercent && newPercent < 100)
{
reportProgress(newPercent);
oldPercent = newPercent;
}
}
}
RetryHelper.Retry(
() =>
{
fileId = WebApiHelper.Post<string>(this._serverUrl, EndUploadChunk, new { ChunkCount = chunkCount, FileHash = fileHash, FileName = fileName });
},
3,
typeof(Exception)
);
if (reportProgress != null)
{
reportProgress(100);
}
return fileId;
}
public async Task<byte[]> DownloadFile(string fileId, Action<int> reportProgress)
{
long rangeStart = 0;
long rangeEnd = this._chunkSize - 1;
long maxLength = 0;
var oldPercent = 0;
using (var memoryStream = new MemoryStream())
{
while (true)
{
var response = await WebApiHelper.GetResponse(this._serverUrl, string.Format(DownloadUrl, fileId), new Dictionary<string, string> { { "Range", string.Format("bytes={0}-{1}", rangeStart, rangeEnd) } }).ConfigureAwait(false);
if (response.Content.Headers.GetValues("Content-Type").First().Contains("application/json"))
{
var webApiResponse = JsonHelper.Deserialize<WebApiResponse<string>>(response.Content.ReadAsStringAsync().Result);
throw new Exception(webApiResponse.Message);
}
if (maxLength == 0)
{
var contentRange = response.Content.Headers.GetValues("Content-Range").First();
maxLength = long.Parse(contentRange.Split('/')[1]);
}
var responseStream = await response.Content.ReadAsStreamAsync().ConfigureAwait(false);
await responseStream.CopyToAsync(memoryStream).ConfigureAwait(false);
if (rangeEnd >= maxLength)
{
if (reportProgress != null)
{
reportProgress(100);
}
return memoryStream.ToArray();
}
if (reportProgress != null)
{
if (reportProgress != null)
{
var newPercent = Convert.ToInt32(rangeEnd * 100 / maxLength);
if (newPercent > oldPercent && newPercent < 100)
{
reportProgress(newPercent);
oldPercent = newPercent;
}
}
}
rangeStart = rangeEnd + 1;
rangeEnd += this._chunkSize;
}
}
}
public void File2Doc(DocInfoUnput input)
{
try
{
bool bExist = IsExistDoc(input.BusiId, input.FileType);
if (!bExist)
{
string bSave = ForceCovertDoc(input);
}
}
catch (Exception ex)
{
}
}
private bool IsExistDoc(string sBusId, string sFileType)
{
return WebApiHelper.Get<bool>(this._serverUrl, string.Format(IsExistUrl, sBusId, sFileType));
}
private string ForceCovertDoc(DocInfoUnput input)
{
try
{
return WebApiHelper.Post<string>(this._serverUrl, ForceCovertDocUrl, input);
}
catch (Exception ex)
{
throw ex;
}
}
/// <summary>
/// 生成二维码文件流
/// </summary>
/// <param name="content"></param>
/// <param name="level"></param>
/// <param name="imgFormat"></param>
/// <param name="size"></param>
/// <param name="bgColor"></param>
/// <param name="filePath"></param>
/// <returns></returns>
public byte[] CreateQRByte(string content, ErrorCorrectionLevel level, ImageFormat imgFormat, int size, Color bgColor, string filePath)
{
#region
#region 生成二维码,并保存二维码图片
QrEncoder qrEncoder = new QrEncoder(level);
QrCode qrCode = qrEncoder.Encode(content);//生成二维码
System.Drawing.Bitmap image;
image = new Bitmap(qrCode.Matrix.Width * size, qrCode.Matrix.Height * size);
image.SetResolution(180f, 180f);
Graphics gdiobj = Graphics.FromImage(image);
gdiobj.CompositingQuality = CompositingQuality.HighQuality;
gdiobj.SmoothingMode = SmoothingMode.HighQuality;
gdiobj.InterpolationMode = InterpolationMode.HighQualityBicubic;
gdiobj.PixelOffsetMode = PixelOffsetMode.HighQuality;
for (Int32 i = 0; i < qrCode.Matrix.Height; i++)
{
for (Int32 j = 0; j < qrCode.Matrix.Width; j++)
{
if (qrCode.Matrix.InternalArray[i, j])
{
gdiobj.FillRectangle(new SolidBrush(bgColor), i * size, j * size, size, size);
}
}
}
System.Drawing.Imaging.EncoderParameters ep = new System.Drawing.Imaging.EncoderParameters(1);
ep.Param[0] = new System.Drawing.Imaging.EncoderParameter(System.Drawing.Imaging.Encoder.Quality, 800L);
image.Save(filePath);//保存二维码图片
#endregion
#region 根据二维码图片生成字节返回数据
if (File.Exists(filePath))//生成二维码字节返回数据
{
byte[] datas = null;
using (FileStream stream = new FileStream(filePath, FileMode.Open))
{
datas = new byte[stream.Length];
stream.Read(datas, 0, datas.Length);
}
try
{
File.Delete(filePath);//删除当前生成的二维码,初始化
}
catch
{
}
return datas;
}
return null;
#endregion
#endregion
}
}
public class FileInfoListOutput
{
public FileInfoListOutput()
{
}
/// <summary>
/// 文件名称
/// </summary>
public string FileName { set; get; }
/// <summary>
/// 文件ID,文件存储到文件服务器后生成的ID,用于后续操作改文件
/// </summary>
public string FileId { set; get; }
/// <summary>
/// 文件编码,文件的业务编码
/// </summary>
public string FileCode { set; get; }
/// <summary>
/// 业务ID,业务系统中文件管理的业务数据的ID,用于从业务加载对应文件
/// </summary>
public string BusiId { set; get; }
public string FileSize { set; get; }
/// <summary>
/// 文件类型,业务端自定义,可以用于后续查询和其他操作
/// </summary>
public string FileType { set; get; }
/// <summary>
/// 上传时间
/// </summary>
public DateTime UploadDate { get; set; }
public string ExtProperty { set; get; }
public IDictionary<string, string> Metadata { set; get; }
}
public class FullTextSearchInput
{
public string Keywords { get; set; }
public int From { get; set; }
public int Size { get; set; }
public IDictionary<string, string> Metadata { get; set; }
}
public class FileFullTextSearchOutput
{
public IEnumerable<File> Files { get; set; }
public long Count { get; set; }
public class File
{
public string Id { get; set; }
public string FileName { get; set; }
public DateTime UploadDate { get; set; }
public Dictionary<string, List<string>> Highlights { get; set; }
}
}
public class DocInfoUnput
{
public DocInfoUnput()
{
}
/// <summary>
/// 业务ID,业务系统中文件管理的业务数据的ID,用于从业务加载对应文件
/// </summary>
public string BusiId { set; get; }
/// <summary>
/// 文件类型,业务端自定义,可以用于后续查询和其他操作
/// </summary>
public string FileType { set; get; }
/// <summary>
/// 业务编码
/// </summary>
public string BusiCode { set; get; }
/// <summary>
/// 业务明恒
/// </summary>
public string BusiTitle { set; get; }
/// <summary>
/// 合同ID
/// </summary>
public string ContractId { set; get; }
/// <summary>
/// 所属WBS
/// </summary>
public string QWBSId { set; get; }
/// <summary>
/// 上传单位ID
/// </summary>
public string UploadDeptId { set; get; }
/// <summary>
/// 上传单位
/// </summary>
public DateTime UploadDate { set; get; }
/// <summary>
/// 所属模块
/// </summary>
public string FromModule { set; get; }
}
}
using ICSharpCode.SharpZipLib.Checksum;
using ICSharpCode.SharpZipLib.Zip;
using System;
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace InsHR.Business.Util
{
public class ZipHelper
{
/// <summary>
/// 压缩文件
/// </summary>
/// <param name="sourceFilePath"></param>
/// <param name="destinationZipFilePath"></param>
public static void CreateZip(string sourceFilePath, string destinationZipFilePath)
{
if (sourceFilePath[sourceFilePath.Length - 1] != System.IO.Path.DirectorySeparatorChar)
sourceFilePath += System.IO.Path.DirectorySeparatorChar;
ZipOutputStream zipStream = new ZipOutputStream(File.Create(destinationZipFilePath));
zipStream.SetLevel(0); // 压缩级别 0-9
CreateZipFiles(sourceFilePath, zipStream, sourceFilePath);
zipStream.Finish();
zipStream.Close();
}
/// <summary>
/// 递归压缩文件
/// </summary>
/// <param name="sourceFilePath">待压缩的文件或文件夹路径</param>
/// <param name="zipStream">打包结果的zip文件路径(类似 D:\WorkSpace\a.zip),全路径包括文件名和.zip扩展名</param>
/// <param name="staticFile"></param>
private static void CreateZipFiles(string sourceFilePath, ZipOutputStream zipStream, string staticFile)
{
Crc32 crc = new Crc32();
string[] filesArray = Directory.GetFileSystemEntries(sourceFilePath);
foreach (string file in filesArray)
{
if (Directory.Exists(file)) //如果当前是文件夹,递归
{
CreateZipFiles(file, zipStream, staticFile);
}
else //如果是文件,开始压缩
{
FileStream fileStream = File.OpenRead(file);
byte[] buffer = new byte[fileStream.Length];
fileStream.Read(buffer, 0, buffer.Length);
string tempFile = file.Substring(staticFile.LastIndexOf("\\") + 1);
ZipEntry entry = new ZipEntry(tempFile);
entry.DateTime = DateTime.Now;
entry.Size = fileStream.Length;
fileStream.Close();
crc.Reset();
crc.Update(buffer);
entry.Crc = crc.Value;
zipStream.PutNextEntry(entry);
zipStream.Write(buffer, 0, buffer.Length);
}
}
}
/// <summary>
/// 压缩文件夹
/// </summary>
/// <param name="dirToZip"></param>
/// <param name="zipedFileName"></param>
/// <param name="compressionLevel">压缩率0(无压缩)9(压缩率最高)</param>
public static void ZipDir(string dirToZip, string zipedFileName, int compressionLevel = 4)
{
if (Path.GetExtension(zipedFileName) != ".zip")
{
zipedFileName = zipedFileName + ".zip";
}
using (var zipoutputstream = new ZipOutputStream(File.Create(zipedFileName)))
{
zipoutputstream.SetLevel(compressionLevel);
Crc32 crc = new Crc32();
Hashtable fileList = GetAllFies(dirToZip);
foreach (DictionaryEntry item in fileList)
{
FileStream fs = new FileStream(item.Key.ToString(), FileMode.Open, FileAccess.Read, FileShare.ReadWrite);
byte[] buffer = new byte[fs.Length];
fs.Read(buffer, 0, buffer.Length);
// ZipEntry entry = new ZipEntry(item.Key.ToString().Substring(dirToZip.Length + 1));
ZipEntry entry = new ZipEntry(Path.GetFileName(item.Key.ToString()))
{
DateTime = (DateTime)item.Value,
Size = fs.Length
};
fs.Close();
crc.Reset();
crc.Update(buffer);
entry.Crc = crc.Value;
zipoutputstream.PutNextEntry(entry);
zipoutputstream.Write(buffer, 0, buffer.Length);
}
}
}
/// <summary>
/// 获取所有文件
/// </summary>
/// <returns></returns>
public static Hashtable GetAllFies(string dir)
{
Hashtable filesList = new Hashtable();
DirectoryInfo fileDire = new DirectoryInfo(dir);
if (!fileDire.Exists)
{
throw new FileNotFoundException("目录:" + fileDire.FullName + "没有找到!");
}
GetAllDirFiles(fileDire, filesList);
GetAllDirsFiles(fileDire.GetDirectories(), filesList);
return filesList;
}
/// <summary>
/// 获取一个文件夹下的所有文件夹里的文件
/// </summary>
/// <param name="dirs"></param>
/// <param name="filesList"></param>
public static void GetAllDirsFiles(IEnumerable<DirectoryInfo> dirs, Hashtable filesList)
{
foreach (DirectoryInfo dir in dirs)
{
foreach (FileInfo file in dir.GetFiles("*.*"))
{
filesList.Add(file.FullName, file.LastWriteTime);
}
GetAllDirsFiles(dir.GetDirectories(), filesList);
}
}
/// <summary>
/// 获取一个文件夹下的文件
/// </summary>
/// <param name="dir">目录名称</param>
/// <param name="filesList">文件列表HastTable</param>
public static void GetAllDirFiles(DirectoryInfo dir, Hashtable filesList)
{
foreach (FileInfo file in dir.GetFiles("*.*"))
{
filesList.Add(file.FullName, file.LastWriteTime);
}
}
/// <summary>
/// 功能:解压zip格式的文件。
/// </summary>
/// <param name="zipFilePath">压缩文件路径</param>
/// <param name="unZipDir">解压文件存放路径,为空时默认与压缩文件同一级目录下,跟压缩文件同名的文件夹</param>
/// <returns>解压是否成功</returns>
public static void UnZip(string zipFilePath, string unZipDir)
{
if (zipFilePath == string.Empty)
{
throw new Exception("压缩文件不能为空!");
}
if (!File.Exists(zipFilePath))
{
throw new FileNotFoundException("压缩文件不存在!");
}
//解压文件夹为空时默认与压缩文件同一级目录下,跟压缩文件同名的文件夹
if (unZipDir == string.Empty)
unZipDir = zipFilePath.Replace(Path.GetFileName(zipFilePath), Path.GetFileNameWithoutExtension(zipFilePath));
if (!unZipDir.EndsWith("/"))
unZipDir += "/";
if (!Directory.Exists(unZipDir))
Directory.CreateDirectory(unZipDir);
using (var s = new ZipInputStream(File.OpenRead(zipFilePath)))
{
ZipEntry theEntry;
while ((theEntry = s.GetNextEntry()) != null)
{
string directoryName = Path.GetDirectoryName(theEntry.Name);
string fileName = Path.GetFileName(theEntry.Name);
if (!string.IsNullOrEmpty(directoryName))
{
Directory.CreateDirectory(unZipDir + directoryName);
}
if (directoryName != null && !directoryName.EndsWith("/"))
{
}
if (fileName != String.Empty)
{
using (FileStream streamWriter = File.Create(unZipDir + theEntry.Name))
{
int size;
byte[] data = new byte[2048];
while (true)
{
size = s.Read(data, 0, data.Length);
if (size > 0)
{
streamWriter.Write(data, 0, size);
}
else
{
break;
}
}
}
}
}
}
}
/// <summary>
/// 压缩单个文件
/// </summary>
/// <param name="filePath">被压缩的文件名称(包含文件路径),文件的全路径</param>
/// <param name="zipedFileName">压缩后的文件名称(包含文件路径),保存的文件名称</param>
/// <param name="compressionLevel">压缩率0(无压缩)到 9(压缩率最高)</param>
public static void ZipFile(string filePath, string zipedFileName, int compressionLevel = 9)
{
// 如果文件没有找到,则报错
if (!File.Exists(filePath))
{
throw new FileNotFoundException("文件:" + filePath + "没有找到!");
}
// 如果压缩后名字为空就默认使用源文件名称作为压缩文件名称
if (string.IsNullOrEmpty(zipedFileName))
{
string oldValue = Path.GetFileName(filePath);
if (oldValue != null)
{
zipedFileName = filePath.Replace(oldValue, "") + Path.GetFileNameWithoutExtension(filePath) + ".zip";
}
}
// 如果压缩后的文件名称后缀名不是zip,就是加上zip,防止是一个乱码文件
if (Path.GetExtension(zipedFileName) != ".zip")
{
zipedFileName = zipedFileName + ".zip";
}
// 如果指定位置目录不存在,创建该目录 C:\Users\yhl\Desktop\大汉三通
string zipedDir = zipedFileName.Substring(0, zipedFileName.LastIndexOf("\\", StringComparison.Ordinal));
if (!Directory.Exists(zipedDir))
{
Directory.CreateDirectory(zipedDir);
}
// 被压缩文件名称
string filename = filePath.Substring(filePath.LastIndexOf("\\", StringComparison.Ordinal) + 1);
var streamToZip = new FileStream(filePath, FileMode.Open, FileAccess.Read);
var zipFile = File.Create(zipedFileName);
var zipStream = new ZipOutputStream(zipFile);
var zipEntry = new ZipEntry(filename);
zipStream.PutNextEntry(zipEntry);
zipStream.SetLevel(compressionLevel);
var buffer = new byte[2048];
Int32 size = streamToZip.Read(buffer, 0, buffer.Length);
zipStream.Write(buffer, 0, size);
try
{
while (size < streamToZip.Length)
{
int sizeRead = streamToZip.Read(buffer, 0, buffer.Length);
zipStream.Write(buffer, 0, sizeRead);
size += sizeRead;
}
}
finally
{
zipStream.Finish();
zipStream.Close();
streamToZip.Close();
}
}
/// <summary>
/// 压缩单个文件
/// </summary>
/// <param name="fileToZip">要进行压缩的文件名,全路径</param>
/// <param name="zipedFile">压缩后生成的压缩文件名,全路径</param>
public void ZipFile(string fileToZip, string zipedFile)
{
// 如果文件没有找到,则报错
if (!File.Exists(fileToZip))
{
throw new FileNotFoundException("指定要压缩的文件: " + fileToZip + " 不存在!");
}
using (FileStream fileStream = File.OpenRead(fileToZip))
{
byte[] buffer = new byte[fileStream.Length];
fileStream.Read(buffer, 0, buffer.Length);
fileStream.Close();
using (FileStream zipFile = File.Create(zipedFile))
{
using (ZipOutputStream zipOutputStream = new ZipOutputStream(zipFile))
{
// string fileName = fileToZip.Substring(fileToZip.LastIndexOf("\\") + 1);
string fileName = Path.GetFileName(fileToZip);
var zipEntry = new ZipEntry(fileName)
{
DateTime = DateTime.Now,
IsUnicodeText = true
};
zipOutputStream.PutNextEntry(zipEntry);
zipOutputStream.SetLevel(5);
zipOutputStream.Write(buffer, 0, buffer.Length);
zipOutputStream.Finish();
zipOutputStream.Close();
}
}
}
}
/// <summary>
/// 压缩多个目录或文件
/// </summary>
/// <param name="folderOrFileList">待压缩的文件夹或者文件,全路径格式,是一个集合</param>
/// <param name="zipedFile">压缩后的文件名,全路径格式</param>
/// <param name="password">压宿密码</param>
/// <returns></returns>
public static bool ZipManyFilesOrDictorys(IEnumerable<string> folderOrFileList, string zipedFile, string password)
{
bool res = true;
using (var s = new ZipOutputStream(File.Create(zipedFile)))
{
s.SetLevel(6);
if (!string.IsNullOrEmpty(password))
{
s.Password = password;
}
foreach (string fileOrDir in folderOrFileList)
{
//是文件夹
if (Directory.Exists(fileOrDir))
{
res = ZipFileDictory(fileOrDir, s, "");
}
else
{
//文件
res = ZipFileWithStream(fileOrDir, s);
}
}
s.Finish();
s.Close();
return res;
}
}
/// <summary>
/// 带压缩流压缩单个文件
/// </summary>
/// <param name="fileToZip">要进行压缩的文件名</param>
/// <param name="zipStream"></param>
/// <returns></returns>
private static bool ZipFileWithStream(string fileToZip, ZipOutputStream zipStream)
{
//如果文件没有找到,则报错
if (!File.Exists(fileToZip))
{
throw new FileNotFoundException("指定要压缩的文件: " + fileToZip + " 不存在!");
}
//FileStream fs = null;
FileStream zipFile = null;
ZipEntry zipEntry = null;
bool res = true;
try
{
zipFile = File.OpenRead(fileToZip);
byte[] buffer = new byte[zipFile.Length];
zipFile.Read(buffer, 0, buffer.Length);
zipFile.Close();
zipEntry = new ZipEntry(Path.GetFileName(fileToZip));
zipStream.PutNextEntry(zipEntry);
zipStream.Write(buffer, 0, buffer.Length);
}
catch
{
res = false;
}
finally
{
if (zipEntry != null)
{
}
if (zipFile != null)
{
zipFile.Close();
}
GC.Collect();
GC.Collect(1);
}
return res;
}
/// <summary>
/// 递归压缩文件夹方法
/// </summary>
/// <param name="folderToZip"></param>
/// <param name="s"></param>
/// <param name="parentFolderName"></param>
private static bool ZipFileDictory(string folderToZip, ZipOutputStream s, string parentFolderName)
{
bool res = true;
ZipEntry entry = null;
FileStream fs = null;
Crc32 crc = new Crc32();
try
{
//创建当前文件夹
entry = new ZipEntry(Path.Combine(parentFolderName, Path.GetFileName(folderToZip) + "/")); //加上 “/” 才会当成是文件夹创建
s.PutNextEntry(entry);
s.Flush();
//先压缩文件,再递归压缩文件夹
var filenames = Directory.GetFiles(folderToZip);
foreach (string file in filenames)
{
//打开压缩文件
fs = File.OpenRead(file);
byte[] buffer = new byte[fs.Length];
fs.Read(buffer, 0, buffer.Length);
entry = new ZipEntry(Path.Combine(parentFolderName, Path.GetFileName(folderToZip) + "/" + Path.GetFileName(file)));
entry.DateTime = DateTime.Now;
entry.Size = fs.Length;
fs.Close();
crc.Reset();
crc.Update(buffer);
entry.Crc = crc.Value;
s.PutNextEntry(entry);
s.Write(buffer, 0, buffer.Length);
}
}
catch
{
res = false;
}
finally
{
if (fs != null)
{
fs.Close();
}
if (entry != null)
{
}
GC.Collect();
GC.Collect(1);
}
var folders = Directory.GetDirectories(folderToZip);
foreach (string folder in folders)
{
if (!ZipFileDictory(folder, s, Path.Combine(parentFolderName, Path.GetFileName(folderToZip))))
{
return false;
}
}
return res;
}
public static void BytesToFile(byte[] bytes, string filePath, string filename)
{
//生成解压目录
Directory.CreateDirectory(filePath);
using (MemoryStream ms = new MemoryStream(bytes))
{
using (FileStream fs = new FileStream(filename, FileMode.Create, FileAccess.Write))
{
ms.WriteTo(fs);
}
}
}
}
}
using InsSysLib.Core.Util;
using System;
using System.IO;
using System.Web;
namespace InsHR.WebUI
{
public partial class DownLoadFile : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
string filepath = UrlParams.GetParam("FILE_PATH");
string filePath = filepath.Replace("|", "\\");
string filename = Path.GetFileName(filePath);
FileStream iStream = null;
try
{
iStream = new System.IO.FileStream(filePath, System.IO.FileMode.Open, System.IO.FileAccess.Read, System.IO.FileShare.Read);
long dataToRead;
int length;
byte[] buffer = new Byte[100000000];
dataToRead = iStream.Length;
Response.Clear();
Response.ClearHeaders();
Response.ClearContent();
Response.ContentType = "application/octet-stream";
Response.AddHeader("Content-Disposition", "attachment; filename=" + HttpUtility.UrlEncode(filename, System.Text.Encoding.UTF8)); //下载的时候下载原来的文件名称
while (dataToRead > 0)
{
if (Response.IsClientConnected)
{
length = iStream.Read(buffer, 0, 100000000);
Response.OutputStream.Write(buffer, 0, length);
Response.Flush();
dataToRead = dataToRead - length;
}
else
{
dataToRead = -1;
}
}
//POIExcelUtils.DeleteDir(filePath);
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
finally
{
if (iStream != null)
{
iStream.Close();
if (File.Exists(filePath))
{
//File.Delete(filePath);//删除临时打包压缩文件
POIExcelUtils.DeleteDir(filePath);//删除指定目录下的所有文件及其文件夹
}
}
Response.End();
}
}
}
}
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="DownLoadFile.aspx.cs" Inherits="InsHR.WebUI.DownLoadFile" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
</div>
</form>
</body>
</html>

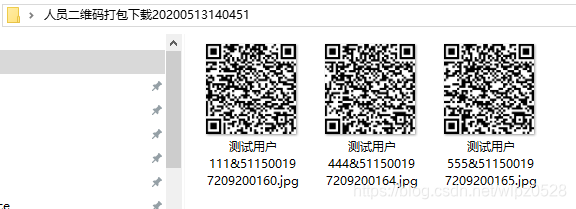