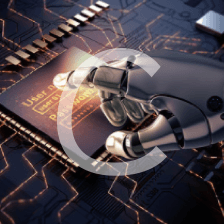
算法实践
基础算法实践
timeshark
猫头鹰是我的好朋友~
展开
-
80. Remove Duplicates from Sorted Array II
Follow up for "Remove Duplicates":What if duplicates are allowed at most twice?For example,Given sorted array nums = [1,1,1,2,2,3],Your function should return length = 5, with the first fi原创 2016-01-19 14:58:30 · 306 阅读 · 0 评论 -
95. Unique Binary Search Trees II
Given n, generate all structurally unique BST's (binary search trees) that store values 1...n.For example,Given n = 3, your program should return all 5 unique BST's shown below. 1 3原创 2016-01-19 14:01:19 · 348 阅读 · 0 评论 -
105. Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree.Note:You may assume that duplicates do not exist in the tree.根据先序序列和中序序列构建改二叉树思路:根据先序序列确定树的根值,在中续序列中根值左侧的序列的为左原创 2016-01-10 22:14:44 · 346 阅读 · 0 评论 -
104. Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.题意:求二叉树的树高。思路:递归求解。/** *原创 2016-03-15 21:26:40 · 248 阅读 · 0 评论 -
107. Binary Tree Level Order Traversal II
Given a binary tree, return the bottom-up level order traversal of its nodes' values. (ie, from left to right, level by level from leaf to root).For example:Given binary tree {3,9,20,#,#,15,7},原创 2016-03-15 23:45:58 · 288 阅读 · 0 评论 -
110. Balanced Binary Tree
Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never diffe原创 2016-03-16 21:32:43 · 205 阅读 · 0 评论 -
2. Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2016-03-29 14:52:40 · 179 阅读 · 0 评论 -
3. Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for "abcabcbb" is "abc", which the length is 3. Fo原创 2016-03-29 23:41:51 · 261 阅读 · 0 评论 -
81. Search in Rotated Sorted Array II
Follow up for "Search in Rotated Sorted Array":What if duplicates are allowed?Would this affect the run-time complexity? How and why?Write a function to determine if a given target is in the原创 2016-02-19 20:48:55 · 330 阅读 · 0 评论 -
16. 3Sum Closest
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exact原创 2016-02-21 21:58:16 · 301 阅读 · 0 评论 -
15. 3Sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triplet (a,b,c原创 2016-02-21 22:18:30 · 300 阅读 · 0 评论 -
111. Minimum Depth of Binary Tree
Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.题意:求树的最短高度(由树根到叶子的最短的距离)。思路:层序原创 2016-03-18 23:14:51 · 215 阅读 · 0 评论 -
112. Path Sum
Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.For example:Given the below binary tree and sum原创 2016-03-18 23:38:41 · 216 阅读 · 0 评论 -
113. Path Sum II
Given a binary tree and a sum, find all root-to-leaf paths where each path's sum equals the given sum.For example:Given the below binary tree and sum = 22, 5 / \原创 2016-03-19 00:25:27 · 219 阅读 · 0 评论 -
118. Pascal's Triangle
Given numRows, generate the first numRows of Pascal's triangle.For example, given numRows = 5,Return[ [1], [1,1], [1,2,1], [1,3,3,1], [1,4,6,4,1]]题意:给定一个数值,返回该数量下的杨辉三角。思路:逐原创 2016-03-19 08:20:20 · 181 阅读 · 0 评论 -
119. Pascal's Triangle II
Given an index k, return the kth row of the Pascal's triangle.For example, given k = 3,Return [1,3,3,1].Note:Could you optimize your algorithm to use only O(k) extra space?题意:返回杨辉三角的第k原创 2016-03-19 09:25:16 · 168 阅读 · 0 评论 -
125. Valid Palindrome
Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.For example,"A man, a plan, a canal: Panama" is a palindrome."race a car" is not a原创 2016-03-19 20:06:25 · 201 阅读 · 0 评论 -
9. Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.click to show spoilers.Some hints:Could negative integers be palindromes? (ie, -1)If you are thinking of convertin原创 2016-03-08 14:19:46 · 203 阅读 · 0 评论 -
5. Longest Palindromic Substring
Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.题意:找一个字符串的最大回文子字符串原创 2016-04-01 01:05:57 · 205 阅读 · 0 评论 -
155. Min Stack
Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.push(x) -- Push element x onto stack.pop() -- Removes the element on top of the stack.top() -- Get原创 2016-03-20 16:19:49 · 227 阅读 · 0 评论 -
160. Intersection of Two Linked Lists
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists:A: a1 → a2 ↘原创 2016-03-20 17:05:29 · 252 阅读 · 0 评论 -
165. Compare Version Numbers
Compare two version numbers version1 and version2.If version1 > version2 return 1, if version1 version2 return -1, otherwise return 0.You may assume that the version strings are non-empty and co原创 2016-03-21 00:17:58 · 279 阅读 · 0 评论 -
168. Excel Sheet Column Title
Given a positive integer, return its corresponding column title as appear in an Excel sheet.For example: 1 -> A 2 -> B 3 -> C ... 26 -> Z 27 -> AA 28 -> AB 题意:26个英文字原创 2016-03-21 08:39:50 · 206 阅读 · 0 评论 -
14. Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.题意:找出多个字符串的公共前缀。思路:从最小的字符串开始,然后一一比较求出最长的公共前缀。class Solution {public: string longestCommonPrefix(vector& st原创 2016-03-09 14:37:11 · 277 阅读 · 0 评论 -
13. Roman to Integer
Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.题意:罗马数字转换为整数。思路:存储一下罗马基本字母,搜索一下罗马字构成规则,然后进行转换。注意:默认题目给出的是正确的字符串,所以不用其他检验了,省了很多事。原创 2016-03-09 00:15:06 · 317 阅读 · 0 评论 -
171. Excel Sheet Column Number
Related to question Excel Sheet Column TitleGiven a column title as appear in an Excel sheet, return its corresponding column number.For example: A -> 1 B -> 2 C -> 3 ...原创 2016-03-21 14:23:24 · 190 阅读 · 0 评论 -
169. Majority Element
Given an array of size n, find the majority element. The majority element is the element that appears more than ⌊ n/2 ⌋ times.You may assume that the array is non-empty and the majority element原创 2016-03-21 14:32:12 · 213 阅读 · 0 评论 -
19. Remove Nth Node From End of List
Given a linked list, remove the nth node from the end of list and return its head.For example, Given linked list: 1->2->3->4->5, and n = 2. After removing the second node from the end, the原创 2016-03-09 18:06:00 · 198 阅读 · 0 评论 -
20. Valid Parentheses
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.The brackets must close in the correct order, "()" and "()[]{}" are all va原创 2016-03-09 18:36:39 · 194 阅读 · 0 评论 -
21. Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.题意:合并两个有序链表。思路:两个指针边比较边移动。class Solution {public:原创 2016-03-09 22:05:50 · 184 阅读 · 0 评论 -
27. Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.题原创 2016-03-09 23:55:30 · 212 阅读 · 0 评论 -
229. Majority Element II
Given an integer array of size n, find all elements that appear more than ⌊ n/3 ⌋ times. The algorithm should run in linear time and in O(1) space.Hint:How many majority elements could i原创 2016-03-21 22:06:45 · 196 阅读 · 0 评论 -
38. Count and Say
The count-and-say sequence is the sequence of integers beginning as follows:1, 11, 21, 1211, 111221, ...1 is read off as "one 1" or 11.11 is read off as "two 1s" or 21.21 is read off as原创 2016-03-10 14:41:12 · 190 阅读 · 0 评论 -
11. Container With Most Water
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Fin原创 2016-04-03 17:10:01 · 228 阅读 · 0 评论 -
172. Factorial Trailing Zeroes
Given an integer n, return the number of trailing zeroes in n!.Note: Your solution should be in logarithmic time complexity.题意:求n的阶乘结果里会有多少个0思路:这个问题跟5有关,找跟5有关的个数。class Solution {publi原创 2016-03-21 23:35:05 · 200 阅读 · 0 评论 -
189. Rotate Array
Rotate an array of n elements to the right by k steps.For example, with n = 7 and k = 3, the array [1,2,3,4,5,6,7] is rotated to [5,6,7,1,2,3,4].Note:Try to come up as many solutions as yo原创 2016-03-21 23:59:45 · 211 阅读 · 0 评论 -
190. Reverse Bits
Reverse bits of a given 32 bits unsigned integer.For example, given input 43261596 (represented in binary as 00000010100101000001111010011100), return 964176192 (represented in binary as0011100101原创 2016-03-22 09:49:58 · 189 阅读 · 0 评论 -
191. Number of 1 Bits
Write a function that takes an unsigned integer and returns the number of ’1' bits it has (also known as the Hamming weight).For example, the 32-bit integer ’11' has binary representation 000000原创 2016-03-22 10:53:08 · 210 阅读 · 0 评论 -
12. Integer to Roman
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.题意:整数转为罗马序列。思路:把1000,900,500,400,100,90,50,40,10,9,5,4,1作为基数,然后进行除法和取余运算。class原创 2016-04-03 22:21:17 · 239 阅读 · 0 评论 -
17. Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit st原创 2016-04-03 23:03:39 · 207 阅读 · 0 评论