概述
- 定义:使用原型实例指定创建对象的种类,并且通过拷贝这些原型创建新的对象。
- 原型模式是一种对象创建型模式。
- 实现:需要实现Cloneable接口;
- 核心:clone()方法。通过clone()方式,返回对应的对象;clone()方法不会执行类的构造方法;ShadowCopy & DeepCopy
- 学习难度:★★★☆☆
- 使用频率:★★★☆☆
优缺点
- 优点
- 使用clone 能够动态的抽取当前对象运行时的状态并且克隆到新的对象中,新对象就可以在此基础上进行操作而不损坏原有对象。
- 缺点
- 就是每个原型必须含有clone 方法,由于clone方法在java 实现中有着一定的弊端和风险,所以clone 方法是不建议使用的。
类图
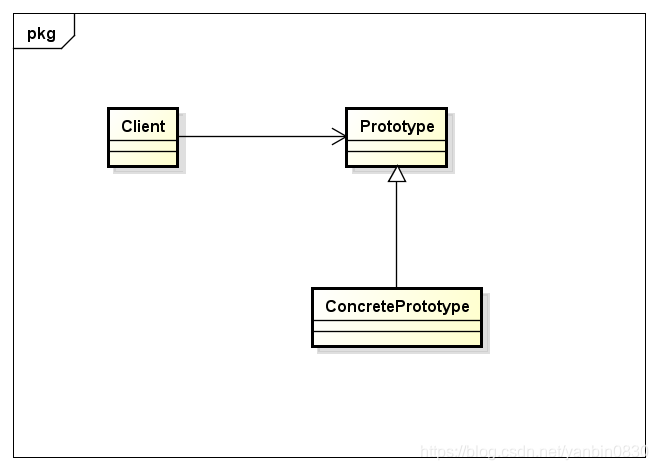
组成角色
- 抽象原型角色(Prototype)
- 具体原型角色(ConcretePrototype)
- 客户角色(Client)
- 原型管理器(可选)(PrototypeManager)
Code Example
抽象原型角色(Prototype)
public class Prototype implements Cloneable {
@Override
public Object clone() {
Prototype p = null;
try {
p = (Prototype) super.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return p;
}
}
具体原型角色(ConcretePrototype)
public class ConcretePrototype extends Prototype {
public ConcretePrototype() {
System.out.println("具体原型角色!");
}
}
客户角色(Client)
public class Client {
public void test() {
ConcretePrototype concretePrototype = new ConcretePrototype();
Prototype prototype = (Prototype) concretePrototype.clone();
System.out.println(concretePrototype);
System.out.println(prototype);
Prototype p = PrototypeManager.getManager().getPrototype("prototype.ConcretePrototype");
Prototype pClone = PrototypeManager.getManager().getPrototype("prototype.ConcretePrototype");
System.out.println(p);
System.out.println(pClone);
}
}
原型管理器(可选)(PrototypeManager)
public class PrototypeManager {
private static PrototypeManager pm;
private Map<String, Object> prototypes = null;
private PrototypeManager() {
prototypes = new HashMap<String, Object>();
}
public static PrototypeManager getManager() {
if (pm == null) {
pm = new PrototypeManager();
}
return pm;
}
public void add(String name, Object prototype) {
prototypes.put(name, prototype);
}
public void delete(String name) {
prototypes.remove(name);
}
public Prototype getPrototype(String name) {
Prototype object = null;
if (prototypes.containsKey(name)) {
Prototype p = (Prototype) prototypes.get(name);
object = (Prototype) p.clone();
} else {
try {
object = (Prototype) Class.forName(name).newInstance();
add(name, object);
} catch (Exception e) {
System.err.println("Class " + name + "没有定义!");
}
}
return object;
}
}
客户端
public class PrototypePatten {
public static void main(String[] args) {
Client client = new Client();
client.test();
}
}