1、二叉树递归创建 ,二叉树先中后序遍历,二叉树计算节点,二叉树计算深度
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef char datatype;
//定义节点结构体
typedef struct Node
{
//数据域
datatype data;
//指针域:存储左子孩子节点地址
struct Node *lchild;
//指针域:存储右子孩子节点地址
struct Node *rchild;
}*Btree;
//创建节点
Btree create_node()
{
Btree s=(Btree)malloc(sizeof(struct Node));
if(s==NULL)
return NULL;
//初始化
s->data='\0';
s->lchild=s->rchild=NULL;
return s;
}
/*
* function: 创建二叉树
* @param [ in]
* @param [out]
* @return 返回二叉树
*/
Btree create_tree()
{
datatype element;
printf("please enter element:");
scanf(" %c",&element);
if(element=='#')
return NULL;
//创建节点
Btree tree=create_node();
tree->data=element;
//递归实现循环左孩子
puts("左");
tree->lchild=create_tree();
//递归实现循环右孩子
puts("右");
tree->rchild=create_tree();
return tree;
}
/*
* function: 先序遍历
* @param [ in]
* @param [out] tree
* @return
*/
void first(Btree tree)
{
if(NULL==tree)
return ;
//遍历根节点
printf("%c",tree->data);
//递归遍历左孩子
first(tree->lchild);
//递归遍历右孩子
first(tree->rchild);
}
//中序遍历
void mid(Btree tree)
{
if(NULL==tree)
return ;
//递归遍历左孩子
mid(tree->lchild);
//遍历根节点
printf("%c",tree->data);
//递归遍历右孩子
mid(tree->rchild);
}
//后序遍历
void rear(Btree tree)
{
if(NULL==tree)
return ;
//递归遍历左孩子
rear(tree->lchild);
//递归遍历右孩子
rear(tree->rchild);
//遍历根节点
printf("%c",tree->data);
}
/*
* function: 计算 度
* @param [ in]
* @param [out]
* @return
*/
void count(Btree tree,int *n0,int *n1,int *n2)
{
if(NULL==tree)
return ;
if(!tree->lchild&&!tree->rchild)
++*n0;
else if(tree->lchild&&tree->rchild)
++*n2;
else
++*n1;
//递归遍历左孩子
count(tree->lchild,n0,n1,n2);
//递归遍历右孩子
count(tree->rchild,n0,n1,n2);
}
/*
* function: 计算深度
* @param [ in]
* @param [out]
* @return 深度
*/
int high(Btree tree)
{
if(NULL==tree)
return 0;
//递归计算左子树的深度
int left=1+high(tree->lchild);
//递归计算右子树的深度
int right=1+high(tree->rchild);
return left>right?left:right;
}
int main(int argc, const char *argv[])
{
Btree tree=create_tree();
//先序遍历
// first(tree);
// puts("");
//中序遍历
mid(tree);
puts("");
//后序遍历
rear(tree);
puts("");
int n0=0,n1=0,n2=0;
//计算各个度的个数
count(tree,&n0,&n1,&n2);
printf("no=%d n1=%d n2=%d n=%d\n",n0,n1,n2,n0+n1+n2);
int len=high(tree);
printf("len=%d\n",len);
return 0;
}
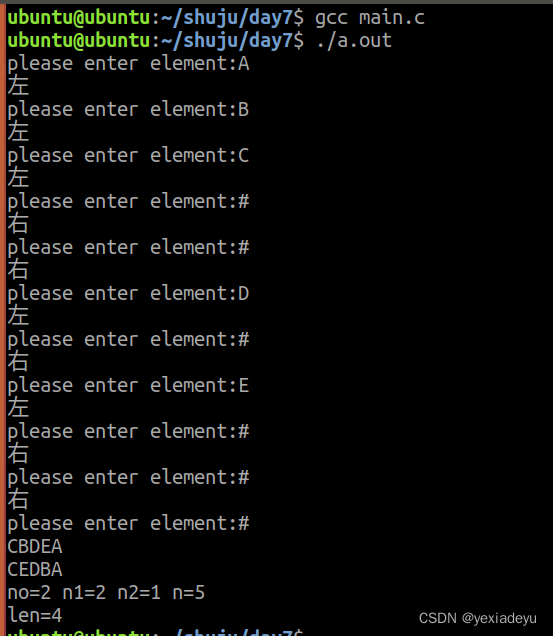
2、2、编程实现快速排序降序
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
//一次排序
//返回基准值下表
int one_sort(int *p,int low,int high)
{
//确定基准值
int key=*(p+low);
//当low==high 结束
//循环low<high
while(low<high)
{
while(low<high&&key>=*(p+high))
high--;
*(p+low)=*(p+high);
//从low开始比较
while(low<high&&key<=*(p+low))
low++;
*(p+high)=*(p+low);
}
*(p+low)=key;
return low;
}
//快速排序
void quick_sort(int *p,int low,int high)
{
//如果只有一个元素或或没有元素
if(low>=high)
return ;
//一次排序
int mid=one_sort(p,low,high);
//递归左边子序列
quick_sort(p,low,mid-1);
//递归右边子序列
quick_sort(p,mid+1,high);
}
int main(int argc, const char *argv[])
{
int arr[]={12,3,45,23,345,2,56,34,7};
int len=sizeof(arr)/sizeof(arr[0]);
quick_sort(arr,0,len-1);
for(int i=0;i<len;i++)
{
printf("%d ",arr[i]);
}
puts("");
return 0;
}
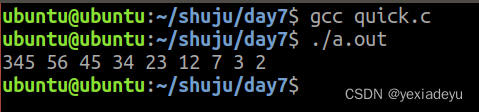
3、思维导图
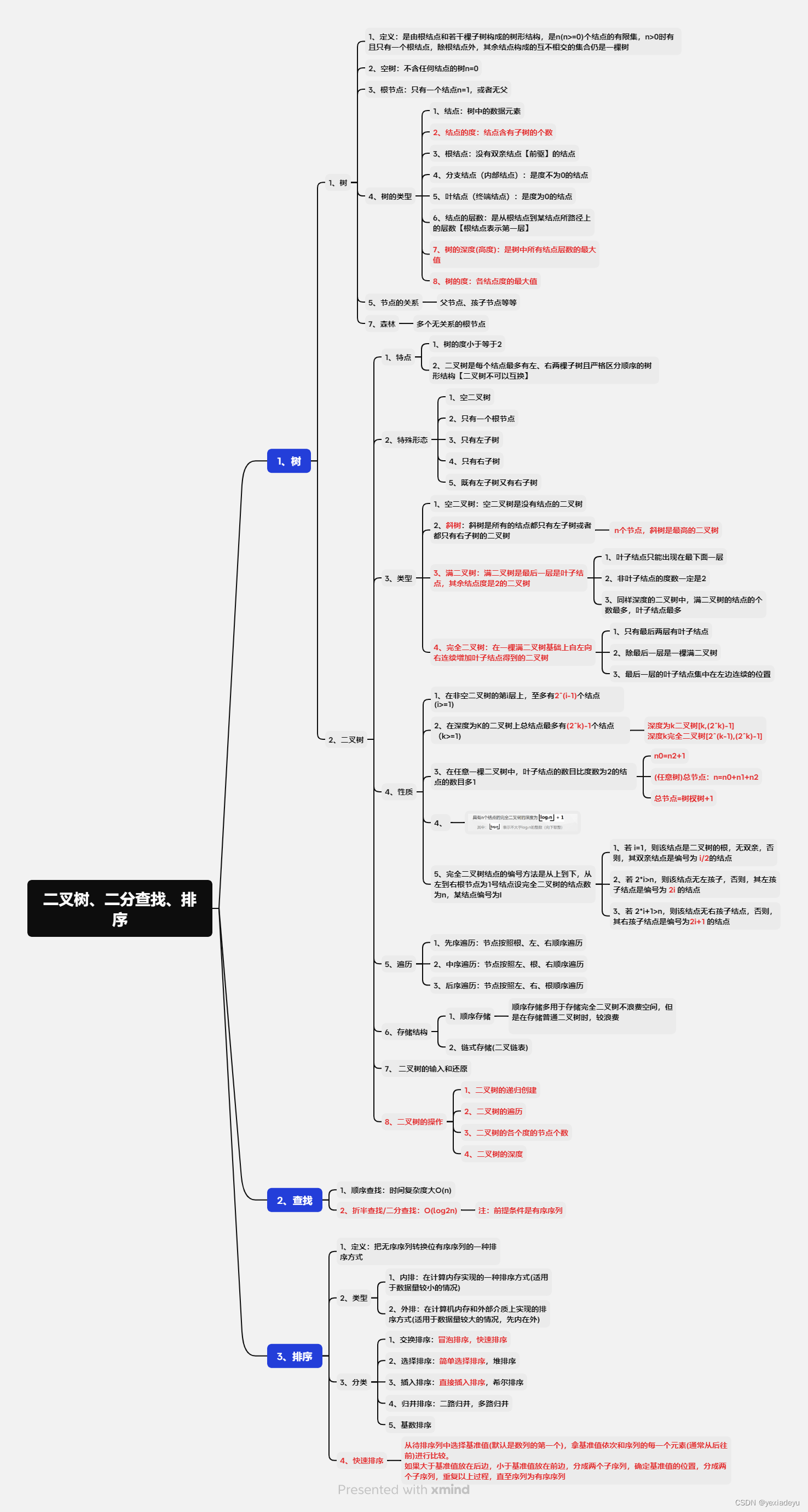