import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Properties;
/**
* Properties属性文件操作工具类
*
*/
public class PropUtil {
private static PropUtil pu = null;
private Properties properties = new Properties();
private String propfilepath = LConst.Cookie_File;
private String description = "";
public synchronized static PropUtil getInstance() {
if (pu == null)
pu = new PropUtil();
return pu;
}
/** 向默认属性文件中写入键值对 **/
public void putV(String key, String value) {
OutputStream outputStream;
InputStream fis = null;
try {
fis = new FileInputStream(getPropfilepath());
properties.load(fis);
fis.close();
File configFile = new File(getPropfilepath());
outputStream = new FileOutputStream(configFile);
properties.setProperty(key, value);
properties.store(outputStream, description);
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/** 从默认属性文件中读取指定键对应的值 **/
public String getV(String key) {
try {
InputStream ips = new BufferedInputStream(new FileInputStream(
getPropfilepath()));
properties.load(ips);
String value = properties.getProperty(key);
return value;
} catch (FileNotFoundException e) {
e.printStackTrace();
return null;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
/** 向指定属性文件中写入键值对 **/
public void putV(String key, String value, String path) {
OutputStream outputStream;
Properties properties = new Properties();
InputStream fis = null;
try {
fis = new FileInputStream(path);
properties.load(fis);
fis.close();
File configFile = new File(path);
outputStream = new FileOutputStream(configFile);
properties.setProperty(key, value);
properties.store(outputStream, description);
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/** 从指定属性文件中读取指定键对应的值 **/
public String getV(String key, String path) {
Properties properties = new Properties();
try {
InputStream ips = new BufferedInputStream(new FileInputStream(path));
properties.load(ips);
String value = properties.getProperty(key);
return value;
} catch (FileNotFoundException e) {
e.printStackTrace();
return null;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public String getPropfilepath() {
return propfilepath;
}
public void setPropfilepath(String propfilepath) {
this.propfilepath = propfilepath;
}
}
Properties操作接口
最新推荐文章于 2023-07-11 10:59:18 发布
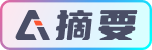