动态字母瀑布背景
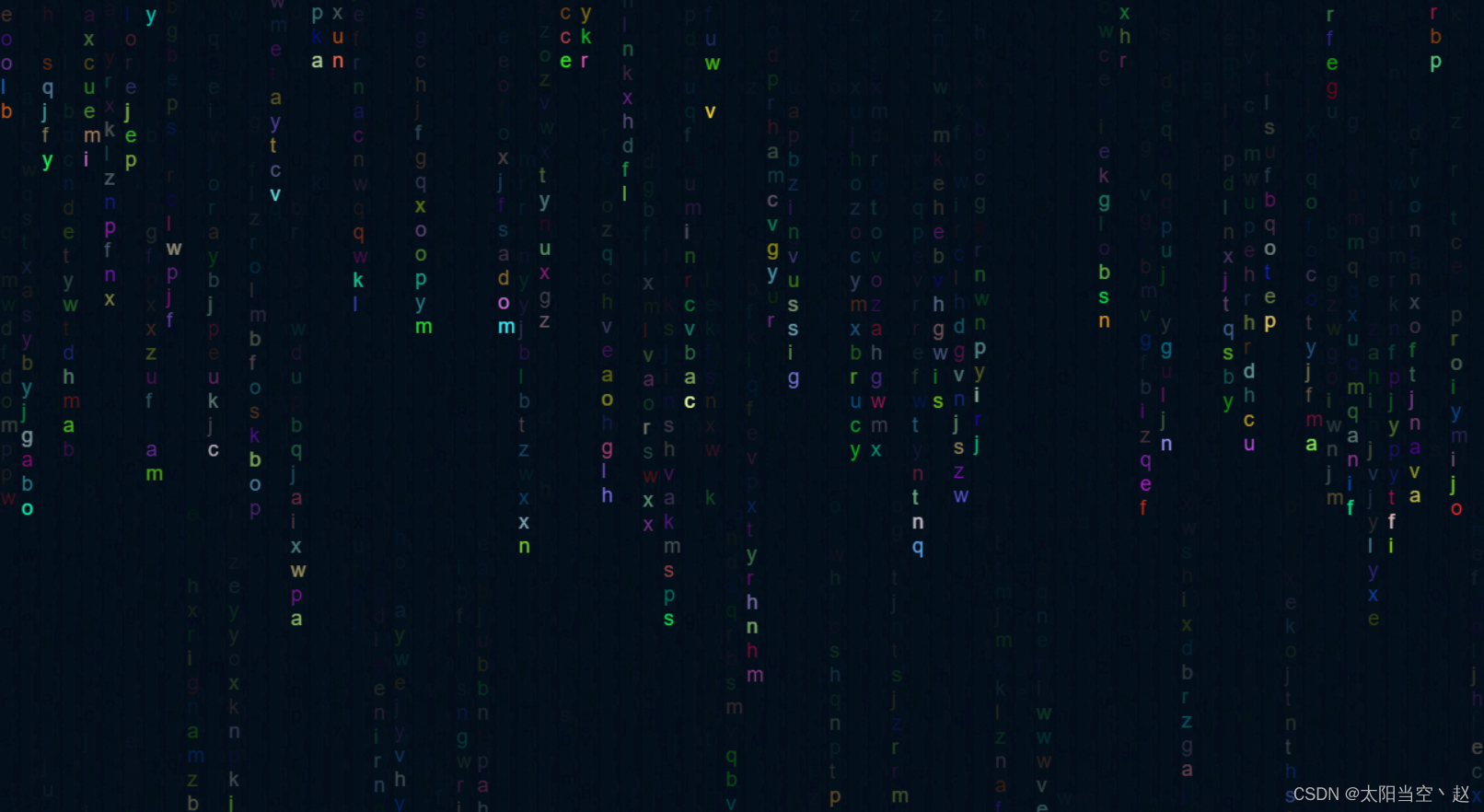
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>动态字母瀑布背景</title>
<style>
* {
margin: 0;
padding: 0;
}
body {
height: 100vh;
}
#canvas-box {
width: 100vw;
height: 100vh;
position: absolute;
}
</style>
</head>
<body>
<canvas id="canvas-box">
</canvas>
<script>
const dom = document.getElementById("canvas-box")
const ctx = dom.getContext('2d')
const width = window.innerWidth
const height = window.innerHeight
dom.width = width
dom.height = height
const bgColor = 'rgba(2,15,26,0.16)'
const columnWidth = 18
const columnHeight = columnWidth + 3
const columnNum = parseInt(width / columnWidth)
function randomCode() {
const str = "abcdefghijklmnopqrstuvwxyz"
const randNum = parseInt(Math.random() * str.length)
return str.split('')[randNum]
}
function randomColor() {
const r = Math.random() * 255
const g = Math.random() * 255
const b = Math.random() * 255
return `rgb(${r},${g},${b})`
}
function randomRows() {
const rowNum = height / columnWidth
list.forEach((num, index) => {
const h = parseInt(Math.random() * rows)
list[index] = h < rowNum / 2? h + rowNum : h
})
return list
}
let list = new Array(columnNum)
const rows = parseInt(height / columnHeight)
list.fill(rows)
ctx.font = `${columnWidth}px Arial`;
let column = 0
let timer = null
function drow() {
if (timer) {
clearInterval(timer)
}
timer = setInterval(() => {
ctx.fillStyle = bgColor
ctx.fillRect(0, 0, width, height)
for (let i = 0; i < columnNum; i++) {
let thisHeight = 0
ctx.fillStyle = randomColor()
thisHeight = (column % list[i]) * columnHeight
ctx.fillText(randomCode(), i * columnWidth, thisHeight)
}
column++
if (column % rows === 0) {
randomRows()
}
}, 80)
}
drow()
</script>
</body>
</html>
雪花飘落背景
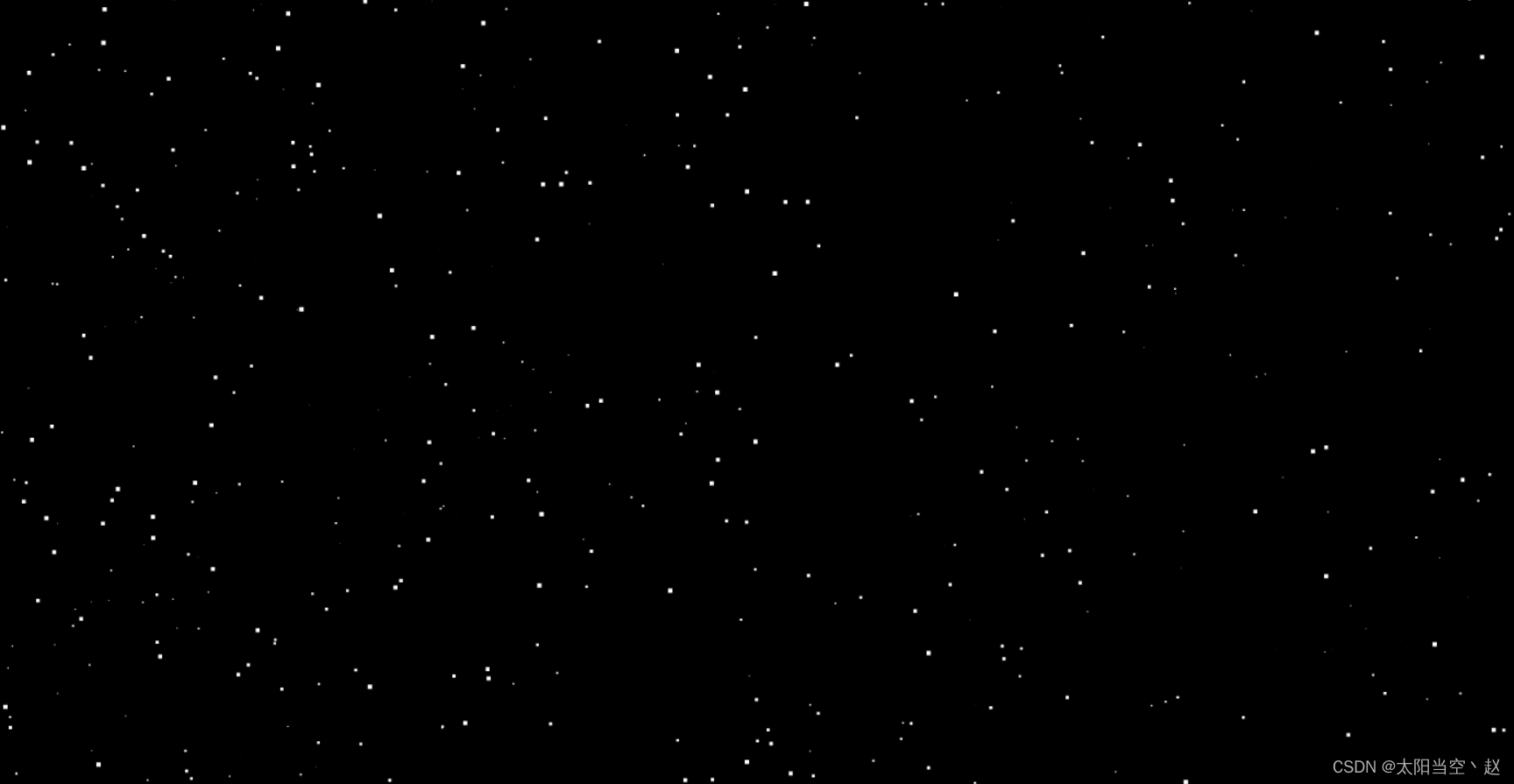
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>demo6</title>
<style>
body{
margin: 0;
width: 100vw;
height: 100vh;
overflow: hidden;
}
#canvas{
background: black;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
const canvas = document.querySelector("#canvas")
const ctx = canvas.getContext("2d")
function init(){
const {clientWidth:width,clientHeight:height} = document.body
canvas.width = width
canvas.height = height
const snowList = Array.from(new Array(Math.floor(width / 3))).map(()=> {
return {
X: Math.random() * width,
Y: Math.random() * height,
W: Math.random() * 4,
stepX: Math.random() * 2 - 1,
stepY: Math.random() + .2
}
})
ctx.fillStyle = "#ffffff"
const draw = () => {
ctx.clearRect(0,0,width,height)
ctx.beginPath()
for (const snow of snowList) {
ctx.rect(snow.X,snow.Y,snow.W,snow.W)
snow.X = snow.X >= width || snow.X < 0 ? snow.stepX = -snow.stepX : snow.X + snow.stepX
snow.Y = snow.Y > height? 0 :snow.Y + snow.stepY
}
ctx.fill()
}
setInterval(()=>{
draw()
},.01)
}
init()
window.onresize = init
</script>
</body>
</html>
带线的粒子😄
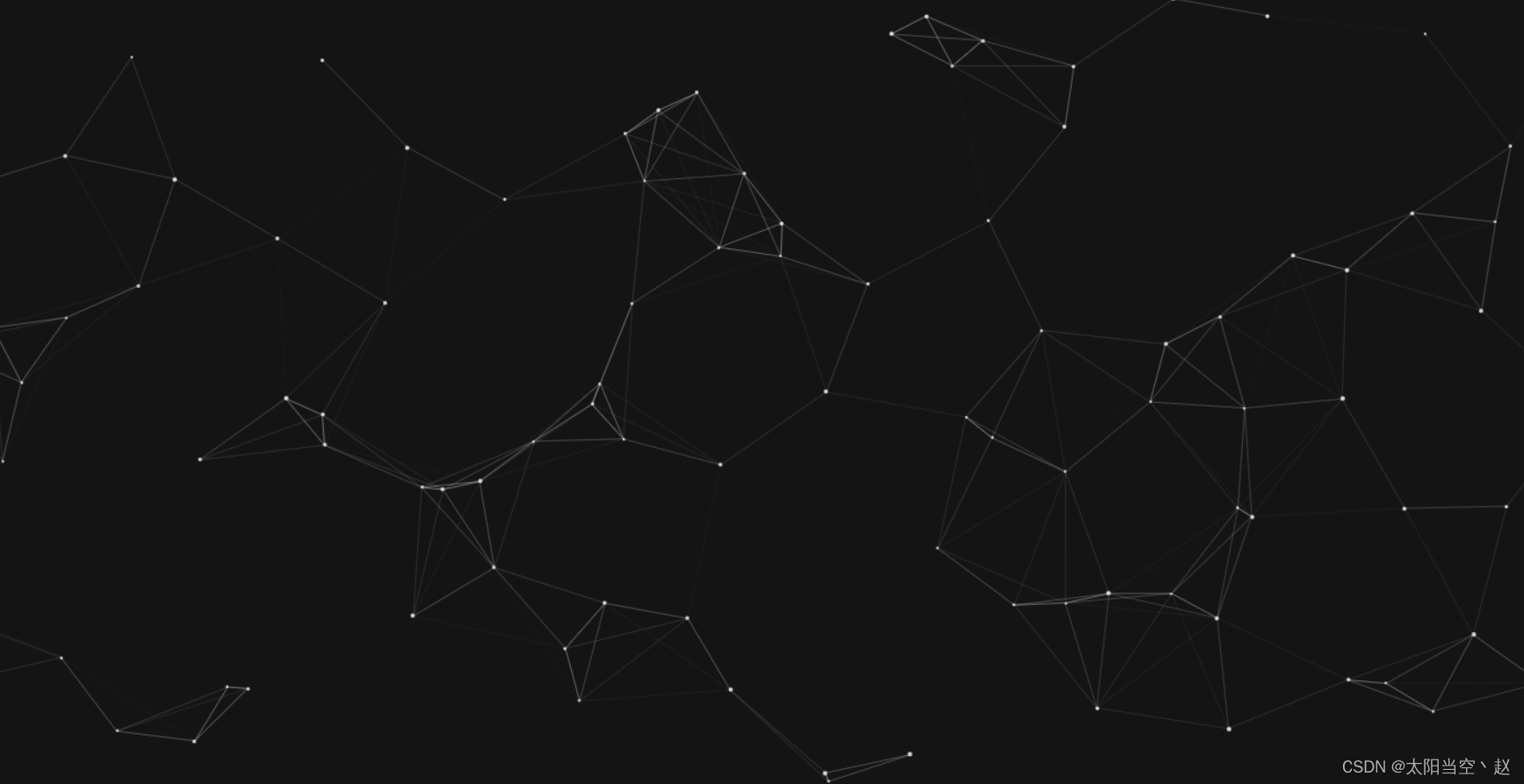
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>动态粒子背景</title>
<style>
body {
padding: 0;
margin: 0;
}
#canvas {
position: fixed;
width: 100vw;
height: 100vh;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
class Point {
constructor(size) {
const canvasNode = document.querySelector("#canvas")
this.stepX = Math.random() > 0.5 ? this.getRandom(.4, .6) : -this.getRandom(.4, .6)
this.stepY = Math.random() > 0.5 ? this.getRandom(.3, .5) : -this.getRandom(.3, .5)
this.canvasWidth = canvasNode.offsetWidth
this.canvasHeight = canvasNode.offsetHeight
this.x = Math.random() * this.canvasWidth
this.y = Math.random() * this.canvasHeight
this.size = size ? size : this.getRandom(1, 2)
}
getRandom(min, max) {
return Math.random() * (max - min) + min
}
sport() {
this.x += this.stepX
this.y += this.stepY
if (this.x < 0 || this.x > this.canvasWidth) {
this.stepX = -this.stepX
}
if (this.y < 0 || this.y > this.canvasHeight) {
this.stepY = -this.stepY
}
}
}
class Particle {
canvasNode = null
canvas = null
pointNum = 0
pointList = []
velocity = 0
drawLineDistance = 150
timer = null
constructor(pointNum = 20, valocity = 10) {
this.canvasNode = document.querySelector("#canvas")
this.canvas = this.canvasNode.getContext("2d")
const {offsetWidth, offsetHeight} = this.canvasNode
this.canvasNode.width = offsetWidth
this.canvasNode.height = offsetHeight
this.pointNum = pointNum
this.velocity = valocity
this.canvasNode.style.backgroundColor = "rgba(0,0,0,0.92)"
this.createPoints()
const that = this
this.draw(that)
}
createPoints() {
for (let i = 0; i < this.pointNum; i++) {
const point = new Point()
this.pointList.push(point)
}
}
clearCanvas() {
const {offsetWidth, offsetHeight} = this.canvasNode
this.canvas.clearRect(0, 0, offsetWidth, offsetHeight)
}
clearTimer(){
clearTimeout(this.timer)
}
getPointsDistance(pointOne, pointTwo) {
const {x: xOne, y: yOne} = pointOne
const {x: xTwo, y: yTwo} = pointTwo
const x = Math.abs(xOne - xTwo)
const y = Math.abs(yOne - yTwo)
return Math.sqrt(x * x + y * y)
}
drawPoint() {
this.canvas.fillStyle = "#d3d3d3"
for (const point of this.pointList) {
this.canvas.beginPath()
this.canvas.arc(point.x, point.y, point.size, 0, Math.PI * 2, false)
this.canvas.fill()
this.canvas.closePath()
point.sport()
}
}
drawLine() {
this.canvas.lineWidth = ".7"
for (let i = 0; i < this.pointList.length; i++) {
for (let j = i + 1; j < this.pointList.length; j++) {
const distance = this.getPointsDistance(this.pointList[i], this.pointList[j])
if (distance > this.drawLineDistance) continue
this.canvas.beginPath()
this.canvas.strokeStyle = `rgba(234, 234, 234, ${(this.drawLineDistance - distance) / this.drawLineDistance * 0.8})`
this.canvas.moveTo(this.pointList[i].x, this.pointList[i].y)
this.canvas.lineTo(this.pointList[j].x, this.pointList[j].y)
this.canvas.stroke()
this.canvas.closePath()
}
}
}
draw(that) {
that.clearCanvas()
that.drawPoint()
that.drawLine()
clearTimeout(that.timer)
that.timer = setTimeout(() => {
that.draw(that)
}, that.velocity)
}
}
let particle = new Particle(100, 10)
window.onresize = function () {
particle.clearTimer()
particle = new Particle(100,10)
}
</script>
</body>
</html>
图片验证码
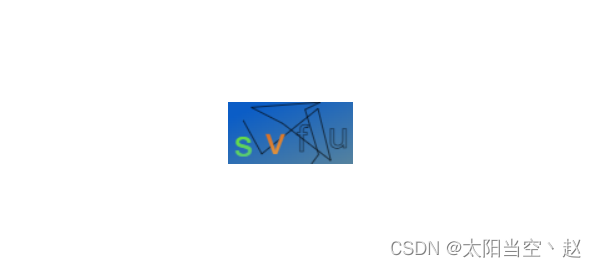
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>canvas验证码</title>
<style>
* {
margin: 0;
padding: 0;
}
body {
height: 100vh;
display: grid;
place-items: center;
}
#canvas{
width: 100px;
aspect-ratio: 2 / 1;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
const dom = document.getElementById("canvas")
const ctx = dom.getContext("2d")
const width = dom.clientWidth
const height = dom.clientHeight
dom.width = width
dom.height = height
let str = randomCode(4)
function randomColor() {
const r = Math.random() * 255
const g = Math.random() * 255
const b = Math.random() * 255
return `rgb(${r},${g},${b})`
}
function GradualChangeColor(){
let grd=ctx.createLinearGradient(width*0.2,width*0.05,width*0.5,width*0.75);
grd.addColorStop(0,randomColor());
grd.addColorStop(1,randomColor());
return grd
}
function bgDrow(){
ctx.fillStyle = GradualChangeColor()
ctx.fillRect(0, 0, width, height)
}
function lineDrow(){
let nodeNum = parseInt(Math.random() * 5 + 5)
let lineWidth = Math.random() * 2
function pos(){
let x = Math.random() * width
let y = Math.random() * height
return [x,y]
}
ctx.strokStyle = GradualChangeColor()
ctx.beginPath();
ctx.moveTo(...pos());
ctx.lineWidth = lineWidth
for (let i = 0; i < nodeNum; i++) {
ctx.lineTo(...pos())
}
ctx.stroke();
}
function textDraw(){
str = randomCode(4)
ctx.clearRect(0, 0, width, height)
bgDrow()
lineDrow()
const fontSize = 0.6 * height
const list = str.split('')
const font = ["Arial", "Inter", "Helvetica"]
let top = 0
let left = 5
for (const number of list) {
let i = Math.floor(Math.random() * font.length)
ctx.font=`${fontSize}px ${font[i]}`;
ctx.fillStyle = GradualChangeColor()
ctx.strokStyle = GradualChangeColor()
top = Math.random() * height
top = top > fontSize? top : top + fontSize / 2
Math.random() > 0.5 ? ctx.fillText(number,left,top) : ctx.strokeText(number,left,top)
left += width / list.length
}
}
function randomCode(length) {
const strArr = "1234567890abcdefghijklmnopqrstuvwxyz"
let randNum = 0
let strList = []
for (let i = 0; i < length; i++) {
randNum = parseInt(Math.random() * strArr.length)
strList.push(strArr.split('')[randNum])
}
return strList.join('')
}
textDraw()
dom.onclick = textDraw
</script>
</body>
</html>