https://blog.csdn.net/weixin_43754049/article/details/125895330
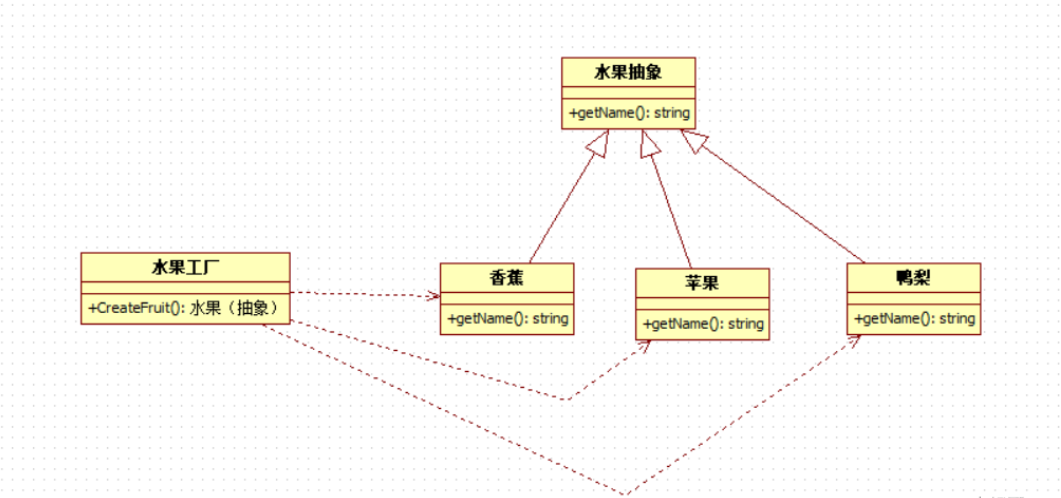
#include <iostream> //简单工厂案例
using namespace std;
class AbstractFruit {
public:
virtual void ShowName() = 0;
};
class Apple : public AbstractFruit { //苹果
public:
virtual void ShowName() {
cout << "我是苹果" << endl;
}
};
class Banana : public AbstractFruit { //香蕉
public:
virtual void ShowName() {
cout << "我是香蕉" << endl;
}
};
class Pear : public AbstractFruit { //鸭梨
public:
virtual void ShowName() {
cout << "我是鸭梨" << endl;
}
};
class FruitFactory { //水果工厂
public:
//设置为静态 为该类的所有对象共享
static AbstractFruit* CreateFruit(string flag) {
if (flag == "apple") {
return new Apple;
}
else if (flag == "banana") {
return new Banana;
}
else if (flag == "pear") {
return new Pear;
}
else {
return NULL;
}
}
static int a;
};
int FruitFactory::a = 8;
void test01() {
//创建一个工厂对象
//FruitFactory* factory = new FruitFactory; // zane
//这里我们发现不再像普通的多态一样需要new Apple;
//而是在工厂的成员函数参数中传入一个string
//让工厂类自动帮我们创建一个Apple对象
//不用考虑创建过程 拿来直接用 减少了耦合性
cout <<FruitFactory::a << endl;
AbstractFruit* fruit = FruitFactory::CreateFruit("apple");
fruit->ShowName();
delete fruit;
fruit = FruitFactory::CreateFruit("banana");
fruit->ShowName();
delete fruit;
fruit = FruitFactory::CreateFruit("pear");
fruit->ShowName();
delete fruit;
//delete factory; // zane
}
int main() {
test01();
return 0;
}
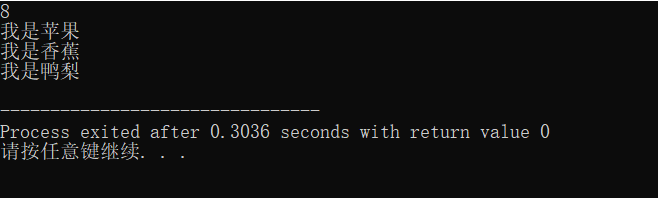
总结:好代码就是让耦合性不断的变低 这里直接传一个string就可以创建对象了 比传统的多态更进了一步