import pandas as pd 1.用列表生成Series s=pd.Series([1,3,5,np.nan,6,8]) print(s) 2.用Series,字典,对象生成DataFrame df2=pd.DataFrame({'A':1., 'B':pd.Timestamp('20130102'), 'C':pd.Series(1,index=list(range(4)),dtype='float32'), 'D':np.array([3]*4,dtype='int32'), 'E':pd.Categorical(["test","train","test","train"]), 'F':'foo' }) print(df2) 3.用含日期时间索引,标签,Numpy数组生成DataFrame d=pd.date_range('2013-01-01',periods=6) df=pd.DataFrame(np.random.randn(6,4),index=d,columns=list('ABCD')) print(df) print(df.dtypes)#查看数据类型 print(df.head(2))#查看头部数据 print(df.tail(2))#查看尾部数据 print(df.columns)#查看列表名 print(df.describe())#查看数据的统计摘要 print(df.sort_index(axis=1,ascending=False))#按轴排序,降序 print(df.sort_values(by='B'))#按值排序(默认升值) print(df['A'])#打印某一列 print(df[1:3])#切片打印 print(df['2013-01-02':'2013-01-03'])#求一行元素的数据 print(df.loc['2013-01-02']) print(df.loc[:,['A','B']])#用标签选择多列数据 print(df.loc['2013-01-01':'2013-01-03',['A','B','C']])#标签切片 print(df.loc['2013-01-01','A'])#取出某个标签的数值 print(df.at['2013-01-01','A']) print(df.iloc[3])#按照位置来取,取出第三行元素 print(df.iloc[1:2,2:3])#切片也可以 print(df.iloc[[1,2,3],[0,2,3]])#取出2,3,4行,1,3,4列 print(df.iloc[1:3,:])#整行切片 print(df.iloc[:,1:3])#整列切片 print(df.iloc[1,1])#提取某一个数值 print(df.iat[1,1]) print(df[df['B']>0])#帅选B中大于0的元素 print((df[df>0]))#筛选所有大于0的元素 用isin()筛选 df3=df.copy() df3['E']=['one','one','two','three','four','three'] # print(df3) print(df3[df3['E'].isin(['two','four'])]) 用索引自动对齐新增列的数据 s1=pd.Series([1,2,3,4,5,6],index=d) print(s1) df['F']=s1 print(df) 按标签赋值 df.at['2013-01-01','A']=0 print(df) #按位置赋值 df.iat[0,2]=0 print(df) #按数组赋值 df['A']=[1,2,3,4,5,6] print(df) where 条件赋值 df3=df.copy() df3[df3<0]=0 print(df3) 空值 df1=df.reindex(index=d[0:4],columns=list(df.columns)+['E']) df1.loc[d[0]:d[1],'E']=1 print(df1) print(df1.dropna(how='any'))#删掉空值的行 print(df1.fillna(value=5))#将空值赋值为5 #判断是否存在空值 print(pd.isna(df1)) 算数运算 df1=pd.DataFrame(np.random.randn(4,5)) df2=pd.DataFrame(np.random.randn(3,4)) print(df1+df2) #比较大小 print("df1=df2",df1.eq(df2)) print("df1!=df2",df1.ne(df2)) print("df1>df2",df1.gt(df2)) print("df1<df2",df1.lt(df2)) print("df1>=df2",df1.ge(df2)) print("df1<=df2",df1.le(df2)) 统计 print(df1.mean())#求均值 print(df1.cumsum())#累加 print(pd.concat([df1,df2]))#合并 l=pd.DataFrame({'key':['foo','foo','bar'],'rval':[3,4,5]}) r=pd.DataFrame({'key':['foo','foo','bar'],'rval':[0,1,2]}) print(pd.merge(l,r,on='key')) 追加 df=pd.DataFrame(np.random.randn(8,4),columns=['A','B','C','D']) print(df) s=df.iloc[3] print(s) print(df._append(s,ignore_index=True)) 分组 df=pd.DataFrame({'A':['foo','bar','foo','bar', 'foo','bar','foo','bar'], 'B':['one','two','three','four', 'one','two','three','four'], 'C':np.random.randn(8), 'D':np.random.randn(8) }) print(df) print(df.groupby('A').sum()) print(df.groupby(['A','B']).sum()) 时间序列 rng=pd.date_range('1/1/2012',periods=100,freq='S') ts=pd.Series(np.random.randint(0,500,len(rng)),index=rng) print(ts) print(ts.resample('5Min').sum()) 时区表示 rng=pd.date_range('3/6/2012 00:00',periods=5,freq='D') ts=pd.Series(np.random.randn(len(rng)),rng) print(ts) print(ts.plot)
python.数据分析.pandas
最新推荐文章于 2024-09-15 22:31:42 发布
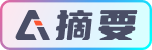