既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
功能点:
- 避免业务逻辑,只做底层的相关操作;比如对用户的增删改查,奖品的增删改查(这些功能只是单纯的操作文件,而不是处理复杂的业务逻辑),直接和
storage
模块的关联。(稍后介绍storage
模块)。知识点:
- 父类的创建。
- son文件的读写,私有函数的定义。
- 字典的练习,循环的练习。
- 条件语句的练习。
- 异常的处理与抛出。
第二个模块:admin 模块,这是给管理员的模块。
该模块实现的功能为:
功能点:
- 继承 base 模块
- 开发用户的增删改查
- 开发奖品的增删改查
- PS:似乎与 base 模块相同,其实不然。admin 模块的
增删改查
是添加业务逻辑处理的,比如满足了某些条件才能够执行修改或删除…知识点:
- 类的继承
- 多态的练习 super 函数
- 条件语句的练习
- 循环语句的练习
第三个功能模块: user 模块,主要实现用户的验证与抽奖操作
该模块的功能未:
功能点:
- 用户身份验证
- 抽奖功能的实现
知识点:
- 类的继承
- 父类私有函数的调用
- 启蒙与强化开发思维
⭐️ 抽奖系统代码结构图
在
cloudstudio
中会自动生成一个工作空间,然后我们分别创建 common 包与 storage 包
- 创建 common 包,在 common 包内分别创建
error.py
、consts.py
、utils.py
模块。- 创建 storage 包(其实就是个文件夹),创建
user.json
、gift.json
分别存储用户信息和奖品信息。- 而我们的
base、admin、user
模块则是直接创建在项目根目录下即可,见下图:PS:这也是一个比较简单的代码结构。
⭐️ 项目基础类 - 文件检查
接下来通过 base.py
来书写基础类,当前要实现的基本功能就是导入 user.json 与 gift.json
进行文件检查
- 我们需要自定义三个异常类用来判断文件的异常:
- 1、判断文件地址路径是否存在 —> NotPathError
- 2、判断文件是否是 json 格式 —> FormatError
- 3、判断是否是文件 —> NotFileError
🌟 base.py 基础类文件检查示例如下:
# coding:utf-8
"""
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
"""
import os
from common import error
class Base(object):
def \_\_init\_\_(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self):
if not os.path.exists(self.user_json): # 判断文件地址路径是否存在
raise error.NotPathError("not found {} ".format(self.user_json))
if not self.user_json.endswith('.json'): # 判断文件是否是 json 格式
raise error.FormatError()
if not os.path.isfile(self.user_json): # 判断是否是文件
raise error.NotFileError()
def \_\_check\_gift\_json(self):
if not os.path.exists(self.gift_json): # 判断文件地址路径是否存在
raise error.NotPathError("not found {} ".format(self.gift_json))
if not self.gift_json.endswith('.json'): # 判断文件是否是 json 格式
raise error.FormatError()
if not os.path.isfile(self.gift_json):
raise error.NotFileError()
if __name__ == '\_\_main\_\_':
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
🌟 common 模块 的 error.py 脚本的代码如下:
# coding:utf-8
class NotPathError(Exception): # 文件路径错误
def \_\_init\_\_(self, message):
self.message = message
class FormatError(Exception): # 文件格式后缀错误
def \_\_init\_\_(self, message="file need json format"):
self.message = message
class NotFileError(Exception): # 非文件错误
def \_\_init\_\_(self, message="It's not file"):
self.message = message
此时在 base.py
基础模块中我们发现, __check_user_json()
与 __check_gift_json()
函数的基本功能是一样的,都是检查文件。这个时候就可以将其封装为一个公共函数 check_file
用以调用,所以我们可以在 utils.py
模块定义一个 check_file
函数。
🌟 utils.py 模块 check_file 函数示例如下
# coding:utf-8
import os
from .error import NotPathError, NotFileError, FormatError
def check\_file(path):
if not os.path.exists(path): # 判断文件地址路径是否存在
raise NotPathError("not found {} ".format(path))
if not path.endswith('.json'): # 判断文件是否是 json 格式
raise FormatError()
if not os.path.isfile(path): # 判断是否是文件
raise NotFileError()
那么此时我们的 base.py
基础模块优化后的脚本代码就如下:
# coding:utf-8
"""
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
"""
import os
from common.utils import check_file
class Base(object):
def \_\_init\_\_(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self):
check_file(self.user_json)
def \_\_check\_gift\_json(self):
check_file(self.gift_json)
if __name__ == '\_\_main\_\_':
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
执行结果如下:
尝试做几个文件异常的判断:
⭐️ base用户相关功能实现
在 base.py
模块基础上,增加 __read_users()
与 __write_user()
函数。
所以接下来,我们就实现一下前三个目标。
# coding:utf-8
"""
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*
3、确定用户表中每个用户的信息字段
4、读取 `user.json` 文件
5、写入 `user.json` 文件(检测该用户是否存在),存在则不可写入
"""
import os
import json
import time
from common.utils import check_file
from common.error import UserExistsError
class Base(object):
def \_\_init\_\_(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 user.json 文件
check_file(self.user_json)
def \_\_check\_gift\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 gift.json 文件
check_file(self.gift_json)
def \_\_read\_user(self): # 读取 user.json 文件
with open(self.user_json) as f:
data = json.loads(f.read())
return data
def \_\_write\_user(self, \*\*user): # 写入用户信息(进行写入时的判断)
if "username" not in user:
raise ValueError("missing username") # 缺少 username 信息
if "role" not in user:
raise ValueError("missing role") # 缺少角色信息(缺少权限信息)
user['active'] = True # 初始化用户基础信息
user['create\_time'] = time.time()
user['update\_time'] = time.time()
user['gifts'] = []
users = self.__read_user() # 读取 user.json
# print(users) # 打印输出 users 是为了调试
# return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
raise UserExistsError('username {} had existe'.format(user['username']))
users.update(
{user['username']: user}
)
json_users = json.dumps(users)
with open(self.user_json, 'w') as f:
f.write(json_users)
if __name__ == '\_\_main\_\_':
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
base.write_user(username='Neo', role='admin')
🌟 将 时间戳 封装为 utils.py 模块的一个公共函数
# coding:utf-8
import os
import time
from .error import NotPathError, NotFileError, FormatError
def timestamp\_to\_string(timestamp):
time_obj = time.localtime(timestamp) # 将传入的时间戳实例化成一个时间对象
time_str = time.strftime('%Y-%m-%d %H:%M:%S ', time_obj)
return time_str
def check\_file(path):
if not os.path.exists(path): # 判断文件地址路径是否存在
raise NotPathError("not found %s " % path)
if not path.endswith('.json'): # 判断文件是否是 json 格式
raise FormatError()
if not os.path.isfile(path): # 判断是否是文件
raise NotFileError()
🌟 对 base.py 模块中的 __read_user() 进行修改
# coding:utf-8
"""
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*
3、确定用户表中每个用户的信息字段
4、读取 `user.json` 文件
5、写入 `user.json` 文件(检测该用户是否存在),存在则不可写入
\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*\*
"""
import os
import json
import time
from common.utils import check_file, timestamp_to_string
from common.error import UserExistsError
class Base(object):
def \_\_init\_\_(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 user.json 文件
check_file(self.user_json)
def \_\_check\_gift\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 gift.json 文件
check_file(self.gift_json)
def \_\_read\_user(self, time_to_str=True): # 读取 user.json 文件;
# timestamp\_to\_string 修改为 True 时时间戳为可读的格式(调试)
with open(self.user_json, 'r') as f:
data = json.loads(f.read())
if time_to_str == True:
for username, t in data.items():
t['create\_time'] = timestamp_to_string(t['create\_time'])
# print(t['create\_time']) # 打印输出时间格式是否改为 可读的 "%Y-%m-%d %H:%M:%S" 格式
t['update\_time'] = timestamp_to_string(t['update\_time'])
data[username] = t
print(data) # 调试打印输出 \_\_read\_user() 的用户信息
return data
def write\_user(self, \*\*user): # 写入用户信息(进行写入时的判断)
if "username" not in user:
raise ValueError("missing username") # 缺少 username 信息
if "role" not in user:
raise ValueError("missing role") # 缺少角色信息(缺少权限信息)
user['active'] = True # 初始化用户基础信息
user['create\_time'] = time.time()
user['update\_time'] = time.time()
user['gifts'] = []
users = self.__read_user() # 读取 user.json
print(users) # 打印输出 users 是为了调试
return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
raise UserExistsError('username {} had existe'.format(user['username']))
users.update(
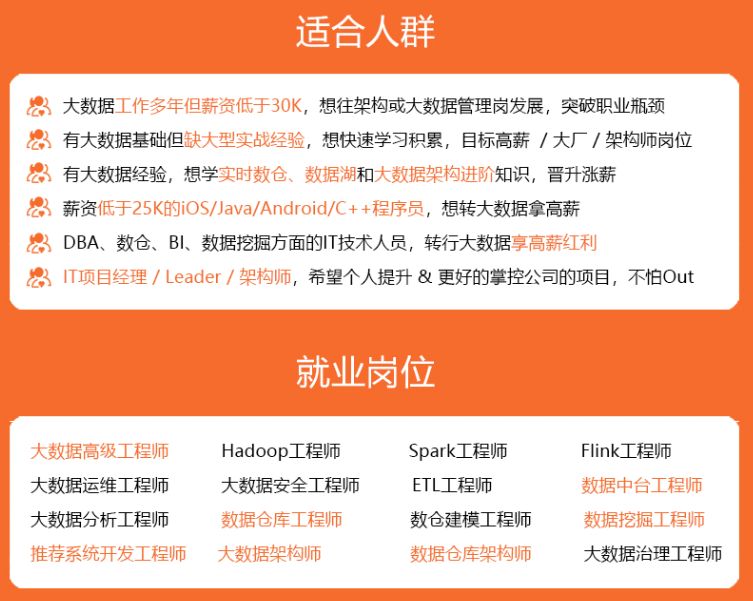
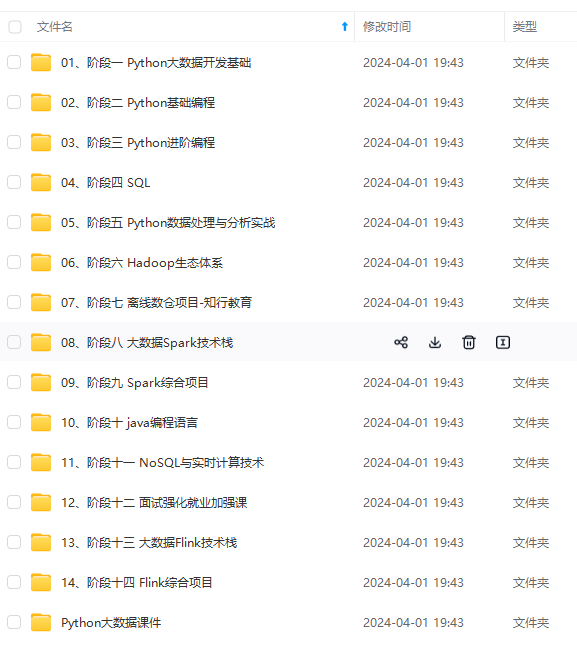
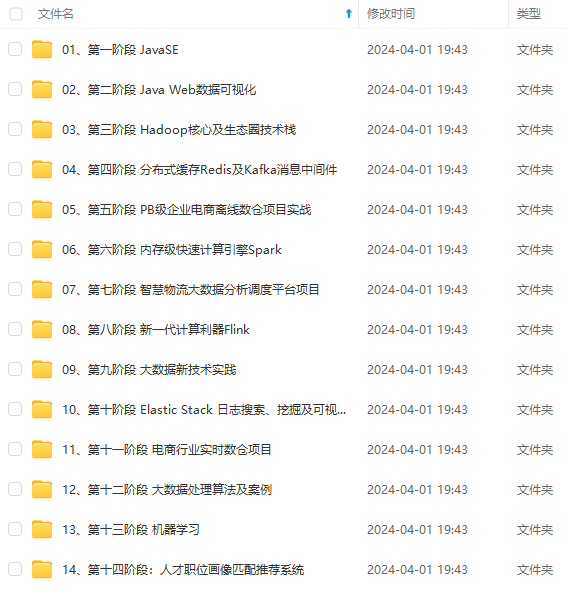
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
raise UserExistsError('username {} had existe'.format(user['username']))
users.update(
[外链图片转存中...(img-9mxMRdG9-1715718543743)]
[外链图片转存中...(img-C1HJJodw-1715718543743)]
[外链图片转存中...(img-q3Rljt69-1715718543743)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**