一、日期时间对象Date
作用:js 提供的内置构造函数,专门用来处理日期时间。
new Date( )不传参时,默认返回当前时间;传入参数时,返回你传入的时间。以中国标准时间形式显示。
<script>
var date = new Date()
console.log(date) //Date Thu Aug 25 2022 09:31:55 GMT+0800 (中国标准时间)
var date1 = new Date(2021,10,1) //以数值型传入
console.log(date1) //Date Mon Nov 01 2021 00:00:00 GMT+0800 (中国标准时间)
//计算机月从0开始,所以输入10月,打印出Nov(九月)
var date2 = new Date('2021/10/1') //以字符串传入
console.log(date2) //Date Fri Oct 01 2021 00:00:00 GMT+0800 (中国标准时间)
var date3 = new Date('2021-10-1')
console.log(date3) //Date Fri Oct 01 2021 08:00:00 GMT+0800 (中国标准时间)
//- 的表示方法,时间默认从08:00:00开始的
</script>
new Date(year, month, day, hour, minute, second)传递参数说明:
year:年
month:当年的第几月,0~11,0表示1月,11表示12月
day:当月的第几天,1~31
hour:当天的几点,0~23
minute:当时的多少分钟,0~60
second:那分钟的多少秒,0~60
常用的八个方法示例:
<script>
function test4() {
var currentTime = new Date()
var year = currentTime.getFullYear() //年
console.log('year: ', year)
var month = currentTime.getMonth() //月
console.log('month:', (month + 1)) //注:计算机月从0开始
var day = currentTime.getDate() //日
console.log('hours :', day)
var hours = currentTime.getHours() //时
console.log('hours :', hours)
var minutes = currentTime.getMinutes() //分
console.log('minutes :', minutes)
var seconds = currentTime.getSeconds() //秒
console.log('seconds :', seconds)
var week = currentTime.getDay() // 星期
console.log('week :', week)
var time = currentTime.getTime() //毫秒
console.log('time', time) //1秒=1000毫秒 1分钟=60秒 1小时=60分
}
test4()
</script>
一月 | January | 七月 | July | 星期一 | Monday |
二月 | February | 八月 | August | 星期二 | Tuesday |
三月 | March | 九月 | September | 星期三 | Wednesday |
四月 | April | 十月 | October | 星期四 | Thursday |
五月 | May | 十一月 | November | 星期五 | Friday |
六月 | June | 十二月 | December | 星期六 | Saturday |
星期天 | Sunday |
二、格式化日期时间
<body>
/*格式化当前日期时间*/
function formateCurrentTime(type) {
var time = new Date() //Thu Aug 25 2022 09:48:16 GMT+0800(中国标准时间)
var year = time.getFullYear()
var month = time.getMonth()
var date = time.getDate()
var hours = time.getHours()
var minutes = time.getMinutes()
var seconds = time.getSeconds()
// var timeStr = `${year}年${month}月$(date}$(hours)时$(minutes}分$(seconds)秒`
// return timeStr
switch(type){
case 0:
return `${year}年${month}月${date}日${hours}时${minutes}分${seconds}秒`
case 1:
return `${year}/${month}/${date} ${hours}:${minutes}:${seconds}`
case 2:
return `${year}-${month}-${date} ${hours}:${minutes}:${seconds}`
default:
return `${year}/${month}/${date} ${hours}:${minutes}:${seconds}`
}
}
<script>
var currentTime = formateCurrentTime()
document.write(currentTime) //2022/7/25 19:5:49
</script>
</body>
三、计算时间差
格林威治时间:计算机时间的原点
规则:1970年1月1日(00:00:00: GMT)
<body>
<script>
//1.计算时间毫秒差值
//2.换算 秒->分->小时->天->年
var time1 = new Date('2019-01-01 00:00:00')
var time2 = new Date('2019-01-03 04:55:34')
var time = time2.getTime()-time1.getTime() //毫秒差
//换算-天 相差的总毫秒/1天的毫秒 = 相差的天数
var day = time/(1000*60*60*24)
day = Math.floor(day)
console.log(day,'天') //2天
//相差的总毫秒 - day天的毫秒
var hoursTime = time - day*(1000*60*60*24)
var hours = hoursTime/(1000*60*60)
console.log(Math.floor(hours),'小时') //4小时
//相差的小时的总毫秒 - 耗时的毫秒
var minTime = hoursTime - Math.floor(hours) * (1000*60*60)
var min = minTime/(1000*60)
console.log(Math.floor(min),'分钟') //55分钟
//输出:
/*
2 天
4 小时
55 分钟
*/
</script>
</body>
四、BOM
BOM,Browser Object Model,即浏览器对象模型。
作用:操作浏览器的能力。核心是 window 对象。
我们可以操作哪些内容:
1.获取一些浏览器的相关信息(窗口的大小)
2. 操作浏览器进行页面跳转
3.获取当前浏览器地址栏的信息
4.操作浏览器的滚动条
5.浏览器的信息(浏览器的版本)
6.让浏览器出现一个弹出框(alert/confirm/prompt)
子对象:
- history 子对象 - 历史记录对象 (方法:back() 后退;forward() 前进)
- location 子对象 - 地址栏对象(位置对象)
- document 子对象 - 文档对象 (重点学习)
- navigator 包含浏览器相关信息
- screen 用户显示屏幕相关属性
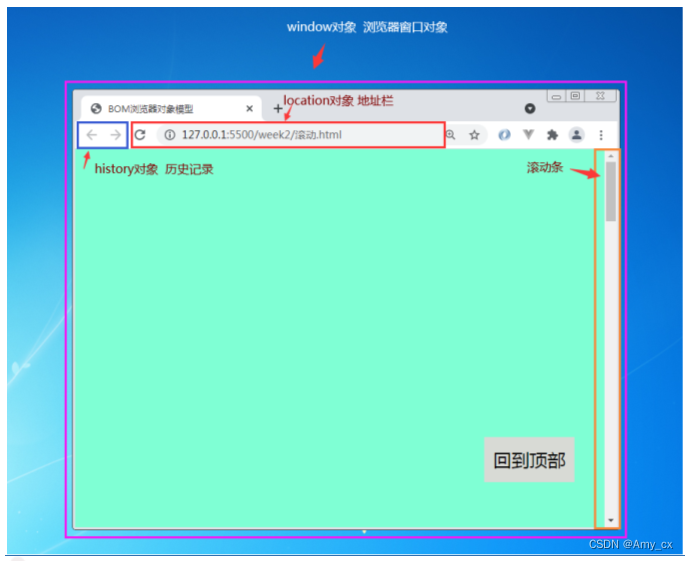
<body>
<h2>首页</h2>
<a href="./detail.html">详情页</a>
<button onclick="history.forward()">前进</button>
</body>
<body>
//detail.html主代码
<h2>详情页</h2>
<button onclick="goBack()">后退</button>
<script>
function goBack(){
history.back()
}
</script>
</body>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<!--
location
位置对象,地址栏对象
刷新 url地址栏输入框
window.location
方法和属性
href属性
location.href
获取当前页面url地址
设置url 跳转url地址对应页面
方法
reload() 刷新页面
-->
</head>
<body>
<button onclick="getUrl()">获取当前页面url地址</button>
<button onclick="setUrl()">跳转url地址对应页面</button>
<button onclick="location.reload()">刷新</button>
<script>
function getUrl(){
var url = location.href
document.write(url)
}
function setUrl(){
location.href='http://www.baidu.com'
}
</script>
</body>
</html>
<body>
<h2>location属性</h2>
<a href="#footer" onclick="test1()">footer</a>
<div id="footer"></div>
<script>
function test1(){
console.log(location.hash)
console.log(location.href)
console.log(location.protocol)
console.log(location.port)
}
</script>
</body>
常用方法:
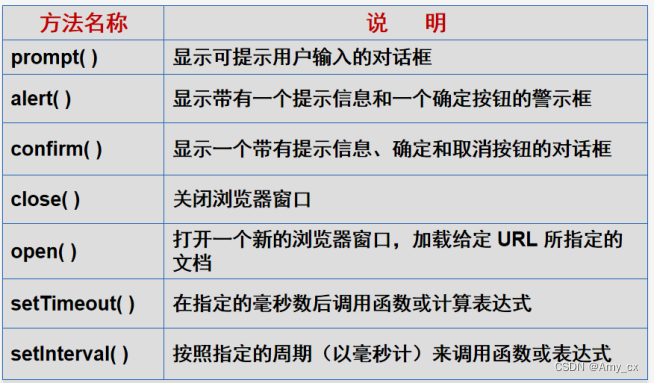
setTimeout() 倒计时定时器
setInterval() 循环执行定时器
示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>window</title>
</head>
<body>
<script>
function test(){
// window.alert('今天是周四!')
var isok = window.confirm('确定删除吗?')
// document.write(isok)
if(isok){
alert('删除成功')
}else{
alert('取消删除')
}
}
// test()
function test2(){
var score = window.prompt('请输入成绩')
var newScore = score - 10 // +号会隐式类型转换,字符串拼接
alert(newScore)
}
// test2()
function test3(){
window.open('http://www.baidu.com','百度','')
}
// test3()
function test4(){
var timer = window.setTimeout(function(){
//定时器执行代码
document.write('today is better')
},1000)
}
// test4()
function test5(){
var n=5
var timer = window.setInterval(function(){
document.write(n--)
// console.log(n--)
if(n == 0){
clearInterval(timer)
}
},1000)
}
test5()
</script>
</body>
</html>
五、浏览器窗口尺寸
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>浏览器窗口尺寸</title>
<!-- innerHeight
innerWidth -->
</head>
<body>
<button onclick="getSize()">获取尺寸</button>
<script>
function getSize(){
var h = window.innerHeight//高
var w = window.innerWidth //宽
document.write(`height: ${h}, width: ${w}` )
}
</script>
</body>
</html>
效果展示:
六、onscroll事件
当浏览器的滚动条滚动或鼠标滚轮滚动时触发。
前提:页面高度超过浏览器的可视窗口。
其实浏览器并没有动,而是页面在向上走。所以不能单纯算是浏览器的内容,而是页面的内容,所以不是用window对象,而是用document对象。
scrollTop 获取的是页面向上滚动的距离。两个获取方式:document.documentElement.scrollrop和document.body.scrollTop
- IE浏览器
- 没有DOCTYPE声明的时候,用这两个都行
- 有DOCTYPE声明的时候,只能用document.documentElement.scrollrop
- Chrome和Firefox
- 没有DOCTYPE声明的时候,用document.body.scrollTop
- 有DOCTYPE声明的时候,用document.documentElement.scrollrop
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>滚动事件属性</title>
<style>
div{
height: 1000px;
}
</style>
</head>
<body>
<h2>滚动事件属性</h2>
<div></div>
<script>
//浏览器滚动条滚动时执行函数代码 获取滚动距离
window.onscroll =function(){
// console.log('scrollTop :', document.documentElement.scrollTop )
// console.log('body :', document.body.scrollTop )
var scrollTop = getScollTop()
console.log(scrollTop)
}
function getScollTop(){
//逻辑或,前面有值(true,文档声明)直接返回,否则返回后面的值
return document.documentElement.scrollrop || document.body.scrollTop
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>回到顶部</title>
<style>
*{
padding: 0;
margin: 0;
}
div img{
max-width: 100%;
}
button{
width: 80px;
height: 30px;
position: fixed;
bottom: 30px;
right: 30px;
}
</style>
</head>
<body>
<div>
<img src="./jingdong.png" alt="" />
</div>
<button onclick="backTop()">^回到顶部</button>
<script>
function backTop(){
var timer = setInterval(function () {
var height = document.documentElement.scrollTop
document.documentElement.scrollTop = height - 100
if(height <= 0){
console.log('结束',height)
clearInterval(timer)
}
},100)
}
</script>
</body>
</html>
效果展示: