一、前言
- 在 iOS 16 中,Apple 引入了三种新的宽度样式字体到 SF 字体库:Compressed、Condensed、Expend,展示效果如下:
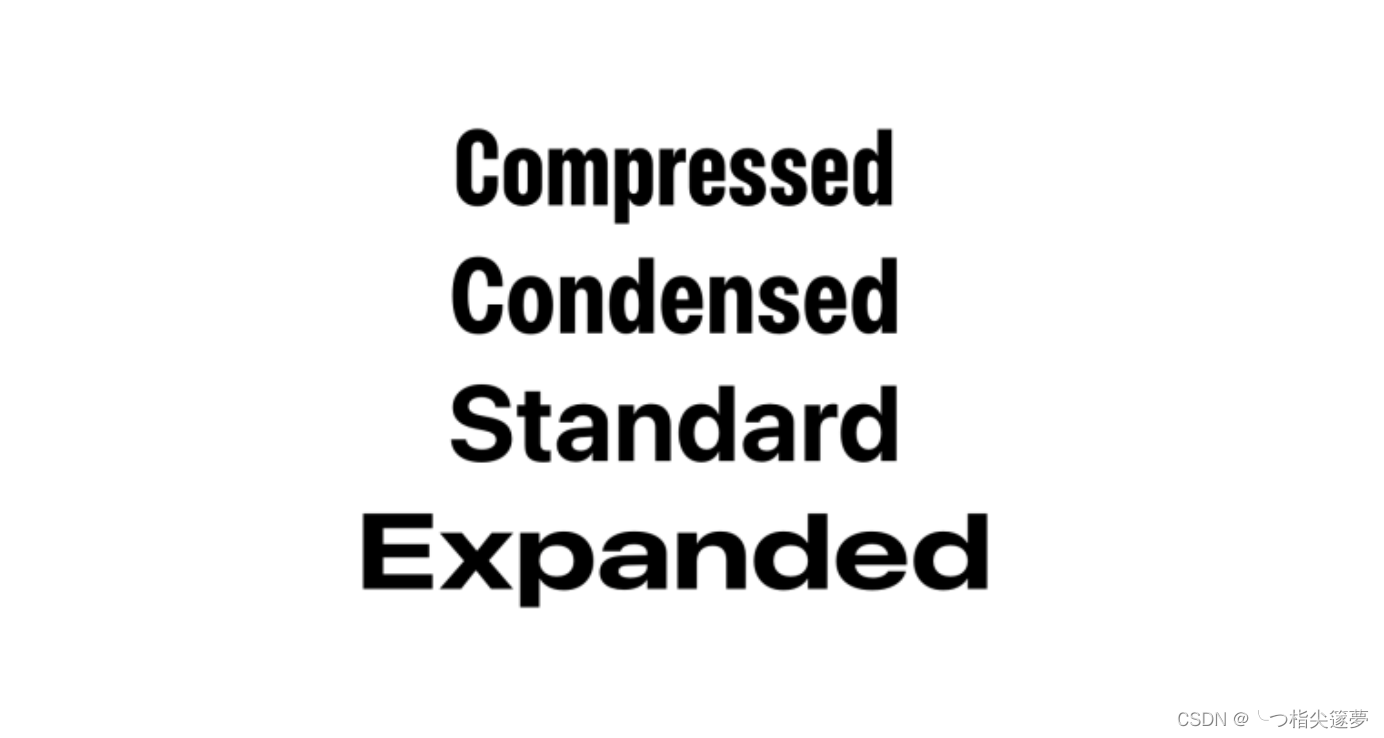
二、UIFont.Width
- Apple 引入了新的结构体 UIFont.Width,这代表了一种新的宽度样式。目前已有的四种样式:
-
-
-
- condensed:介于压缩和标准之间的宽度样式;
-
- 其效果如下:
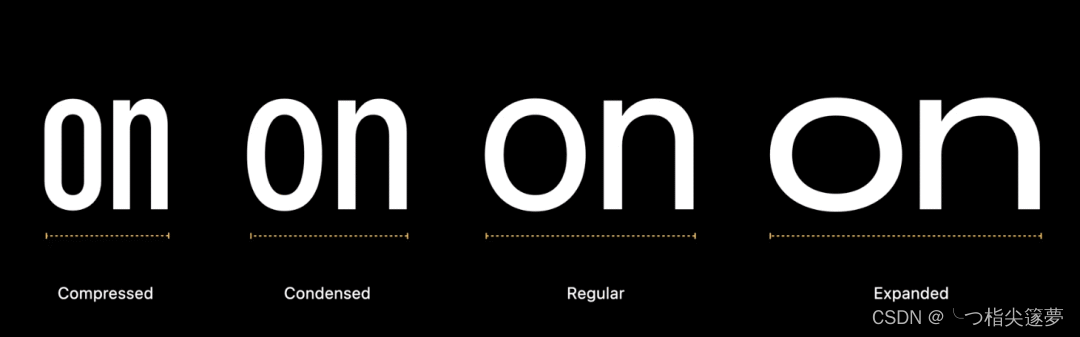
三、SF 字体和新的宽度样式
- 如何将 SF 字体和新的宽度样式一起使用呢?为了使用新的宽度样式,Apple 有一个新的 UIFont 的类方法来接收新的 UIFont.Width:
class UIFont : NSObject {
class func systemFont(
ofSize fontSize: CGFloat,
weight: UIFont.Weight,
width: UIFont.Width
) -> UIFont
}
let condensed = UIFont.systemFont(ofSize: 46, weight: .bold, width: .condensed)
let compressed = UIFont.systemFont(ofSize: 46, weight: .bold, width: .compressed)
let standard = UIFont.systemFont(ofSize: 46, weight: .bold, width: .standard)
let expanded = UIFont.systemFont(ofSize: 46, weight: .bold, width: .expanded)
四、SwiftUI 如何使用新宽度样式?
- 在 Xcode 14.1 中,SwiftUI 提供了两个新的 API 设置这种新的宽度样式:width( _: ) 和 fontWidth( _: )。目前在 iOS 16 中,这种新的宽度样式和初始值设定只能在 UIKit 中使用,幸运的是,可以在 SwiftUI 中轻松的使用它。
- 有很多种方法可以将 UIKit 集成到 SwiftUI,接下来展示在 SwiftUI 中使用新宽度样式的两种方法:
-
-
① 将 UIFont 转为 Font
- 我们知道,将 UIFont 转换到字体,可以使用 init(_ font: CTFont) 初始化器将 UIKit UIFont 转换为 SwiftUI Font。初始化器接收 CTFont 作为参数,CTFont 是一种不透明的类型,代表字体对象。
- NSFont 和 UIFont 都可以自由与 CTFont 连接,如下是将 UIKit UIFont 转换为 SwiftUI Font 示例:
let uiFont = UIFont.systemFont(ofSize: 18, weight: .bold)
let font = Font(uiFont)
- 至于如何将字体转换到 UIFont 呢?虽然可以轻松地将 UIFont 转换为 Font,但是不能反过来,因为 UIFont 比 Font 包含更多的灵活性和方法,可以用 Font 做的任何事情,都可能在 UIFont 中找到一种等效的方法。
- 因此,Font 有初始化方法可以接收 UIFont 作为参数,创建带有新宽度样式的 UIFont,用 UIFont 初始化 Font, 然后传递给 .font 修改。步骤如下:
-
-
-
struct NewFontExample: View {
let condensed = UIFont.systemFont(ofSize: 46, weight: .bold, width: .condensed)
let compressed = UIFont.systemFont(ofSize: 46, weight: .bold, width: .compressed)
let standard = UIFont.systemFont(ofSize: 46, weight: .bold, width: .standard)
let expanded = UIFont.systemFont(ofSize: 46, weight: .bold, width: .expanded)
var body: some View {
VStack {
Text("Compressed")
.font(Font(compressed))
Text("Condensed")
.font(Font(condensed))
Text("Standard")
.font(Font(standard))
Text("Expanded")
.font(Font(expanded))
}
}
}
② 创建一个 Font 扩展
- 这种方法实际上和将 UIfont 转为 Font 是同一种方法,只需要创建一个新的 Font 扩展在 SwiftUI 中使用起来更容易一些:
extension Font {
public static func system(
size: CGFloat,
weight: UIFont.Weight,
width: UIFont.Width) -> Font
{
// 1
return Font(
UIFont.systemFont(
ofSize: size,
weight: weight,
width: width)
)
}
}
- 创建一个静态函数传递 UIFont 需要的参数,然后初始化 UIFont 和创建 Font,就可以像这样使用:
Text("Compressed")
.font(.system(size: 46, weight: .bold, width: .compressed))
Text("Condensed")
.font(.system(size: 46, weight: .bold, width: .condensed))
Text("Standard")
.font(.system(size: 46, weight: .bold, width: .standard))
Text("Expanded")
.font(.system(size: 46, weight: .bold, width: .expanded))
③ 如何使用新的宽度样式?
- 可以在想使用的任何地方使用,不会有任何限制,所有的新宽度都有一样的尺寸,同样的高度,只会有宽度的变化。
- 如下是拥有同样文本,同样字体大小和同样字体样式的不同字体宽度样式展示:
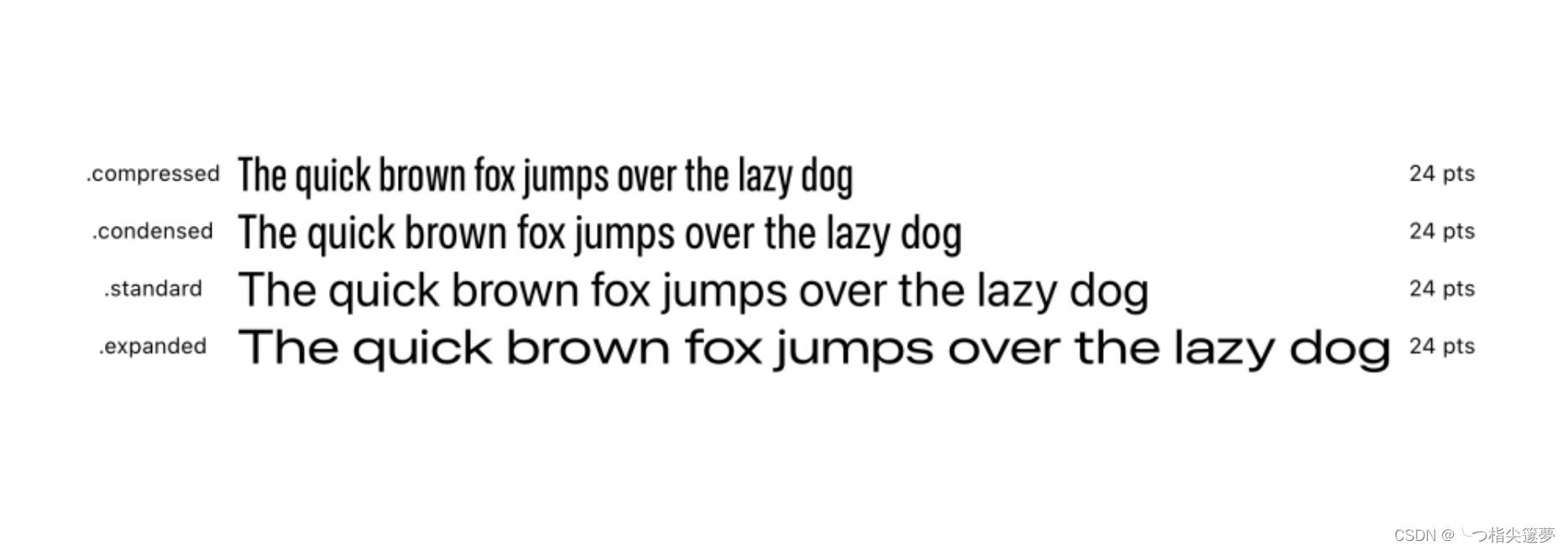
④ 新的宽度样式优点
- 可以使用新的宽度样式在已经存在的字体样式上,比如 thin 或者 bold ,在 App 上创造出独一无二的体验。Apple 将它使用在它们的照片 App ,在“回忆”功能中,通过组合不同的字体宽度和样式在标题或者子标题上。如下所示,有一些不同宽度和样式的字体组合:
Text("Pet Friends")
.font(Font(UIFont.systemFont(ofSize: 46, weight: .light, width: .expanded)))
Text("OVER THE YEARS")
.font(Font(UIFont.systemFont(ofSize: 30, weight: .thin, width: .compressed)))
Text("Pet Friends")
.font(Font(UIFont.systemFont(ofSize: 46, weight: .black, width: .condensed)))
Text("OVER THE YEARS")
.font(Font(UIFont.systemFont(ofSize: 20, weight: .light, width: .expanded)))
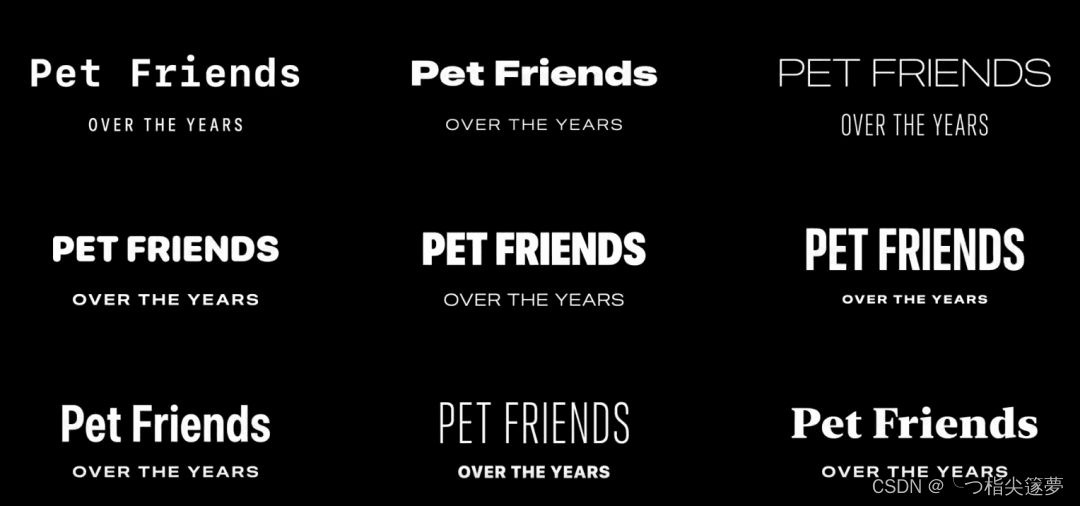
- 也可以用新的宽度样式来控制文本的可读性,如所示,说明了不同宽度样式如何影响每行的字符数和段落长度:
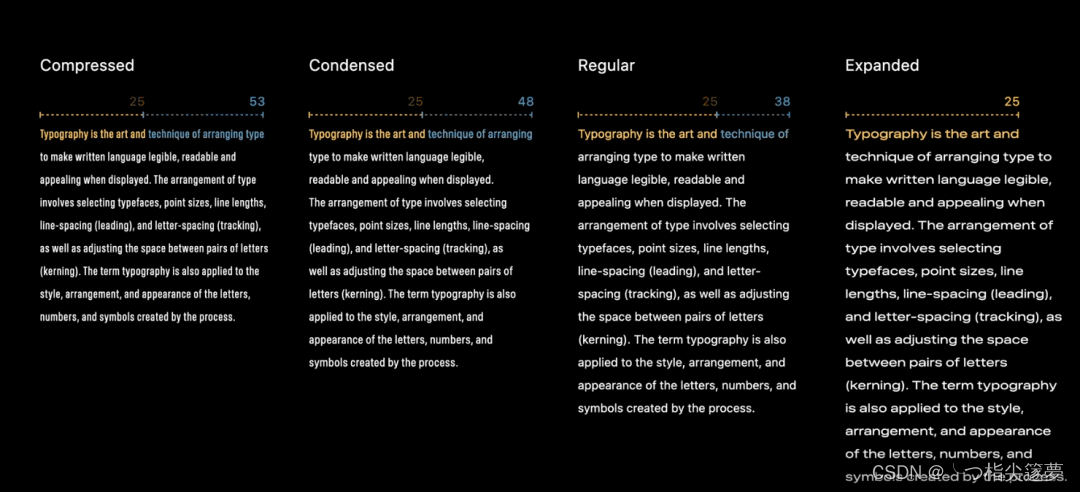
五、下载字体
- 可以在 Apple 字体平台来下载这种新的字体宽度样式,下载安装后,将会发现一种结合了现有宽度和新宽度样式的新样式:
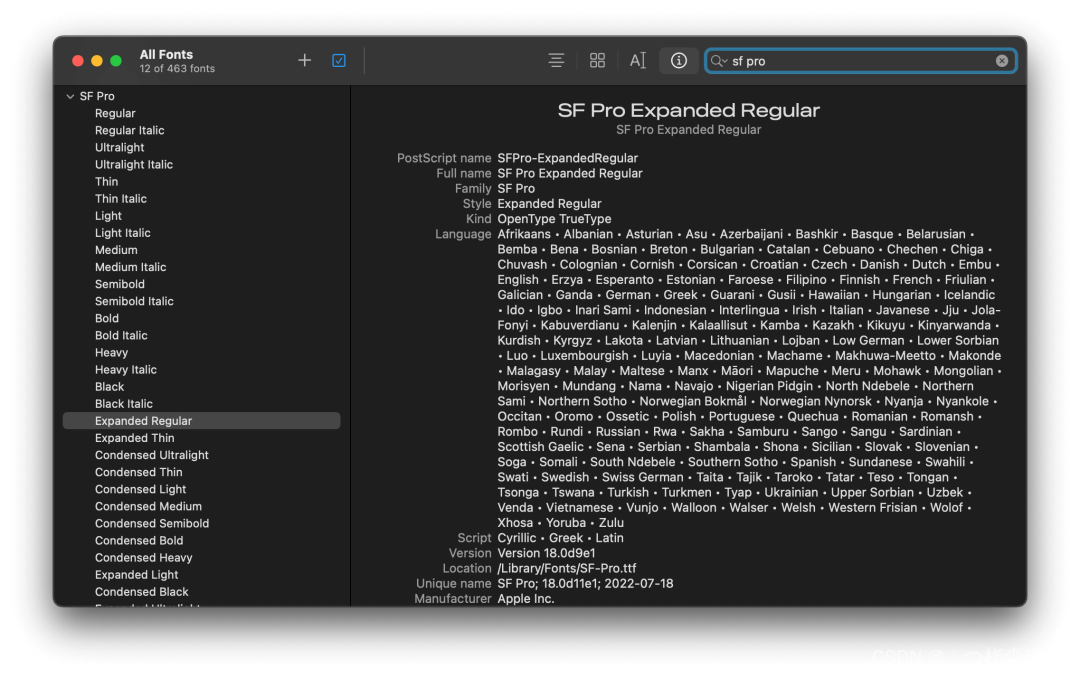
- 需要注意的是,基本上除了在模拟器的模拟系统 UI 中,在任何地方都被禁止使用 SF 字体,因此需要确保在使用前阅读并理解许可证。