1.引入pom依赖
<!--引入springboot父工程依赖-->
<!--引入依赖作用:
可以省去version标签来获得一些合理的默认配置
-->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.2.RELEASE</version>
</parent>
<!--因为用eureka,是springCloud的,所以引入springCloud版本锁定-->
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<!--spring cloud 版本-->
<spring-cloud.version>Hoxton.SR10</spring-cloud.version>
</properties>
<!--引入Spring Cloud 依赖-->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<!--引入提供Web开发场景依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--@LoadBalanced
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-commons</artifactId>
</dependency>
<!--用@Data 减少JavaBean get...set...方法-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!--用于hutool工具类 对象转json字符串-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-json</artifactId>
<version>4.1.21</version>
</dependency>
<!--两个用来做测试的jar包-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
</dependency>
</dependencies>
2.配置启动类并 注入RestTemplate实体
- @LoadBalanced : 负载均衡
通过服务名调用则可以使用个负载均衡(例如:http://MHH/test?age=12)
服务的 IP 和 Port,并把它拼接起来组合成的服务地址,没法实现客户端的负载均衡,会报错。
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
public class RestTemplateApplication {
public static void main(String[] args) {
SpringApplication.run(RestTemplateApplication.class);
}
@Bean
RestTemplate restTemplate() {
return new RestTemplate();
}
}
3. RestTemplate 简单常用使用示例
3.1 入参为空的请求方式
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping("isEmpty")
public String isEmpty() {
return "已通过并返回:-->isEmpty()方法";
}
}
入参为空的请求方式测试结果:
- exchange(RequestEntity<?> requestEntity, Class responseType)
responseType : 返回对象类型
RequestEntity(HttpMethod method, URI url):
method:请求方式(POST/GET…)
url : 请求路径 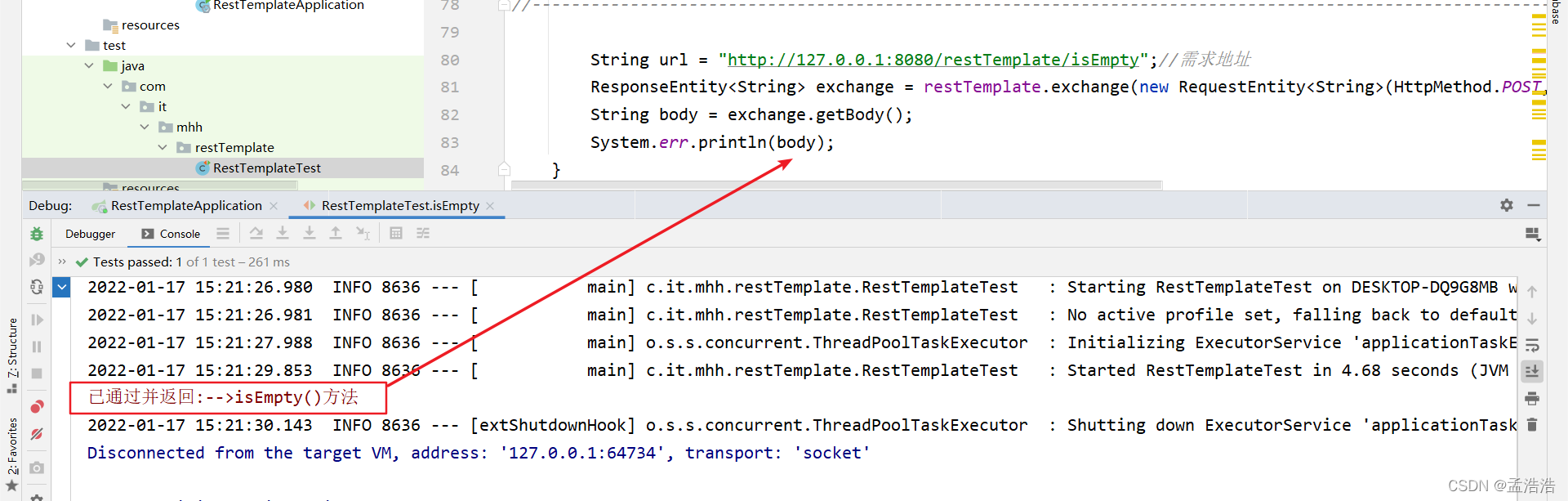
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void isEmpty() throws URISyntaxException {
String url = "http://127.0.0.1:8080/restTemplate/isEmpty";
ResponseEntity<String> exchange = restTemplate.exchange(new RequestEntity<String>(HttpMethod.POST, new URI(url)), String.class);
String body = exchange.getBody();
System.err.println(body);
}
}
3.2 入参为@RequestParam() 请求方式
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@GetMapping("requestParam")
public String requestParam(@RequestParam("id") String id, @RequestParam(value = "age", defaultValue = "1") Integer age) {
return "已通过并返回:-->requestParam方法入参 id为: " + id + " age为: " + age;
}
}
入参为@RequestParam() 请求方式测试结果:
- exchange(RequestEntity<?> requestEntity, Class responseType)
responseType : 返回对象类型
RequestEntity(HttpMethod method, URI url):
method:请求方式(POST/GET…)
url : 请求路径
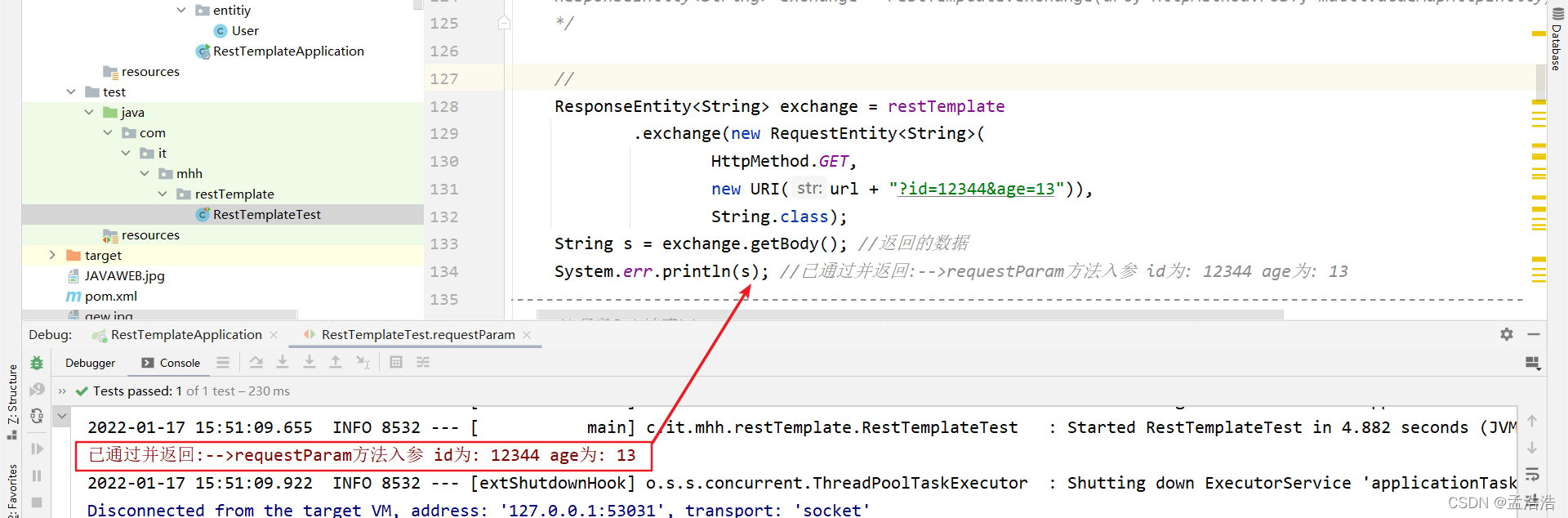
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void requestParam() throws URISyntaxException {
String url = "http://127.0.0.1:8080/restTemplate/requestParam";
ResponseEntity<String> exchange = restTemplate.exchange(new RequestEntity<String>(HttpMethod.GET, new URI(url + "?id=12344&age=13")), String.class);
String s = exchange.getBody();
System.err.println(s);
}
}
3.3 入参为@RequestBody 请求方式
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping("requestBody")
public Map<String, Object> requestBody(@RequestBody Map<String, Object> map) {
return map;
}
}
入参为@RequestBody 请求方式测试结果:
- exchange(String url, HttpMethod method, @Nullable HttpEntity<?> requestEntity, Class responseType, Object… uriVariables)
url: 请求路径
method:请求方式(POST/GET…)
requestEntity : 给对方传递的的数据
responseType :返回对象类型
uriVariables : 表示 url地址上传递的值(适用于restful风格)
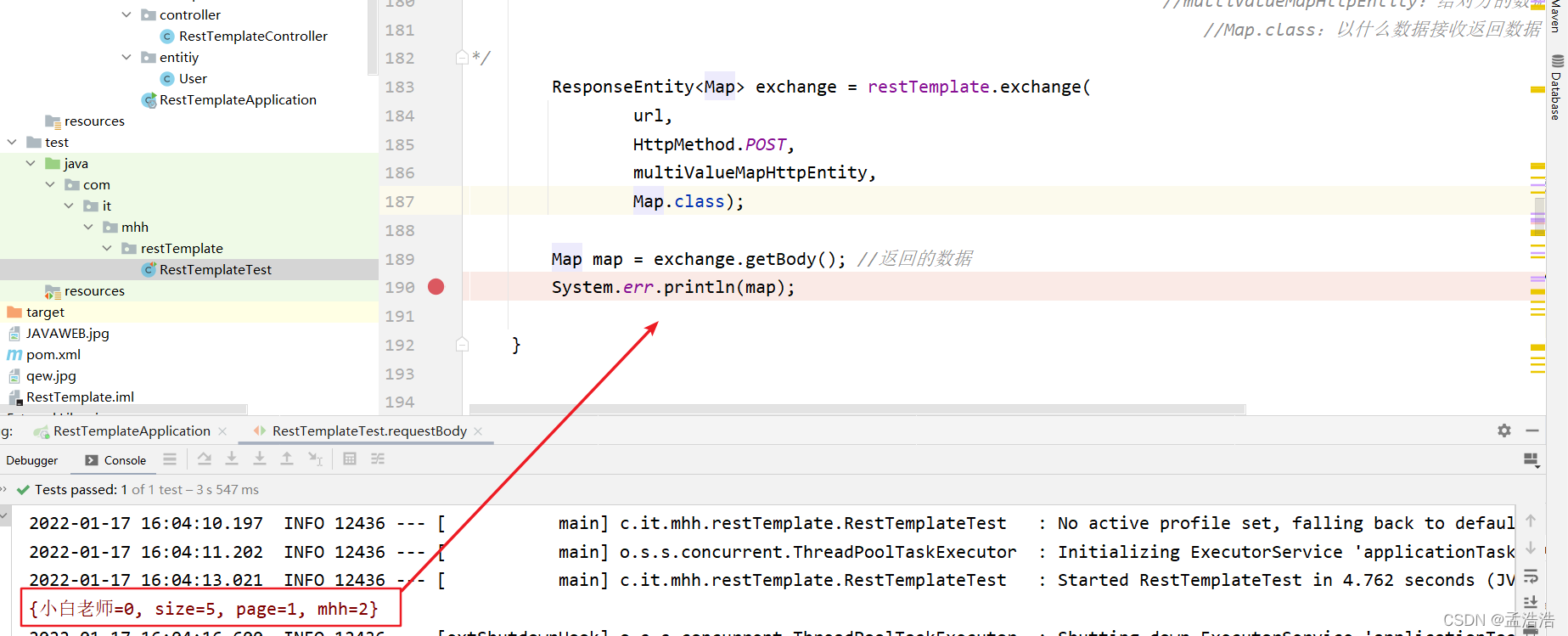
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void requestBody() {
String url = "http://127.0.0.1:8080/restTemplate/requestBody";
MultiValueMap<String, String> headers = new LinkedMultiValueMap<String, String>();
headers.add("content-type", "application/json");
Map<String, Object> body = new HashMap();
body.put("page", 1);
body.put("size", 5);
body.put("mhh", "2");
body.put("小白老师", 0);
HttpEntity<String> multiValueMapHttpEntity = new HttpEntity<String>(JSONUtil.toJsonStr(body), headers);
restTemplate.setErrorHandler(new DefaultResponseErrorHandler() {
@Override
public void handleError(ClientHttpResponse response) throws IOException {
if (response.getRawStatusCode() != 400 && response.getRawStatusCode() != 401) {
super.handleError(response);
}
}
});
ResponseEntity<Map> exchange = restTemplate.exchange(url, HttpMethod.POST, multiValueMapHttpEntity, Map.class);
Map map = exchange.getBody();
System.err.println(map);
}
}
3.4 入参为@RequestParam()与@RequestBody 请求方式
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping("requestBodyAndRequestParam")
public Map<String, Object> requestBody(@RequestParam("name") String name, @RequestBody Map<String, Object> map) {
map.put("name", name);
return map;
}
}
入参为@RequestParam()与@RequestBody 请求方式测试结果:
- exchange(String url, HttpMethod method, @Nullable HttpEntity<?> requestEntity, Class responseType, Object… uriVariables)
url: 请求路径
method:请求方式(POST/GET…)
requestEntity : 给对方传递的的数据
responseType :返回对象类型
uriVariables : 表示 url地址上传递的值(适用于restful风格)
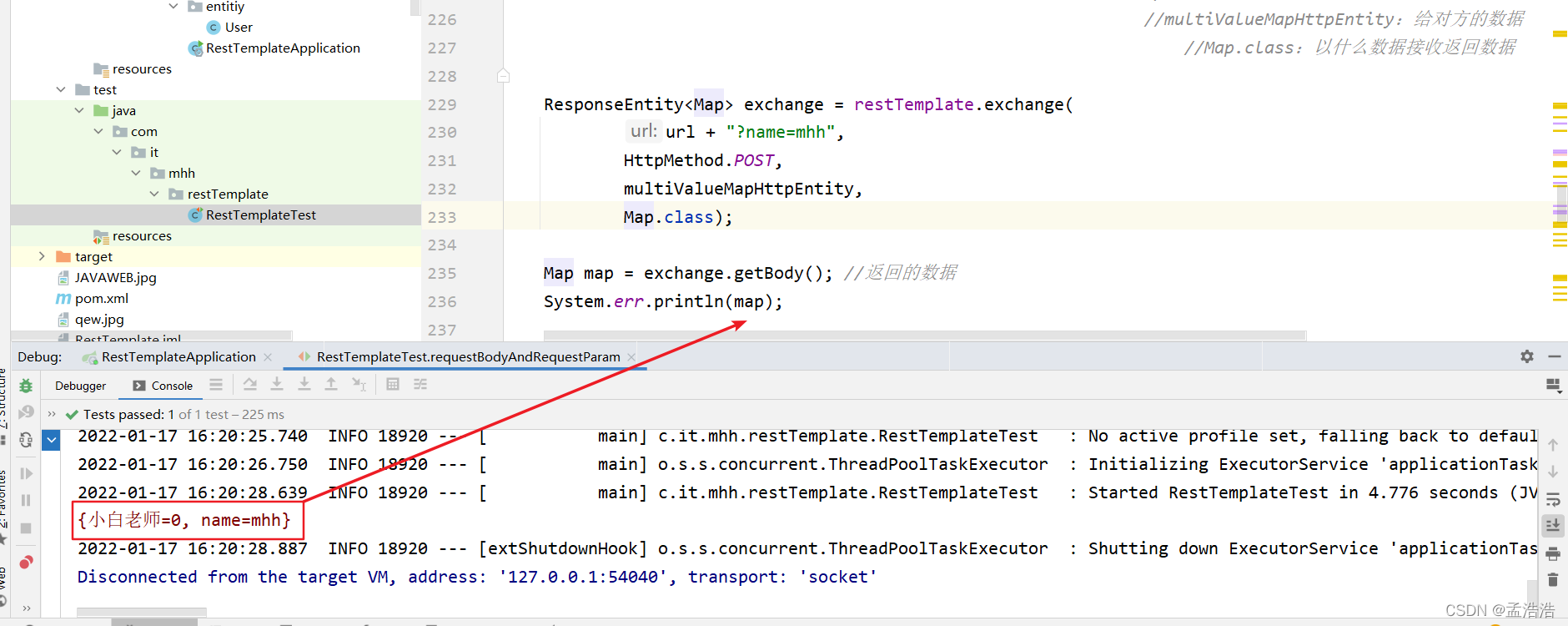
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void requestBodyAndRequestParam() {
String url = "http://127.0.0.1:8080/restTemplate/requestBodyAndRequestParam";
MultiValueMap<String, String> headers = new LinkedMultiValueMap<String, String>();
headers.add("content-type", "application/json");
Map<String, Object> body = new HashMap();
body.put("小白老师", 0);
HttpEntity<String> multiValueMapHttpEntity = new HttpEntity<String>(JSONUtil.toJsonStr(body), headers);
restTemplate.setErrorHandler(new DefaultResponseErrorHandler() {
@Override
public void handleError(ClientHttpResponse response) throws IOException {
if (response.getRawStatusCode() != 400 && response.getRawStatusCode() != 401) {
super.handleError(response);
}
}
});
ResponseEntity<Map> exchange = restTemplate.exchange(url + "?name=mhh", HttpMethod.POST, multiValueMapHttpEntity, Map.class);
Map map = exchange.getBody();
System.err.println(map);
}
}
3.5 入参为@PathVariable 请求方式
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping("{name}/restFul/{age}")
public String restFul(@PathVariable("name")String name,@PathVariable("age")Integer age){
return "已通过并返回:-->restFul方法入参 name为: " + name + " age为: " + age;
}
}
入参为@PathVariable 请求方式测试结果:
- T postForObject(String url, @Nullable Object request, Class responseType, Object… uriVariables)
url: 请求路径
request: 给对方传递的的数据
responseType :返回对象类型
uriVariables : 表示 url地址上传递的值(适用于restful风格)
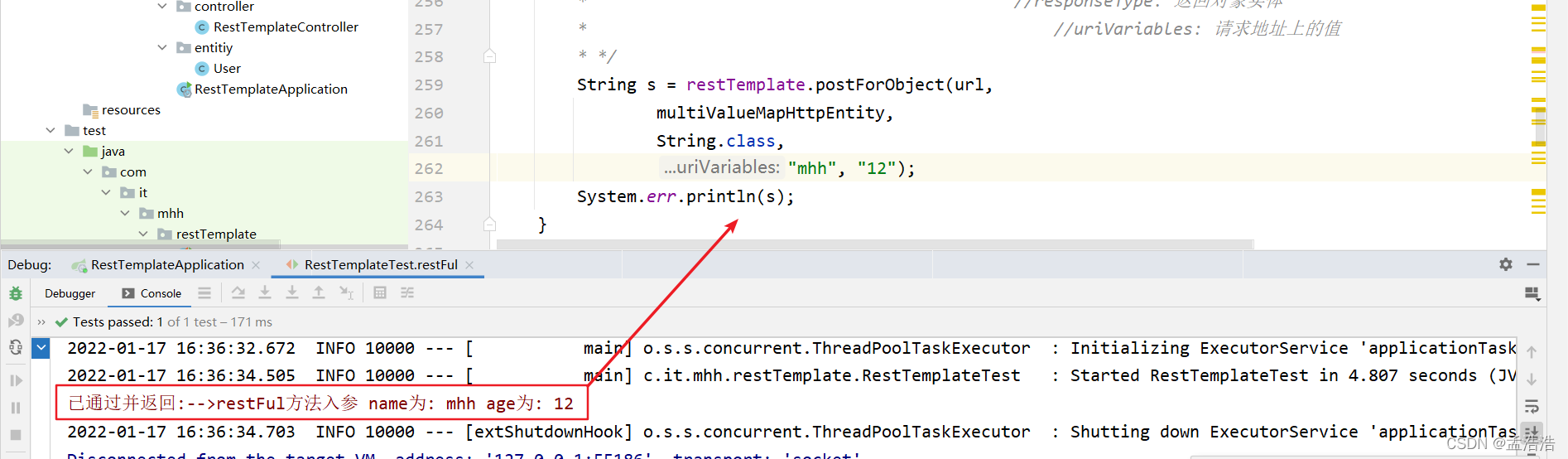
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void restFul() {
String url = "http://127.0.0.1:8080/restTemplate/{name}/restFul/{age}";
MultiValueMap<String, Object> body = new LinkedMultiValueMap<String, Object>();
HttpEntity<MultiValueMap<String, Object>> multiValueMapHttpEntity = new HttpEntity<MultiValueMap<String, Object>>(body);
String s = restTemplate.postForObject(url, multiValueMapHttpEntity, String.class, "mhh", "12");
System.err.println(s);
}
}
3.6 入参为MultipartFile 附件 请求方式
- 入参为附件 请求头headers 必须设置 “content-type=multipart/form-data”
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping(value = "multipartFile", headers = "content-type=multipart/form-data")
public void multipartFile(@RequestParam("multipartFile") MultipartFile multipartFile) {
try {
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(new FileOutputStream(Objects.requireNonNull(multipartFile.getOriginalFilename())));
InputStream inputStream = multipartFile.getInputStream();
int len;
byte[] bytes = new byte[1024];
while ((len = inputStream.read(bytes)) != -1) {
bufferedOutputStream.write(bytes, 0, len);
bufferedOutputStream.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
application.yml配置
spring:
servlet:
multipart:
max-file-size: 10MB
max-request-size: 10MB
入参为MultipartFile 附件 请求方式测试结果:
- ResponseEntity exchange(String url, HttpMethod method, @Nullable HttpEntity<?> requestEntity, Class responseType, Object… uriVariables)
url: 请求路径
method: 请求方式(POST/GET…)
requestEntity: 给对方传递的的数据
responseType:返回对象类型
uriVariables: 表示 url地址上传递的值(适用于restful风格)
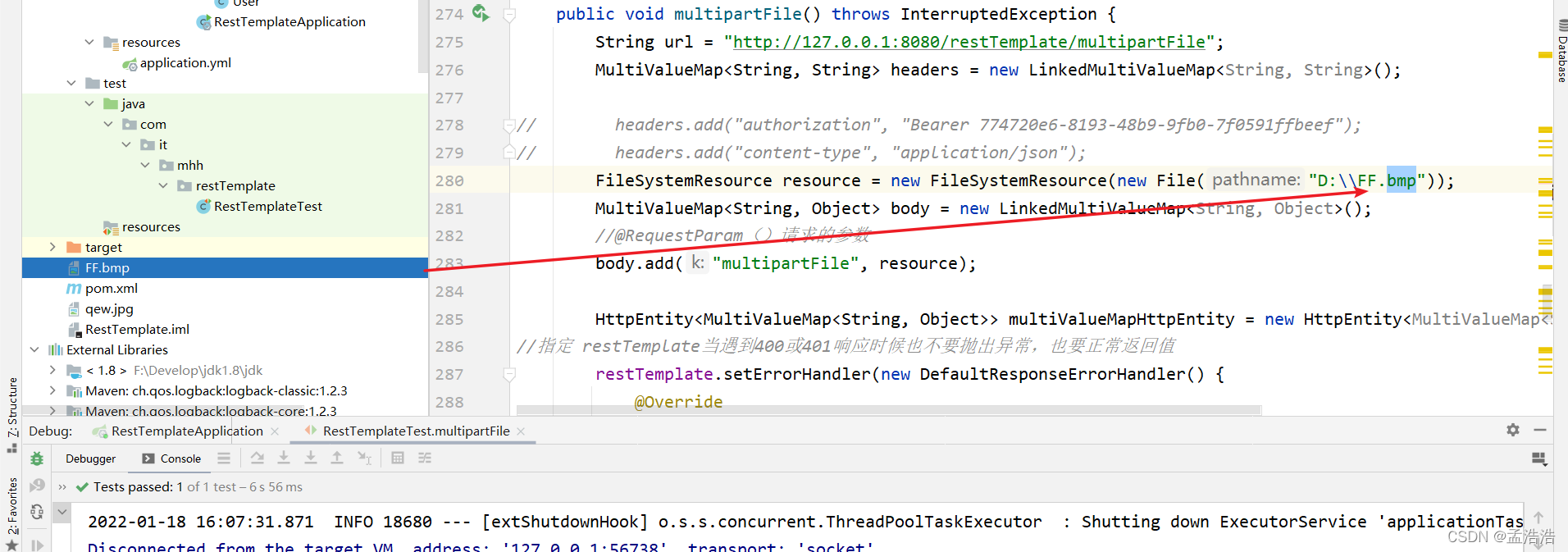
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void multipartFile() {
String url = "http://127.0.0.1:8080/restTemplate/multipartFile";
MultiValueMap<String, String> headers = new LinkedMultiValueMap<String, String>();
FileSystemResource resource = new FileSystemResource(new File("D:\\FF.bmp"));
MultiValueMap<String, Object> body = new LinkedMultiValueMap<String, Object>();
body.add("multipartFile", resource);
HttpEntity<MultiValueMap<String, Object>> multiValueMapHttpEntity = new HttpEntity<MultiValueMap<String, Object>>(body, headers);
restTemplate.setErrorHandler(new DefaultResponseErrorHandler() {
@Override
public void handleError(ClientHttpResponse response) throws IOException {
if (response.getRawStatusCode() != 400 && response.getRawStatusCode() != 401) {
super.handleError(response);
}
}
});
ResponseEntity<Object> exchange = restTemplate.exchange(url, HttpMethod.POST, multiValueMapHttpEntity, Object.class);
Object o = exchange.getBody();
System.out.println(o);
}
}
3.7 入参为javaBean对象且内部有 MultipartFile属性 请求方式
- 入参有附件 请求头headers 必须设置 “content-type=multipart/form-data”
- 请求头为content-type=multipart/form-data 不能使用 @RequestBody注解接收
- @RequestBody 注解标识,通常会用application/json, application/xml处理content-type
import com.it.mhh.restTemplate.entitiy.User;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Map;
import java.util.Objects;
@RestController
@RequestMapping("restTemplate")
public class RestTemplateController {
@PostMapping(value = "multipartFile", headers = "content-type=multipart/form-data")
public void multipartFile(@RequestParam("multipartFile") MultipartFile multipartFile) {
try {
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(new FileOutputStream(Objects.requireNonNull(multipartFile.getOriginalFilename())));
InputStream inputStream = multipartFile.getInputStream();
int len;
byte[] bytes = new byte[1024];
while ((len = inputStream.read(bytes)) != -1) {
bufferedOutputStream.write(bytes, 0, len);
bufferedOutputStream.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
application.yml配置
spring:
servlet:
multipart:
max-file-size: 10MB
max-request-size: 10MB
javaBean User对象配置
import lombok.Data;
import org.springframework.web.multipart.MultipartFile;
@Data
public class User {
private String name;
private MultipartFile multipartFile;
}
入参为javaBean对象且内部有 MultipartFile属性 请求方式 测试结果:
- ResponseEntity exchange(String url, HttpMethod method, @Nullable HttpEntity<?> requestEntity, Class responseType, Object… uriVariables)
url: 请求路径
method: 请求方式(POST/GET…)
requestEntity: 给对方传递的的数据
responseType:返回对象类型
uriVariables: 表示 url地址上传递的值(适用于restful风格)
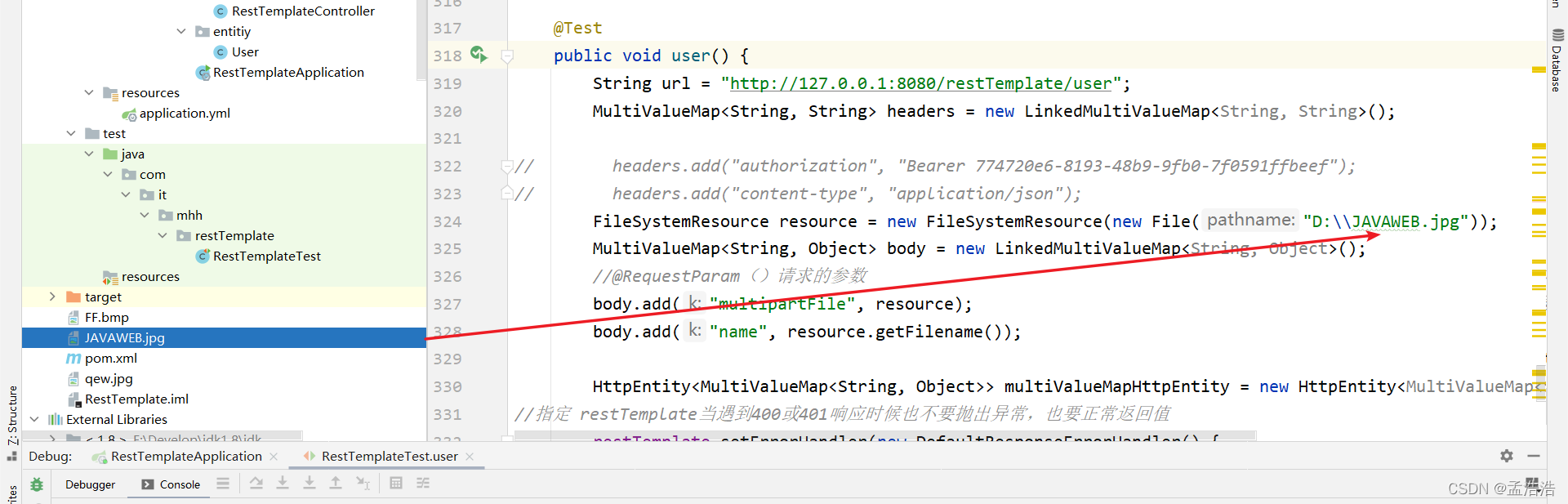
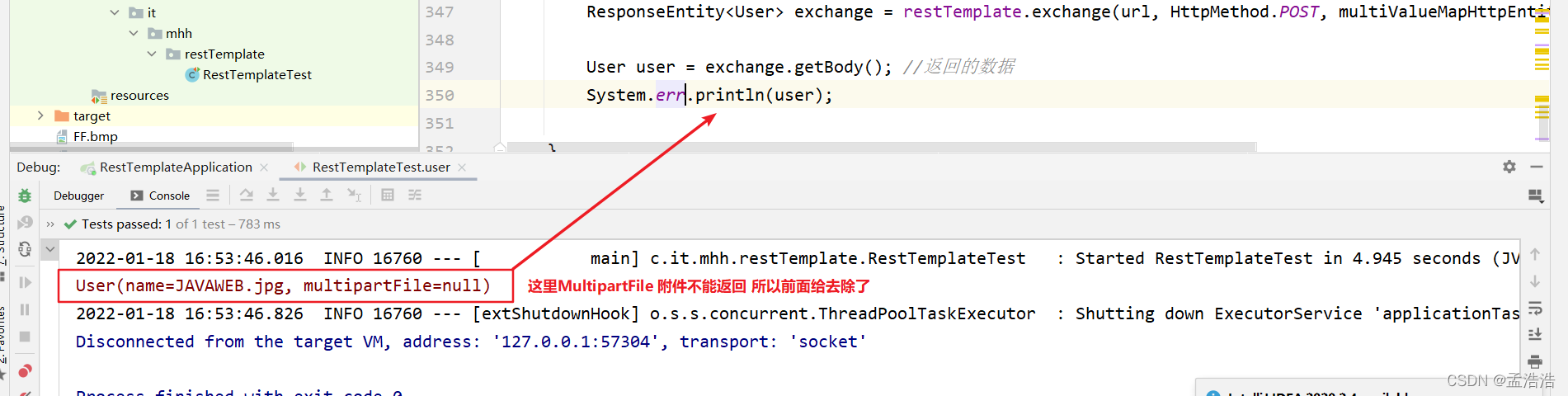
import cn.hutool.json.JSONUtil;
import com.it.mhh.restTemplate.entitiy.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.RequestEntity;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.ClientHttpResponse;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.DefaultResponseErrorHandler;
import org.springframework.web.client.RestTemplate;
import java.io.File;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.Map;
@SpringBootTest
@RunWith(SpringRunner.class)
public class RestTemplateTest {
@Autowired
RestTemplate restTemplate;
@Test
public void user() {
String url = "http://127.0.0.1:8080/restTemplate/user";
MultiValueMap<String, String> headers = new LinkedMultiValueMap<String, String>();
FileSystemResource resource = new FileSystemResource(new File("D:\\JAVAWEB.jpg"));
MultiValueMap<String, Object> body = new LinkedMultiValueMap<String, Object>();
body.add("multipartFile", resource);
body.add("name", resource.getFilename());
HttpEntity<MultiValueMap<String, Object>> multiValueMapHttpEntity = new HttpEntity<MultiValueMap<String, Object>>(body, headers);
restTemplate.setErrorHandler(new DefaultResponseErrorHandler() {
@Override
public void handleError(ClientHttpResponse response) throws IOException {
if (response.getRawStatusCode() != 400 && response.getRawStatusCode() != 401) {
super.handleError(response);
}
}
});
ResponseEntity<User> exchange = restTemplate.exchange(url, HttpMethod.POST, multiValueMapHttpEntity, User.class);
User user = exchange.getBody();
System.err.println(user);
}
}