封装一个学生类(Student):包括受保护成员:姓名、年龄、分数,完成其相关函数,以及show
在封装一个领导类(Leader):包括受保护成员:岗位、工资,完成其相关函数及show
由以上两个类共同把派生出学生干部类:引入私有成员:管辖人数,完成其相关函数以及show函数
在主程序中,测试学生干部类:无参构造、有参构造、拷贝构造、拷贝赋值、show
头文件
#include <iostream>
#include <header.h>
using namespace std;
int main()
{
//无参构造
cout<<"******************无参构造******************"<<endl;
Student_cadre s1;
s1.show();
//有参构造
cout<<"******************有参构造******************"<<endl;
Student_cadre s2("chenx",18,99.5,"ccc",10000,9);
s2.show();
//拷贝构造
cout<<"******************拷贝构造******************"<<endl;
Student_cadre s3 = s2;
s3.show();
//拷贝赋值
cout<<"******************拷贝赋值******************"<<endl;
Student_cadre s4;
s4 = s2;
s4.show();
return 0;
}
源文件
#include <iostream>
#include <header.h>
using namespace std;
//无参构造
Stu::Stu(){
cout << "stu:: 无参构造" << endl;
}
//有参构造
Stu::Stu(string n, int a, double s):name(n), age(a), score(s)
{
cout<<"Stu::有参构造"<<endl;
}
//析构函数
Stu::~Stu()
{
cout<<"Stu::析构函数"<<endl;
}
//定义拷贝构造函数
Stu::Stu(const Stu &other):name(other.name), age(other.age), score(other.score)
{
cout<<"Stu::拷贝构造函数"<<endl;
}
//定义拷贝赋值函数
Stu&Stu::operator=(const Stu &other)
{
this->name = other.name;
this->age = other.age;
this->score = other.score;
cout<<"Stu::拷贝赋值函数"<<endl;
return *this;
}
void Stu::show()
{
cout<<"name = "<<name<<endl;
cout<<"age = "<<age<<endl;
cout<<"score = "<<score<<endl;
}
//无参构造
Leader::Leader() {cout<<"Leader::无参构造"<<endl;}
//有参构造
Leader::Leader(string j,double w):job(j),wage(w)
{
cout<<"Leader::有参构造"<<endl;
}
//析构函数
Leader::~Leader()
{
cout<<"Leader::析构函数"<<endl;
}
//拷贝构造函数
Leader::Leader(const Leader &other):job(other.job),wage(other.wage)
{
cout<<"Lerder::拷贝构造函数"<<endl;
}
//拷贝赋值函数
Leader &Leader::operator=(const Leader &other)
{
if(this!=&other)
{
this->job=other.job;
this->wage=other.wage;
}
cout<<"Lerder::拷贝赋值函数"<<endl;
return *this;
}
void Leader::show()
{
cout<<"job = "<<job<<endl;
cout<<"wage = "<<wage<<endl;
}
//无参构造
Student_cadre::Student_cadre() {cout<<"Student_cadre::无参构造"<<endl;}
//有参构造
Student_cadre::Student_cadre(string n,int a,double s,string j,double w,int p):Stu(n,a,s),Leader(j,w),people(p)
{
cout<<"Student_cadre::有参构造"<<endl;
}
//拷贝构造函数
Student_cadre::Student_cadre(const Student_cadre &other):Stu(other),Leader(other),people(other.people)
{
cout<<"Student_cadre::拷贝构造函数"<<endl;
}
//拷贝赋值函数
Student_cadre &Student_cadre:: operator=(const Student_cadre &other)
{
if(this!=&other)
{
this->people=other.people;
Stu:: operator =(other);
Leader:: operator=(other);
}
cout<<"Student_cadre::拷贝赋值函数"<<endl;
return *this;
}
Student_cadre::~Student_cadre()
{
cout<<"Student_cadre::析构函数"<<endl;
}
void Student_cadre::show()
{
cout<<"name = "<<name<<endl;
cout<<"age = "<<age<<endl;
cout<<"score = "<<score<<endl;
cout<<"job = "<<job<<endl;
cout<<"wage = "<<wage<<endl;
cout<<"people = "<<people<<endl;
}
main文件
#ifndef HEAD_H
#define HEAD_H
#include <iostream>
using namespace std;
class Stu{
protected:
string name;
int age;
double score;
public:
//无参构造函数
Stu();
//有参构造函数
Stu(string n, int a, double s);
//拷贝构造函数
Stu(const Stu &other);
//拷贝赋值函数
Stu&operator=(const Stu &other);
//析构函数
~Stu();
//遍历函数
void show();
};
class Leader{
protected:
string job;
double wage;
public:
//无参构造函数
Leader();
//有参构造函数
Leader(string j, double w);
//拷贝构造函数
Leader(const Leader &other);
//拷贝赋值函数
Leader &operator=(const Leader &other);
//析构函数
~Leader();
//遍历函数
void show();
};
class Student_cadre:public Stu,public Leader
{
private:
int people;
public:
//无参构造函数
Student_cadre();
//有参构造函数
Student_cadre(string n,int a,double s,string j,double w,int p);
//拷贝构造函数
Student_cadre(const Student_cadre &other);
//拷贝赋值函数
Student_cadre &operator=(const Student_cadre &other);
//析构函数
~Student_cadre();
//遍历函数
void show();
};
#endif // HEAD_H
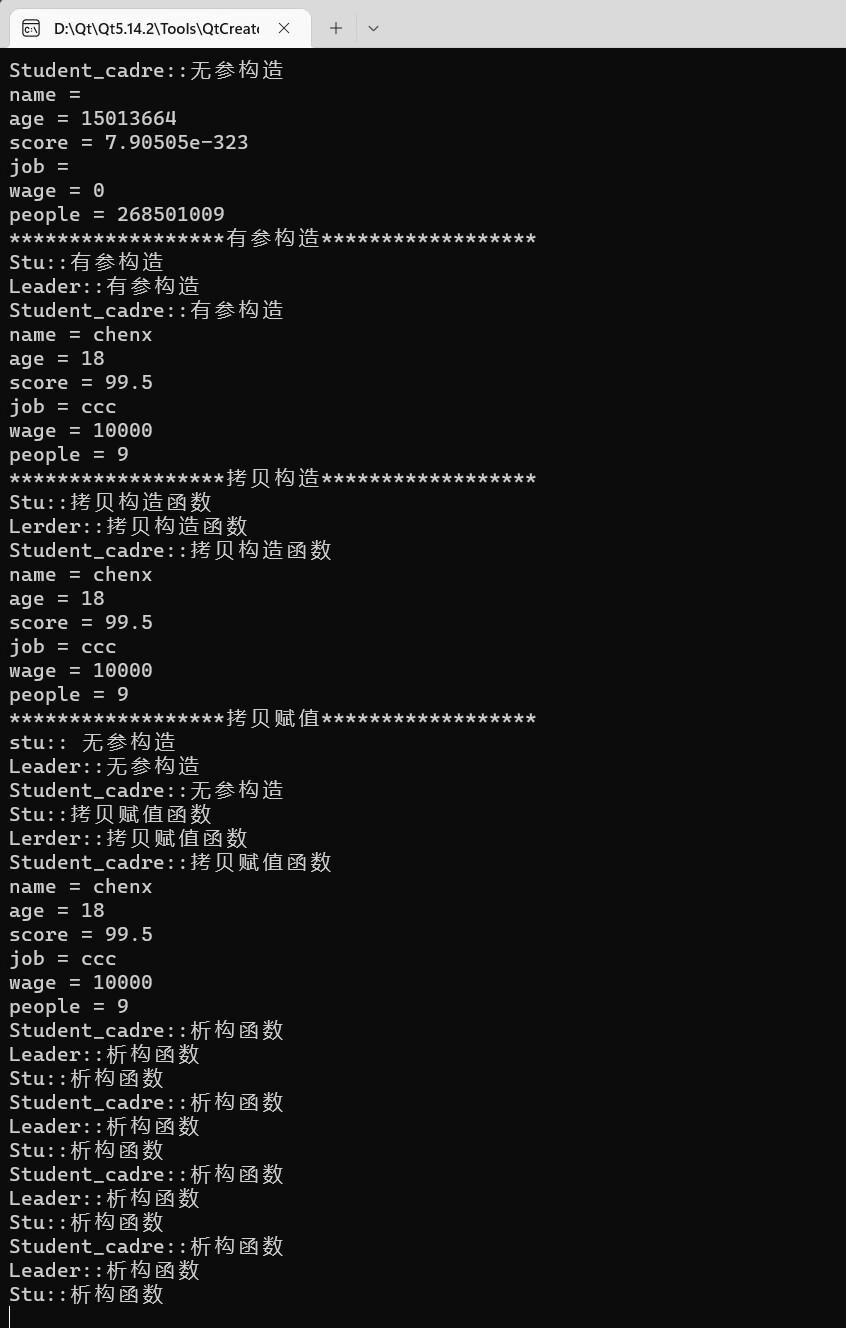