W1 - Arrays
Declaring Array Variables
Creating Arrays
length and Indexed Variables
Default values and Shortcut initialization
Common algorithms with arrays: initialization, printing, sum, min, max, shuffling, shifting, copying
Enhanced for-Loops (for-each loops)
Passing and returning arrays to/from methods (Pass By Value)
The Heap Segment and the Call Stack Memory
Returning an Array from a Method: reverse an array
Searching Arrays: Linear and Binary Search
Sorting Arrays: Selection Sort and Insertion Sort
数组是一种容器,可以用来存储同种数据类型的多个值
(但是需要结合隐式转换考虑)
定义 Declaring Array Variables
只用这一种就ok了
初始化 Creating Arrays
静态初始化
Shortcut initialization 就是简化格式
动态初始化
静vs动
默认值 Default values
0for the numeric data types
'\u0000' for char types
false for boolean types
null for reference types
元素访问
数组名[索引]
匿名数组 anonymous Array
new dataType[]{literal0, literal1, ..., literalk}
There is no explicit reference variable for the array. Such array is called an anonymous array.
数组没有显式的引用变量。这样的数组称为匿名数组。
常用方法
在这里我先初始化一个 int arr[] = new int[5];
length
int length = arr.length;
arraycopy
public static void arraycopy (Object src, int srcPos, Object dest, int destPos, int length)
src
:源数组。srcPos
:源数组中开始复制的起始位置。dest
:目标数组。destPos
:目标数组中开始粘贴的起始位置。length
:要复制的元素数量。
binarySearch
java.util.Arrays.binarySearch(arr, 6));
会返回被搜索的数的索引
sort
java.util.Arrays.sort(arr);
给的点把子小练习
Initializing arrays with random values
Initializing arrays with input values
Printing arrays
Summing all elements 所有元素累加
Finding the largest/smallest element
Random shuffling 参考lab3
Shifting Left/right
Pass by Value
Java uses pass by value to pass arguments to a method.
储存 Heap Segment and the Call Stack Memory
Array是被储存在一个堆中的
查找 search
Liner Search
Binary Search
java.util.Arrays.binarySearch(list, 6));
会返回被搜索的数的索引
排序 Sort
Selection Sort
Insertion Sort
W1 - Object and Classes
Motivating Problems: Complex objects and GUIs
Classes, objects, object state and behavior
Object-oriented Design
Constructors
Accessing fields and methods
Static vs. Non-static
Static Variables and Methods
Default values for Class Fields
Primitive Data Types and Object Types, Copying
Garbage Collection
Example classes in Java API: the Date class
The Random class
Visibility Modifiers and Accessor/Mutator Methods
Classes
A Java class uses:
non-static/instance variables to define data fields
non-static/instance methods to define behaviors
A class provides a special type of methods called constructors which are invoked to construct objects from the class
Object-oriented Design
The Unified Modeling Language统一建模语言 (UML)is a general-purpose modeling language in the field of software engineering that is intended to provide a standard way to visualize the design of a object-oriented system.
Constructors
- must have the same name as the class itself.
- do not have a return type鈥攏ot even void.
A class may be declared without constructors: a no-arg default constructor with an empty body is implicitly declared in the class 某些编程语言中,如果在类的声明中没有明确地定义构造函数,编译器会自动为该类声明一个无参的默认构造函数,该构造函数的主体为空。
Accessing fields and methods
普普通通的方法和数据访问
myCircle.radius
myCircle.getArea()
Static vs. Non-static
静态成员用于在类级别上管理数据和行为,而不是在对象级别上。这使得它们可以在不创建类的实例的情况下使用。静态成员包括静态变量和静态方法。
静态是和自己相关的,非静态是和对象相关的,静态成员可以被看作是属于整个类的固有属性,而不是属于类的单个实例。它们存在于类的内部,而不是对象的内部。因此,无需创建类的实例,就可以直接访问和使用静态成员。
Static Variable
static variables are shared by all the instances of the class:
特点:
被该类所有对象共享
不属于对象,属于类。
随着类的加载而加载,优先于对象存在
调用方式:
类名调用(推荐)
对象名调用
Static method
特点:
多用在测试类和工具类中
Javabean类中很少会用
调用方式:
类名调用(推荐)
对象名调用
e.g Math.pow()
注意事项:
静态方法只能访问静态变量和静态方法
·非静态方法可以访问静态变量或者静态方法,也可以访问非静态的成员变量和非静态的成员方法
·静态方法中是没有this关键字
静态方法中,只能访问静态。
非静态方法可以访问所有。
静态方法中没有this关键字
Default values for Class Fields
local variable inside a method no default value
方法内部声明的局部变量(即在方法内部直接声明的变量)不会被赋予默认值。这意味着,如果您在方法内声明一个变量但未显式为其赋值,那么该变量将不会拥有任何初始值,编译器会在编译时报错(Compilation errors: the variables are not initialized)。
Data fields have default values
类的成员变量(也称为数据字段或成员字段,包括实例变量和静态变量)会被Java自动赋予默认值。这意味着,如果您在类中声明一个成员变量但未显式为其赋值,Java会自动为其赋予默认值。
Primitive Data Types and Object Types, Copying
当一个类的对象c1复制c2时,c1和c2同时指向c2的地址,这个时候原有的c1被当作垃圾删除
Garbage Collection
The object previously referenced by c1 is no longer referenced, it is called garbage
Garbage is automatically collected by the JVM, a process called garbage collection
Example classes in Java API
java.util.Date
java.util.Random
Visibility Modifiers and Accessor(存取器,访问器)
权限修饰
getField (accessors) and setField (mutators) methods are used to read and modify private properties.
Array of objects
An array of objects is an array of reference variables (like the multi-dimensional arrays seen before)
W2 - OOP Thinking
Immutable Objects and Classes
Scope of Variables and Default values
The this Keyword
Calling Overloaded Constructors
Class Abstraction and Encapsulation
Designing and implementing the Loan Class
Designing and implementing the BMI Class
Designing and implementing the Course Class
Designing and implementing the StackOfIntegers Class
The String Class in detail
Regular Expressions
Command-Line Parameters
StringBuilder and StringBuffer
The Character Class
Designing Classes
Immutable Objects and Classes 不可变对象和类
Immutable object: the contents of an object cannot be changed once the object is created
(不可变对象和类)在编程中是指那些一旦创建就不能被修改的对象或类。换句话说,创建不可变对象后,其状态(即对象中的数据)就不能被改变。Java中常见的不可变类是String
类。
Immutable class
1. It must mark all data fields private!
2. Provide no mutator (set) methods!
3. Provide no accessor methods that would return a reference to a mutable data field object!(不要提供会返回对可变数据字段对象的引用的访问方法!)
Scope of Variables and Default values
Local vairable:
- The scope starts from its declaration and continues to the end of the block that contains the variable
- must be initialized explicitly before it can be used.
Data Field Variables:
- can be declared anywhere inside a class
- scope of instance and static variables is the entire class!
- Initialized with default values.
The this Keyword
用处:
1. Reference a class’s “hidden” data fields.
2. To enable a constructor to invoke another constructor of the same class as the first statement in the constructor.
class Abstraction抽象 and Encapsulation封装
Class Abstraction = separate class implementation from the use of the class (API)将类实现与类(API)的使用分开
The user does not need to know how the class is implemented: it is encapsulated (private fields and only the public API is used).
The String Class in detail
Construct
String message = new String("Welcome to Java");/= "Welcome to Java";
A String object is immutable; its contents cannot be changed 注意不是不能改,而是会赋值给一个新的对象,旧对象内容不变,但是地址指向新对象了
Interning
is a method of storing only one copy of each distinct compile-time constant/explicit string in the source code stored in a string intern pool (e.g., s1 and s3).
通过维护一个字符串池(String Pool),即一个保存字符串字面量的集合,确保相同内容的字符串只存储一份。这样,当多个字符串变量拥有相同的值时,它们实际上引用的是同一个内存地址,而不是创建多个相同内容的字符串对象。
池中的字符串在JVM生命周期内不会被垃圾回收。
Method
String Length, Characters, and Combining Strings
Extracting Substrings
Finding a Character or a Substring in a String
Converting, Replacing, and Splitting Strings
Matching, Replacing and Splitting by Patterns
Regular Expression
Comparison
.equals是比较字符串内容,==是比较对象
compareTo(Object object):
- Use
compareTo
when you need to sort or order objects (e.g., in a sorted collection or array). - Use
.equals()
when you need to compare the logical equality of two objects (e.g., to see if two instances represent the same conceptual entity). - Use
==
when you need to check if two references point to the same object in memory (e.g., checking if two variables reference the exact same instance).
Command-Line Parameters
是在运行程序时通过命令行界面(CLI)传递给程序的参数。这些参数可以影响程序的行为或输入值。命令行参数是用户在终端或命令提示符中运行程序时附加在程序名称后的字符串。
在Java程序中,命令行参数可以通过main
方法的参数数组String[] args
来获取。
StringBuilder & StringBuffer
The StringBuilder/StringBufferclasses are alternatives to the String class:
StringBuilder/StringBuffercan be used wherever a string is used, and more flexible
Constructor
method
modifying
The toString, capacity, length, setLength, and charAtMethods
Character
Designing Classes
Coherence: A class should describe a single entity
Separating responsibilities: A single entity with too many responsibilities can be broken into several classes to separate responsibilities
Reuse: Classes are designed for reuse!
Follow standard Java programming style and naming conventions:
1)Choose informative names for classes, data fields, and methods
2)Place the data declaration before the constructor, and place constructors before methods.将数据声明放在构造函数之前,并将构造函数放在方法之前。
3)Provide a public no-arg constructor and override the equals method and the toStringmethod
(returns a String) whenever possible提供一个公共的无参数构造函数,并覆盖equalsmethod和toStringmethod
W2 - Inheritance and Polymorphism
Motivation: Model classes with similar properties and methods
Declaring a Subclass
Constructor Chaining
Calling Superclass Methods with super
Overriding Methods in the Superclass
The Object Class and Its Methods: toString()
Overloading vs. Overriding
Method Matching vs. Binding
Polymorphism, Dynamic Binding and Generic Programming
Casting Objects and instance of Operator
The equals method
The ArrayList Class
The MyStack Class
The protected and final Modifiers
Motivation
Model classes with similar properties and methods
Inheritance is the mechanism of basing a sub-class on extending another super-class
will help us design and implement classes so to avoid redundancy(重复)
Declaring a Subclass
A subclass extends/inherits properties and methods from the superclass.
You can also:
Add new properties
Add new methods
Override the methods of the superclass
Constructor
superclass’s Constructors don't Inherited,They are invoked explicitly or implicitly:
Explicitly using the superkeyword and the arguments of the superclass constructors
Implicitly: if the keyword superis not explicitly used, the superclass's no-arg constructor is automatically invoked as the first statement in the constructor, unless another constructor is invoked with the keyword this(in this case, the last constructor in the chain will invoke the superclass constructor)
- 超类的构造器不被继承:子类不会自动继承父类的构造器。
- 显式调用:使用
super
关键字并传递必要参数,显式调用父类构造器。 - 隐式调用:如果子类构造器中没有显式调用
super
,Java会自动调用父类的无参构造器。 - 使用
this
调用另一个构造器:如果使用this
关键字调用同一个类中的另一个构造器,最终被调用的那个构造器会负责调用父类构造器。
.super
The keyword superrefers to the superclass of the class in which superappears
This keyword is used in two ways:
To call a superclass constructor (through constructor chaining)
To call a superclass method (hidden by the overriding method)
can also Calling Superclass Methods with super
Constructor Chaining
constructing an instance of a class invokes all the superclasses’ constructors along the inheritance chain.
构造一个类的实例调用继承链上所有父类的构造函数。
Overriding Methods in the Superclass
modify in the subclass the implementation of a method defined in the superclass
The default Object implementation returns a string Classname@地址
The Object Class and Its Methods: toString()
java.lang.Object
The toString() method returns a string representation of the object
Overloading vs. Overriding
Method overloading (discussed in Methods) is the ability to create multiple methods of the same name, but with different signatures and implementations:
Method overriding requires that the subclass has the same method signature as in the superclass.
Overloading(重载):
- 重载指的是在同一个类中定义多个方法,它们具有相同的名称但具有不同的参数列表(参数类型、参数个数或参数顺序不同)。
- 在重载中,方法的签名(方法名及参数列表)必须不同,但方法的返回类型可以相同也可以不同。
- 编译器根据方法调用时提供的参数列表来确定调用哪个重载方法。
class OverloadExample {
void foo(int x) {
System.out.println("foo(int)");
}
void foo(double x) {
System.out.println("foo(double)");
}
void foo(int x, double y) {
System.out.println("foo(int, double)");
}
}
Overriding(覆盖):
- 覆盖指的是子类重新定义(覆盖)了从其父类继承而来的方法,重新定义的方法具有相同的名称、参数列表和返回类型。
- 在覆盖中,子类方法可以增加访问权限(例如从protected变为public),但不能减少访问权限。
- 覆盖用于实现子类对于父类方法的定制化行为。
class Parent {
void display() {
System.out.println("Parent's display method");
}
}
class Child extends Parent {
void display() {
System.out.println("Child's display method");
}
}
Method Matching vs. Binding
For overloaded methods, the compiler finds a matching method according to parameter type, number of parameters, and order of the parameters at compilation time.
重载方法指的是在同一个类中,可以有多个方法名称相同但参数不同的方法。这些方法可以通过不同的参数类型、参数数量或参数顺序进行区分。
编译时匹配:
- 编译器在编译时会根据方法的参数类型、参数数量和参数顺序来确定调用哪个具体的方法。
- 这是静态绑定(Static Binding),即在编译阶段确定调用哪个方法
For overridden methods, the Java Virtual Machine dynamically binds the implementation of the most specific overridden method implementation at runtime.
重写方法指的是子类重新定义从父类继承的方法。子类的重写方法与父类方法具有相同的方法签名(方法名、参数类型、参数数量和顺序)。
运行时动态绑定:
- Java虚拟机(JVM)在运行时根据对象的实际类型来决定调用哪个方法。
- 这是动态绑定(Dynamic Binding),即在运行时确定调用哪个方法。
Dynamic Binding
动态绑定(Dynamic Binding)是指在运行时确定对象的方法调用,而不是在编译时确定。这也被称为后期绑定(Late Binding)或运行时多态(Runtime Polymorphism)
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog(); // 向上转型
animal.makeSound(); // 运行时确定实际调用的方法
}
}
在上面的示例中,Dog
类覆盖了 Animal
类的 makeSound()
方法。即使引用变量 animal
声明为 Animal
类型,但由于对象实际上是 Dog
类型,因此在运行时会调用 Dog
类的 makeSound()
方法。这是动态绑定的典型示例。
Polymorphism
an object of a subtype can be used wherever its supertype value is required:
The method mtakes a parameter of the Objecttype, so can be invoked with any object.
多态性是面向对象编程的一个核心概念,它允许一个对象可以有多种形式。具体来说,在Java中,多态性允许一个对象的子类型对象可以在任何需要其超类型对象的地方使用。
- 在面向对象编程中,子类型(subtype)是指从一个父类型(supertype)继承或实现而来的类型。
- 由于子类型对象具有父类型对象的所有特性和行为,因此可以在任何需要父类型对象的地方使用子类型对象。
Casting Objects and Instances Operator
Explicit Casting显式转换
Casting can be used to convert an object of one class type to another within an inheritance hierarchy在继承层次结构中,可以使用强制类型转换将一个类类型的对象转换为另一个类类型
Object o = new Student();
Student b = o; // Syntax Error,会发生编译错误,因为Object o不一定是Student的实例
我们必须使用显式强制转换来告诉编译器o是一个学生对象
Student b = (Student)o;
显式强制转换语法类似于用于基本数据类型之间强制转换的语法
instanceof()
显式强制转换可能并不总是成功(即,如果对象不是子类的实例),可以使用instanceof操作符来测试一个对象是否是类的实例
if (myObject instanceof Student) {...}
equals()
The equals()method compares the contents of two objects
e.g字符串,半径相等的圆
Generic Programming泛型编程
泛型编程是一种编程范式,允许程序员编写代码时使用泛型类型,使得代码可以处理多种数据类型而无需重复编写相似的代码。
polymorphism allows methods to be used generically for a wide range of object arguments:
- if a method’s parameter type is a superclass (e.g.,Object), you may pass an object to this method of any of the parameter’s subclasses (e.g., Student or String) and the particular implementation of the method of the object that is invoked is determined dynamically如果一个方法的参数类型是一个超类(例如,Object),你可以传递一个对象给这个方法的任何一个参数的子类(例如,Student或String),并且被调用的对象的方法的具体实现是动态确定的
- very useful for data-structures
The ArrayList Class
你可以创建数组来存储对象——但是一旦数组被创建,数组的大小就固定了。
Java提供了Java .util. arraylistclass,可以用来存储无限数量的对象:
The MyStack Class(自定义的)
protected 权限修饰符
A protected data or a protected method in a public class can be accessed by any class in the same package or its subclasses, even if the subclasses are in a different package
公共类中的受保护数据或受保护方法可以由同一包或其子类中的任何类访问,即使这些子类位于不同的包中
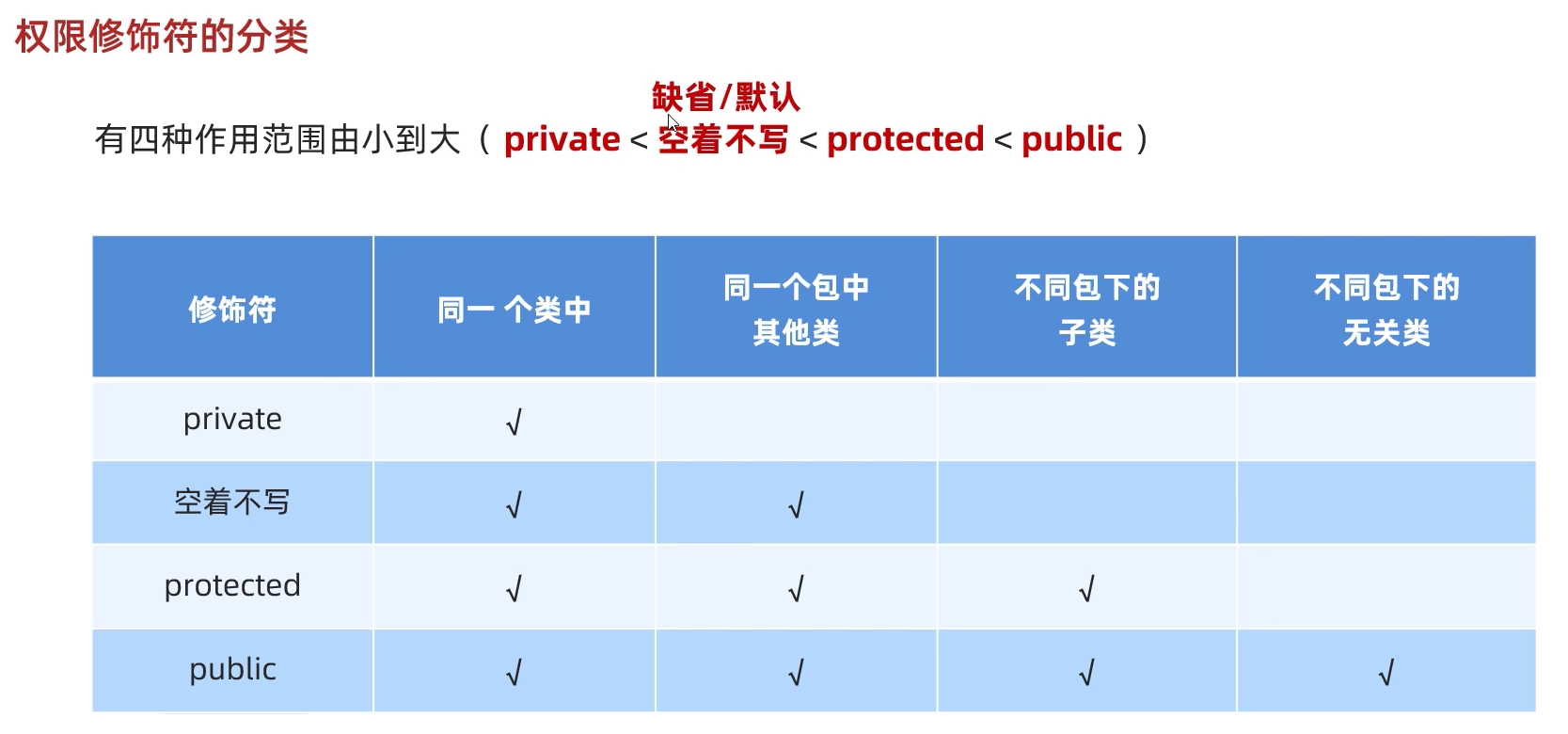
UML中
+ = public
- = private
~ = default/package
# = protected
underlined = static
Accessibility
子类可以覆盖父类中的protected方法,并将其可见性更改为public。
但是,子类不能削弱父类中定义的方法的可访问性。例如,如果一个方法在父类中定义为public,那么它必须在子类中定义为public。总之就是子类可以扩大可见性(Visibility)但是不能缩小可见性。
An instance method can be overridden only if it is accessible实例方法只有在可访问时才能被覆盖
Private
私有方法不能被重写,因为它不能在自己的类之外访问
如果在子类中定义的方法在其超类中是私有的,则这两个方法完全无关
Static
A static method can be inherited
A static method cannot be overridden
If a static method defined in the superclass is redefined in a subclass, the method defined in the superclass is hidden
Final
A final method cannot be overridden by its subclasses
A final class cannot be extended
W3 - Abstract
Abstract Classes and Abstract Methods
The abstract Calendar class and its GregorianCalendar subclass
Interfaces
Define an Interface
Omitting Modifiers in Interfaces
The Comparable Interface
Writing a generic max Method
The Cloneable Interface
Shallow vs. Deep Copy
Interfaces vs. Abstract Classes
Conflicting interfaces
Wrapper Classes: The Number Class and subclasses
BigInteger and BigDecimal
The Rational Class
Abstract Classes and Abstract Methods
抽象方法所在的类,必须是抽象类。但是抽象类中不一定要有抽象方法。它可以只作为定义新子类的基类(base class)
抽取共性时,无法确定方法体,就把方法定义为抽象的。
In a nonabstract (a.k.a., concrete) subclass extended from an abstract super-class, all the abstract methods MUST be implemented. 强制让子类按照某种格式重写。
抽象类的子类:
1) to implement the inherited abstract methods OR
2)to postpone the constraint to implement the abstract methods to its nonabstract subclasses.
抽象子类也可以选择不实现继承的抽象方法,而是将这个责任推迟到它的具体子类。这意味着具体子类必须实现这些抽象方法。
An object cannot be created from abstract class
An abstract class cannot be instantiated using the new operator
We still define its constructors, which are invoked in the constructors of its subclasses through constructor chaining.
定义
注意事项
其中不能实例化意思是不能创建抽象类对象
其次接口中定义的方法不能有方法体,只能被声明
The abstract Calendar class and its GregorianCalendar subclass
Interfaces
An interface is a class-like construct that contains only abstract methods and constants.
An interface is similar to an abstract class, but the intent of an interface is to specify behavior for objects.
接口类似于抽象类,但接口的目的是指定对象的行为。e.g:specify that the objects are comparable, edible, cloneable, …
Allows multiple inheritance: a class can implement multiple interfaces.
是一种规则,是对行为的抽象
Define an Interface 定义
public interface 接口名{}
注意这里新建的是interface文件而不是class文件!
使用
接口不能实例化,和类之间是实现关系,通过implements关键字表示
*可以单实现,也可以多实现,也可以同时继承一个类
public class 类名 implements 接口名{}
接口的子类(实现类):重写接口中的所有抽象方法/本身是抽象类
成员特点***
Omitting Modifiers in Interfaces 可以省略修饰符
Interface vs Class
An interface is treated like a special class in Java, Each interface is compiled into a separate bytecode file just like a regular class.每个接口被编译成一个单独的字节码文件,就像一个普通的类一样。
Like an abstract class, you cannot create an instance from an interface using the new operator
Uses of interfaces are like for abstract classes:1)as a data type for a variable 2) as the result of casting
p.s如果实现子接口则需要重写子接口+父接口里面所有的方法
一个接口可以扩展任意数量的其他接口,一个类可以实现任意数量的接口
接口没有根
使用时机
Class
Strong is-a: a relationship that clearly describes a parent-child relationship
For example: a student is a person
Interface
Weak is-a (or is-kind-of): indicates that an object possesses a certain property
For example: all strings are comparable, all dates are comparable
Interface vs Genetic
继承表达的是"is-a"关系,即子类是父类的一种类型。而接口表达的是"has-a"关系,即类具有某些行为或特征。
- 继承通常用于在已有类的基础上创建新的类,扩展其功能或修改其行为,同时保留了原有类的特性。
- 接口通常用于定义一组规范,规定了实现类需要具备的方法或属性,从而增强了代码的灵活性和可扩展性。
The Comparable Interface
为啥要用comparable interface呢?
算法可以用于各种类型的数据,例如 String
、Double
、Date
甚至是用户自定义的类,只要这些类实现了 Comparable
接口。要比较对象类型(如 Integer
、String
),则需要使用对象的比较方法。因此,compareTo
方法是比较对象类型的标准方式。
Comparable Interface vs comparator
Comparable
接口用于定义对象的自然排序,需要修改类本身,并实现compareTo
方法。Comparator
接口用于定义类外的排序规则,可以创建多个不同的比较器,实现不同的排序逻辑,使用compare
方法
Writing a generic max Method
The Cloneable Interface
Marker Interface: is an empty interface (does not contain constants or methods), but it is used to denote that a class possesses certain desirable properties to the compiler and the JVM.标记接口:是一个空接口(不包含常量或方法),但它用于表示类具有编译器和JVM所需的某些属性。
A class that implements the Cloneable interface is marked cloneable: 它的对象可以通过在Object中的clone()方法克隆,然后我们可以在这个类中重写override这个方法
注意,克隆后地址还是不一样的,所以不是==,而是.equals
Shallow vs. Deep Copy
浅克隆通过创建一个新对象,然后将原对象的非静态字段值复制到新对象来实现。对于基本数据类型(如int、float等)和不可变对象(如String)的字段,浅克隆会复制它们的值。但是,对于引用类型(如数组、对象等),浅克隆只复制引用,即新对象中的引用字段指向原对象中的引用字段所指向的同一内存位置。
深克隆不仅创建一个新对象,而且还递归地复制所有引用类型的字段。这意味着新对象和原对象中的引用类型字段指向的是不同的内存位置,因此修改新对象中的引用类型字段不会影响原对象中的相应字段。
Interfaces vs. Abstract Classes
1)In an interface, the data fields must be constants; an abstract class can have variable data fields
2) Interfaces don't have constructors; all abstract classes have constructors
3) Each method in an interface has only a signature without implementation (i.e., only abstract methods); an abstract class can have concrete methods
1)在接口中,数据字段必须是常量;抽象类可以有可变的数据字段
2)接口没有构造函数;所有抽象类都有构造函数
3)接口中的每个方法只有一个签名而没有实现(即只有抽象方法);抽象类可以有具体的方法
的来说,接口更加纯粹地用于定义类之间的契约,强调了类应该具备的行为,而抽象类则更适用于具有一些通用实现的类的情况。通常情况下,如果要表示多个类具有共同的行为但又不需要共享状态或实现代码,则使用接口;如果需要在类之间共享代码或状态,则使用抽象类。
Conflicting interfaces 接口错误
编译器检测到的错误:如果一个类实现了两个具有冲突信息的接口,例如:两个具有不同值的相同常量,或者两个具有相同签名但返回类型不同的方法
Wrapper Classes 封装类
Java中的原始数据类型性能更好,然而,数据结构(ArrayList)期望对象作为元素,所以Each primitive type has a wrapper class: Boolean, Character, Short, Byte, Integer, Long, Float, Double
The wrapper classes do not have no-arg constructors
The instances of all wrapper classes are immutable: their internal values cannot be changed once the objects are created 所有包装器类的实例都是不可变的:一旦创建了对象,它们的内部值就不能更改。如果想改变就是新建一个然后再指向过去。
Each wrapper class overrides the toString() and equals() methods defined in the Object class
Since these classes implement the Comparable interface, the compareTo() method is also implemented in these classes
Nuber Class
每个数字包装类都继承了抽象的Number class:
The abstract Numberclass contains the methods doubleValue, floatValue, intValue, longValue, shortValue, and byteValueto “convert” objects into primitive type values
The methods doubleValue, floatValue, intValue, longValueare abstract
The methods byteValueand shortValueare not abstract, which simply return (byte)intValue()and (short)intValue(), respectively
Each numeric wrapper class implements the abstract methods doubleValue, floatValue, intValueand longValue
Numeric Wrapper Class Constants
每个数字类都有一个常量的最大值和最小值
valueof()
数值包装器类有一个静态方法valueOf(String s)来创建一个初始化为指定字符串表示的值的新对象:
Double doubleObject = Double.valueOf("12.4");
Integer integerObject = Integer.valueOf("12");
每个数字包装器类都有重载的解析方法overloaded parsing methods来将数字字符串解析为适当的数字值:
double d = Double.parseDouble("12.4");
int i = Integer.parseInt("12");
*不能将int[]数组赋值给double[]数组变量:compiler error: double[] a = new int[10];
boxing & unboxing
装箱(Boxing):将基本数据类型转换为对应的包装类对象。例如,将int
转换为Integer
。
拆箱(Unboxing):将包装类对象转换为对应的基本数据类型。例如,将Integer
转换为int
。
boxing of primitive types into wrapper types when objects are needed
unboxing of wrapper types into primitive types when primitive types are needed
Sorting的代码实现*
BigInteger and BigDecimal
BigIntegerand BigDecimalclasses in the java.mathpackage:
For computing with very large integers or high precision floating-point values
BigIntegercan represent an integer of any size
BigDecimalhas no limit for the precision (as long as it’s finite=terminates)
Both are immutable
Both extend the Number class and implement the Comparable interface.
BigInteger
构造方法
对象一旦创建,内部记录的值不能发生改变!
只要进行计算都会产生一个新的BigInteger的对象
表示的数字没有超出long的范围可以用静态方法获取,超过了就用构造方法获取
把内部常用数字进行了优化(-16~16)如果多次获取不会重新创建新的
方法
底层
BigDecima
用于小数的精确运算,并且用来表示很大的小数
创建对象
1.如果要表示的数字不大,没有超出double的取值范围,建议使用静态方法
2.如果要表示的数字比较大,超出了double的取值范围,建议使用构造方法
3.如果我们传递的是0~10之间的整数,包含8,包含10,那么方法会返回已经创建好的对象,不会重新new
方法
底层
ascII码
The Rational Class???
W4 - Generics 泛型
To know the benefits of generics
To use generic classes and interfaces
To declare generic classes and interfaces
To understand why generic types can improve reliability and readability
To declare and use generic methods and bounded generic types
To use raw types for backward compatibility
To know wildcard types and understand why they are necessary
To convert legacy code using JDK 1.5 generics
To understand that generic type information is erased by the compiler and all instances of a generic class share the same runtime class file
To know certain restrictions on generic types caused by type erasure
To design and implement generic matrix classes
benefits of generics
Generics is the capability to parameterize types
With this capability, you can define a class or a method with generic types that can be substituted using concrete types by the compiler
e.g You may define a generic stack class that stores the elements of a generic type
1) Compiler Errors instead of Runtime Errors
The key benefit of generics is to enable errors to be detected at compile time rather than at runtime
A generic class or method permits you to specify allowable types of objects that the class or method may work with
Most important advantage: If you attempt to use the class or method with an incompatible object, a compile error occurs如果试图将类或方法与不兼容的对象一起使用,则会发生编译错误
2) No Casting Needed
3) No Primitive types
泛型类型必须是引用类型!不能用int、double或char等基本类型替换泛型类型
ArrayList<int> intList = new ArrayList<>(); (X)
ArrayList<Integer> intList = new ArrayList<>; (V)
4)No Casting for Get and Unboxing
generic classes and interfaces
declare generic classes and interfaces
Bounded 限制
泛型类型可以指定为另一类型的子类型: 考虑Circle和Rectangle extend GeometricObject
e.g. public static <E extends GeometricObject> boolean
e.g Sorting an Array of Objects
Raw Type and Backward Compatibility
使用不指定具体类型的泛型类或接口,称为原始类型,可以向后兼容早期版本的Java
ArrayList list = new ArrayList();
is roughly equivalent to:
ArrayList<Object> list = new ArrayList<Object>();
* Backward compatibility: a technology is backward compatible if the input developed for an older technology can work with the newer technology:
**注意,原始类型不安全,e.g在一个列表中输入不同类型的数据
所以如何让它安全呢?↓
Wildcard 通配符
通配符类型(wildcard types)是一种Java泛型类型系统中的特性,用于表示不确定的类型。通配符类型使用 ?
符号来表示,可以用在泛型类、泛型方法、泛型接口等地方,用于灵活地处理泛型类型的参数。
可以使用无界通配符、有界通配符或下限通配符来指定泛型类型的范围:
e.g <? extends Number> is a wildcard type that represents Number or a subtype of Number
GenericStack<Integer>is a subclass of GenericStack<? extends Number> but not a subclass of GenericStack<Number>
代码*
Erasure 擦除
泛型在Java中使用一种称为类型擦除的方法实现:
类型擦除(Type Erasure):在编译时移除所有泛型类型信息,将泛型类型参数替换为原始类型,并插入必要的类型转换,以确保类型安全。The compiler uses the generic type information to compile the code, but erases it afterwards,This approach enables the generic code to be backward-compatible with the legacy code that uses raw types
So the generic information is not available at run time
Once the compiler confirms that a generic type is used safely, it converts the generic type back to a raw type Object
e.g a→b
The generic class is shared by all its instances regardless of its actual generic type
虽然GenericStack<String>和GenericStack<Integer>是两种类型,但是只有一个类GenericStack被加载到JVM中,因此
因为GenericStack<String>和GenericStack<Integer>不是作为单独的类存储在JVM中,所以在运行时使用它们是没有意义的
、
Restrictions限制
泛型的限制(Restrictions):由于类型擦除,所以对于如何使用泛型类型有一定的限制,如不能实例化泛型类型、不能创建泛型数组、不能使用基本类型作为泛型参数、静态成员不能使用泛型类型参数以及泛型类型在运行时的多态性问题。
Restriction 1: Cannot Create an Instance of a Generic Type 不能实例化泛型
The reason is that new E()is executed at runtime, but the generic type Eis not available at runtime
Restriction 2: Generic Array Creation is Not Allowed (i.e., new E[100]).
Restriction 3: Since all instances of a generic class have the same runtime class, the static variables and methods of a generic class are shared by all its instances.由于泛型类的所有实例具有相同的运行时类,因此泛型类的静态变量和方法由其所有实例共享。Therefore, it is illegal to refer to a generic type parameter for a class in a static method, field, or initializer 泛型类型参数是与对象实例相关联的,而不是与类本身相关联的。由于这个原因,在静态上下文中使用泛型类型参数是非法的
如果你需要在静态上下文中使用类型参数,可以考虑使用静态方法中的局部类型参数或者将方法变为泛型方法。
Restriction 4: Exception Classes Cannot be Generic