// --01.cpp--
#include <iostream>
#include <string>
int main()
{
using namespace std;
string fname;
string lname;
cout << "What is your first name? ";
getline(cin, fname);
cout << "What is your last name? ";
getline(cin, lname);
char grade;
cout << "What letter grade do you deserve? ";
cin >>grade;
int age;
cout << "What is your age? ";
cin >> age;
cout << "Name: " << lname << ", " << fname << endl;
cout << "Grade: " << (char)(grade + 1) << endl;
cout << "Age: " << age << endl;
cin.get();
cin.get();
return 0;
}
// --02.cpp-- 修改程序清单4.4,使用C++ string类而不是char数组
#include <iostream>
#include <string>
int main()
{
using namespace std;
string name;
string dessert;
cout << "Enter your name:\n";
getline(cin, name);
cout << "Enter your favorite dessert:\n";
getline(cin, dessert);
cout << "I have some delicious " << dessert;
cout << " for you, " << name << endl;
cin.get();
return 0;
}
// --03.cpp--
/*
编写一个程序,它要求用户首先输入其名,然后输入姓;然后程序使用一个逗号和空格将姓和
名组合起来,并存储和显示组合结果。请使用char数组和头文件cstring中的函数。下面是该
程序运行的情形:
Enter your first name: Flip
Enter your last name: Fleming
Here's the information in a single string: Fleming, Flip
*/
#include <iostream>
#include <cstring>
int main()
{
using namespace std;
char fname[20];
char lname[20];
char full[40];
cout << "Enter your first name: ";
cin.get(fname, 20).get();
cout << "Enter your last name: ";
cin.get(lname, 20).get();
strcpy(full, lname);
strcat(full, ", ");
strcat(full, fname);
cout << "Here's the information in a single string: " << full << endl;
cin.get();
return 0;
}
// --04.cpp--
/*
编写一个程序,它要求用户首先输入其名,在输入其姓;然后程序使用一个逗号和空格将姓和名
组合起来,并存储和显示结果。请使用string对象和头文件string中的函数。下面是该程序运行时
的情形:
Enter your first name: Flip
Enter your last name: Fleming
Here's the information in a single string: Fleming, Flip
*/
#include <iostream>
#include <string>
int main()
{
using namespace std;
string fname;
string lname;
string full;
cout << "Enter your first name: ";
getline(cin, fname);
cout << "Enter your last name: ";
getline(cin, lname);
full = lname + ", " + fname;
cout << "Here's the information in a single string: " << full << endl;
cin.get();
return 0;
}
// --05.cpp--
/*
结构CandyBar包含3个成员。第一个成员存储了糖块的品牌;第二个成员存储糖块的重量(可以
有小数):第三个成员存储了糖块的卡路里含量(整数)。请编写一个程序,声明这个结构,创建一个名为
snack的CandyBar变量,并将其成员分别初始化为"Mocha Munch"、2.3 和 350。初始化应在声明snack
时进行。最后,程序显示snack变量的内容。
*/
#include <iostream>
#include <string>
using namespace std;
struct CandyBar
{
string brand;
float weight;
int calorie;
};
int main()
{
CandyBar snack
{
"Mocha Munch",
2.3,
350
};
cout << snack.brand << endl;
cout << snack.weight << endl;
cout << snack.calorie << endl;
cin.get();
return 0;
}
// --06.cpp--
/*
结构CandyBar包含3个成员,如编程练习5所示。请编写一个程序,创建一个包含3个元素的
CandyBar数组,并将它们初始化为所选择的值,然后显示每个结构的内容。
*/
#include <iostream>
#include <string>
using namespace std;
struct CandyBar
{
string brand;
float weight;
int calorie;
};
int main()
{
CandyBar candy[3]
{
{"hana momo", 2.1, 300},
{"bobo mi", 1.8, 180},
{"Gururu", 2.5, 280}
};
cout << "The first candy:\n";
cout << "name: " << candy[0].brand << endl;
cout << "weight: " << candy[0].weight << endl;
cout << "calorie: " << candy[0].calorie << endl;
cout << "The second candy:\n";
cout << "name: " << candy[1].brand << endl;
cout << "weight: " << candy[1].weight << endl;
cout << "calorie: " << candy[1].calorie << endl;
cout << "The third candy:\n";
cout << "name: " << candy[2].brand << endl;
cout << "weight: " << candy[2].weight << endl;
cout << "calorie: " << candy[2].calorie << endl;
cin.get();
return 0;
}
// --07.cpp--
/*
William Wingate从事比萨饼分析服务。对于每个比萨饼,他都需要纪录下列信息:
● 披萨饼公司的名称,可以有多个单词组成。
● 披萨饼的直径。
● 披萨饼的重量。
请设计一个能够存储这些信息的结构,并编写一个使用这种结构变量的程序。程序将请求用户输入上
述信息,然后显示这些信息。请使用cin(或它的方法)和cout。
*/
#include <iostream>
#include <string>
using namespace std;
struct Pizza{
string company;
float diameter;
float weight;
};
int main()
{
Pizza info;
cout << "Enter a pizza company name which you like:";
getline(cin, info.company);
cout << "Then enter their pizza's diameter:";
cin >> info.diameter;
cout << "Then enter pizza's weight:";
cin >> info.weight;
cout << "Here is infomation of pizza which you like\n";
cout << "company: " << info.company << endl;
cout << "diameter: " << info.diameter << endl;
cout << "weight: " << info.weight << endl;
cout << endl;
system("pause");
return 0;
}
// --08.cpp--
/*
完成编程练习7,但使用new来为结构分配内存,而不是声明一个结构变量。另外,让程序在请求
输入披萨饼公司名称之前输入披萨饼的直径。
*/
#include <iostream>
#include <string>
using namespace std;
struct Pizza{
string company;
float diameter;
float weight;
};
int main()
{
Pizza * ps = new Pizza;
cout << "Enter a pizza's diameter which you like: ";
cin >> ps->diameter;
cin.get();
cout << "The pizza's company name: ";
getline(cin, ps->company);
cout << "The pizza's weight: ";
cin >> ps->weight;
cout << "Here is infomation of pizza which you like\n";
cout << "company: " << ps->company << endl;
cout << "diameter: " << ps->diameter << endl;
cout << "weight: " << ps->weight << endl;
cout << endl;
delete ps;
system("pause");
return 0;
}
// --09.cpp-- 完成编程练习6,但使用new来分配动态数组,而不是声明一个包含3个元素的CandyBar数组。
#include <iostream>
#include <string>
using namespace std;
struct CandyBar
{
string brand;
float weight;
int calorie;
};
int main()
{
CandyBar * ps = new CandyBar[3];
*ps = {"hana momo", 2.1, 300};
*(ps + 1) = {"bobo mi", 1.8, 180};
*(ps + 2) = {"Gururu", 2.5, 280};
for (int i = 0;i < 3; i++)
{
cout << "name: " << ps[i].brand << endl;
cout << "weight: " << ps[i].weight << endl;
cout << "calorie: " << ps[i].calorie << endl;
}
delete [] ps;
system("pause");
return 0;
}
// --10.cpp--
/*
编写一个程序,让用户输入三次40码跑的成绩(如果您愿意,也可让用户输入40米跑的成绩),
并显示次数和平均成绩。请使用一个array对象来存储数据(如果编译器不支持array类,请使用数组)。
*/
#include <iostream>
#include <array>
int main()
{
using namespace std;
array<double, 3> seconds;
double sum = 0.0;
int times;
cout << "Enter your 40 whatever result for 3 times:\n";
for (times = 0; times < 3; times++)
{
cout << "Time" << times + 1 << ": ";
cin >> seconds[times];
sum += seconds[times];
}
times = 3;
double aver;
aver = sum / times;
cout << "Your average result in " << times << " times" << "is " << aver << " seconds" << endl;
system("pause");
return 0;
}
c++ primer plus第4章编程练习
最新推荐文章于 2023-04-14 02:35:17 发布
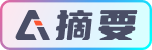