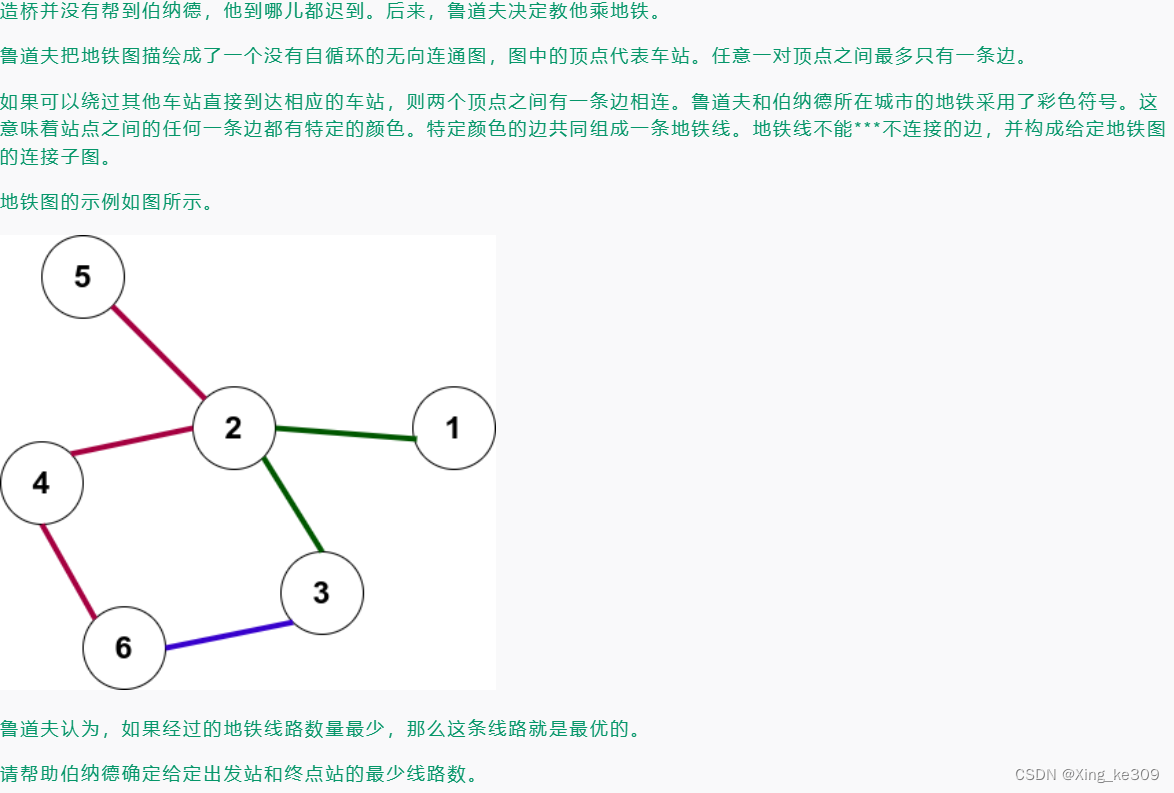
解题思路
- 每条边的边权可选,由颜色决定
- 同一颜色的线路可以直达
- 颜色最多有
种 - 考虑将颜色视作链接点,进行分层图跑最短路

- 最终结果除2
- 最多建
条边 - (直接存状态Map跑最短路被毙掉了)
import java.io.*;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Objects;
import java.util.PriorityQueue;
import java.util.Stack;
import java.util.StringTokenizer;
import java.util.TreeSet;
import java.util.Vector;
//implements Runnable
public class Main{
static long md=(long)20020219;
static long Linf=Long.MAX_VALUE/2;
static int inf=Integer.MAX_VALUE/2;
static int M=200000;
static int N=300;
static int n=0;
static int m=0;
static int k=0;
static
class Node{
int x;
int y;
public Node(int u,int v) {
x=u;
y=v;
}
}
static
class Edge{
int fr,to,nxt;
int val;
public Edge(int u,int v,int w) {
fr=u;
to=v;
val=w;
}
}
static Edge[] e=new Edge[4*M+1];
static int[] head;
static int cnt=0;
static int col=0;
static void addEdge(int fr,int to,int val) {
cnt++;
e[cnt]=new Edge(fr, to, val);
e[cnt].nxt=head[fr];
head[fr]=cnt;
}
static int Dij(int s,int t) {
int[] dis=new int[n+col+1];
Arrays.fill(dis, inf);
boolean[] vis=new boolean[n+col+1];
PriorityQueue<Node> q=new PriorityQueue<Node>((o1,o2)->{
return o1.y-o2.y;
});
dis[s]=0;
q.add(new Node(s,0));
while(!q.isEmpty()) {
Node now=q.peek();q.poll();
int x=now.x;
if(vis[x])continue;
vis[x]=true;
for(int i=head[x];i>0;i=e[i].nxt) {
int v=e[i].to;
int w=e[i].val;
if(vis[v])continue;
if(dis[v]>dis[x]+w) {
dis[v]=dis[x]+w;
q.add(new Node(v, dis[v]));
}
}
}
return dis[t];
}
public static void main(String[] args) throws Exception{
AReader input=new AReader();
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
int T=input.nextInt();
while(T>0) {
n=input.nextInt();
m=input.nextInt();
head=new int[n+m*2];
cnt=0;
HashMap<Integer, Integer> hs=new HashMap<Integer, Integer>();
col=n;
for(int i=1;i<=m;++i) {
int u=input.nextInt();
int v=input.nextInt();
int c=input.nextInt();
int x=0;
if(hs.get(c)==null) {
col++;x=col;
hs.put(c, col);
}else {
x=hs.get(c);
}
addEdge(u, x, 1);
addEdge(x, u, 1);
addEdge(v, x, 1);
addEdge(x, v, 1);
}
int s=input.nextInt();
int t=input.nextInt();
out.println(Dij(s, t)/2);
T--;
}
out.flush();
out.close();
}
// public static final void main(String[] args) throws Exception {
// new Thread(null, new Main(), "线程名字", 1 << 27).start();
// }
// @Override
// public void run() {
// try {
// //原本main函数的内容
// solve();
//
// } catch (Exception e) {
// }
// }
// static
// class Node{
// int x;
// int y;
// public Node(int u,int v) {
// x=u;
// y=v;
// }
// @Override
// public boolean equals(Object o) {
// if (this == o) return true;
// if (o == null || getClass() != o.getClass()) return false;
// Node may = (Node) o;
// return x == may.x && y==may.y;
// }
//
// @Override
// public int hashCode() {
// return Objects.hash(x, y);
// }
// }
static
class AReader{
BufferedReader bf;
StringTokenizer st;
BufferedWriter bw;
public AReader(){
bf=new BufferedReader(new InputStreamReader(System.in));
st=new StringTokenizer("");
bw=new BufferedWriter(new OutputStreamWriter(System.out));
}
public String nextLine() throws IOException{
return bf.readLine();
}
public String next() throws IOException{
while(!st.hasMoreTokens()){
st=new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
public char nextChar() throws IOException{
//确定下一个token只有一个字符的时候再用
return next().charAt(0);
}
public int nextInt() throws IOException{
return Integer.parseInt(next());
}
public long nextLong() throws IOException{
return Long.parseLong(next());
}
public double nextDouble() throws IOException{
return Double.parseDouble(next());
}
public float nextFloat() throws IOException{
return Float.parseFloat(next());
}
public byte nextByte() throws IOException{
return Byte.parseByte(next());
}
public short nextShort() throws IOException{
return Short.parseShort(next());
}
public BigInteger nextBigInteger() throws IOException{
return new BigInteger(next());
}
public void println() throws IOException {
bw.newLine();
}
public void println(int[] arr) throws IOException{
for (int value : arr) {
bw.write(value + " ");
}
println();
}
public void println(int l, int r, int[] arr) throws IOException{
for (int i = l; i <= r; i ++) {
bw.write(arr[i] + " ");
}
println();
}
public void println(int a) throws IOException{
bw.write(String.valueOf(a));
bw.newLine();
}
public void print(int a) throws IOException{
bw.write(String.valueOf(a));
}
public void println(String a) throws IOException{
bw.write(a);
bw.newLine();
}
public void print(String a) throws IOException{
bw.write(a);
}
public void println(long a) throws IOException{
bw.write(String.valueOf(a));
bw.newLine();
}
public void print(long a) throws IOException{
bw.write(String.valueOf(a));
}
public void println(double a) throws IOException{
bw.write(String.valueOf(a));
bw.newLine();
}
public void print(double a) throws IOException{
bw.write(String.valueOf(a));
}
public void print(char a) throws IOException{
bw.write(String.valueOf(a));
}
public void println(char a) throws IOException{
bw.write(String.valueOf(a));
bw.newLine();
}
}
}