#include <opencv2/opencv.hpp>
#include <iostream>
#include <math.h>
#include <opencv2\imgproc\types_c.h>
using namespace std;
using namespace cv;
Mat src, gray_src, drawImg;
int threshold_v = 170;
int threshold_max = 255;
const char* output_win = "rectangle-demo";
RNG rng(12345);
void Contours_Callback(int, void*);
int main(int argc, char** argv) {
src = imread("D:\\test.jpg");
if (!src.data) {
printf("could not load image...\n");
return -1;
}
cvtColor(src, gray_src, CV_BGR2GRAY);//变成灰度图像
blur(gray_src, gray_src, Size(3, 3), Point(-1, -1));//均值模糊
const char* source_win = "input image";
namedWindow(source_win, WINDOW_AUTOSIZE);
namedWindow(output_win, WINDOW_AUTOSIZE);
imshow(source_win, src);
createTrackbar("Threshold Value:", output_win, &threshold_v, threshold_max, Contours_Callback);//创建滑动条
Contours_Callback(0, 0);
waitKey(0);
return 0;
}
void Contours_Callback(int, void*) {
Mat binary_output;
vector<vector<Point>> contours;
vector<Vec4i> hierachy;
threshold(gray_src, binary_output, threshold_v, threshold_max, THRESH_BINARY);//二值图像
//imshow("binary image", binary_output);
findContours(binary_output, contours, hierachy, RETR_TREE, CHAIN_APPROX_SIMPLE, Point(-1, -1));//找轮廓
vector<vector<Point>> contours_ploy(contours.size());
vector<Rect> ploy_rects(contours.size());
vector<Point2f> ccs(contours.size());
vector<float> radius(contours.size());
vector<RotatedRect> minRects(contours.size());
vector<RotatedRect> myellipse(contours.size());
//approxPolyDP(InputArray curve, OutputArray approxCurve, double epsilon, bool closed)基于RDP算法实现, 目的是减少多边形轮廓点数
//cv::boundingRect(InputArray points)得到轮廓周围最小矩形左上交点坐标和右下角点坐标,绘制一个矩形
//cv::minAreaRect(InputArray points)得到一个旋转的矩形,返回旋转矩形
//找到最小包含矩形和圆,旋转矩形与椭圆。
/*cv::minEnclosingCircle(InputArray points, //得到最小区域圆形
Point2f& center, // 圆心位置
float& radius)// 圆的半径
cv::fitEllipse(InputArray points)得到最小椭圆
*/
for (size_t i = 0; i < contours.size(); i++) {
approxPolyDP(Mat(contours[i]), contours_ploy[i], 3, true);//减少多边形轮廓点数
ploy_rects[i] = boundingRect(contours_ploy[i]);//得到轮廓周围最小矩形左上交点坐标和右下角点坐标,绘制一个矩形
minEnclosingCircle(contours_ploy[i], ccs[i], radius[i]);//得到最小区域圆形
if (contours_ploy[i].size() > 5) {//点数大于5才绘制出来
myellipse[i] = fitEllipse(contours_ploy[i]);//得到最小椭圆
minRects[i] = minAreaRect(contours_ploy[i]);//得到一个旋转的矩形,返回旋转矩形
}
}
// 绘制
drawImg = Mat::zeros(src.size(), src.type());
Point2f pts[4];
for (size_t t = 0; t < contours.size(); t++) {
Scalar color = Scalar(rng.uniform(0, 255), rng.uniform(0, 255), rng.uniform(0, 255));
//rectangle(drawImg, ploy_rects[t], color, 2, 8);
//circle(drawImg, ccs[t], radius[t], color, 2, 8);
if (contours_ploy[t].size() > 5) {
ellipse(drawImg, myellipse[t], color, 1, 8);//绘制最小的椭圆
minRects[t].points(pts);//绘制最小的矩形
for (int r = 0; r < 4; r++) {
line(drawImg, pts[r], pts[(r + 1) % 4], color, 1, 8);
}
}
}
imshow(output_win, drawImg);
return;
}
轮廓周围绘制矩形框和圆形框
最新推荐文章于 2021-04-22 10:38:14 发布
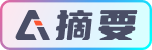