Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 58187 Accepted Submission(s): 25285
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Note: the number of first circle should always be 1.
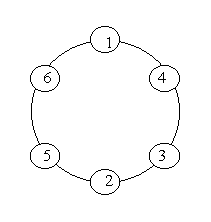
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
给定数字1-n
填入n个格子里面,要求每个数字与相邻的数字和为素数,第一个和最后一个数字和也是素数且第一位必须为1
其实还有个有趣的规律
当 n为奇数且n不为1时,不能满足这个条件,也就是说一个也打印不出来
AC代码如下
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define maxn 50 //因为n<20所以可能的最大两个数的和为20+19,这里开50够用了
int a[maxn];
int v[maxn];
int n;
int t = 1;//打印Case t:
int prime[maxn];
void Prime() { //素数打表
prime[1] = 1;
for(int i = 2; i *i <maxn; i++)
if(!prime[i])
for(int j = i*i; j < maxn; j += i)
prime[j] = 1;
}
void Type() { //如果满足了所有条件,打印该数组
for(int i = 1; i <= n; i++) {
if(i > 1)
printf(" ");
printf("%d",a[i]);
}
printf("\n");
}
void dfs(int q) {
if(q == n+1 && !prime[a[q-1] + a[1]]) { //判断是不是到了最后一个数 && 最后一个数和第一个数的和是素数
Type(); //是证明满足条件,打印
return;
}
for(int i = 2; i <= n; i++) { //因为第一位1是固定的,所以从2开始
if(!v[i] && !prime[i + a[q-1]]) {
a[q] = i; //往数组里填数
v[i]= 1;
dfs(q+1);
v[i] = 0; //这里我卡了较长时间,其实就是每个递归栈返回后才会进行这一步
//每个递归函数返回时都会将该位的数清0,也就保证了每个数只能拿一次
//且每个数都会被拿一次
}
}
}
int main() { //主函数里都很简单,就不解释了
Prime();
while(~scanf("%d",&n)) {
memset(a,0,sizeof(a));
memset(v,0,sizeof(v));
printf("Case %d:\n",t);
t++;
a[1] = 1;
v[1] = 1;
dfs(2);
printf("\n");
}
return 0;
}
还有其他类似的搜索题,链接如下:
Oil Deposits http://blog.csdn.net/bestsort/article/details/79237415