joystickLibrary
joystickLibrary_SRC
TestProjects
VisualC++2008 redistribution
History
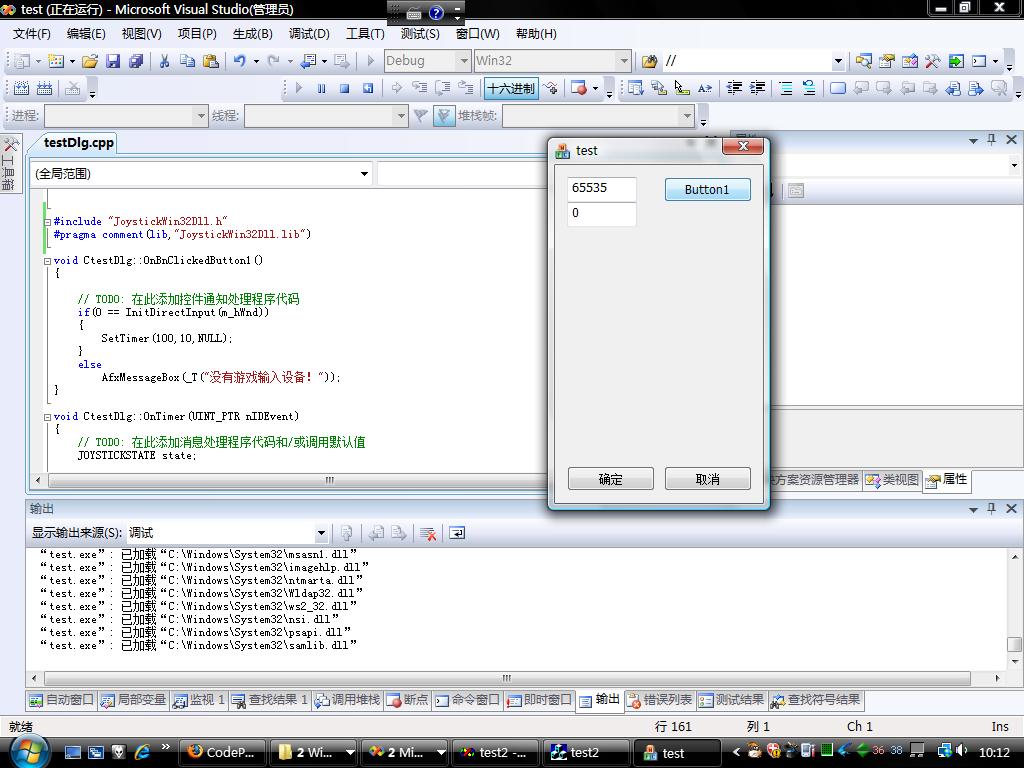
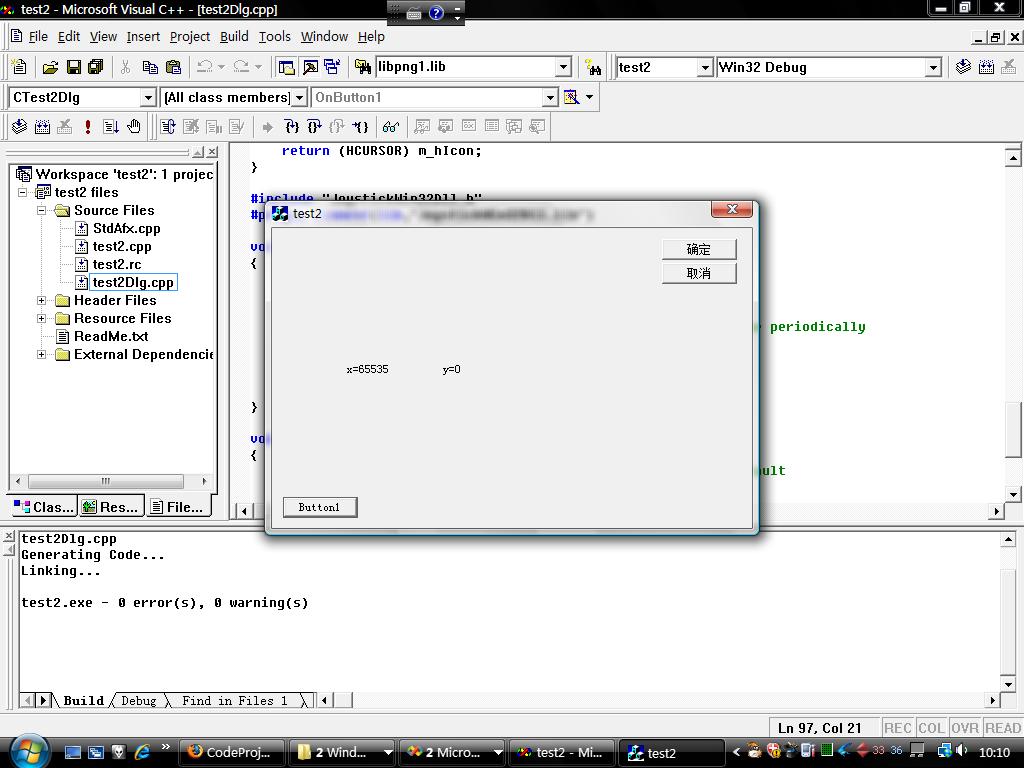
Introduction
This is a dll for getting state of the joystick. Simply Initialize the joystick, and Get the state. Works done,perfect!!
Background
One day, when i was writting a control program with a joystick, i found there is no simple way to control the joystick,the directx architecture is too complicate to understand for beginners. And also, there is many repeated works in writting this kind of codes. The last problem is that when we write a directinput program, we should input large amount of codes. So i wrote this dll, only have 3 functions, do all of the works.
Using the code
Step by step, i take a visual studio 6.0 project for an example:
step1: Download the four packages.
step2: Integrade the library in your project, include the header file and announce the library name as follows:
- #include "JoystickWin32Dll.h"
- #pragma comment(lib,"JoystickWin32Dll.lib")
step3: Edit your code, initialize the library in your program initialize zone or any position you need to initialize it:
- // TODO: Add your control notification handler code here
- // initialize the joystick library
- if(S_OK==InitDirectInput(m_hWnd))
- { // if initialize secceed, start a timer to get the state periodically, the cycle time is 100ms
- SetTimer(100,100,NULL);
- }
- else
- AfxMessageBox(_T("Initialze failed"));
step4: Edit your code, in OnTimer() function, update the joystick
state every time. And now we can get the current joystick state, and
put it in a structure JOYSTICKSTATE.
- JOYSTICKSTATE sta;
- if(S_OK == UpdateInputState(&sta))
- m_show.Format(_T("x=%d/t/ty=%d"),sta.lX,sta.lY);
- else
- m_show.Format(_T("Not Succeed!"));
if you still have some questions on using the library ,please review at
the tests program or you can contact me, email cbeast@gmail.com at your
service!
SRC
header file (the comments hear are writen in chinese, i will change it into english some day):
- /*************************************************************************************
- * 文 件:JoystickWin32Dll.h
- * 相关文件:JoystickWin32Dll.lib 导入库文件
- * JoystickWin32Dll.dll 二进制库文件
- *
- * 注意事项:本库采用visual C++ 2008开发,所以在没有visual studio 2008的机器上使用必须先安装
- * visual c++ 2008的可再分发包。与库一起提供使用。
- *
- * 说 明:本库是用来简化游戏杆开发的,将原本繁复的DirectInput技术进行了封装,
- * 封装为三个函数,分别是:
- * LRESULT InitDirectInput(HWND hwnd);初始化函数,用来初始化DirectInput库。
- * VOID FreeDirectInput();清理函数,用来在程序中清理DirectInput相关的数据。
- * HRESULT UpdateInputState( LPJOYSTICKSTATE pstate);更新状态函数,更新当前游戏杆状态数据。
- *
- * 作 者:林茂
- * 完成日期:2008-9-27
- * 修改记录:2008-9-27 21:00 完成库的开发
- * 2008-9-28 12:00 解决库在vc6上无法使用的问题
- * 2008-9-28 15:00 交付
- **************************************************************************************/
- // 下列 ifdef 块是创建使从 DLL 导出更简单的
- // 宏的标准方法。此 DLL 中的所有文件都是用命令行上定义的 JOYSTICKWIN32DLL_EXPORTS
- // 符号编译的。在使用此 DLL 的
- // 任何其他项目上不应定义此符号。这样,源文件中包含此文件的任何其他项目都会将
- // JOYSTICKWIN32DLL_API 函数视为是从 DLL 导入的,而此 DLL 则将用此宏定义的
- // 符号视为是被导出的。
- #ifdef JOYSTICKWIN32DLL_EXPORTS
- #define JOYSTICKWIN32DLL_API __declspec(dllexport)
- #else
- #define JOYSTICKWIN32DLL_API __declspec(dllimport)
- #endif
- typedef struct JOYSTICKSTATE {
- LONG lX; /* x-axis position */
- LONG lY; /* y-axis position */
- LONG lZ; /* z-axis position */
- LONG lRx; /* x-axis rotation */
- LONG lRy; /* y-axis rotation */
- LONG lRz; /* z-axis rotation */
- LONG rglSlider[2]; /* extra axes positions */
- DWORD rgdwPOV[4]; /* POV directions */
- BYTE rgbButtons[128]; /* 128 buttons */
- LONG lVX; /* x-axis velocity */
- LONG lVY; /* y-axis velocity */
- LONG lVZ; /* z-axis velocity */
- LONG lVRx; /* x-axis angular velocity */
- LONG lVRy; /* y-axis angular velocity */
- LONG lVRz; /* z-axis angular velocity */
- LONG rglVSlider[2]; /* extra axes velocities */
- LONG lAX; /* x-axis acceleration */
- LONG lAY; /* y-axis acceleration */
- LONG lAZ; /* z-axis acceleration */
- LONG lARx; /* x-axis angular acceleration */
- LONG lARy; /* y-axis angular acceleration */
- LONG lARz; /* z-axis angular acceleration */
- LONG rglASlider[2]; /* extra axes accelerations */
- LONG lFX; /* x-axis force */
- LONG lFY; /* y-axis force */
- LONG lFZ; /* z-axis force */
- LONG lFRx; /* x-axis torque */
- LONG lFRy; /* y-axis torque */
- LONG lFRz; /* z-axis torque */
- LONG rglFSlider[2]; /* extra axes forces */
- } JOYSTICKSTATE, *LPJOYSTICKSTATE;
- /// 检测是否游戏杆库是否初始化
- JOYSTICKWIN32DLL_API bool IsJoystickInitialized();
- /// 初始化游戏杆设备以及DirectInput库,
- /// 参数:HWND,游戏杆相关联的窗口句柄
- /// 返回值:如果成功则返回S_OK(零值),失败则返回错误码(非零值)
- JOYSTICKWIN32DLL_API LRESULT InitDirectInput(HWND hwnd);
- /// 清理库,释放游戏杆设备
- JOYSTICKWIN32DLL_API VOID FreeDirectInput();
- /// 更新游戏杆状态
- /// 参数:LPJOYSTICKSTATE pstate指向数据结构JOYSTICKSTATE的指针
- /// 返回值:如果成功则返回S_OK(零值),失败则返回错误码(非零值)
- JOYSTICKWIN32DLL_API HRESULT UpdateInputState( LPJOYSTICKSTATE pstate);
this is the first release version----20080929.