#include <stdio.h>
#include <malloc.h>
typedef struct LinkNode{
int coefficient;
int exponent;
struct LinkNode *next;
} *LinkList, *NodePtr;
//创建
LinkList initLinkList(){
LinkList tempHeader = (LinkList)malloc(sizeof(struct LinkNode));
tempHeader->coefficient = 0;
tempHeader->exponent = 0;
tempHeader->next = NULL;
return tempHeader;
}
void printList(LinkList paraHeader){
NodePtr p = paraHeader->next;
while (p != NULL) {
printf("%d * x^%d + ", p->coefficient, p->exponent);
p = p->next;
}
printf("\r\n");
}
void printNode(NodePtr paraPtr, char paraChar){
if (paraPtr == NULL) {
printf("NULL\r\n");
} else {
printf("The element of %c is (%d * x^%d)\r\n", paraChar, paraPtr->coefficient, paraPtr->exponent);
}
}
void appendElement(LinkList paraHeader, int paraCoefficient, int paraExponent){
NodePtr p, q;
q = (NodePtr)malloc(sizeof(struct LinkNode));
q->coefficient = paraCoefficient;
q->exponent = paraExponent;
q->next = NULL;
p = paraHeader;
while (p->next != NULL) {
p = p->next;
}
p->next = q;
}
void add(NodePtr paraList1, NodePtr paraList2){
NodePtr p, q, r, s;
p = paraList1->next;
printNode(p, 'p');
q = paraList2->next;
printNode(q, 'q');
r = paraList1;
printNode(r, 'r');
free(paraList2);
while ((p != NULL) && (q != NULL)) {
if (p->exponent < q->exponent) {
printf("case 1\r\n");
r->next = p;
r = p;
printNode(r, 'r');
p = p->next;
printNode(p, 'p');
} else if ((p->exponent > q->exponent)) {
printf("case 2\r\n");
r->next = q;
r = q;
printNode(r, 'r');
q = q->next;
printNode(q, 'q');
} else {
printf("case 3\r\n");
p->coefficient = p->coefficient + q->coefficient;
printf("The coefficient is: %d.\r\n", p->coefficient);
if (p->coefficient == 0) {
printf("case 3.1\r\n");
s = p;
p = p->next;
printNode(p, 'p');
} else {
printf("case 3.2\r\n");
r = p;
printNode(r, 'r');
p = p->next;
printNode(p, 'p');
}
s = q;
q = q->next;
free(s);
}
printf("p = %p, q = %p\r\n", p, q);
}
printf("End of while.\r\n");
if (p == NULL) {
r->next = q;
} else {
r->next = p;
}
printf("Addition ends.\r\n");
}
void additionTest1(){
LinkList tempList1 = initLinkList();
appendElement(tempList1, 7, 0);
appendElement(tempList1, 3, 1);
appendElement(tempList1, 9, 8);
appendElement(tempList1, 5, 17);
printList(tempList1);
LinkList tempList2 = initLinkList();
appendElement(tempList2, 8, 1);
appendElement(tempList2, 22, 7);
appendElement(tempList2, -9, 8);
printList(tempList2);
add(tempList1, tempList2);
printf("The result is: ");
printList(tempList1);
printf("\r\n");
}
void additionTest2(){
LinkList tempList1 = initLinkList();
appendElement(tempList1, 7, 0);
appendElement(tempList1, 3, 1);
appendElement(tempList1, 9, 8);
appendElement(tempList1, 5, 17);
printList(tempList1);
LinkList tempList2 = initLinkList();
appendElement(tempList2, 8, 1);
appendElement(tempList2, 22, 7);
appendElement(tempList2, -9, 10);
printList(tempList2);
add(tempList1, tempList2);
printf("The result is: ");
printList(tempList1);
printf("\r\n");
}
int main(){
additionTest1();
additionTest2();
printf("Finish.\r\n");
return 0;
}
【作业】多项式加法2.5
最新推荐文章于 2024-10-31 16:16:13 发布
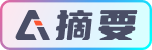