wordpress系统配置
If you’ve been developing websites with WordPress (including plugin and theme development) chances are you’ve heard these terms: Hooks
, Actions
, and Filters
. These are part of the Event-driven Architecture Pattern, which WordPress uses.
如果您一直在使用WordPress开发网站(包括插件和主题开发),那么您可能已经听说过以下术语: Hooks
, Actions
和Filters
。 这些是WordPress使用的事件驱动架构模式的一部分。
Are you new to WordPress development or finding it difficult to understand the basic concepts? I can’t recommend highly enough Simon Codrington’s Introduction to WordPress Plugin Development tutorial. He did a great job of explaining Actions
, and Filters
.
您是WordPress开发的新手还是发现难以理解基本概念? 我不能推荐足够高的Simon Codrington的 WordPress插件开发入门教程 。 他在解释Actions
和Filters
做得非常出色。
In this tutorial, I’ll be demystifying the WordPress hook system, leaving no stone unturned. Without further ado, let’s get started.
在本教程中,我将揭开WordPress钩子系统的神秘面纱,不遗余力。 事不宜迟,让我们开始吧。
挂钩,动作,过滤器。 这些是什么? (Hooks, Actions, Filters. What Are They?)
‘Hooks’ are basically events triggered by WordPress core, themes and plugins at various stages of their execution or interpretation by PHP. When these events are triggered, all the functions and/or class methods hooked or attached to them are executed in their correct order.
“挂钩”基本上是由WordPress核心,主题和插件在PHP执行或解释的各个阶段触发的事件。 当这些事件被触发时,挂钩或附加到它们的所有函数和/或类方法均以其正确顺序执行。
Hooks come in two forms, Actions and Filters. While the former is used to add and remove features or functionality at various stages of process execution, the latter modifies the behavior of various features and implementations. Don’t worry if you still don’t understand. You will when we start seeing some code examples below.
挂钩有两种形式,动作和过滤器。 前者用于在流程执行的各个阶段添加和删除功能部件,而后者则用于修改各种功能部件和实现的行为。 如果您仍然不了解,请不要担心。 当我们开始在下面看到一些代码示例时,您将看到。
WordPress中Hook系统的重要性 (Importance of the Hook System in WordPress)
The importance of the hook system in WordPress is simply extensibility. It makes it possible to add and remove features, as well as tweak/modify the implementation of features in WordPress core, plugins and themes.
钩子系统在WordPress中的重要性仅仅是可扩展性 。 它使添加和删除功能以及调整/修改WordPress核心,插件和主题中功能的实现成为可能。
When you write extensible plugins and themes, you make it possible for other developers to improve and extend them without ever editing the core source code.
在编写可扩展的插件和主题时,其他开发人员无需编辑核心源代码就可以改进和扩展它们。
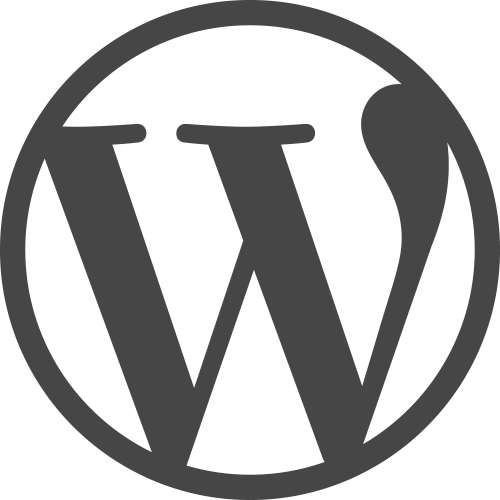
Allow me to cite an example. Unlike most payment gateways, my 2Checkout Payment Gateway for WooCommerce plugin does not include an icon displaying the supported credit card types on the checkout page because I felt it is unnecessary. But you know what; I added a filter
in case a user feels otherwise.
请允许我举一个例子。 与大多数付款网关不同,我的WooCommerce 2Checkout付款网关插件不包含在结帐页面上显示支持的信用卡类型的图标,因为我认为这是不必要的。 但是你知道吗? 我添加了一个filter
,以防用户感到不适。
It just so happened that we received a support request from a customer requesting for the inclusion of the icon. We were able to provide a code snippet to the customer that hooks into the filter and includes the icon.
碰巧的是,我们收到了客户的支持请求,要求添加图标。 我们能够为客户提供一个代码段,该代码段与过滤器相关联并包含图标。
深入研究WordPress挂钩系统 (Delving into the WordPress Hook System)
At various stages of WordPress execution, a large number of events are triggered commonly using the do_actions()
and apply_filters()
PHP functions. These events can be subscribed or hooked to via add_action()
and add_filter()
.
在WordPress执行的各个阶段,通常会使用do_actions()
和apply_filters()
PHP函数来触发大量事件。 这些事件可以通过add_action()
和add_filter()
进行订阅或挂接。
Please note the use of the word ‘commonly’. There are other ways events can be triggered. We’ll explore that in the second part of this tutorial.
请注意使用“普通”一词。 还有其他触发事件的方法。 我们将在本教程的第二部分中对此进行探讨。
Below is an example of an action
in a plugin. This action is fired after a successful user registration in my ProfilePress user registration plugin.
以下是插件中action
的示例。 在我的ProfilePress用户注册插件中成功注册用户后,将触发此操作。
/**
* Fires after a user registration is completed.
*
* @param int $form_id ID of the registration form.
* @param mixed $user_data array of registered user info.
* @param int $user_id ID of the registered user.
*/
do_action( 'pp_after_registration', $form_id, $user_data, $user_id );
During WordPress execution, all the functions hooked into this action will be processed.
在WordPress执行期间,将处理与该操作相关的所有功能。
An example of a filter hook is the_content
in WordPress core which filters every posts contents.
过滤器挂钩的一个示例是WordPress核心中的the_content
,它过滤每个帖子内容。
/**
* Filter the post content.
*
* @since 0.71
*
* @param string $content Content of the current post.
*/
$content = apply_filters( 'the_content', $content );
做记录 (Take Note)
In do_action()
, the first argument is the name of the action hook and subsequent arguments are variables available to functions that hook into the action.
在do_action()
,第一个参数是动作挂钩的名称,后续参数是可供挂钩到动作的函数使用的变量。
And in apply_filters()
, the first argument is the name of the filter hook, the second is the data or value on which the functions hooked to the filter are modified or applied. Subsequent arguments are variables/values available to functions that hook into the filter.
在apply_filters()
,第一个参数是过滤器挂钩的名称,第二个参数是修改或应用连接到过滤器的函数的数据或值。 后面的参数是挂钩到过滤器的函数可用的变量/值。
Don’t worry, all this will make more sense as we examine code examples.
不用担心,当我们检查代码示例时,所有这些将更有意义。
动作挂钩示例 (Action Hook Examples)
例子1 (Example #1)
Taking my ProfilePress plugin’s pp_after_registration
action for a spin; let’s say we want to implement a feature where users will receive an SMS (via an assumed messaging service called Dolio) welcoming them to your website immediately after registration. Our function hook could be in this form:
进行我的ProfilePress插件的pp_after_registration
操作; 假设我们要实现一项功能,使用户在注册后会立即收到一条SMS(通过称为Dolio的消息服务),欢迎他们访问您的网站。 我们的函数挂钩可以采用以下形式:
add_action( 'pp_after_registration', 'send_users_welcome_sms', 20, 3 );
function send_users_welcome_sms( $form_id, $user_data, $user_id ) {
global $service_locator;
$username = $user_data['username'];
$firstName = $user_data['first_name'];
$lastName = $user_data['last_name'];
$phoneNumber = $user_data['phone_number'];
$text = <<<SMS_CONTENT
Hello $firstName $lastName, Welcome to SitePoint. "\r\n"
User ID: $user_id "\r\n"
Username: $username "\r\n"
Password: The password you sign up with "\r\n"
SMS_CONTENT;
$dolio = $service_locator->get( 'dolio_sdk' );
$dolio->phone_number( $phoneNumber );
$dolio->sms_content( $text );
$dolio->send();
}
The third argument of add_action
in the code above is the hook priority which specifies the order in which the function hooked to pp_after_registration
action will be executed. Leaving this empty will default to 10
. While the fourth argument specifies the number of arguments the function hook will accept. Default to 1
if empty.
上面代码中add_action
的第三个参数是挂接优先级,该优先级指定了挂接到pp_after_registration
动作的函数的执行顺序。 保留为空将默认为10
。 第四个参数指定函数挂钩将接受的参数数量。 如果为空,则默认为1
。
Assuming I left out the fourth argument, thus defaulting to 1
, the $user_data
and $user_id
variable will be null
because we only told the function to accept just one argument.
假设我省略了第四个参数,因此默认为1
,则$user_data
和$user_id
变量将为null
因为我们只告诉函数仅接受一个参数。
范例#2 (Example #2)
WordPress includes the following action
hooks — wp_head
and wp_footer
— that are both triggered in the head tag and before the closing body tag on the front end respectively.
WordPress包含以下action
挂钩wp_head
和wp_footer
,它们分别在head标签和前端的wp_footer
body标签之前触发。
These hooks can be used to display script and data at those strategic locations.
这些挂钩可用于在那些关键位置显示脚本和数据。
Let’s check out some code examples.
让我们看看一些代码示例。
The code below uses wp_head
to include Google’s site verification meta tag to the header of the WordPress front end.
下面的代码使用wp_head
将Google的站点验证元标记包含到WordPress前端的标头中。
add_action( 'wp_head', 'google_site_verification' );
function google_site_verification() {
echo '<meta name="google-site-verification" content="ytl89rlFsAzH7dWLs_U2mdlivbrr_jgV4Gq7wClHDUJ8" />';
}
All hook functions will be anonymous in lieu of the named function to avoid unnecessary repetition of function’s names. For example, the code for Google site verification Meta tag above will now become:
所有挂钩函数都将是匿名的,代替命名函数,以避免不必要的函数名称重复。 例如,上面的Google网站验证元标记的代码将变为:
add_action( 'wp_head', function () {
echo '<meta name="google-site-verification" content="ytl89rlFsAzH7dWLs_U2mdlivbrr_jgV4Gq7wClHDUJ8" />';
});
The code below uses wp_footer
to add JavaScript in the footer area of WordPress front end.
下面的代码使用wp_footer
在WordPress前端的页脚区域中添加JavaScript。
add_action( 'wp_footer', function () {
echo '<script type="text/javascript" src="http://example.com/wp-content/plugins/site-specific-plugin/hello-bar.js"></script>';
});
Enough of the action hooks code example, let’s check out filters.
动作钩子代码示例足够多,让我们看看过滤器。
滤钩示例 (Filter Hook Examples)
例子1 (Example #1)
Say we are developing an ad inserter
plugin that will programmatically insert ads before and after every post content, the the_content
filter is what we need.
假设我们正在开发一个ad inserter
插件,该插件将以编程方式在每个帖子内容前后插入广告,因此我们需要the_content
过滤器。
The code below includes the text ‘We love SitePoint’ before and after every post content.
下面的代码在每个帖子内容前后都包含“ We love SitePoint”文本。
add_filter( 'the_content',
function ( $content ) {
$text = sprintf( '<div class="notice alert">%s</div>', __( 'We love SitePoint', 'sp' ) );
$content = $text . $content . $text;
return $content;
});
Code explanation: the content of $text
variable is the same as <div class="notice alert">We love SitePoint</div>
albeit internationalized so that it can be localized. Confused? Please take a look at my tutorials on WordPress i18n and l10n.
代码说明: $text
变量的内容与<div class="notice alert">We love SitePoint</div>
尽管它已经国际化,所以可以本地化。 困惑? 请看一下我关于WordPress i18n和l10n的教程。
Mind you, the function parameter $content
, is the variable that supplies the post content.
请注意,函数参数$content
是提供帖子内容的变量。
We then append the custom text before and after the post content, save the resultant data to $content
and subsequently return it.
然后,我们在帖子内容前后添加自定义文本,将结果数据保存到$content
,然后返回。
Note: all filter
hook functions must return the variable parameter after manipulation or modification.
注意:所有filter
挂钩函数必须在操作或修改后返回变量参数。
范例#2 (Example #2)
Another filter example we’ll look at is the_title
. Below is how it is defined in line 158 of wp-includes/post-template.php
.
我们将看到的另一个过滤器示例是the_title
。 以下是wp-includes/post-template.php
第158行中的定义方式。
/**
* Filter the post title.
*
* @since 0.71
*
* @param string $title The post title.
* @param int $id The post ID.
*/
return apply_filters( 'the_title', $title, $id );
The code below modifies only the title of a post with ID 5978 by appending - WeLoveSitePoint
to it. This is possible thanks to the $id
argument.
下面的代码通过在其后面附加- WeLoveSitePoint
来仅修改ID为5978的帖子的标题。 这要归功于$id
参数。
add_filter( 'the_title', function ( $title, $id ) {
if ( $id == '5978' ) {
$title .= ' - WeLoveSitePoint';
}
return $title;
}, 10, 2
);
结论 (Conclusion)
The reason WordPress continues to be the leading content management system is because of its extensibility.
WordPress之所以继续成为领先的内容管理系统的原因是其可扩展性。
The WordPress Hook system has made it possible for WordPress to be transformed into powerful web applications, whether that be an ecommerce store with WooCommerce, a forum with bbPress or even a social networking site with BuddyPress.
WordPress Hook系统使WordPress可以转换为强大的Web应用程序,无论是WooCommerce的电子商务商店,bbPress的论坛还是BuddyPress的社交网站。
In Part 2 of this tutorial, I will sharing some cool and little known facts about the hook system in WordPress such as how to: use them in a class, hook static and non-static methods to actions and filters, use namespaces, its caveats and solutions and a whole lot more. So stay tuned and happy coding.
在本教程的第2部分中,我将分享有关WordPress中钩子系统的一些鲜为人知的鲜为人知的事实,例如:如何在类中使用它们,将静态和非静态方法钩接到操作和过滤器,使用名称空间,其警告和解决方案等等。 因此,请继续关注并愉快地编码。
wordpress系统配置