wordpress 代码
We can do a lot of different things with shortcodes in WordPress, as we explored in our article describing the WordPress Shortcode API. However, by default, WordPress only allows the use of shortcodes in posts (and pages), and not anywhere else.
正如我们在描述WordPress短代码API的文章中所探讨的, 我们可以使用WordPress中的短代码来做很多事情。 但是,默认情况下,WordPress仅允许在帖子(和页面)中使用简码,而在其他任何地方均不允许。
If you want to use shortcodes in widgets, it’s not possible by default. However, in this quick tip, I’ll cover how you can enable this functionality.
如果要在小部件中使用短代码,则默认情况下是不可能的。 但是,在本快速提示中,我将介绍如何启用此功能。
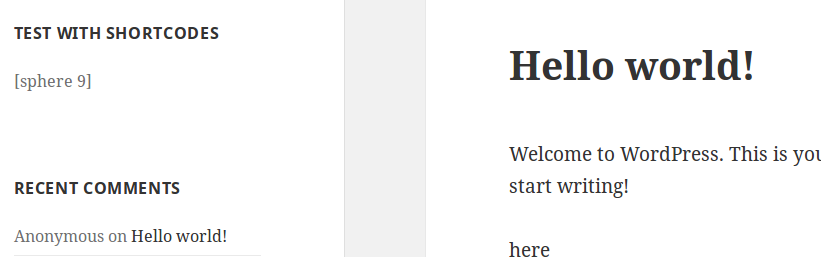
允许在文本小部件中使用简码 (Allowing the Use of Shortcodes in the Text Widget)
WordPress provides several widgets by default. One of them is the ‘Text’ widget, which, as its name suggests, allows you to add any text to a widget. You can also use it to add any HTML code.
WordPress默认提供了几个小部件。 其中之一是“文本”小部件,顾名思义,它允许您将任何文本添加到小部件。 您也可以使用它添加任何HTML代码。
That means that you can also play with JavaScript code in this widget, so it’s pretty powerful. However, if you need more, like a PHP script to access some data stored in the server, this widget won’t help you by default.
这意味着您也可以在此小部件中使用JavaScript代码,因此它非常强大。 但是,如果您需要更多(例如PHP脚本)来访问服务器中存储的某些数据,则默认情况下,此小部件将无济于事。
It’s similar to a post. That’s why, like in a post, we would like to be able to use shortcodes to do anything we want. To do that, we can use the widget_text
filter. This filter is called to allow modifications in the content of a ‘Text’ widget. We’ll use it here to ask WordPress to parse shortcodes in this widget.
这类似于帖子。 这就是为什么像在帖子中一样,我们希望能够使用短代码来完成我们想要的任何事情。 为此,我们可以使用widget_text
过滤器。 调用此过滤器以允许修改“文本”窗口小部件的内容。 我们将在这里使用它来要求WordPress解析此小部件中的短代码。
Parsing shortcodes in WordPress is achieved thanks to the do_shortcode()
function. It accepts one required parameter, the text to parse, and it returns the parsed text. That means that we can directly use this function as a callback function in the widget_text
filter.
do_shortcode()
函数,可以在WordPress中解析短代码。 它接受一个必需的参数,即要解析的文本,并返回已解析的文本。 这意味着我们可以直接在widget_text
过滤器中将此函数用作回调函数。
The code below can be used in a plugin file or in the functions.php
file of your theme.
以下代码可以在主题的插件文件中或在functions.php
文件中使用。
<?php
add_filter('widget_text', 'do_shortcode');
?>
And we’re done. Now, any existing shortcode you type in the ‘Text’ widget will be parsed.
我们完成了。 现在,您在“文本”小部件中键入的所有现有简码都将被解析。
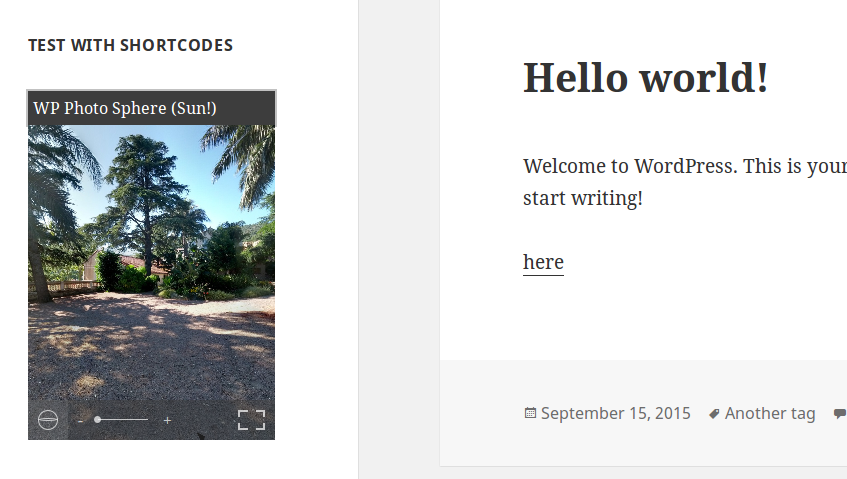
创建一个新的简码小部件 (Creating a New Shortcodes Widget)
Alternatively, we can also create our own widget. As the default ‘Text’ widget works perfectly, we can simply adapt its code from the following (found in the /wp-includes/default-widgets.php
file). It’s worth noting that we should create our own plugin, never modify core WordPress files.
另外,我们也可以创建自己的小部件。 由于默认的“文本”窗口小部件可以完美工作,因此我们可以从以下代码(在/wp-includes/default-widgets.php
文件中找到)简单地改编其代码。 值得注意的是,我们应该创建自己的插件,而不要修改核心WordPress文件。
<?php
class WP_Widget_Text extends WP_Widget {
public function __construct() {
$widget_ops = array('classname' => 'widget_text', 'description' => __('Arbitrary text or HTML.'));
$control_ops = array('width' => 400, 'height' => 350);
parent::__construct('text', __('Text'), $widget_ops, $control_ops);
}
/**
* @param array $args
* @param array $instance
*/
public function widget( $args, $instance ) {
/** This filter is documented in wp-includes/default-widgets.php */
$title = apply_filters( 'widget_title', empty( $instance['title'] ) ? '' : $instance['title'], $instance, $this->id_base );
/**
* Filter the content of the Text widget.
*
* @since 2.3.0
*
* @param string $widget_text The widget content.
* @param WP_Widget $instance WP_Widget instance.
*/
$text = apply_filters( 'widget_text', empty( $instance['text'] ) ? '' : $instance['text'], $instance );
echo $args['before_widget'];
if ( ! empty( $title ) ) {
echo $args['before_title'] . $title . $args['after_title'];
} ?>
<div class="textwidget"><?php echo !empty( $instance['filter'] ) ? wpautop( $text ) : $text; ?></div>
<?php
echo $args['after_widget'];
}
/**
* @param array $new_instance
* @param array $old_instance
* @return array
*/
public function update( $new_instance, $old_instance ) {
$instance = $old_instance;
$instance['title'] = strip_tags($new_instance['title']);
if ( current_user_can('unfiltered_html') )
$instance['text'] = $new_instance['text'];
else
$instance['text'] = stripslashes( wp_filter_post_kses( addslashes($new_instance['text']) ) ); // wp_filter_post_kses() expects slashed
$instance['filter'] = ! empty( $new_instance['filter'] );
return $instance;
}
/**
* @param array $instance
*/
public function form( $instance ) {
$instance = wp_parse_args( (array) $instance, array( 'title' => '', 'text' => '' ) );
$title = strip_tags($instance['title']);
$text = esc_textarea($instance['text']);
?>
<p><label for="<?php echo $this->get_field_id('title'); ?>"><?php _e('Title:'); ?></label>
<input class="widefat" id="<?php echo $this->get_field_id('title'); ?>" name="<?php echo $this->get_field_name('title'); ?>" type="text" value="<?php echo esc_attr($title); ?>" /></p>
<p><label for="<?php echo $this->get_field_id( 'text' ); ?>"><?php _e( 'Content:' ); ?></label>
<textarea class="widefat" rows="16" cols="20" id="<?php echo $this->get_field_id('text'); ?>" name="<?php echo $this->get_field_name('text'); ?>"><?php echo $text; ?></textarea></p>
<p><input id="<?php echo $this->get_field_id('filter'); ?>" name="<?php echo $this->get_field_name('filter'); ?>" type="checkbox" <?php checked(isset($instance['filter']) ? $instance['filter'] : 0); ?> /> <label for="<?php echo $this->get_field_id('filter'); ?>"><?php _e('Automatically add paragraphs'); ?></label></p>
<?php
}
}
?>
We don’t have a lot of details to change here. The first thing to change is the name of the class. I chose to name it WP_Widget_Shortcodes
but feel free to choose any name you want. As the constructor of this class sets some information about the widget itself, we also need to modify it.
我们在这里没有很多要更改的细节。 首先要更改的是类的名称。 我选择将其命名为WP_Widget_Shortcodes
但可以随意选择您想要的任何名称。 当此类的构造函数设置有关小部件本身的一些信息时,我们还需要对其进行修改。
<?php
public function __construct() {
$widget_ops = array('classname' => 'widget_shortcodes', 'description' => __('Arbitrary text or HTML with shortcodes.'));
$control_ops = array('width' => 400, 'height' => 350);
parent::__construct('shortcodes', __('Shortcodes'), $widget_ops, $control_ops);
}
?>
The other thing to change is in the widget()
method which describes to WordPress how to display the widget. We change the content of the $text
variable which contains the text to display. We remove the call to the widget_text
filter, and we apply the do_shortcode()
function to this content.
要更改的另一件事是在widget()
方法中,该方法向WordPress描述了如何显示小部件。 我们更改$text
变量的内容,该变量包含要显示的文本。 我们删除对widget_text
过滤器的调用,并将do_shortcode()
函数应用于此内容。
<?php
$text = empty( $instance['text'] ) ? '' : do_shortcode($instance['text']);
?>
We then need to register our widget, to be able to add it like any other widget. This can be achieved thanks to the widgets_init
action triggered once WordPress registered the default widgets.
然后,我们需要注册我们的小部件,以便能够像其他任何小部件一样添加它。 由于WordPress注册了默认小部件后触发了widgets_init
操作,因此可以实现这一目标。
<?php
add_action('widgets_init', function() {
register_widget('WP_Widget_Shortcodes');
});
?>
Now you can find our widget in the list of the available widgets. You can add it to any compatible zone, and it will interpret any shortcode you use.
现在,您可以在可用小部件的列表中找到我们的小部件。 您可以将其添加到任何兼容区域,并且它将解释您使用的任何短代码。
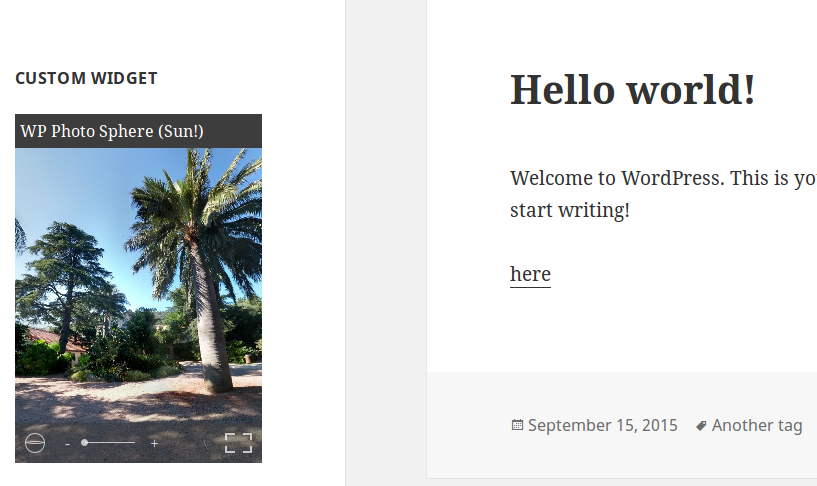
结束语 (Closing Words)
As we saw above, enabling the use of shortcodes in widgets is not very complicated, but you need to be careful. In fact, not every shortcode will fit in the place where widgets are displayed. If the shortcode has a fixed width, that can be a problem.
正如我们在上面看到的,在小部件中启用短代码并不是很复杂,但是您需要小心。 实际上,并非每个短代码都适合显示小部件的位置。 如果简码具有固定的宽度,则可能会出现问题。
Note that the changes we used in the default ‘Text’ widget are minimal. You can change anything else if you want to customize your widget. You can retrieve the filter we used and the widget we created in an example plugin available to download here if you’d like to experiment further.
请注意,我们在默认的“文本”小部件中使用的更改很小。 如果要自定义窗口小部件,则可以更改其他任何内容。 如果您想进一步试验,可以在示例插件中检索我们使用的过滤器和我们创建的小部件,可以在此处下载。
翻译自: https://www.sitepoint.com/quick-tip-use-shortcodes-within-widgets-wordpress/
wordpress 代码