php中向量化编程
Finagle’s Law of Dynamic Negatives: Anything that can go wrong, will – at the worst possible moment.
Finagle的动态负数定律 : 在最糟糕的时刻,任何会出错的都会。
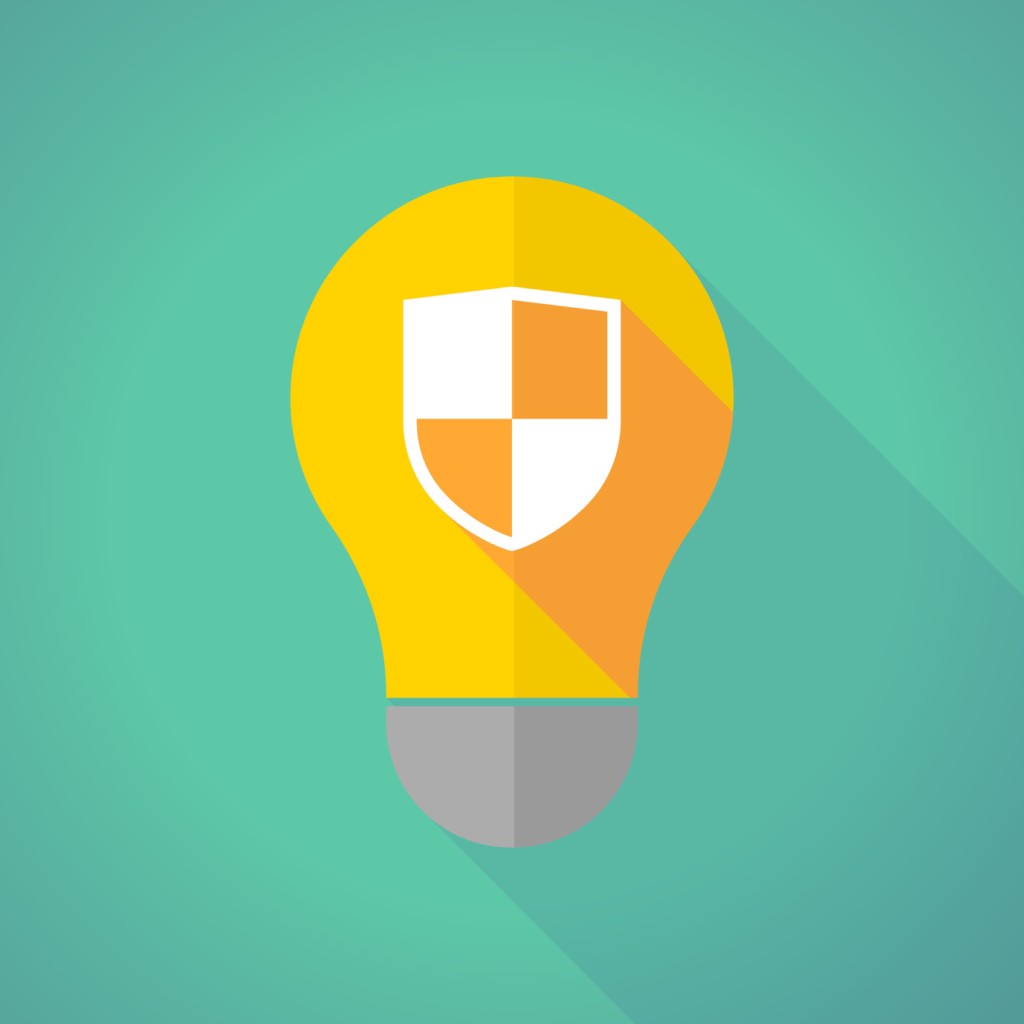
“防御性编程”是什么意思? (What Does “Defensive Programming” Mean?)
Defensive programming, simply put, is programming with the intent to anticipate likely failure points. The goal is to circumvent those likely problems before they occur. You see the problem, right? There’s something inherently difficult with the advice “expect the unexpected” and it’s made many times worse when one alters it to “expect the unexpected and try to prevent it”.
简而言之,防御性编程是为了预期可能的故障点而进行的编程。 目的是在可能出现的问题发生之前就规避它们。 你看到问题了吧? “预期意外”的建议存在一些内在的困难,而当人们对其进行更改以“预期意外并试图阻止它”时,它变得更加糟糕。
Let’s look at some practical examples.
让我们看一些实际的例子。
条件语句 (Conditional Statements)
This is one of the easiest places to program defensively, and one where it’s easiest to be complacent. In many cases while programming in PHP, you simply won’t need an “else” case.
这是最容易进行防御性编程的地方之一,也是最容易沾沾自喜的地方。 在许多情况下,使用PHP编程时,您根本不需要“ else”情况。
Hypothetically, you are working on a function and need a conditional. You only have three possible states for your particular variable at this point – and you have those covered in a block like this:
假设地,您正在处理一个函数,并且需要一个条件。 此时,您的特定变量只有三种可能的状态–像这样的块中覆盖了这些状态:
if($var == a){ }
else if($var == b){ }
else if($var == c){ }
There are no other possibilities, you say, and you move on with your code. Let me stop you there, though. I know that you know there are no other possibilities. And I believe you. But sometimes (unexpected) things happen. We forget about something. We look over mistakes. We end up reusing a bit of code outside of its originally intended scope. And all of a sudden we have leaking and sometimes silent error states because there’s no catch here. Make use of else
blocks. Make use of default
in switch
es. Use them to return or log an error so that you’re aware of what’s happened, should it ever happen. It may be an extra couple of lines of code, but it’s worth it when something happens that you did not expect.
您说,没有其他可能性,然后继续进行代码。 不过,让我在那里阻止您。 我知道您知道没有其他可能性。 我相信你。 但是有时候(意想不到的)事情发生了。 我们忘记了一些事情。 我们审视错误。 我们最终在原先打算的范围之外重用了一些代码。 突然之间,我们出现了泄漏,有时甚至是无声的错误状态,因为这里没有问题。 利用else
块。 在switch
es中使用default
。 使用它们返回或记录错误,以便您知道发生了什么(如果有发生)。 这可能需要额外的几行代码,但是当您发生意外的事情时,这是值得的。
永不信任用户输入 (Never Trust User Input)
Have you heard this statement before? Most programmers have. It is a bit of a vague, generalized thing to say, granted. But it’s true. You should never trust user input. This does not mean that you assume that all users are crazed hackers out to destroy your application with a single set of well crafted commands. There is no need for paranoia. But, you should assume that users don’t know your code. They don’t know what parameters you need filled or how long the input can be. They don’t know what file types they can upload (even if the app tells them) or what size. And occasionally, they are a bot or a hacker and they are going to be trying to run scripts in their inputs. Sometimes even from inputs behind a login wall. How do you know when you can trust things like authentication or captchas to provide a safe barrier before users arrive at input forms?
您以前听过这句话吗? 大多数程序员都有。 说,这有点含糊,笼统。 但这是真的。 您永远不应信任用户输入。 这并不意味着你认为所有的用户都疯狂的黑客攻击与一组精心设计的命令摧毁你的应用程序。 不需要妄想症。 但是,您应该假设用户不知道您的代码。 他们不知道您需要填写哪些参数或输入可以输入多长时间。 他们不知道可以上传哪种文件类型(即使应用程序告诉了他们)或大小。 有时,他们是机器人还是黑客,他们将尝试在输入中运行脚本。 有时甚至来自登录墙后面的输入。 您如何知道何时可以信任身份验证或验证码之类的东西来在用户到达输入表单之前提供安全屏障?
The answer: Never.
答案:永远不会。
If you never trust user input, then you never have a breach because you trusted user input. See? So always validate your input, and always ensure that you’re using appropriate techniques when handling data, especially when storing it in the database or retrieving it for display. For that matter – don’t trust input at all, even when from somewhere besides your users – input validation is always your friend. Check out Survive the Deep End: PHP Security and look into using a validation library.
如果您从不信任用户输入,那么您就永远不会违反协议, 因为您信任用户输入。 看到? 因此,在处理数据时,尤其是在将数据存储在数据库中或检索以进行显示时,请务必验证输入,并始终确保使用适当的技术。 因此,即使在用户之外的任何地方也完全不信任输入,输入验证始终是您的朋友。 查看Survive the Deep End:PHP安全性,并研究使用验证库 。
关于您的代码的假设 (Assumptions About Your Code)
Don’t assume anything. If the previous two topics teach us anything, it’s that we should not make any assumptions. As programmers, especially when focusing on a single project for too long, we begin to assume a lot. We begin to assume that the user knows some of the things we know. Not necessarily technical details, but functional details about the program. We assume that the user will know how big files can be because… we already know that fact. Or that they’ll know that in order for the mailing script to… but no, they have no idea about any of that. This can sometimes matter more for front-end work, but obviously you still deal with user behavior and user input in the back-end as well, so it’s worth thinking about.
不要承担任何责任 。 如果前两个主题教给我们任何东西,那就是我们不应做任何假设。 作为程序员,尤其是在专注于单个项目的时间过长时,我们开始承担很多责任。 我们开始假设用户知道一些我们知道的东西。 不一定是技术细节,而是有关程序的功能细节。 我们假设用户将知道文件的大小,因为……我们已经知道了这一事实。 否则,他们会知道为了使邮件脚本运行……但不,他们对此一无所知。 有时这对于前端工作可能更为重要,但是显然您仍然需要处理后端的用户行为和用户输入,因此值得考虑。
Another staggering assumption that many programmers make is the assumption of the obvious nature of our functions, classes, or whatever other bit of code we’re working on at any given time. A defensive programmer will try to think carefully about not only normal documentation and describing what a bit of functionality does – but they will also document any assumptions they are making about input, parameters, use cases, or any number of other similar things. Because we are all humans, and we sometimes forget things later. We also will all most likely end up with someone maintaining, extending, or replacing our code someday. If nothing else, recall that programming is happening in a world full of technological changes. If your application is still around in several years, it may need to be updated to a newer version of PHP and lose some of its functionality, or any number of components that it interacts with may change and necessitate changes in your own code. It is very difficult to predict these things, so good comments and documentation are very important.
许多程序员做出的另一个令人震惊的假设是,我们的函数,类或我们在任何给定时间正在处理的任何其他代码的明显性质的假设。 防御性程序员不仅会尝试仔细考虑常规文档并描述一些功能的作用,而且还将记录他们对输入,参数,用例或许多其他类似事物所做的任何假设。 因为我们都是人类,有时我们以后会忘记事情。 我们也很可能最终有一天会有人维护,扩展或替换我们的代码。 如果没有别的,回想一下编程是在充满技术变化的世界中发生的。 如果您的应用程序仍存在数年,则可能需要将其更新到PHP的较新版本,并失去某些功能,或者与之交互的任何数量的组件都可能会更改,因此您必须更改自己的代码。 预测这些情况非常困难,因此好的注释和文档非常重要。
管视角 (Tunnel Vision)
Another thing that can cause us to both forget good commenting practices as well as standards is tunnel vision. Tunnel vision happens a lot to programmers. You know the feeling. You’re solving a problem, you’re in the groove. You’re feeling isolated in your own little world, with your music (or lack thereof) and you’re just coding and all of a sudden two hours have passed since you last checked the clock and you realize that you’ve written countless lines of code without any comments. It happens to all of us at one time or another, but the important thing is to, at some point, catch that and add some where appropriate.
可能使我们同时忘记良好评论实践和标准的另一件事是隧道愿景。 程序员对隧道的愿景经常发生。 你知道那种感觉。 您正在解决问题,陷入困境。 您在自己的小世界里感到孤独,音乐(或缺乏音乐)使您感到孤独,而您只是在编码 ,自上次检查时钟以来突然过去了两个小时,并且您意识到自己已经写了无数行没有任何注释的代码。 这一次或一次都发生在我们所有人身上,但是重要的是在某个时候抓住它并在适当的地方添加一些。
语法和命名的一致性 (Consistency in Syntax and Naming)
Consistency is a bit of a grey area – that delves a bit more into coding standards and the like, but it is relevant to defensive programming. In PHP, there are standards that can be followed to streamline your code for others who might view it, or for your own future use. But often, no one is actually making you code to standard. However, whether you’re coding to some set of standards or not, you should at very least be internally consistent – because it will make you less error prone. This applies especially to small syntax errors that take an aggravating amount of time to return to and fix after being caught. If you always use the same spacing, the same formats and syntax, naming conventions, etc., than you are less likely to make a mistake resulting in your misreading of your own code. You are also more likely to be able to quickly scan and find things that you need to find.
一致性是一个灰色地带,它会更多地研究编码标准等,但它与防御性编程有关。 在PHP中,可以遵循一些标准来为可能查看它的其他人或您自己将来的使用简化代码。 但是通常,没有人真正使您的代码达到标准。 但是,无论您是否按照某些标准进行编码,都至少应在内部保持一致–因为这将使您减少出错的可能性。 这尤其适用于较小的语法错误,这些错误在被捕获后需要花费大量时间才能返回并修复。 如果您始终使用相同的间距,相同的格式和语法,命名约定等,那么您出错的可能性就较小,从而导致您误读自己的代码。 您也更有可能能够快速扫描并找到需要查找的内容。
结论 (Conclusion)
Beyond user behaviors and actions, don’t assume anything in general about your program. Assumptions are one of the biggest enemies of a defensive programmer. Don’t assume you won’t need that default case or else statement. Don’t avoid creating appropriate user error messages, alerts, logs, and whatever else you need just because you assume they won’t be needed. Your assumptions are often right – but we do not care about that. What we care about are the times that they are wrong. Always plan as though you may need to return to your code in a few hours, weeks, months or even years, or that someone else will – and document it accordingly. Don’t assume it will never need to be updated, extended, or maintained. That’s naive at best, and negligent in more cases than not. Sometimes just keeping the idea of defensive programming in the back of your mind can help you to estimate, plan, and program more effectively and more safely.
除了用户的行为和动作外,不要对程序有任何一般性的假设。 假设是防御型程序员的最大敌人之一。 不要假设您不需要该默认大小写或else语句。 不要仅仅因为您认为不需要它们而创建适当的用户错误消息,警报,日志以及其他所需的内容。 您的假设通常是正确的-但我们对此并不在乎。 我们关心的是他们错了的时代。 始终进行计划,好像您可能需要在几个小时,几周,几个月甚至几年内返回代码,否则其他人会–并对其进行记录。 不要以为它永远不需要更新,扩展或维护。 充其量是幼稚的,而且在更多情况下是疏忽大意。 有时,只是将防御性编程的想法放在脑海中,可以帮助您更有效,更安全地进行估计,计划和编程。
翻译自: https://www.sitepoint.com/defensive-programming-in-php/
php中向量化编程