javascript绘制圆
Some time ago I see this fun demo in Codepen, by Akimitsu Hamamuro. Then I think that it would be great to be able to draw things programmatically in the web. Unfortunately, I could not find any library to do it, so I've developed one, using that pen as base.
前段时间,我在Akamitsu Hamamuro的Codepen中看到了这个有趣的演示 。 然后,我认为能够以编程方式在网络上绘制东西将是很棒的。 不幸的是,我找不到任何可做的图书馆,所以我已经开发了一个以该笔为基础的图书馆。
In this tutorial, we'll see how to use this new library to draw creative brushstrokes directly in the browser with a nice API. We'll be able to draw solid colors, images, or even HTML!
在本教程中,我们将看到如何使用这个新库通过一个不错的API在浏览器中直接绘制创意笔触。 我们将能够绘制纯色,图像甚至HTML!
Specifically, we will be learning how to build a creative poster like this:
具体来说,我们将学习如何制作像这样的创意海报:

入门 ( Getting Started )
Getting started with the Brushstroke
library is pretty simple. You only need to include the script and start drawing things:
Brushstroke
库的入门非常简单。 您只需要包括脚本并开始绘图即可:
<!-- Optional dependencies goes here -->
<script src="dist/brushstroke.min.js"></script>
<script>
// Options for customization
var options = {
duration: 1,
queue: true
};
// Initialization
var bs = new Brushstroke(options);
// Draw, erase, etc...
bs.draw();
bs.erase();
bs.draw();
</script>
This is just a very basic example. You can find a detailed documentation in the github repo.
这只是一个非常基本的例子。 您可以在github repo中找到详细的文档。
绘制背景图像 ( Drawing the Background Image )
As you may see, our poster consists of a background image and a text. Let's see how to animate the drawing of the background image.
如您所见,我们的海报由背景图片和文字组成。 让我们看看如何为背景图片的绘制制作动画。
First we need to initialize a new Brushstroke
instance for the background image, with all the options we want. Please learn more about each option in the github repo.
首先,我们需要使用所需的所有选项为背景图像初始化一个新的Brushstroke
实例。 请在github repo中了解有关每个选项的更多信息。
// Declaring variables
var width = window.innerWidth || document.body.clientWidth;
var height = window.innerHeight || document.body.clientHeight;
var optionsBackground, bsBackground;
// Random curves for background
optionsBackground = {
animation: 'points',
points: 10,
inkAmount: 5,
size: 300,
frames: 10,
frameAnimation: true,
splashing: false,
image: 'images/background.jpg',
centered: true,
queue: true,
width: width,
height: height
};
bsBackground = new Brushstroke(optionsBackground);
Now we can start drawing things, and more! Let's see how to achieve the effect we want for our poster:
现在我们可以开始画图了,还有更多! 让我们看看如何实现我们想要的海报效果:
// Function to start the animation
function runAnimation() {
// Draw a straight line
bsBackground.draw({
points: [0, height / 2 - 40, width, height / 3]
});
// Draw another straight line
bsBackground.draw({
points: [width, height / 2, 0, height / 1.5 - 40]
});
// Draw a curve generated using 20 random points
bsBackground.draw({
inkAmount: 3,
frames: 100,
size: 200,
splashing: true,
points: 20
});
}
// Start
runAnimation();
绘制文字 ( Drawing the Text )
Our library is not able to draw text as is, but it can draw along any SVG path
provided. So we can draw our text as SVG paths, and then we can pass the paths to the library. For this demo, we've used the "Hershey Text" extension available in the Inkscape vector editor (from version 0.91 or newer). You can find more info about it here.
我们的库无法按原样绘制文本,但可以沿提供的任何SVG path
进行绘制。 因此,我们可以将文本绘制为SVG路径,然后将路径传递给库。 在此演示中,我们使用了Inkscape矢量编辑器中的“ Hershey Text”扩展名(0.91版或更高版本)。 您可以在此处找到有关它的更多信息。
After render, scale up, and simplify the paths (Ctrl + L
) to create smother shapes of our text, our SVG looks like this:
渲染,放大并简化路径( Ctrl + L
)以创建文本的更平滑形状后,SVG如下所示:
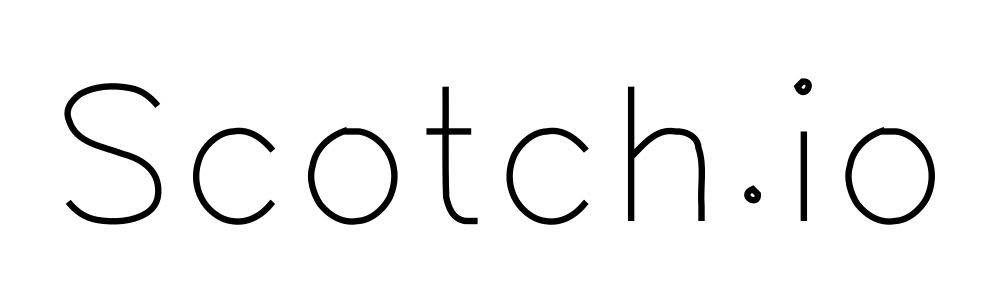
Now we have our text as single-stroke SVG paths, and we can use them to draw with the Brushstroke
library!
现在,我们将文本作为单笔画SVG路径,并且可以使用它们通过Brushstroke
库进行绘制!
First, we need to include the SVG in the HTML:
首先,我们需要在HTML中包含SVG:
<!-- Each path is a letter in "Scotch.io" string -->
<svg width="1000" height="300" style="display: none">
<path d="m157.76 105.88c-7.4053-8.9862-16.122-15.85-27.865-17.818-16.604-3.2803-35.244-2.2435-50.148 6.2719-8.4329 6.4228-15.194 15.018-10.757 25.919 6.9636 23.286 33.81 26.063 53.267 33.499 15.84 4.0064 32.554 13.238 35.503 30.658 1.8468 11.869-0.78168 21.884-11.233 28.659-14.576 8.9259-33.167 9.5932-49.689 6.8414-12.29-2.3318-20.767-8.5079-28.636-18.075"/>
<path d="m272.9 150.66c-9.8598-11.768-23.423-22.368-39.709-19.191-13.362 0.5304-25.61 11.328-31.775 22.54-6.7138 13.934-7.1853 29.748-0.25697 43.707 5.5237 11.364 17.648 21.717 30.492 23.311 15.813 2.8302 30.126-5.4593 39.688-17.63l1.2482-1.2482 0.31262-0.31262"/>
<path d="m343.27 131.47c-15.039 5.7827-27.96 17.873-30.612 34.262-4.0296 12.888 0.41254 27.839 7.52 38.602 9.0267 11.179 22.414 19.394 37.213 16.695 13.362-0.53038 25.61-11.328 31.775-22.54 6.7139-13.934 7.1853-29.748 0.25697-43.707-5.5237-11.364-17.648-21.717-30.492-23.311h-15.661z"/>
<path d="m445.62 86.692c0.37493 36.778-0.74805 73.798 0.55819 110.42 2.5876 11.039 6.138 21.243 18.469 23.832 4.2802 0.18855 8.6454 0.0288 12.958 0.0819m-51.176-89.559h44.779"/>
<path d="m586.36 150.66c-9.8598-11.768-23.423-22.368-39.709-19.191-13.362 0.5304-25.61 11.328-31.775 22.54-6.7139 13.934-7.1853 29.748-0.25697 43.707 5.5237 11.364 17.648 21.717 30.492 23.311 15.813 2.8302 30.126-5.4593 39.688-17.63l1.2482-1.2482 0.31262-0.31262"/>
<path d="m631.14 86.692v134.34m0-63.971c12.158-12.114 24.92-29.2 44.341-25.588 10.207-0.53645 22.347 4.9455 22.978 16.444 6.3533 18.657 1.559 39.122 3.0479 58.568v14.546"/>
<path d="m752.68 189.04c-15.025 6.5899 6.2843 18.544 5.1489 5.1489l-5.1489-5.1489z"/>
<path d="m797.46 86.692c6.5899 15.025 18.544-6.2843 5.1489-5.1489l-5.1489 5.1489zm6.3971 44.779v89.559"/>
<path d="m880.62 131.47c-15.039 5.7827-27.96 17.873-30.612 34.262-4.0295 12.888 0.41253 27.839 7.52 38.602 9.0268 11.179 22.414 19.394 37.213 16.695 13.362-0.53038 25.61-11.328 31.775-22.54 6.7139-13.934 7.1853-29.748 0.25697-43.707-5.5237-11.364-17.648-21.717-30.492-23.311h-15.661z"/>
</svg>
Then we can use those SVG paths in our library to draw the text:
然后,我们可以使用库中的SVG路径绘制文本:
// Declaring variables
var optionsPath, bsPath;
// Options for text (SVG paths)
optionsPath = {
animation: 'path',
inkAmount: 2,
frames: 20,
frameAnimation: true,
color: 'white',
width: 1000,
height: 300
};
// Initializing
bsPath = new Brushstroke(optionsPath);
// Draw each letter of the text, with a delay among them
var paths = document.querySelectorAll('path');
var delay = 0;
for (var i = 0; i < paths.length; i++) {
bsPath.draw({path: paths[i], delay: delay});
delay += 0.5;
}
添加“再次运行”功能 ( Adding "Run Again" Functionality )
Finally, we'd like to provide an option to repeat the animation in our poster, so we can see it again and again :)
最后,我们想提供一个选项来重复海报中的动画,因此我们可以一次又一次地看到它:)
The idea is to draw the entire screen with a solid black
color (like erasing), and then run our poster animation again. Let's see the implementation:
这个想法是用纯black
绘制整个屏幕(如擦除),然后再次运行我们的海报动画。 让我们看一下实现:
// Declaring variables
var button = document.querySelector('button');
var animating = true;
var optionsErase, bsErase;
// Erase and run again
optionsErase = {
queue: true,
size: 300,
padding: 0,
overlap: 100,
inkAmount: 20,
frames: 100,
frameAnimation: true,
color: '#000',
width: width,
height: height,
end: function () {
// Clear all canvas and run animation
bsBackground.clear();
bsPath.clear();
bsErase.clear();
runAnimation();
}
};
bsErase = new Brushstroke(optionsErase);
// Run again button
button.addEventListener('click', function () {
if (!animating) {
toggleButton();
bsErase.draw();
}
});
function toggleButton() {
button.classList.toggle('hidden');
animating = !animating;
}
结论 ( Conclusion )
And that's all!
就这样!
With a bit of styling to get things in the right place, we should have a beautiful and creative poster using brushstroke animations :)
借助一些样式使事物正确放置,我们应该使用笔触动画制作出美观且富有创意的海报:)
As always, you can check the final demo here, and also get the full code and documentation on github. There are more exciting features waiting for you there!
与往常一样,您可以在此处查看最终演示 ,并在github上获取完整的代码和文档 。 还有更多激动人心的功能等着您!
We really hope you liked this tutorial and find it useful!
我们真的希望您喜欢本教程并发现它有用!
翻译自: https://scotch.io/tutorials/drawing-creative-brushstrokes-with-javascript
javascript绘制圆