opencv中礼帽和黑帽
Problem statement:
问题陈述:
There is a class of N students and the task is to find the top K marks-scorers. Write a program that will print the index of the toppers of the class which will be same as the index of the student in the input array (use 0-based indexing). First print the index of the students having highest marks then the students with second highest and so on. If there are more than one students having same marks then print their indices in ascending order.
有一班N名学生,任务是找到得分最高的K分。 编写一个程序,该程序将打印班级排行榜的索引,该索引将与输入数组中学生的索引相同(使用基于0的索引)。 首先打印得分最高的学生的索引,然后打印第二高的学生的索引,依此类推。 如果有多个具有相同分数的学生,则以升序打印索引。
Input Example:
输入示例:
Suppose k = 2 and the students having highest marks have indices 0 and 5 and students having second highest marks have indices 6 and 7 then output will be 0 5 6 7.
假设k = 2,并且分数最高的学生的索引为0和5,分数第二高的学生的索引为6和7,那么输出将为0 5 6 7。
K=2
N=10
Marks are:
97 84 82 89 84 97 95 95 84 86
Output:
0 5 6 7, so there are four toppers for K=2
97 & 95 is to be considered. There are four students who has got this. Their indices are 0 5 6 7 (For a particular marks if there is more than one student, their indices needs to printed in ascending order).
97和95将被考虑。 有四个学生已经得到了这个。 他们的索引是0 5 6 7(对于一个特定的标志,如果有一个以上的学生,他们的索引需要以升序打印)。
Solution:
解:
Data structure used:
使用的数据结构:
Set (ordered in decreasing fashion)
集合(以降序排列)
Map (ordered in decreasing fashion)
地图(以降序排列)
Algorithm:
算法:
Need to store the marks in sorted way descending order.
需要以降序存储标记。
The marks are key and we need to map student indices to the key value. While mapping indices needed to be mapped in ascending fashion.
分数是关键,我们需要将学生索引映射到关键值。 而映射索引需要以升序方式进行映射。
Print indices for K keys ( marks value already sorted in decreasing fashion, thus top K keys are top K marks).
K个键的打印索引(标记值已经按降序排序,因此,前K个键是前K个标记)。
Implementation with the data structures used:
用所使用的数据结构实现:
Declare records as a map.
将记录声明为地图。
map<int, vector<int>, greater <int>> records;
map<> = ordered map usually ordered in ascending fashion as per key value greater <int> is used to order in descending fashion.Here our key is integer type which maps to a vector of integer. Clearly the key is marks & which maps to a list of indices of students.
在这里,我们的键是整数类型,它映射到整数向量。 显然,关键是标记&,它映射到学生的索引列表。
Declare numbers as a set.
将数字声明为一组。
set<int, greater<int>> numbers;
set<> = ordered set usually ordered in ascending fashion as per element value greater <int> is used to order in descending fashion.Here the elements are the marks which are stored in sorted descending fashion.
这里的元素是标记,它们以降序存储。
After completion of the input taking, both records&numbers are filled with datas as per mentioned previously.
输入完成后,两个记录和编号都填充了前面提到的数据。
Let's consider the above input example:
让我们考虑上面的输入示例:
K=2
N=10
Marks are:
97 84 82 89 84 97 95 95 84 86
So after completion of input taking:
因此,在完成输入之后:
Records looks like:
记录如下:
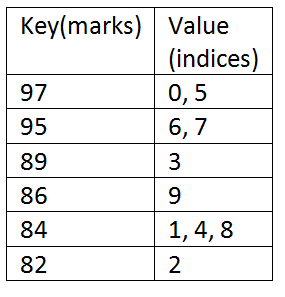
Numbers looks like:
数字看起来像:
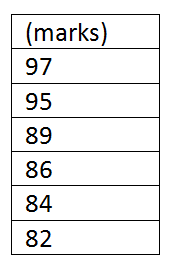
K=2, thus we need to print indices only for marks 97, 95
K = 2,因此我们只需要为标记97、95打印索引
Thus the indices to be print are: 0 5 6 7
因此要打印的索引为: 0 5 6 7
So to print:
所以要打印:
For i =0: K
Set iterator tonumbers.begin() //points to 97
Advance iterator by i; //to point at ith mark from the top
Print the vector list associated with the key (marks) pointed to.
END FOR
类的Toppers的C ++实现 (C++ implementation for Toppers of Class)
#include <bits/stdc++.h>
using namespace std;
int main()
{
int n,k;
//n is no of students, k is the input K
cout<<"enter no of student\n";
scanf("%d",&n);
map<int,vector<int>,greater <int>> records;//declare records
set<int,greater<int>> numbers;//declare numbers
int no;
cout<<"enter the marks of the students\n";
for(int i=0;i<n;i++){
cin>>no;
//for key value build the vector list
records[no].push_back(i);
//to avoid duplicate
if(numbers.find(no)==numbers.end())
numbers.insert(no); //insert marks to set
}
cout<<"enter K\n";
cin>>k; //input K
cout<<"Toppers are: ";
//printing the indices
for(int i=0;i<k;i++){
auto ij=numbers.begin();
advance(ij,i);
//printing the associated vector
for(auto it=records[*ij].begin();it!=records[*ij].end();it++){
printf("%d ",*it);
}
}
cout<<endl;
return 0;
}
Output
输出量
enter no of student
10
enter the marks of the students
97 84 82 89 84 97 95 95 84 86
enter K
2
Toppers are: 0 5 6 7
opencv中礼帽和黑帽