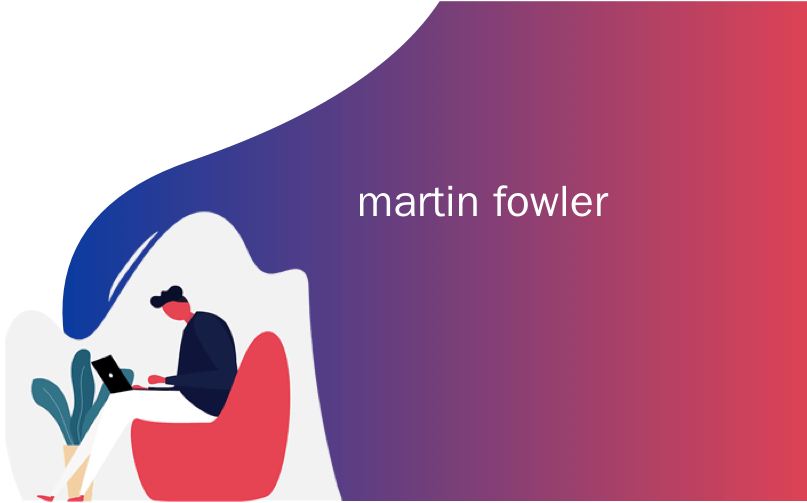
martin fowler
Open source ASP.NET Core 2.1 is out, and Architect David Fowler took to twitter to share some hidden gems that not everyone knows about. Sure, it's faster, builds faster, runs faster, but there's a number of details and fun advanced techniques that are worth a closer look at.
开源ASP.NET Core 2.1已经发布,架构师David Fowler到Twitter分享了一些并非所有人都知道的隐藏的宝石。 当然,它速度更快,构建速度更快,运行速度更快,但是还有许多细节和有趣的高级技术值得我们仔细研究。
.NET通用主机 (.NET Generic Host)
ASP.NET Core introduced a new hosting model. .NET apps configure and launch a host.
ASP.NET Core引入了新的托管模型。 .NET应用程序配置并启动主机。
The host is responsible for app startup and lifetime management. The goal of the Generic Host is to decouple the HTTP pipeline from the Web Host API to enable a wider array of host scenarios. Messaging, background tasks, and other non-HTTP workloads based on the Generic Host benefit from cross-cutting capabilities, such as configuration, dependency injection (DI), and logging.
主机负责应用程序启动和生命周期管理。 通用主机的目标是将HTTP管道与Web主机API分离开来,以实现更广泛的主机方案。 基于通用主机的消息传递,后台任务和其他非HTTP工作负载受益于诸如配置,依赖项注入(DI)和日志记录之类的跨领域功能。
This means that there's not just a WebHost anymore, there's a Generic Host for non-web-hosting scenarios. You get the same feeling as with ASP.NET Core and all the cool features like DI, logging, and config. The sample code for a Generic Host is up on GitHub.
这意味着不再有WebHost,还有用于非Web托管方案的通用主机。 您将获得与ASP.NET Core以及所有出色功能(如DI,日志记录和配置)相同的感觉。 通用主机的示例代码在GitHub上。
IHostedService (IHostedService)
A way to run long running background operations in both the generic host and in your web hosted applications. ASP.NET Core 2.1 added support for a BackgroundService base class that makes it trivial to write a long running async loop. The sample code for a Hosted Service is also up on GitHub.
一种在通用主机和Web托管应用程序中运行长时间运行的后台操作的方法。 ASP.NET Core 2.1添加了对BackgroundService基类的支持,这使得编写长时间运行的异步循环变得微不足道。 托管服务的示例代码也在GitHub上。
Check out a simple Timed Background Task:
查看一个简单的定时后台任务:
public Task StartAsync(CancellationToken cancellationToken)
{
_logger.LogInformation("Timed Background Service is starting.");
_timer = new Timer(DoWork, null, TimeSpan.Zero,
TimeSpan.FromSeconds(5));
return Task.CompletedTask;
}
Fun!
好玩!
.NET Core上的Windows服务 (Windows Services on .NET Core)
You can now host ASP.NET Core inside a Windows Service! Lots of people have been asking for this. Again, no need for IIS, and you can host whatever makes you happy. Check out Microsoft.AspNetCore.Hosting.WindowsServices on NuGet and extensive docs on how to host your own ASP.NET Core app without IIS on Windows as a Windows Service.
您现在可以在Windows服务中托管ASP.NET Core! 许多人一直在要求这个。 同样,不需要IIS,您可以托管任何让自己满意的东西。 在NuGet上查看Microsoft.AspNetCore.Hosting.WindowsServices ,以及有关如何在Windows上将IIS作为Windows Service托管没有ASP的ASP.NET Core应用程序的大量文档。
public static void Main(string[] args)
{
var pathToExe = Process.GetCurrentProcess().MainModule.FileName;
var pathToContentRoot = Path.GetDirectoryName(pathToExe);
var host = WebHost.CreateDefaultBuilder(args)
.UseContentRoot(pathToContentRoot)
.UseStartup<Startup>()
.Build();
host.RunAsService();
}
IHostingStartup-使用程序集属性配置IWebHostBuilder (IHostingStartup - Configure IWebHostBuilder with an Assembly Attribute)
Simple and clean with source on GitHub as always.
[assembly: HostingStartup(typeof(SampleStartups.StartupInjection))]
共享源包(Shared Source Packages)
This is an interesting one you should definitely take a moment and pay attention to. It's possible to build packages that are used as helpers to share source code. We internally call these "shared source packages." These are used all over ASP.NET Core for things that should be shared BUT shouldn't be public APIs. These get used but won't end up as actual dependencies of your resulting package.
这是一个有趣的事情,您一定要花点时间并注意。 可以构建用作帮助程序来共享源代码的软件包。 我们内部将这些称为“共享源程序包”。 这些都是在ASP.NET Core上用于应该共享的东西,但不应是公共API 。 这些会被使用,但不会最终成为您生成的程序包的实际依赖项。
They are consumed like this in a CSPROJ. Notice the PrivateAssets attribute.
它们在CSPROJ中是这样消耗的。 请注意PrivateAssets属性。
<PackageReference Include="Microsoft.Extensions.ClosedGenericMatcher.Sources" PrivateAssets="All" Version="" />
<PackageReference Include="Microsoft.Extensions.ObjectMethodExecutor.Sources" PrivateAssets="All" Version="" />
ObjectMethodExecutor(ObjectMethodExecutor)
If you ever need to invoke a method on a type via reflection and that method could be async, we have a helper that we use everywhere in the ASP.NET Core code base that is highly optimized and flexible called the ObjectMethodExecutor.
如果您需要通过反射在类型上调用某个方法,并且该方法可能是异步的,那么我们将在ASP.NET Core代码库中的每个地方都使用一个高度优化和灵活的助手,称为ObjectMethodExecutor 。
The team uses this code in MVC to invoke your controller methods. They use this code in SignalR to invoke your hub methods. It handles async and sync methods. It also handles custom awaitables and F# async workflows
该团队在MVC中使用此代码来调用您的控制器方法。 他们在SignalR中使用此代码来调用您的集线器方法。 它处理异步和同步方法。 它还处理定制的等待和F#异步工作流程
SuppressStatusMessages(SuppressStatusMessages)
A small and commonly requested one. If you hate the output that dotnet run gives when you host a web application (printing out the binding information) you can use the new SuppressStatusMessages extension method.
小型且通常要求的一个。 如果您讨厌托管网络应用程序(打印出绑定信息)时dotnet运行提供的输出,则可以使用新的SuppressStatusMessages扩展方法。
WebHost.CreateDefaultBuilder(args)
.SuppressStatusMessages(true)
.UseStartup<Startup>();
添加选项(AddOptions)
They made it easier in 2.1 to configure options that require services. Previously, you would have had to create a type that derived from IConfigureOptions<TOptions>, now you can do it all in ConfigureServices via AddOptions<TOptions>
在2.1中,它们使配置需要服务的选项变得更加容易。 以前,您必须创建一个从IConfigureOptions <TOptions>派生的类型,现在您可以通过AddOptions <TOptions>在ConfigureServices中完成所有操作
public void ConfigureServicdes(IServiceCollection services)
{
services.AddOptions<MyOptions>()
.Configure<IHostingEnvironment>((o,env) =>
{
o.Path = env.WebRootPath;
});
}
通过AddHttpContextAccessor的IHttpContext(IHttpContext via AddHttpContextAccessor)
You likely shouldn't be digging around for IHttpContext, but lots of folks ask how to get to it and some feel it should be automatic. It's not registered by default since having it has a performance cost. However, in ASP.NET Core 2.1 a PR was put in for an extension method that makes it easy IF you want it.
您可能不应该去研究IHttpContext,但是很多人都问如何使用它,并且有些人认为它应该是自动的。 由于具有性能成本,因此默认情况下未注册它。 但是,在ASP.NET Core 2.1中,为扩展方法添加了PR ,使您可以轻松地扩展它。
services.AddHttpContextAccessor();
So ASP.NET Core 2.1 is out and ready to go.
New features in this release include:
此版本中的新功能包括:
SignalR – Add real-time web capabilities to your ASP.NET Core apps.
SignalR –将实时Web功能添加到ASP.NET Core应用程序。
Razor class libraries – Use Razor to build views and pages into reusable class libraries.
Razor类库–使用Razor将视图和页面构建到可重用的类库中。
Identity UI library & scaffolding – Add identity to any app and customize it to meet your needs.
身份UI库和脚手架–将身份添加到任何应用程序并对其进行自定义以满足您的需求。
HTTPS – Enabled by default and easy to configure in production.
HTTPS –默认启用,易于在生产中进行配置。
Template additions to help meet some GDPR requirements – Give users control over their personal data and handle cookie consent.
添加模板以帮助满足某些GDPR要求–使用户可以控制其个人数据并处理Cookie同意。
MVC functional test infrastructure – Write functional tests for your app in-memory.
MVC功能测试基础架构–在内存中为您的应用编写功能测试。
[ApiController], ActionResult<T> – Build clean and descriptive web APIs.
[ApiController] , ActionResult <T> –构建干净且描述性的Web API。
IHttpClientFactory – HttpClient client as a service that you can centrally manage and configure.
IHttpClientFactory – HttpClient客户端即服务,您可以集中管理和配置。
Kestrel on Sockets – Managed sockets replace libuv as Kestrel's default transport.
套接字上的Kestrel –托管套接字将libuv替换为Kestrel的默认传输方式。
Generic host builder – Generic host infrastructure decoupled from HTTP with support for DI, configuration, and logging.
通用主机构建器–通用主机基础结构与HTTP分离,支持DI,配置和日志记录。
Updated SPA templates – Angular, React, and React + Redux templates have been updated to use the standard project structures and build systems for each framework (Angular CLI and create-react-app).
更新的SPA模板– Angular,React和React + Redux模板已更新,以使用标准项目结构并为每个框架构建系统(Angular CLI和create-react-app)。
Check out What's New in ASP.NET Core 2.1 in the ASP.NET Core docs to learn more about these features. For a complete list of all the changes in this release, see the release notes.
在ASP.NET Core文档中查看ASP.NET Core 2.1的新增功能,以了解有关这些功能的更多信息。 有关此发行版中所有更改的完整列表,请参见发行说明。
Go give it a try. Follow this QuickStart and you can have a basic Web App up in 10 minutes.
去试试看。 按照此快速入门,您可以在10分钟内建立一个基本的Web应用程序。
Sponsor: Check out JetBrains Rider: a cross-platform .NET IDE. Edit, refactor, test and debug ASP.NET, .NET Framework, .NET Core, Xamarin or Unity applications. Learn more and download a 30-day trial!
赞助商:查看JetBrains Rider:一个跨平台的.NET IDE 。 编辑,重构,测试和调试ASP.NET,.NET Framework,.NET Core,Xamarin或Unity应用程序。 了解更多信息并下载30天试用版!
翻译自: https://www.hanselman.com/blog/aspnet-core-architect-david-fowlers-hidden-gems-in-21
martin fowler