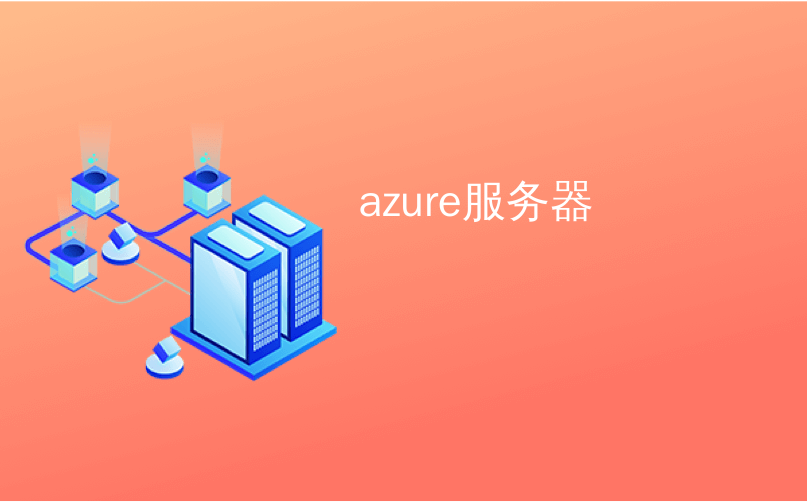
azure服务器
There's a lot of confusing terms in the Cloud space. And that's not counting the term "Cloud." ;)
云空间中有很多令人困惑的术语。 这还不包括“云”一词。 ;)
- IaaS (Infrastructure as a Services) - Virtual Machines and stuff on demand. IaaS(基础架构即服务)-虚拟机和随需应变的东西。
- PaaS (Platform as a Service) - You deploy your apps but try not to think about the Virtual Machines underneath. They exist, but we pretend they don't until forced. PaaS(平台即服务)-您部署了应用程序,但尽量不要考虑下面的虚拟机。 它们存在,但是我们假装它们直到被强迫才存在。
- SaaS (Software as a Service) - Stuff like Office 365 and Gmail. You pay a subscription and you get email/whatever as a service. It Just Works. SaaS(软件即服务)-诸如Office 365和Gmail之类的东西。 您支付订阅费用,就可以获得电子邮件/任何服务。 它只是工作。
"Serverless Computing" doesn't really mean there's no server. Serverless means there's no server you need to worry about. That might sound like PaaS, but it's higher level that than.
“无服务器计算”实际上并不意味着没有服务器。 无服务器意味着您无需担心服务器。 听起来可能像PaaS,但要比PaaS高。
Serverless Computing is like this - Your code, a slider bar, and your credit card. You just have your function out there and it will scale as long as you can pay for it. It's as close to "cloudy" as The Cloud can get.
无服务器计算就是这样-您的代码,一个滑块和您的信用卡。 您只需拥有自己的功能,并且只要您能支付费用,它就会扩展。 它可以像The Cloud一样接近“阴天”。
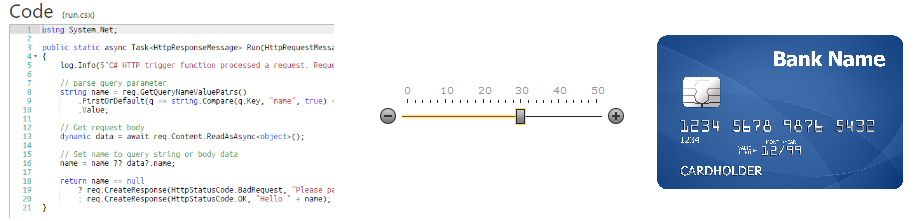
With Platform as a Service, you might make a Node or C# app, check it into Git, deploy it to a Web Site/Application, and then you've got an endpoint. You might scale it up (get more CPU/Memory/Disk) or out (have 1, 2, n instances of the Web App) but it's not seamless. It's totally cool, to be clear, but you're always aware of the servers.
使用平台即服务,您可以制作一个Node或C#应用程序,将其检入Git,将其部署到网站/应用程序,然后获得一个端点。 您可能会扩大规模(获取更多的CPU /内存/磁盘)或扩大规模(拥有1,2,2,n个Web应用程序实例),但这并不是无缝的。 显然,这非常酷,但是您始终知道服务器。
New cloud systems like Amazon Lambda and Azure Functions have you upload some code and it's running seconds later. You can have continuous jobs, functions that run on a triggered event, or make Web APIs or Webhooks that are just a function with a URL.
像Amazon Lambda和Azure Functions这样的新云系统可以让您上传一些代码,并且几秒钟后即可运行。 您可以具有连续的作业,在触发的事件上运行的功能,也可以使Web API或Webhooks只是具有URL的功能。
I'm going to see how quickly I can make a Web API with Serverless Computing.
我将看到我可以用无服务器计算制作Web API的速度。
I'll go to http://functions.azure.com and make a new function. If you don't have an account you can sign up free.
我将转到http://functions.azure.com并创建一个新功能。 如果您没有帐户,则可以免费注册。
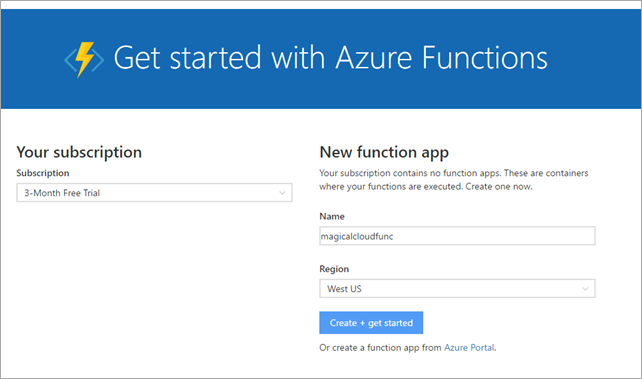
You can make a function in JavaScript or C#.
您可以使用JavaScript或C#编写函数。
Once you're into the Azure Function Editor, click "New Function" and you've got dozens of templates and code examples for things like:
进入Azure Function编辑器后,单击“ New Function”,您将获得许多模板和代码示例,例如:
- Find a face in an image and store the rectangle of where the face is. 在图像中找到一个人脸并存储该人脸所在位置的矩形。
- Run a function and comment on a GitHub issue when a GitHub webhook is triggered 触发GitHub Webhook时运行函数并评论GitHub问题
- Update a storage blob when an HTTP Request comes in 发出HTTP请求时更新存储Blob
- Load entities from a database or storage table 从数据库或存储表加载实体
I figured I'd change the first example. It is a trigger that sees an image in storage, calls a cognitive services API to get the location of the face, then stores the data. I wanted to change it to:
我想我会改变第一个例子。 它是一个触发器,可以看到存储中的图像,然后调用认知服务API来获取人脸的位置,然后存储数据。 我想将其更改为:
- Take an image as input from an HTTP Post 从HTTP Post获取图像作为输入
- Draw a rectangle around the face 围绕脸部绘制一个矩形
- Return the new image返回新图片
You can do this work from Git/GitHub but for easy stuff I'm literally doing it all in the browser. Here's what it looks like.
您可以从Git / GitHub上完成这项工作,但是对于简单的事情,我实际上是在浏览器中完成的。 这是它的样子。
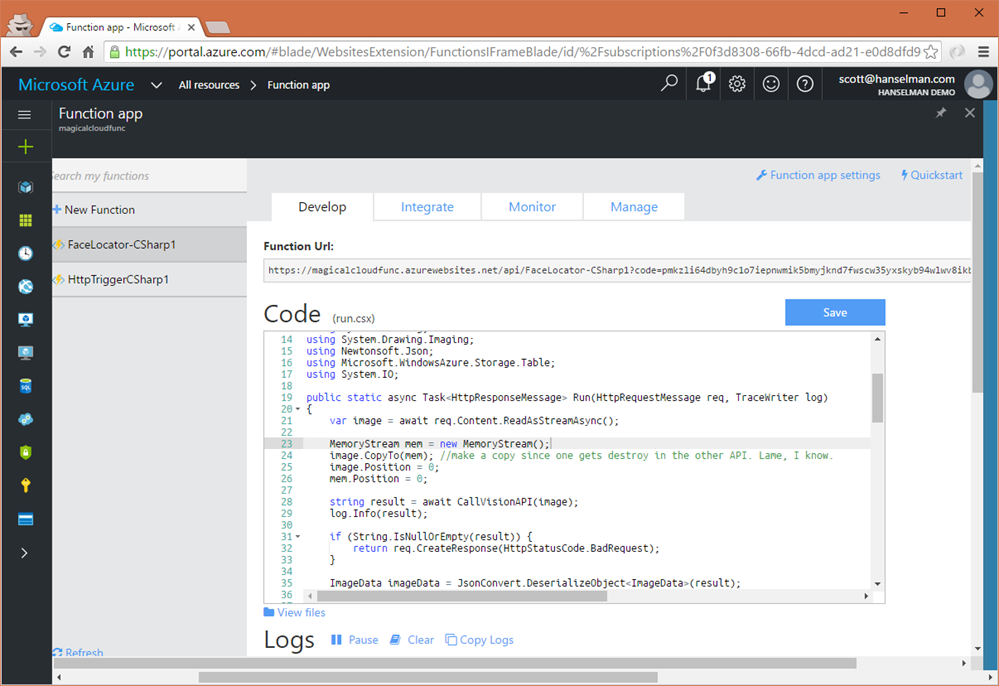
I code and iterate and save and fail fast, fail often. Here's the starter code I based it on. Remember, that this is a starter function that runs on a triggered event, so note its Run()...I'm going to change this.
我进行编码和迭代,然后保存并快速失败,经常失败。 这是我基于的入门代码。 请记住,这是一个在触发事件上运行的启动函数,因此请注意其Run()...我将对此进行更改。
#r "Microsoft.WindowsAzure.Storage"
#r "Newtonsoft.Json"
using System.Net;
using System.Net.Http;
using System.Net.Http.Headers;
using Newtonsoft.Json;
using Microsoft.WindowsAzure.Storage.Table;
using System.IO;
public static async Task Run(Stream image, string name, IAsyncCollector<FaceRectangle> outTable, TraceWriter log)
{
var image = await req.Content.ReadAsStreamAsync();
string result = await CallVisionAPI(image); //STREAM
log.Info(result);
if (String.IsNullOrEmpty(result))
{
return req.CreateResponse(HttpStatusCode.BadRequest);
}
ImageData imageData = JsonConvert.DeserializeObject<ImageData>(result);
foreach (Face face in imageData.Faces)
{
var faceRectangle = face.FaceRectangle;
faceRectangle.RowKey = Guid.NewGuid().ToString();
faceRectangle.PartitionKey = "Functions";
faceRectangle.ImageFile = name + ".jpg";
await outTable.AddAsync(faceRectangle);
}
return req.CreateResponse(HttpStatusCode.OK, "Nice Job");
}
static async Task<string> CallVisionAPI(Stream image)
{
using (var client = new HttpClient())
{
var content = new StreamContent(image);
var url = "https://api.projectoxford.ai/vision/v1.0/analyze?visualFeatures=Faces";
client.DefaultRequestHeaders.Add("Ocp-Apim-Subscription-Key", Environment.GetEnvironmentVariable("Vision_API_Subscription_Key"));
content.Headers.ContentType = new MediaTypeHeaderValue("application/octet-stream");
var httpResponse = await client.PostAsync(url, content);
if (httpResponse.StatusCode == HttpStatusCode.OK){
return await httpResponse.Content.ReadAsStringAsync();
}
}
return null;
}
public class ImageData {
public List<Face> Faces { get; set; }
}
public class Face {
public int Age { get; set; }
public string Gender { get; set; }
public FaceRectangle FaceRectangle { get; set; }
}
public class FaceRectangle : TableEntity {
public string ImageFile { get; set; }
public int Left { get; set; }
public int Top { get; set; }
public int Width { get; set; }
public int Height { get; set; }
}
GOAL: I'll change this Run() and make this listen for an HTTP request that contains an image, read the image that's POSTed in (ya, I know, no validation), draw rectangle around detected faces, then return a new image.
目标:我将更改此Run()并使其侦听包含图像的HTTP请求,读取张贴在其中的图像(是的,我知道,没有验证),在检测到的面Kong周围绘制矩形,然后返回一个新图像。
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log) {
var image = await req.Content.ReadAsStreamAsync();
As for the body of this function, I'm 20% sure I'm using too many MemoryStreams but they are getting disposed so take this code as a initial proof of concept. However, I DO need at least the two I have. Regardless, happy to chat with those who know more, but it's more subtle than even I thought. That said, basically call out to the API, get back some face data that looks like this:
至于这个函数的主体,我有20%的把握是我使用了太多的MemoryStreams,但是它们已经被废弃了,因此可以将此代码作为概念的初步证明。 但是,我确实至少需要两个。 无论如何,我们很乐意与认识更多的人聊天,但这比我想象的还要微妙。 就是说,基本上调用API,取回一些看起来像这样的面部数据:
2016-08-26T23:59:26.741 {"requestId":"8be222ff-98cc-4019-8038-c22eeffa63ed","metadata":{"width":2808,"height":1872,"format":"Jpeg"},"faces":[{"age":41,"gender":"Male","faceRectangle":{"left":1059,"top":671,"width":466,"height":466}},{"age":41,"gender":"Male","faceRectangle":{"left":1916,"top":702,"width":448,"height":448}}]}
Then take that data and DRAW a Rectangle over the faces detected.
然后获取该数据并在检测到的脸上绘制一个矩形。
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
var image = await req.Content.ReadAsStreamAsync();
MemoryStream mem = new MemoryStream();
image.CopyTo(mem); //make a copy since one gets destroy in the other API. Lame, I know.
image.Position = 0;
mem.Position = 0;
string result = await CallVisionAPI(image);
log.Info(result);
if (String.IsNullOrEmpty(result)) {
return req.CreateResponse(HttpStatusCode.BadRequest);
}
ImageData imageData = JsonConvert.DeserializeObject<ImageData>(result);
MemoryStream outputStream = new MemoryStream();
using(Image maybeFace = Image.FromStream(mem, true))
{
using (Graphics g = Graphics.FromImage(maybeFace))
{
Pen yellowPen = new Pen(Color.Yellow, 4);
foreach (Face face in imageData.Faces)
{
var faceRectangle = face.FaceRectangle;
g.DrawRectangle(yellowPen,
faceRectangle.Left, faceRectangle.Top,
faceRectangle.Width, faceRectangle.Height);
}
}
maybeFace.Save(outputStream, ImageFormat.Jpeg);
}
var response = new HttpResponseMessage()
{
Content = new ByteArrayContent(outputStream.ToArray()),
StatusCode = HttpStatusCode.OK,
};
response.Content.Headers.ContentType = new MediaTypeHeaderValue("image/jpeg");
return response;
}
I also added a reference to System. Drawing using this syntax at the top of the file and added a few namespaces with usings like System.Drawing and System.Drawing.Imaging. I also changed the input in the Integrate tab to "HTTP" as my input.
我还添加了对系统的引用。 使用此语法在文件顶部进行绘图,并使用诸如System.Drawing和System.Drawing.Imaging之类的名称添加了一些命名空间。 我还将“集成”选项卡中的输入更改为“ HTTP”作为输入。
#r "System.Drawing
Now I go into Postman and POST an image to my new Azure Function endpoint. Here I uploaded a flattering picture of me and unflattering picture of The Oatmeal. He's pretty in real life just NOT HERE. ;)
现在,我进入Postman并将图像发布到新的Azure Function终结点。 在这里,我上传了一张令我欣喜的照片和一幅《燕麦粥》的令人欣喜的照片。 他在现实生活中很漂亮,只是不在这里。 ;)
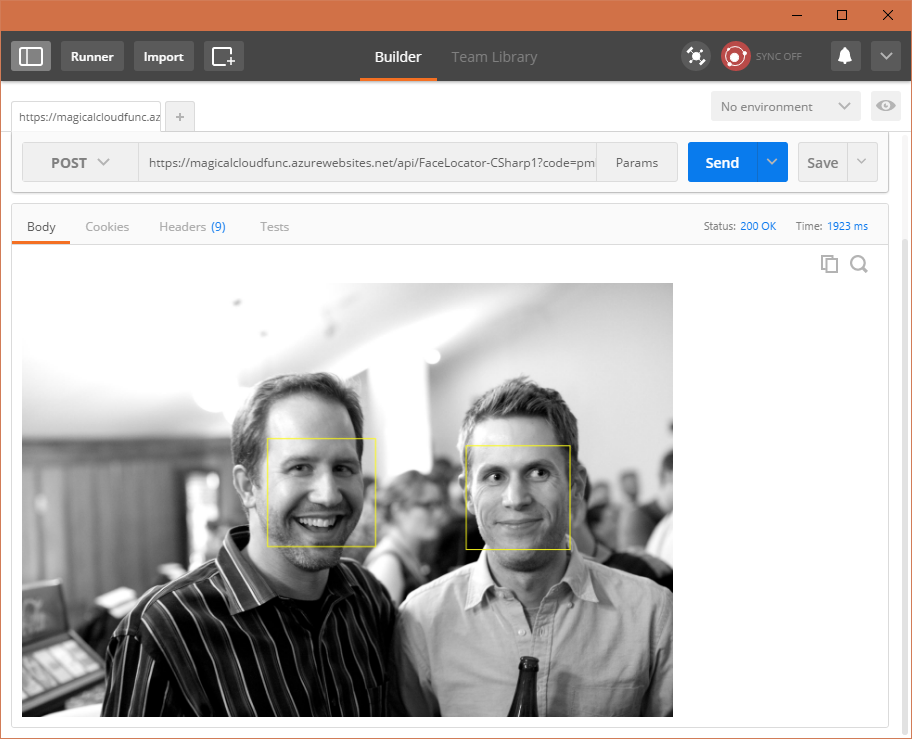
So in just about 15 min with no idea and armed with just my browser, Postman (also my browser), Google/StackOverflow, and Azure Functions I've got a backend proof of concept.
因此,在短短约15分钟内,我一无所知,仅使用我的浏览器,Postman(也是我的浏览器),Google / StackOverflow和Azure Functions,我就获得了概念的后端证明。
Azure Functions supports Node.js, C#, F#, Python, PHP *and* Batch, Bash, and PowerShell, which really opens it up to basically anyone. You can use them for anything when you just want a function (or more) out there on the web. Send stuff to Slack, automate your house, update GitHub issues, act as a Webhook, etc. There's some great 3d party Azure Functions sample code in this GitHub repo as well. Inputs can be from basically anywhere and outputs can be basically anywhere. If those anywheres are also cloud services like Tables or Storage, you've got a "serverless backed" that is easy to scale.
Azure Functions支持Node.js,C#,F#,Python,PHP *和* Batch,Bash和PowerShell,这实际上使它几乎可以向任何人开放。 当您只想在网络上使用某个功能(或更多功能)时,就可以将它们用于任何用途。 将内容发送到Slack,自动化您的房子,更新GitHub问题,充当Webhook,等等。此GitHub存储库中还有一些很棒的3d派对Azure Functions示例代码。 输入基本上可以来自任何地方,输出基本上可以位于任何地方。 如果这些地方也是Tables或Storage等云服务,那么您将获得易于扩展的“无服务器支持”。
I'm still learning, but I can see when I'd want a VM (total control) vs a Web App (near total control) vs a "Serverless" Azure Function (less control but I didn't need it anyway, just wanted a function in the cloud.)
我仍在学习,但是我可以看到何时需要VM(总控制权),Web App(近总控制权)和“无服务器” Azure Function(更少的控制权,但我还是不需要它)想要在云中发挥作用。)
Sponsor: Aspose makes programming APIs for working with files, like: DOC, XLS, PPT, PDF and countless more. Developers can use their products to create, convert, modify, or manage files in almost any way. Aspose is a good company and they offer solid products. Check them out, and download a free evaluation.
赞助者: Aspose制作用于处理文件的编程API,例如DOC,XLS,PPT,PDF等。 开发人员几乎可以使用其产品来创建,转换,修改或管理文件。 Aspose是一家优秀的公司,他们提供可靠的产品。 签出并下载免费评估版。
翻译自: https://www.hanselman.com/blog/what-is-serverless-computing-exploring-azure-functions
azure服务器