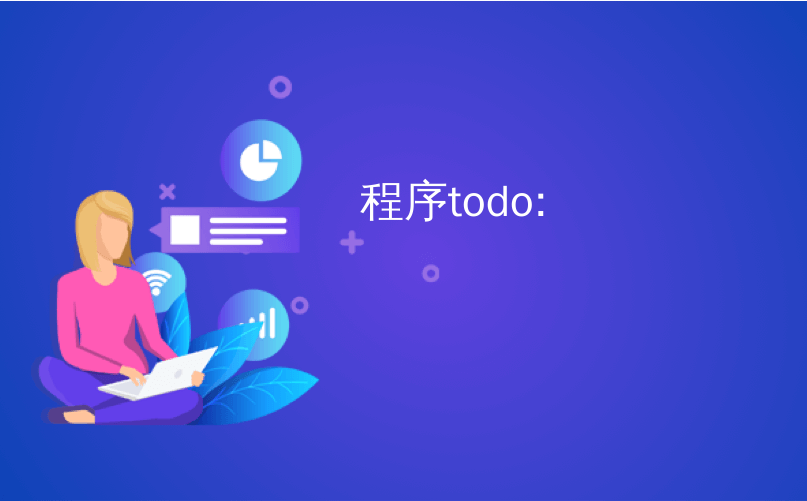
程序todo:
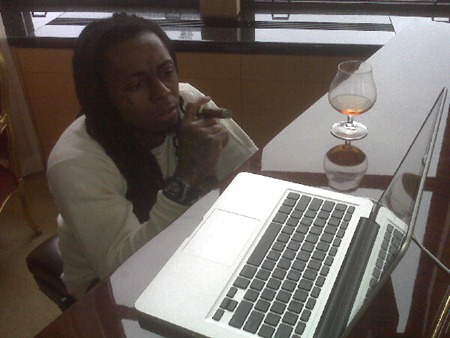
I've blogged before about ASP.NET Architect David Fowler's hidden gems in ASP.NET. His GitHub is worth following because he's always exploring and thinking and he's doing it in public. I love reading other people's source code.
我以前写过关于ASP.NET架构师David Fowler在ASP.NET中隐藏的宝石的博客。 他的GitHub值得关注,因为他一直在探索和思考,并且在公共场合进行。 我喜欢阅读别人的源代码。
He's been working on a local orchestrator called Micronetes that is worth reading about, but for this blog post I want to focus on his "Todos" repository.
他一直在研究一个名为Micronetes的本地协调器,值得一读,但是对于这篇博客文章,我想重点关注他的“ Todos ”存储库。
Making a Todo List is a form of Hello World on the web, similar to making a blog or a simple website. Everyone knows what a Todo app should look and act like, so you can just focus on your tools and not on the requirements. You may feel that a Todo app "isn't complex enough" or isn't a good example app to make. That's fine, but it is worth exploring and reading the different ways the same thing can be done.
制作待办事项列表是网络上Hello World的一种形式,类似于制作博客或简单网站。 每个人都知道Todo应用程序的外观和行为,因此您可以只关注工具而不是需求。 您可能会觉得Todo应用程序“不够复杂”或不是一个很好的示例应用程序。 很好,但是值得探索和阅读完成同一件事的不同方法。
David's repository https://github.com/davidfowl/Todos is of note because it's not ONE Todo App. As of the time of this writing it's 8 todo apps, each with a different reason to exist.
David的存储库https://github.com/davidfowl/Todos值得注意,因为它不是一个Todo应用程序。 在撰写本文时,它是8个待办事项应用程序,每个都有不同的原因。
What's a basic app look like? What if you add Auth? What if you add Dependency Injection? What about Controllers? You get the idea.
基本的应用程序是什么样的? 如果添加身份验证怎么办? 如果添加依赖注入怎么办? 那控制器呢? 你明白了。
Some languages and platforms (*ahem* enterprise) get a reputation for being too complex, too layered, too many projects. Others may get the opposite reputation - that's a toy, it'll never scale (in size, traffic, size of team, whatever).
某些语言和平台(* ahem *企业)因过于复杂,分层以及太多项目而享有盛誉。 其他人可能会获得相反的声誉-这是一个玩具,它永远不会扩展(在规模,流量,团队规模等方面)。
The point is that not everything is a hammer and not everything is a screw. You may think this is a cop out, but the answers is always "It depends." The more experience you get in software and the more mistakes you make and the more systems you put into production the more you'll realize that - wait for it - it depends. Disagree if you like, but one size doesn't fit all.
关键是并不是所有东西都是锤子,也不是螺丝钉。 您可能认为这是个解决方案,但答案始终是“取决于情况”。 您获得的软件经验越多,您犯的错误越多,投入生产的系统越多,您就会意识到-等待它-取决于它。 如果您愿意,则不同意,但一种尺寸并不适合所有尺寸。
关于David的Todo代码的一些很棒的东西 (Some cool stuff about David's Todo code)
All that said, there's some cool "before and afters" if you look at the code for earlier ideomatic C# and what newer APIs and language features allow. For example, if we assume some extensions and new APIs added for clarity, here's a POST
综上所述,如果您查看较早的意识形态C#的代码以及较新的API和语言功能所允许的内容,那么前后会有一些很棒的变化。 例如,如果为了清晰起见,我们假设添加了一些扩展和新的API,则此为POST
static async Task PostAsync(HttpContext context)
{
var todo = await context.Request.ReadJsonAsync<Todo>(_options);
using var db = new TodoDbContext();
await db.Todos.AddAsync(todo);
await db.SaveChangesAsync();
context.Response.StatusCode = StatusCodes.Status204NoContent;
}
and the GET
和GET
static async Task GetAllAsync(HttpContext context)
{
using var db = new TodoDbContext();
var todos = await db.Todos.ToListAsync();
await context.Response.WriteJsonAsync(todos, _options);
}
I personally do think that stuff like this is too complex. I hate that out parameter.
我个人确实认为东西,这样太复杂。 我讨厌那个参数。
static async Task GetAsync(HttpContext context)
{
if (!context.Request.RouteValues.TryGet("id", out long id))
{
context.Response.StatusCode = StatusCodes.Status400BadRequest;
return;
}
using var db = new TodoDbContext();
var todo = await db.Todos.FindAsync(id);
if (todo == null)
{
context.Response.StatusCode = StatusCodes.Status404NotFound;
return;
}
await context.Response.WriteJsonAsync(todo);
}
This is made-up code from me that doesn't work. It's even still a little too much.
这是我的虚构代码,不起作用。 甚至还太多了。
static async Task GetAsync(HttpContext context)
{
if (!RouteValues.Exist("id")) return Http.400;
using var db = new TodoDbContext();
var todo = await db.Todos.FindAsync(RouteValues["id"] as int);
if (todo == null) return Http.404
await Json(todo);
}
These are all useful exercises and are fun to explore. It also brings up some hard questions:
这些都是有用的练习,很有趣。 它还提出了一些难题:
- What is the difference between terse and clear versus obscure and inaccessible? 简洁明了与晦涩难懂之间有什么区别?
- How important is the Law of Demeter? 得墨meter耳定律有多重要?
- Are some problems better solved by language changes or by main library changes? 通过更改语言或更改主库可以更好地解决某些问题吗?
How many things should/can be put into extension methods?
扩展方法应该/可以放几件东西?
- And when those basic scenarios break down, are you dropped into a Func<T<T<T<T<T<T>>>>> hellscape? 当这些基本情况崩溃时,您是否陷入了Func <T <T <T <T <T <T <T >>>>>地狱?
Do you enjoy reading code like this as much as I do, Dear Reader? I think it's a great learning tool. I could do a whole day-long class facilitating conversation around this code https://github.com/davidfowl/Todos
亲爱的读者,您是否像我一样喜欢阅读这样的代码? 我认为这是一个很棒的学习工具。 我可以做一整天的课,以促进围绕此代码的对话https://github.com/davidfowl/Todos
Enjoy!
请享用!
翻译自: https://www.hanselman.com/blog/your-todo-application-is-too-complex-or-not-complex-enough
程序todo: