TagHelpers are a new feature of ASP.NET 5 (formerly and colloquially ASP.NET vNext) but it's taken me (and others) some time to fully digest them and what they mean.
TagHelpers是ASP.NET 5(以前和口语中的ASP.NET vNext)的新功能,但是我(和其他人)花了一些时间来完全理解它们及其含义。
Note that this, and all of ASP.NET 5 is a work in progress. TagHelpers can and will change. There is NO tooling support in Visual Studio for them, as they are changing day to day, so just be aware. That's why this post (and series is called Work in Progress.)
请注意,这以及所有ASP.NET 5都在开发中。 TagHelpers可以并且将会改变。 由于它们每天都在变化,因此Visual Studio中没有针对它们的工具支持,因此请注意。 这就是为什么这篇文章(和系列被称为正在进行的工作)。
Historically we've used HtmlHelpers within a Razor View, so when you wanted a Label or a TextBox you'd do this. This is from the ASP.NET 5 Starter Web example.
从历史上看,我们在Razor View中使用了HtmlHelpers,因此,当您需要Label或TextBox时,可以这样做。 这来自ASP.NET 5 Starter Web示例。
<li>@Html.ActionLink("Home", "Index", "Home")</li>
There you have some HTML, then we start some C# with @ and then switch out. It's inline C#, calling a function that will return HTML.
那里有一些HTML,然后我们以@开头一些C#,然后切换出来。 它是内联C#,调用将返回HTML的函数。
Here's the same thing, using a TagHelper.
使用TagHelper也是一样。
<li><a controller="Home" action="Index">Home</a></li>
The source for TagHelpers is (as with all ASP.NET source) up on GitHub, here. This is an anchor, A, so it'll be in AnchorTagHelper. The code is very simple, in fact, gets a collection of attributes and decides which to act upon.
TagHelpers的源代码(与所有ASP.NET源代码一样)位于GitHub上,此处。 这是一个锚点A,因此它将位于AnchorTagHelper中。 该代码非常简单,实际上,它获取属性的集合并决定要对之采取行动。
In this case, "controller" and "action" are not HTML5 attributes, but rather ones that ASP.NET is looking for.
在这种情况下,“控制器”和“动作”不是HTML5属性,而是ASP.NET寻找的属性。
Question for You - Would you rather have these attributes and ones like them (including your own) be prefixed? Perhaps asp:controller or asp-controller? That's an open issue you can comment on! You could do [HtmlAttributeName("asp:whatever")] on a property or [TagName("foo")] for a tag if you liked.
给您的问题-您是否愿意为这些属性以及像它们一样的属性(包括您自己的属性)添加前缀? 也许是asp:controller还是asp-controller? 您可以对此发表评论,这是一个开放性问题! 您可以对属性执行[HtmlAttributeName(“ asp:whatever”)]或对标签执行[TagName(“ foo”)]。
How do these attributes get mapped to a TagHelper? Well, an attribute name is mapped directly to a C# property and automatically injected. See how AnchorTagHelper has public properties Action and Controller?
这些属性如何映射到TagHelper? 好吧,属性名称直接映射到C#属性并自动注入。 看看AnchorTagHelper如何具有公共属性Action和Controller?
It's important to note that this isn't the second coming of WebForms controls, while the possible asp:foo syntax may look familiar (even though a prefix is optional.) This is more like syntactic sugar that gives you a compact way to express your intent. It doesn't give you any "control lifecycle" or anything like that.
重要的是要注意,这并不是WebForms控件的第二次出现,尽管可能的asp:foo语法看起来很熟悉(即使前缀是可选的。)这更像是语法糖,它为您提供了一种紧凑的方式来表达您的意图。 它不会给您任何“控制生命周期”或类似的东西。
Personally, I'd love to see them look different in the editor. For example, rather than
就个人而言,我希望看到它们在编辑器中看起来有所不同。 例如,而不是
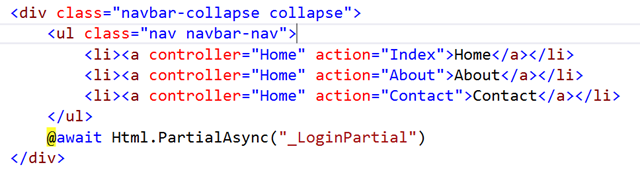
I'd like to see italics, or maybe a desaturation to show what's server-side and what's not, which will be super important if I'm NOT using a prefix to distinguish my attributes.
我想看到斜体字,或者是去饱和度以显示什么是服务器端的内容,什么不是,那么如果我不使用前缀来区分我的属性,这将非常重要。
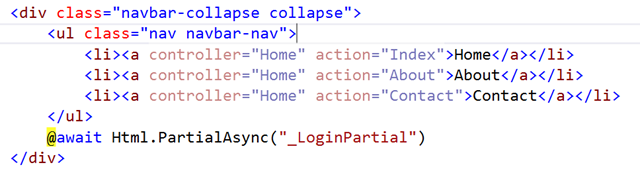
The code below in this Before and After results in the same HTML and the same behavior. A nice aspect of TagHelpers it that you avoid the context switch from markup to C#.
此之前和之后下面的代码将导致相同HTML和相同的行为。 TagHelpers的一个不错的方面是,您可以避免上下文从标记切换到C#。
Here is another example, a login partial form, before...
这是另一个示例,之前是登录部分表单。
@using System.Security.Principal
@if (User.Identity.IsAuthenticated)
{
using (Html.BeginForm("LogOff", "Account", FormMethod.Post, new { id = "logoutForm", @class = "navbar-right" }))
{
@Html.AntiForgeryToken()
<ul class="nav navbar-nav navbar-right">
<li>
@Html.ActionLink("Hello " + User.Identity.GetUserName() + "!", "Manage", "Account", routeValues: null, htmlAttributes: new { title = "Manage" })
</li>
<li><a href="javascript:document.getElementById('logoutForm').submit()">Log off</a></li>
</ul>
}
}
else
{
<ul class="nav navbar-nav navbar-right">
<li>@Html.ActionLink("Register", "Register", "Account", routeValues: null, htmlAttributes: new { id = "registerLink" })</li>
<li>@Html.ActionLink("Log in", "Login", "Account", routeValues: null, htmlAttributes: new { id = "loginLink" })</li>
</ul>
}
and after...with the Microsoft.AspNet.Mvc.TagHelpers package added in project.json and then @addtaghelper "MyAssemblyName" in either your ViewStart.cshtml to get this in all views, or separately within a single view page.
然后...在project.json中添加Microsoft.AspNet.Mvc.TagHelpers程序包,然后在ViewStart.cshtml中添加@addtaghelper“ MyAssemblyName”,以在所有视图中或单独在单个视图页面中获取它。
@using System.Security.Principal
@if (User.Identity.IsAuthenticated)
{
<form method="post" controller="Account" action="LogOff" id="logoutForm" class="navbar-right">
<ul class="nav navbar-nav navbar-right">
<li>
<a controller="Account" action="Manage" title="Manage">Hello @User.Identity.GetUserName()!</a>
</li>
<li><a href="javascript:document.getElementById('logoutForm').submit()">Log off</a></li>
</ul>
</form>
}
else
{
<ul class="nav navbar-nav navbar-right">
<li><a id="registerLink" controller="Account" action="Register">Register</a></li>
<li><a id="loginLink" controller="Account" action="Login">Log in</a></li>
</ul>
}
This makes for much cleaner markup-focused Views. Note that this Sample is a spike that Damian Edwards has on his GitHub, but you have TagHelpers in the Beta 1 build included with Visual Studio 2015 preview or OmniSharp. Get involved!
这样可以使标记视图更加清晰。 请注意,此示例是Damian Edwards在其GitHub上的峰值,但您的Beta 1版本中的TagHelpers包含在Visual Studio 2015预览版或OmniSharp中。 参与其中!
Remember also to check out http://www.asp.net/vnext and subscribe to my YouTube Channel and this playlist of the ASP.NET Weekly Community Standup. In this episode we talked about TagHelpers in depth!
还要记住,请访问http://www.asp.net/vnext并订阅我的YouTube频道和ASP.NET每周社区脱口秀的播放列表。 在这一集中,我们深入讨论了TagHelpers !
相关文章 (Related Posts)
翻译自: https://www.hanselman.com/blog/aspnet-5-vnext-work-in-progress-exploring-taghelpers