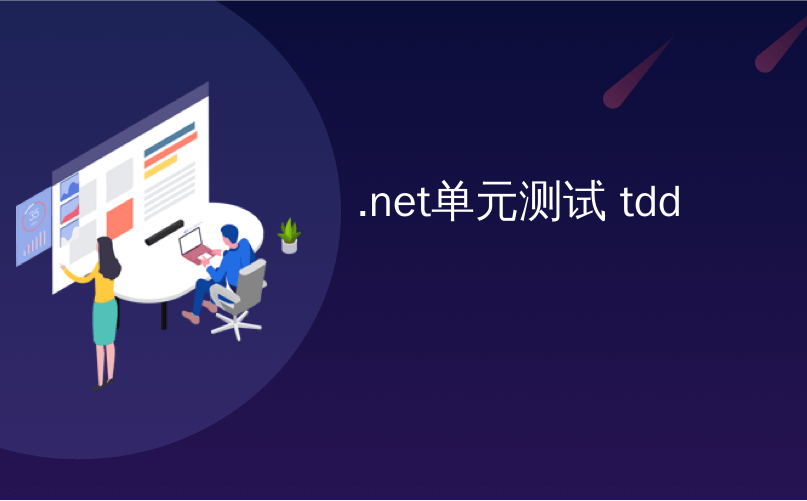
.net单元测试 tdd
NOTE: This post is based on an older preview version of ASP.NET MVC and details have very likely CHANGED. Go to http://www.asp.net/mvc or http://www.asp.net/forums for updated details and the latest version of ASP.NET MVC.
注意:这篇文章基于ASP.NET MVC的较早的预览版本,并且很可能已更改了详细信息。 请访问http://www.asp.net/mvc或http://www.asp.net/forums,以获取更新的详细信息和最新版本的ASP.NET MVC。
All the sessions from Mix are up on http://sessions.visitmix.com/ for your viewing pleasure. I had a total blast giving the ASP.NET MVC Talk. The energy was really good and the crowd (of around 600, I hear) was really cool.
您可以在http://sessions.visitmix.com/上打开Mix的所有会话,以获取观看乐趣。 我全力以赴 ASP.NET MVC对话。 能量真的很好,我的人群(大约600人)真的很酷。
You can download the MVC talk in these formats:
您可以通过以下格式下载MVC演讲:
ASP.NET MVC Preview 2 - PowerPoint (PPTX)
ASP.NET MVC预览版2- PowerPoint (PPTX)
I think the sound is a little quiet, so I had to turn it up some. It's better turned up a bit so you can hear the interaction with the crowd.
我认为声音有点安静,所以我不得不将其调高一些。 最好打开一点,以便您可以听到与人群的互动。
Here's some of the code from the talk you might be interested in. I'll post the rest very soon.
这是您可能感兴趣的演讲中的一些代码。我将很快发布其余的代码。
MvcMockHelpers (MvcMockHelpers)
The first are the MVCMockHelpers used in the Test Driven Development part of the talk, and also in the 4th ASP.NET MVC Testing Video up at www.asp.net/mvc.
首先是MVCMockHelpers,它们在本次演讲的“测试驱动开发”部分中使用,也在www.asp.net/mvc上的第4期ASP.NET MVC测试视频中使用。
NOTE AND DISCLAIMER: This is just a little chunks of helper methods, and I happened to use Rhino Mocks, an Open Source Mocking Framework, the talk at Mix. At my last company I introduced TypeMock and we bought it and lately I've been digging on Moq also. I'm not qualified yet to have a dogmatic opinion about which one is better, because they all do similar things. Use the one that makes you happy. I hope to see folks (that's YOU Dear Reader) repost and rewrite these helpers (and better, more complete ones) using all the different mocking tools. Don't consider this to be any kind of "stamp of approval" for one mocking framework over another. Cool?
注意和免责声明:这只是帮助方法的一小部分,而我碰巧使用了Rhino Mocks (一个开源的模拟框架),在Mix上进行了演讲。 在我的上一家公司,我介绍了TypeMock ,我们买了它,最近我也一直在研究Moq 。 我还没有资格对哪个更好的观点有教条式的看法,因为他们都做类似的事情。 使用使您快乐的那一种。 我希望看到人们(即YOU Dear Reader)使用所有不同的模拟工具重新发布并重写这些帮助程序(以及更好,更完善的帮助程序)。 不要认为这是一个嘲笑框架相对于另一个嘲笑框架的“认可印章”。 凉?
Anyway, here's the mocking stuff I used in the demo. This is similar to the stuff PhilHa did last year but slightly more complete. Still, this is just the beginning. We'll be hopefully releasing ASP.NET MVC bits on CodePlex maybe monthly. The goal is to release early and often. Eilon and the team have a lot more planned around testing, so remember, this is Preview 2 not Preview 18.
无论如何,这是我在演示中使用的模拟内容。 这类似于PhilHa去年所做的事情,但更加完整。 尽管如此,这仅仅是开始。 我们希望可以每月在CodePlex上发布ASP.NET MVC位。 目标是尽早发布并经常发布。 Eilon和团队在测试方面还有很多计划,因此请记住,这是Preview 2而不是Preview 18。
MvcMockHelpers-RhinoMocks (MvcMockHelpers - RhinoMocks)
using System;
using System.Web;
using Rhino.Mocks;
using System.Text.RegularExpressions;
using System.IO;
using System.Collections.Specialized;
using System.Web.Mvc;
using System.Web.Routing;
namespace UnitTests
{
public static class MvcMockHelpers
{
public static HttpContextBase FakeHttpContext(this MockRepository mocks)
{
HttpContextBase context = mocks.PartialMock<httpcontextbase>();
HttpRequestBase request = mocks.PartialMock<httprequestbase>();
HttpResponseBase response = mocks.PartialMock<httpresponsebase>();
HttpSessionStateBase session = mocks.PartialMock<httpsessionstatebase>();
HttpServerUtilityBase server = mocks.PartialMock<httpserverutilitybase>();
SetupResult.For(context.Request).Return(request);
SetupResult.For(context.Response).Return(response);
SetupResult.For(context.Session).Return(session);
SetupResult.For(context.Server).Return(server);
mocks.Replay(context);
return context;
}
public static HttpContextBase FakeHttpContext(this MockRepository mocks, string url)
{
HttpContextBase context = FakeHttpContext(mocks);
context.Request.SetupRequestUrl(url);
return context;
}
public static void SetFakeControllerContext(this MockRepository mocks, Controller controller)
{
var httpContext = mocks.FakeHttpContext();
ControllerContext context = new ControllerContext(new RequestContext(httpContext, new RouteData()), controller);
controller.ControllerContext = context;
}
static string GetUrlFileName(string url)
{
if (url.Contains("?"))
return url.Substring(0, url.IndexOf("?"));
else
return url;
}
static NameValueCollection GetQueryStringParameters(string url)
{
if (url.Contains("?"))
{
NameValueCollection parameters = new NameValueCollection();
string[] parts = url.Split("?".ToCharArray());
string[] keys = parts[1].Split("&".ToCharArray());
foreach (string key in keys)
{
string[] part = key.Split("=".ToCharArray());
parameters.Add(part[0], part[1]);
}
return parameters;
}
else
{
return null;
}
}
public static void SetHttpMethodResult(this HttpRequestBase request, string httpMethod)
{
SetupResult.For(request.HttpMethod).Return(httpMethod);
}
public static void SetupRequestUrl(this HttpRequestBase request, string url)
{
if (url == null)
throw new ArgumentNullException("url");
if (!url.StartsWith("~/"))
throw new ArgumentException("Sorry, we expect a virtual url starting with \"~/\".");
SetupResult.For(request.QueryString).Return(GetQueryStringParameters(url));
SetupResult.For(request.AppRelativeCurrentExecutionFilePath).Return(GetUrlFileName(url));
SetupResult.For(request.PathInfo).Return(string.Empty);
}
}
}
MvcMockHelpers-最小起订量 (MvcMockHelpers - Moq )
Here's the same thing in Moq. Muchas gracias, Kzu.
Moq中也是一样。 Muzus gracias, Kzu 。
using System;
using System.Web;
using System.Text.RegularExpressions;
using System.IO;
using System.Collections.Specialized;
using System.Web.Mvc;
using System.Web.Routing;
using Moq;
namespace UnitTests
{
public static class MvcMockHelpers
{
public static HttpContextBase FakeHttpContext()
{
var context = new Mock<httpcontextbase>();
var request = new Mock<httprequestbase>();
var response = new Mock<httpresponsebase>();
var session = new Mock<httpsessionstatebase>();
var server = new Mock<httpserverutilitybase>();
context.Expect(ctx => ctx.Request).Returns(request.Object);
context.Expect(ctx => ctx.Response).Returns(response.Object);
context.Expect(ctx => ctx.Session).Returns(session.Object);
context.Expect(ctx => ctx.Server).Returns(server.Object);
return context.Object;
}
public static HttpContextBase FakeHttpContext(string url)
{
HttpContextBase context = FakeHttpContext();
context.Request.SetupRequestUrl(url);
return context;
}
public static void SetFakeControllerContext(this Controller controller)
{
var httpContext = FakeHttpContext();
ControllerContext context = new ControllerContext(new RequestContext(httpContext, new RouteData()), controller);
controller.ControllerContext = context;
}
static string GetUrlFileName(string url)
{
if (url.Contains("?"))
return url.Substring(0, url.IndexOf("?"));
else
return url;
}
static NameValueCollection GetQueryStringParameters(string url)
{
if (url.Contains("?"))
{
NameValueCollection parameters = new NameValueCollection();
string[] parts = url.Split("?".ToCharArray());
string[] keys = parts[1].Split("&".ToCharArray());
foreach (string key in keys)
{
string[] part = key.Split("=".ToCharArray());
parameters.Add(part[0], part[1]);
}
return parameters;
}
else
{
return null;
}
}
public static void SetHttpMethodResult(this HttpRequestBase request, string httpMethod)
{
Mock.Get(request)
.Expect(req => req.HttpMethod)
.Returns(httpMethod);
}
public static void SetupRequestUrl(this HttpRequestBase request, string url)
{
if (url == null)
throw new ArgumentNullException("url");
if (!url.StartsWith("~/"))
throw new ArgumentException("Sorry, we expect a virtual url starting with \"~/\".");
var mock = Mock.Get(request);
mock.Expect(req => req.QueryString)
.Returns(GetQueryStringParameters(url));
mock.Expect(req => req.AppRelativeCurrentExecutionFilePath)
.Returns(GetUrlFileName(url));
mock.Expect(req => req.PathInfo)
.Returns(string.Empty);
}
}
}
Maybe
RoyO will do the same thing in TypeMock
in the next few hours and I'll copy/paste it here.
;)
也许
RoyO将
在接下来的几个小时
内在TypeMock
中
执行相同的操作
,我将在此处复制/粘贴它。
;)
MvcMockHelpers-TypeMock (MvcMockHelpers - TypeMock)
Thanks to Roy at TypeMock.
感谢TypeMock的Roy 。
using System;
using System.Collections.Specialized;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
using TypeMock;
namespace Typemock.Mvc
{
static class MvcMockHelpers
{
public static void SetFakeContextOn(Controller controller)
{
HttpContextBase context = MvcMockHelpers.FakeHttpContext();
controller.ControllerContext = new ControllerContext(new RequestContext(context, new RouteData()), controller);
}
public static void SetHttpMethodResult(this HttpRequestBase request, string httpMethod)
{
using (var r = new RecordExpectations())
{
r.ExpectAndReturn(request.HttpMethod, httpMethod);
}
}
public static void SetupRequestUrl(this HttpRequestBase request, string url)
{
if (url == null)
throw new ArgumentNullException("url");
if (!url.StartsWith("~/"))
throw new ArgumentException("Sorry, we expect a virtual url starting with \"~/\".");
var parameters = GetQueryStringParameters(url);
var fileName = GetUrlFileName(url);
using (var r = new RecordExpectations())
{
r.ExpectAndReturn(request.QueryString, parameters);
r.ExpectAndReturn(request.AppRelativeCurrentExecutionFilePath, fileName);
r.ExpectAndReturn(request.PathInfo, string.Empty);
}
}
static string GetUrlFileName(string url)
{
if (url.Contains("?"))
return url.Substring(0, url.IndexOf("?"));
else
return url;
}
static NameValueCollection GetQueryStringParameters(string url)
{
if (url.Contains("?"))
{
NameValueCollection parameters = new NameValueCollection();
string[] parts = url.Split("?".ToCharArray());
string[] keys = parts[1].Split("&".ToCharArray());
foreach (string key in keys)
{
string[] part = key.Split("=".ToCharArray());
parameters.Add(part[0], part[1]);
}
return parameters;
}
else
{
return null;
}
}
public static HttpContextBase FakeHttpContext(string url)
{
HttpContextBase context = FakeHttpContext();
context.Request.SetupRequestUrl(url);
return context;
}
public static HttpContextBase FakeHttpContext()
{
HttpContextBase context = MockManager.MockObject<HttpContextBase>().Object;
HttpRequestBase request = MockManager.MockObject<HttpRequestBase>().Object;
HttpResponseBase response = MockManager.MockObject<HttpResponseBase>().Object;
HttpSessionStateBase sessionState = MockManager.MockObject<HttpSessionStateBase>().Object;
HttpServerUtilityBase serverUtility = MockManager.MockObject<HttpServerUtilityBase>().Object;
using (var r = new RecordExpectations())
{
r.DefaultBehavior.RepeatAlways();
r.ExpectAndReturn(context.Response, response);
r.ExpectAndReturn(context.Request, request);
r.ExpectAndReturn(context.Session, sessionState);
r.ExpectAndReturn(context.Server, serverUtility);
}
return context;
}
}
}
翻译自: https://www.hanselman.com/blog/aspnet-mvc-session-at-mix08-tdd-and-mvcmockhelpers
.net单元测试 tdd