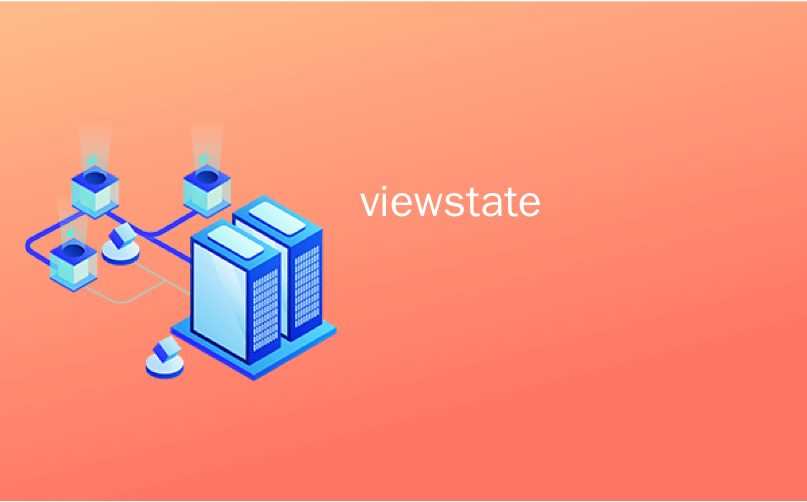
viewstate
Here's an interesting, odd, but obvious idea. If you're not able to use HttpCompression like the Blowery HttpCompression module that we use with dasBlog due to the pile of bugs in older versions of IE around compression, you can "zip" up the ViewState on fat (usually DataGrid related bloat).
这是一个有趣,奇怪但显而易见的想法。 如果由于旧版IE中围绕压缩的大量错误而无法像dasBlog那样使用像Blowery HttpCompression模块那样的HttpCompression,则可以在压缩状态下“压缩” ViewState(通常是与DataGrid相关的膨胀)。
This is some VB code that Vlad Olifier did, that he said I could post on my blog. It's also a new submission at GotDotnet. To use it, you just derive your ASP.NET page class from it.
这是Vlad Olifier所做的一些VB代码,他说我可以在我的博客上发布。 这也是GotDotnet的新作品。 要使用它,您只需从中派生ASP.NET页面类。
Using Zipped ViewState:
使用压缩的ViewState:
Public Class CompressPage
Inherits PageViewStateZip
Just deriving from System.Web.UI.Page as usual:
照常从System.Web.UI.Page派生:
Public Class RegularPage
Inherits System.Web.UI.Page
The "trick" is pretty simple. There are little-known virtuals in Page that you can override - specifically LoadPageStateFromPersistanceMedium (where that persistance medium is a hidden input box) and SavePageStateToPersistenceMedium.
“技巧”非常简单。 您可以覆盖Page中鲜为人知的虚拟机-特别是LoadPageStateFromPersistanceMedium(其中,持久性介质是隐藏的输入框)和SavePageStateToPersistenceMedium。
When it's time to load view state, we pull it out of the form and un-base64 the string into a byte array, un-zip the bytes, then deserialize the ViewState. When it's time to save, reverse the process - Serialize, zip, store. There's some overhead, certainly. The amount of compression is usually about 50%, but your mileage may vary (YMMV).
当需要加载视图状态时,我们将其从表单中拉出并将字符串取消base64放入字节数组,解压缩字节,然后反序列化ViewState。 需要保存时,请逆转此过程-序列化,压缩,存储。 当然会有一些开销。 压缩量通常约为50%,但行驶里程可能有所不同(YMMV)。
The real decision flow is this:
真正的决策流程是这样的:
- Can you use HttpCompression (via XCompress or an HttpModule)? 可以使用HttpCompression(通过XCompress或HttpModule)吗?
- If so, use it. 如果是这样,请使用它。
- Can't? (Bugs, SSL, Compatibility, bosses, don't want to take the perf hit, etc) 不行吗(错误,SSL,兼容性,老板,不想受到打击等)
- Got Fat ViewState on a few pages? 在几页上有Fat ViewState?
- Use Zipped ViewState on a few pages as needed. 根据需要在几页上使用Zipped ViewState。
Imports System.IO
Imports Zip = ICSharpCode.SharpZipLib.Zip.Compression
Public Class PageViewStateZip : Inherits System.Web.UI.Page
Protected Overrides Function LoadPageStateFromPersistenceMedium() As Object
Dim vState As String = Me.Request.Form("__VSTATE")
Dim bytes As Byte() = System.Convert.FromBase64String(vState)
bytes = vioZip.Decompress(bytes)
Dim format As New LosFormatter
Return format.Deserialize(System.Convert.ToBase64String(bytes))
End Function
Protected Overrides Sub SavePageStateToPersistenceMedium(ByVal viewState As Object)
Dim format As New LosFormatter
Dim writer As New StringWriter
format.Serialize(writer, viewState)
Dim viewStateStr As String = writer.ToString()
Dim bytes As Byte() = System.Convert.FromBase64String(viewStateStr)
bytes = vioZip.Compress(bytes)
Dim vStateStr As String = System.Convert.ToBase64String(bytes)
RegisterHiddenField("__VSTATE", vStateStr)
End Sub
End Class
Note that this sample uses SharpZipLib from ICSharpCode, but I assume you could use others, and I'd probably use System.IO.Compression if I was using .NET 2.0. The buffer sizes are hard-coded, but the only one that really matters it the one in Compress(). Again, salt to taste.
请注意,此示例使用ICSharpCode的SharpZipLib,但我假设您可以使用其他示例,如果使用的是.NET 2.0,则可能会使用System.IO.Compression。 缓冲区的大小是硬编码的,但实际上最重要的是Compress()中的一个。 同样,加盐调味。
Imports System.IO
Imports Zip = ICSharpCode.SharpZipLib.Zip.Compression
'//--Download ICSharpCode.SharpZipLib from
'//--http://www.icsharpcode.net/OpenSource/SharpZipLib/Download.aspx
Public Class vioZip
Shared Function Compress(ByVal bytes() As Byte) As Byte()
Dim memory As New MemoryStream
Dim stream = New Zip.Streams.DeflaterOutputStream(memory, _
New Zip.Deflater(Zip.Deflater.BEST_COMPRESSION), 131072)
stream.Write(bytes, 0, bytes.Length)
stream.Close()
Return memory.ToArray()
End Function
Shared Function Decompress(ByVal bytes() As Byte) As Byte()
Dim stream = New Zip.Streams.InflaterInputStream(New MemoryStream(bytes))
Dim memory As New MemoryStream
Dim writeData(4096) As Byte
Dim size As Integer
While True
size = stream.Read(writeData, 0, writeData.Length)
If size > 0 Then memory.Write(writeData, 0, size) Else Exit While
End While
stream.Close()
Return memory.ToArray()
End Function
End Class
翻译自: https://www.hanselman.com/blog/zippingcompressing-viewstate-in-aspnet
viewstate