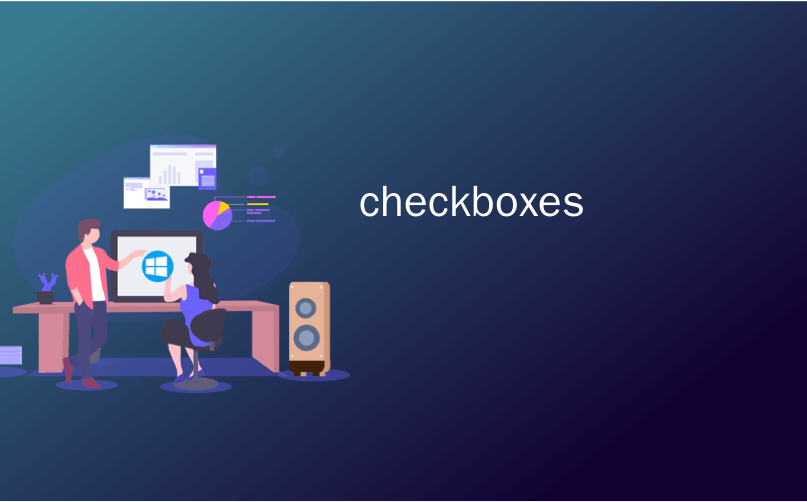
checkboxes
Recently I needed to have a DataGrid that had multiple checkboxes to activate and deactivate something. So, you could check a whole slew of checkboxes in a big grid, and depending on the NEW state of the checkbox (checked or not), cause an operation on a theorectical back end.
最近,我需要一个具有多个复选框的DataGrid来激活和停用某些功能。 因此,您可以在一个较大的网格中检查整个复选框,然后根据复选框的新状态(是否选中)在理论后端进行操作。
Here's some opinion/thought/zen on the DataGrid, and DataGridGirl will have to tell you if I'm wrong or not.
这是对DataGrid的一些看法/想法/禅定, DataGridGirl将不得不告诉您我是否错了。
Work WITH the Grid, not against it: If you find yourself filling up the Grid via DataBinding, THEN running around in the rendered grid with foreach this and foreach that looking for HTML controls, you probably want to rethink things. Look to the Events, my son.
使用网格,而不是不使用网格:如果您发现自己通过DataBinding填充了网格,然后使用foreach this和foreach that寻找HTML控件在渲染的网格中四处运行,您可能想重新考虑一下。 看看事件,我的儿子。
Listen to the Events and Nudge/Influence the Grid: Between OnItemCreated and OnItemDataBound, you've got some serious influence on the grid. If you can't get things to happen declaratively in the ItemTemplate, get them to happen Programmatically in these events.
听事件和微调/影响网格:在OnItemCreated和OnItemDataBound之间,您对网格有一些严重的影响。 如果您无法使事情在ItemTemplate中以声明方式发生,请使它们在这些事件中以编程方式发生。
Avoid Hidden Columns with IDs of things: In my case, I needed to hear when a checkbox's state changed, and at that time, I needed to know the ProductID associated with that checkbox. Rather than wadnering around in the Control Tree (ala DataItem[x].Parent.Parent.Parent, etc.FindControl("yousuck"), just bring the data along for the ride. See my solution below.
避免使用具有事物ID的隐藏列:就我而言,我需要听听复选框状态更改的时间,那时,我需要知道与该复选框关联的ProductID。 与其在控制树中徘徊(例如,DataItem [x] .Parent.Parent.Parent等。FindControl(“ yousuck”)),不如将数据随身携带。请参阅下面的解决方案。
Just because code is on CodeProject or in Google Groups doesn't mean the writer knows the DataGrid from his Ass: <rant>If I see one more solution in CodeProject or Google where someone says, "ya, just (DataTime)(((TextBox)Whatever.Item.Parent.Parent.Child[0].Parent.BrotherInLaw).Text).IntValue.ParseExact('yyyy/mm/dd')) and you're there" I will seriously hurt someone. The DataGrid has it's faults, sure, but it's a lot more subtle that .DataBind and brute force it. This some problem has happened with the XML DOM, and it's all the fault of QuickWatch. "Gosh, I can SEE the data, so it must be OK for me to spelunk for it." </rant>
仅仅因为代码在CodeProject或Google Groups上并不意味着作者从他的屁股知道DataGrid: <rant>如果我在CodeProject或Google中看到另一个解决方案,有人说: “是的,(DataTime)((( TextBox)Whatever.Item.Parent.Parent.Child [0] .Parent.BrotherInLaw).Text).IntValue.ParseExact('yyyy / mm / dd')),您在那儿”我会严重伤害某人。 当然,DataGrid有它的错误,但是.DataBind和蛮力将其微妙得多。 XML DOM发生了一些问题,这都是QuickWatch的错。 “天哪,我可以看到这些数据,所以我可以为它偷偷摸摸。” </ rant>
I needed to know when the checkbox changed, then act on it. So I remembered the order of events during a postback (and you should too):
我需要知道复选框何时更改,然后对其进行操作。 因此,我记得回发期间的事件顺序(您也应该如此):
- Events fire in control tree order 事件按控制树顺序触发
- THEN the control that CAUSED the PostBack fires it's event LAST.然后,导致回发的控件将触发事件LAST。
So, I'd get a bunch of CheckBox_Changed events, and finally a Button_Click (from an 'Update' button).
因此,我将得到一堆CheckBox_Changed事件,最后是一个Button_Click(来自“更新”按钮)。
In the Changed events I loaded a page-scoped ArrayList of things to act on, like, ProductIdsToActivateArrayList or ProductIdsToDeleteArrayList, depending on how many columns in my grid had checkboxes. As the Changed events fire, I just wanted to load up the ProductIDs for that CheckBox's row. But, how to avoid running around in the control tree? (which is, as I said before, gross)
在Changed事件中,我加载了要作用于页面范围的ArrayList,例如ProductIdsToActivateArrayList或ProductIdsToDeleteArrayList,这取决于网格中有多少列复选框。 随着Changed事件的触发,我只想为该CheckBox的行加载ProductID。 但是,如何避免在控制树中四处乱跑? (正如我之前所说的,毛额)
I needed the data to come 'along for the ride' with the CheckBox_Changed Events. So in the .ASPX page:
我需要将数据与CheckBox_Changed事件“一起使用”。 因此,在.ASPX页面中:
<ItemTemplate><asp:CheckBox id='checkbox' ProductId='<%#DataBinder.Eval(Container.DataItem, "ProductID")%> OnChecked='CheckBoxChanged'>
<ItemTemplate> <asp:CheckBox id ='checkbox'ProductId ='<%#DataBinder.Eval(Container.DataItem,“ ProductID”)%> OnChecked ='CheckBoxChanged'>
Notice that! A totally random and unknown attribute in the CheckBox's statement. Is that allowed? How will it render? Well, it is allowed (although VS.NET's Designer will complain).
注意! CheckBox语句中的一个完全随机且未知的属性。 可以吗它将如何呈现? 好吧,这是允许的(尽管VS.NET的Designer会抱怨)。
It renders, interestingly enough, like this:
有趣的是,它像这样渲染:
<span ProductId="4"><input type="checkbox" id="checkbox" name="checkbox"></span>
<span ProductId =“ 4”> <input type =“ checkbox” id =“ checkbox” name =“ checkbox”> </ span>
Look at the extra span! Crazay. Then in the CheckBoxChanged event on the server-side after someone clicks a bunch of CheckBoxes and clicks Update:
看看额外的跨度! 疯了然后,在有人单击一堆CheckBox并单击Update之后,在服务器端的CheckBoxChanged事件中:
public void CheckBoxChanged(object sender, EventArgs e)
{
string ProductID = ((CheckBox)sender).Attributes["ProductID"]).ToString();
ProductsToDeleteArrayList.Add(ProductID);}公共无效CheckBoxChanged(对象发送者,EventArgs e) { 字符串ProductID =((CheckBox)sender).Attributes [“ ProductID”])。ToString(); ProductsToDeleteArrayList.Add(ProductID); }
Then in the Button_Click event (remember, that happens AFTER all this) I spin through the ArrayLists (there are several, one for each action) and perform the actions in a batch. This makes the Button_Click code CLEAN. It makes the CheckBoxChanged code CLEAN, and it bypasses a whole lot of running around in the control tree.
然后在Button_Click事件中(请记住,在所有这些操作之后发生),我遍历ArrayLists(有多个,每个动作一个)并批量执行这些动作。 这使Button_Click代码变得干净。 它使CheckBoxChanged代码变为CLEAN,并绕开了控制树中的所有运行过程。
Also, before I forget: Congratulations to Robert for the birth of the definitive Scrolling Data Grid. Kudos.
另外,在我忘记之前:恭喜罗伯特·最终的滚动数据网格的诞生。 荣誉
checkboxes