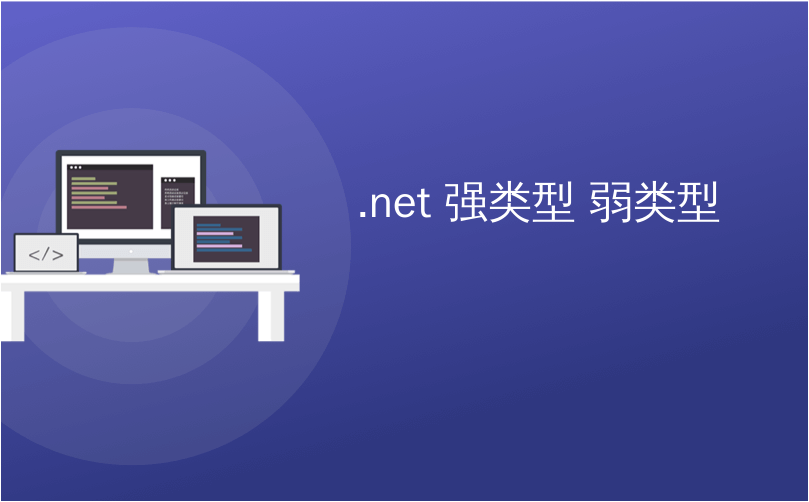
.net 强类型 弱类型
In our first look at the new features of Javascript 2.0, we will focus on the new typing system. We are just going to highlight some of the major changes and potential uses. For a more detailed look, take a look at the ECMAScript 4.0 Language Overview.
在我们初探Javascript 2.0的新功能时,我们将重点介绍新的打字系统。 我们将仅强调一些主要的变化和潜在的用途。 有关更多详细信息,请参阅ECMAScript 4.0语言概述 。
Traditionally, Javascript is a loosely-typed language, meaning that variables are declared without a type. For example:
传统上,Javascript是一种松散类型的语言,这意味着变量的声明没有类型。 例如:
`
`
var a = 42; // Number declaration
var a = 42; //数字声明
var b = “forty-two”; // String declaration
var b =“四十二”; //字符串声明
`
`
Since Javascript is loosely typed, we can get away with simple ‘var’ declarations…the language will determine which data type should be used. In contrast, Javascript 2.0 will be strongly typed, meaning that type declarations will be enforced. The syntax for applying a given type will be a colon (:) followed by the type expression. Type annotation can be added to properties, function parameters, functions (and by doing so declaring the return value type), variables, or object or array initializers. For example:
由于Javascript是松散类型的,因此我们可以避免使用简单的“ var”声明……该语言将确定应使用哪种数据类型。 相反,JavaScript 2.0将被强类型化,这意味着将强制执行类型声明。 应用给定类型的语法将是冒号(:),后跟类型表达式。 可以将类型注释添加到属性,函数参数,函数(并通过声明返回值类型),变量或对象或数组初始化程序。 例如:
`
`
var a:int = 42; //variable a has a type of int
var a:int = 42; //变量a具有int类型
var b:String = “forty-two”; //variable b has a type of String
var b:String =“四十二”; //变量b具有字符串类型
function (a:int, b:string)//the function accepts two parameters, one of type int, one of type string
函数(a:int,b:string)//该函数接受两个参数,一个为int类型,一个为string类型
function(…):int //the function returns a value with a type of int
function(…):int //函数返回一个类型为int的值
`
`
NOTE: There has been some confusion about enforcing type declarations so I thought I’d try to clear it up. Enforcing type declarations simply means that if you define a type, it will be enforced. You can choose to not define a type, in which case the variable or property defaults to a type of ‘Object’ which is the root of the type heirarchy.
注意:关于强制执行类型声明有些困惑,所以我想我会尝试清除它。 强制执行类型声明只是意味着,如果定义一个类型,它将被强制执行。 您可以选择不定义类型,在这种情况下,变量或属性默认为类型“ Object”(类型继承)的根。
类型强制 (Type Coercion)
Being a strongly typed system, Javascript 2.0 will be much less permissive with type coercion. Currently, the following checks both return true:
作为强类型系统,Javascript 2.0在类型强制方面的允许性要低得多。 当前,以下检查均返回true:
`
`
“42” == 42
“ 42” == 42
42 == “42”
42 ==“ 42”
`
`
In both cases, the language performs type coercion…Javascript automatically makes them the same type before performing the check. In Javascript 2.0, both of those statements will resolve to a ‘false’ value instead. We can still perform comparisons like those above; we just need to explicitly convert the data type using type casting. To perform the checks above and have them both resolve to ‘true’, you would have to do the following:
在这两种情况下,该语言都会执行类型强制…Javascript会在执行检查之前自动使它们成为相同的类型。 在Javascript 2.0中,这两个语句都将解析为“ false”值。 我们仍然可以像上面那样进行比较; 我们只需要使用类型转换显式转换数据类型。 要执行上面的检查并使它们都解析为“ true”,您必须执行以下操作:
`
`
int(“42”) == 42
int(“ 42”)== 42
string(42) == “42”
字符串(42)==“ 42”
`
`
While adding a strongly typed system does make the language a bit more rigid, there are some benefits to this change, particularly for applications or libraries that may be worked with elsewhere. For example, for a given method, we can specify what kinds of objects it can be a method for using the special ‘this’ annotation. I’m sure there are many of you who just re-read that sentence and are scratching your heads trying to figure out what the heck that meant. An example may help:
虽然添加强类型系统确实使语言更加僵化,但此更改有一些好处,尤其是对于可能在其他地方使用的应用程序或库。 例如,对于给定的方法,我们可以指定使用特殊的“ this”注释的方法可以是哪种对象。 我敢肯定,你们中有很多人只是重新阅读了这句话,然后挠头想弄清楚到底是什么意思。 一个示例可能会有所帮助:
`
`
- function testing(this:myObject, a:int, b:string):boolean 功能测试(this:myObject,a:int,b:string):布尔值
`
`
The method above accepts two arguments, an int and a string. The first part of the parameters (this:myObject) uses the this: annotation to state that the function can only be a method of objects that have the type of ‘myObject’. This way if someone else is using code we have created, we can restrict which objects they can use that method on, preventing it’s misuse and potential confusion.
上面的方法接受两个参数,一个int和一个字符串。 参数的第一部分(this:myObject)使用this:注释声明该函数只能是类型为'myObject'的对象的方法。 这样,如果其他人正在使用我们创建的代码,则可以限制他们可以使用该方法的对象,从而防止滥用和潜在的混乱。
联合类型 (Union Types)
We can also use union types to add a bit of flexibility. Union types are collections of types that can be applied to a given property. There are four predefined union types in Javascript 2.0:
我们还可以使用联合类型来增加灵活性。 联合类型是可以应用于给定属性的类型的集合。 Javascript 2.0中有四种预定义的联合类型:
`
`
type AnyString = (string, String)
类型AnyString =(字符串,字符串)
type AnyBoolean = (boolean, Boolean)
类型AnyBoolean =(布尔,布尔)
type AnyNumber = (byte, int, uint, decimal, double, Number)
类型AnyNumber =(字节,整数,整数,十进制,双精度,数字)
type FloatNumber = (double, decimal)
类型FloatNumber =(双精度,十进制)
`
`
In addition, we can set up our own union types based on what we need for a particular property:
另外,我们可以根据对特定属性的需要来设置自己的联合类型:
`
`
- type MySpecialProperty = (byte, int, boolean, string) 类型MySpecialProperty =(字节,整数,布尔值,字符串)
`
`
One final thing I would like to mention is that in contrast to Java and C++, Javascript 2.0 is a dynamically typed system, not statically typed. In a statically typed system, the compiler verifies that type errors cannot occur at run-time. Statically typing would catch a lot of potential programming errors, but it also severely alters the way Javascript can be used, and would make the language that much more rigid. Because JS 2.0 is dynamically typed, only the run-time value of a variable matters.
我要说的最后一件事是,与Java和C ++相比,Javascript 2.0是动态类型的系统,而不是静态类型的系统。 在静态类型的系统中,编译器会验证运行时不会发生类型错误。 静态类型化会捕获很多潜在的编程错误,但也会严重改变Javascript的使用方式,并使语言更加僵化。 由于JS 2.0是动态类型的,因此仅变量的运行时值很重要。
翻译自: https://timkadlec.com/2008/04/an-objective-look-at-javascript-2-0-strong-typing/
.net 强类型 弱类型