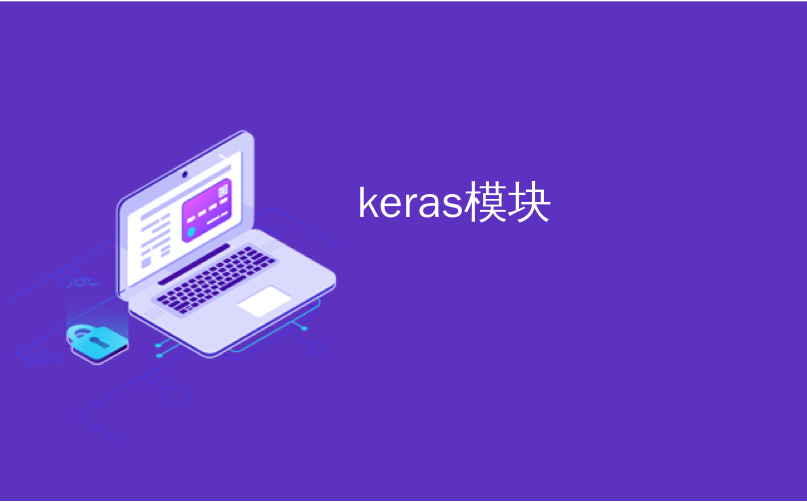
keras模块
Keras-模块 (Keras - Modules)
As we learned earlier, Keras modules contains pre-defined classes, functions and variables which are useful for deep learning algorithm. Let us learn the modules provided by Keras in this chapter.
如前所述,Keras模块包含预定义的类,函数和变量,它们对于深度学习算法很有用。 让我们学习本章中Keras提供的模块。
可用模块 (Available modules)
Let us first see the list of modules available in the Keras.
首先让我们看看Keras中可用的模块列表。
Initializers − Provides a list of initializers function. We can learn it in details in Keras layer chapter. during model creation phase of machine learning.
初始化程序 -提供初始化程序列表。 我们可以在Keras 层一章中详细了解它。 在机器学习的模型创建阶段。
Regularizers − Provides a list of regularizers function. We can learn it in details in Keras Layers chapter.
正则化器 -提供正则化器功能列表。 我们可以在Keras Layers一章中详细了解它。
Constraints − Provides a list of constraints function. We can learn it in details in Keras Layers chapter.
约束 -提供约束功能列表。 我们可以在Keras Layers一章中详细了解它。
Activations − Provides a list of activator function. We can learn it in details in Keras Layers chapter.
激活 -提供激活器功能列表。 我们可以在Keras Layers一章中详细了解它。
Losses − Provides a list of loss function. We can learn it in details in Model Training chapter.
损失 -提供损失功能列表。 我们可以在“ 模型培训”一章中详细了解它。
Metrics − Provides a list of metrics function. We can learn it in details in Model Training chapter.
指标 -提供指标列表功能。 我们可以在“ 模型培训”一章中详细了解它。
Optimizers − Provides a list of optimizer function. We can learn it in details in Model Training chapter.
优化器 -提供优化器功能列表。 我们可以在“ 模型培训”一章中详细了解它。
Callback − Provides a list of callback function. We can use it during the training process to print the intermediate data as well as to stop the training itself (EarlyStopping method) based on some condition.
回调 -提供回调函数列表。 我们可以在训练过程中使用它来打印中间数据,并根据某些条件停止训练本身( EarlyStopping方法)。
Text processing − Provides functions to convert text into NumPy array suitable for machine learning. We can use it in data preparation phase of machine learning.
文本处理 -提供将文本转换为适用于机器学习的NumPy数组的功能。 我们可以在机器学习的数据准备阶段使用它。
Image processing − Provides functions to convert images into NumPy array suitable for machine learning. We can use it in data preparation phase of machine learning.
图像处理 -提供将图像转换为适合机器学习的NumPy数组的功能。 我们可以在机器学习的数据准备阶段使用它。
Sequence processing − Provides functions to generate time based data from the given input data. We can use it in data preparation phase of machine learning.
顺序处理 -提供从给定的输入数据生成基于时间的数据的功能。 我们可以在机器学习的数据准备阶段使用它。
Backend − Provides function of the backend library like TensorFlow and Theano.
后端 -提供TensorFlow和Theano等后端库的功能。
Utilities − Provides lot of utility function useful in deep learning.
实用程序 -提供许多在深度学习中有用的实用程序功能。
Let us see backend module and utils model in this chapter.
让我们在本章中了解后端模块和utils模型。
后端模块 (backend module)
backend module is used for keras backend operations. By default, keras runs on top of TensorFlow backend. If you want, you can switch to other backends like Theano or CNTK. Defualt backend configuration is defined inside your root directory under .keras/keras.json file.
后端模块用于keras后端操作。 默认情况下,keras运行在TensorFlow后端之上。 如果需要,可以切换到Theano或CNTK等其他后端。 Defualt后端配置在.keras / keras.json文件下的根目录中定义。
Keras backend module can be imported using below code
可以使用以下代码导入Keras 后端模块
>>> from keras import backend as k
If we are using default backend TensorFlow, then the below function returns TensorFlow based information as specified below −
如果我们使用默认后端TensorFlow ,则以下函数将返回基于TensorFlow的信息,如下所示-
>>> k.backend()
'tensorflow'
>>> k.epsilon()
1e-07
>>> k.image_data_format()
'channels_last'
>>> k.floatx()
'float32'
Let us understand some of the significant backend functions used for data analysis in brief −
让我们简要地了解一些用于数据分析的重要后端功能-
get_uid() (get_uid())
It is the identifier for the default graph. It is defined below −
它是默认图形的标识符。 它定义如下-
>>> k.get_uid(prefix='')
1
>>> k.get_uid(prefix='') 2
reset_uids (reset_uids)
It is used resets the uid value.
用于重置uid值。
>>> k.reset_uids()
Now, again execute the get_uid(). This will be reset and change again to 1.
现在,再次执行get_uid() 。 它将被重置并再次更改为1。
>>> k.get_uid(prefix='')
1
占位符 (placeholder)
It is used instantiates a placeholder tensor. Simple placeholder to hold 3-D shape is shown below −
用于实例化一个占位符张量。 保持3D形状的简单占位符如下所示-
>>> data = k.placeholder(shape = (1,3,3))
>>> data
<tf.Tensor 'Placeholder_9:0' shape = (1, 3, 3) dtype = float32>
If you use int_shape(), it will show the shape.
>>> k.int_shape(data) (1, 3, 3)
点 (dot)
It is used to multiply two tensors. Consider a and b are two tensors and c will be the outcome of multiply of ab. Assume a shape is (4,2) and b shape is (2,3). It is defined below,
它用于将两个张量相乘。 考虑a和b是两个张量,而c是ab乘积的结果。 假设形状为(4,2),b形状为(2,3)。 定义如下
>>> a = k.placeholder(shape = (4,2))
>>> b = k.placeholder(shape = (2,3))
>>> c = k.dot(a,b)
>>> c
<tf.Tensor 'MatMul_3:0' shape = (4, 3) dtype = float32>
>>>
那些 (ones)
It is used to initialize all as one value.
用于将所有值初始化为一个值。
>>> res = k.ones(shape = (2,2))
#print the value
>>> k.eval(res)
array([[1., 1.], [1., 1.]], dtype = float32)
batch_dot (batch_dot)
It is used to perform the product of two data in batches. Input dimension must be 2 or higher. It is shown below −
它用于批量执行两个数据的乘积。 输入尺寸必须为2或更大。 它显示如下-
>>> a_batch = k.ones(shape = (2,3))
>>> b_batch = k.ones(shape = (3,2))
>>> c_batch = k.batch_dot(a_batch,b_batch)
>>> c_batch
<tf.Tensor 'ExpandDims:0' shape = (2, 1) dtype = float32>
变量 (variable)
It is used to initializes a variable. Let us perform simple transpose operation in this variable.
它用于初始化变量。 让我们在此变量中执行简单的转置操作。
>>> data = k.variable([[10,20,30,40],[50,60,70,80]])
#variable initialized here
>>> result = k.transpose(data)
>>> print(result)
Tensor("transpose_6:0", shape = (4, 2), dtype = float32)
>>> print(k.eval(result))
[[10. 50.]
[20. 60.]
[30. 70.]
[40. 80.]]
If you want to access from numpy −
如果您想从numpy访问-
>>> data = np.array([[10,20,30,40],[50,60,70,80]])
>>> print(np.transpose(data))
[[10 50]
[20 60]
[30 70]
[40 80]]
>>> res = k.variable(value = data)
>>> print(res)
<tf.Variable 'Variable_7:0' shape = (2, 4) dtype = float32_ref>
is_sparse(张量) (is_sparse(tensor))
It is used to check whether the tensor is sparse or not.
用于检查张量是否稀疏。
>>> a = k.placeholder((2, 2), sparse=True)
>>> print(a) SparseTensor(indices =
Tensor("Placeholder_8:0",
shape = (?, 2), dtype = int64),
values = Tensor("Placeholder_7:0", shape = (?,),
dtype = float32), dense_shape = Tensor("Const:0", shape = (2,), dtype = int64))
>>> print(k.is_sparse(a)) True
to_dense() (to_dense())
It is used to converts sparse into dense.
它用于将稀疏转换为密集。
>>> b = k.to_dense(a)
>>> print(b) Tensor("SparseToDense:0", shape = (2, 2), dtype = float32)
>>> print(k.is_sparse(b)) False
random_uniform_variable (random_uniform_variable)
It is used to initialize using uniform distribution concept.
它用于使用均匀分布概念进行初始化。
k.random_uniform_variable(shape, mean, scale)
Here,
这里,
shape − denotes the rows and columns in the format of tuples.
形状 -表示在元组的格式的行和列。
mean − mean of uniform distribution.
均值 -均匀分布的均值。
scale − standard deviation of uniform distribution.
标度 -均匀分布的标准偏差。
Let us have a look at the below example usage −
让我们看看下面的示例用法-
>>> a = k.random_uniform_variable(shape = (2, 3), low=0, high = 1)
>>> b = k. random_uniform_variable(shape = (3,2), low = 0, high = 1)
>>> c = k.dot(a, b)
>>> k.int_shape(c)
(2, 2)
utils模块 (utils module)
utils provides useful utilities function for deep learning. Some of the methods provided by the utils module is as follows −
utils为深度学习提供了有用的实用程序功能。 utils模块提供的一些方法如下-
HDF5矩阵 (HDF5Matrix)
It is used to represent the input data in HDF5 format.
它用于以HDF5格式表示输入数据。
from keras.utils import HDF5Matrix data = HDF5Matrix('data.hdf5', 'data')
to_categorical (to_categorical)
It is used to convert class vector into binary class matrix.
它用于将类向量转换为二进制类矩阵。
>>> from keras.utils import to_categorical
>>> labels = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> to_categorical(labels)
array([[1., 0., 0., 0., 0., 0., 0., 0., 0., 0.],
[0., 1., 0., 0., 0., 0., 0., 0., 0., 0.],
[0., 0., 1., 0., 0., 0., 0., 0., 0., 0.],
[0., 0., 0., 1., 0., 0., 0., 0., 0., 0.],
[0., 0., 0., 0., 1., 0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0., 1., 0., 0., 0., 0.],
[0., 0., 0., 0., 0., 0., 1., 0., 0., 0.],
[0., 0., 0., 0., 0., 0., 0., 1., 0., 0.],
[0., 0., 0., 0., 0., 0., 0., 0., 1., 0.],
[0., 0., 0., 0., 0., 0., 0., 0., 0., 1.]], dtype = float32)
>>> from keras.utils import normalize
>>> normalize([1, 2, 3, 4, 5])
array([[0.13483997, 0.26967994, 0.40451992, 0.53935989, 0.67419986]])
print_summary (print_summary)
It is used to print the summary of the model.
它用于打印模型摘要。
from keras.utils import print_summary print_summary(model)
plot_model (plot_model)
It is used to create the model representation in dot format and save it to file.
它用于以点格式创建模型表示并将其保存到文件中。
from keras.utils import plot_model
plot_model(model,to_file = 'image.png')
This plot_model will generate an image to understand the performance of model.
此plot_model将生成一张图像以了解模型的性能。
keras模块