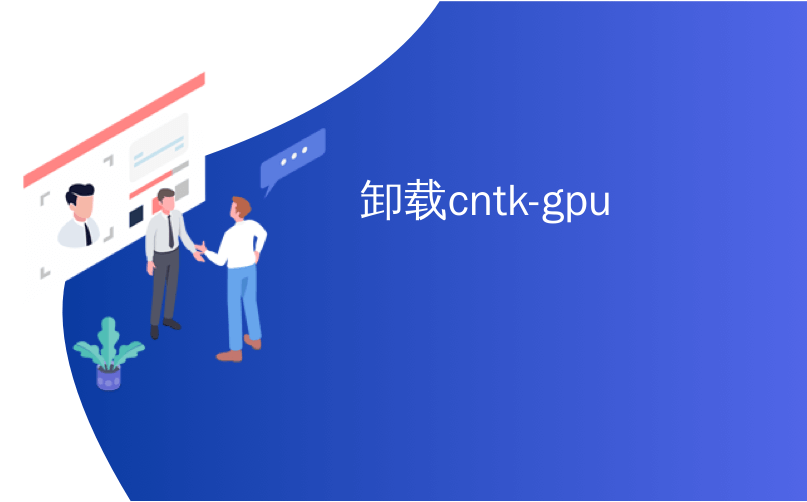
卸载cntk-gpu
CNTK-神经网络回归 (CNTK - Neural Network Regression)
The chapter will help you understand the neural network regression with regards to CNTK.
本章将帮助您了解有关CNTK的神经网络回归。
介绍 (Introduction)
As we know that, in order to predict a numeric value from one or more predictor variables, we use regression. Let’s take an example of predicting the median value of a house in say one of the 100 towns. To do so, we have data that includes −
众所周知,为了从一个或多个预测变量预测数值,我们使用回归。 让我们以预测100个城镇之一的房屋中位数为例。 为此,我们拥有的数据包括-
A crime statistic for each town.
每个城镇的犯罪统计数据。
The age of the houses in each town.
每个镇上房屋的年龄。
A measure of the distance from each town to a prime location.
从每个镇到主要位置的距离的度量。
The student-to-teacher ratio in each town.
每个镇的师生比例。
A racial demographic statistic for each town.
每个镇的种族人口统计数据。
The median house value in each town.
每个城镇的房屋中位价。
Based on these five predictor variables, we would like to predict median house value. And for this we can create a linear regression model along the lines of−
基于这五个预测变量,我们希望预测房屋中位数。 为此,我们可以沿着-
Y = a0+a1(crime)+a2(house-age)+(a3)(distance)+(a4)(ratio)+(a5)(racial)
In the above equation −
在上面的方程式中-
Y is a predicted median value
Y是预测的中值
a0 is a constant and
一个 0是常数,并且
a1 through a5 all are constants associated with the five predictors we discussed above.
一个 1至5的所有都与五个预测我们上面讨论的相关联的常数。
We also have an alternate approach of using a neural network. It will create more accurate prediction model.
我们还有使用神经网络的另一种方法。 它将创建更准确的预测模型。
Here, we will be creating a neural network regression model by using CNTK.
在这里,我们将使用CNTK创建神经网络回归模型。
加载数据集 (Loading Dataset)
To implement Neural Network regression using CNTK, we will be using Boston area house values dataset. The dataset can be downloaded from UCI Machine Learning Repository which is available at https://archive.ics.uci.edu/ml/machine-learning-databases/housing/. This dataset has total 14 variables and 506 instances.
为了使用CNTK进行神经网络回归,我们将使用波士顿地区房屋价值数据集。 可以从UCI机器学习存储库下载该数据集,该存储库可从https://archive.ics.uci.edu/ml/machine-learning-databases/housing/下载 。 该数据集共有14个变量和506个实例。
But, for our implementation program we are going to use six of the 14 variables and 100 instances. Out of 6, 5 as predictors and one as a value-to-predict. From 100 instances, we will be using 80 for training and 20 for testing purpose. The value which we want to predict is the median house price in a town. Let’s see the five predictors we will be using −
但是,对于我们的实施程序,我们将使用14个变量和100个实例中的6个。 在6个预测指标中,有5个是预测指标,一个是预测值。 从100个实例中,我们将使用80个实例进行培训,并使用20个实例进行测试。 我们要预测的值是城镇的房价中位数。 让我们看看我们将使用的五个预测变量-
Crime per capita in the town − We would expect smaller values to be associated with this predictor.
人均城市犯罪率 -我们期望与该预测因子相关的值较小。
Proportion of owner − occupied units built before 1940 - We would expect smaller values to be associated with this predictor because larger value means older house.
所有者的比例 -在1940年之前建造的居住单元-我们期望与该预测变量关联的值较小,因为较大的值表示房屋更旧。
Weighed distance of the town to five Boston employment centers.
镇距波士顿五个就业中心的距离。
Area school pupil-to-teacher ratio.
地区学校的师生比。
An indirect metric of the proportion of black residents in the town.
城镇黑人居民比例的间接指标。
准备培训和测试文件 (Preparing training & test files)
As we did before, first we need to convert the raw data into CNTK format. We are going to use first 80 data items for training purpose, so the tab-delimited CNTK format is as follows −
如我们之前所做的,首先我们需要将原始数据转换为CNTK格式。 我们将使用前80个数据项进行培训,因此制表符分隔的CNTK格式如下-
|predictors 1.612820 96.90 3.76 21.00 248.31 |medval 13.50
|predictors 0.064170 68.20 3.36 19.20 396.90 |medval 18.90
|predictors 0.097440 61.40 3.38 19.20 377.56 |medval 20.00
. . .
Next 20 items, also converted into CNTK format, will used for testing purpose.
接下来的20个项目(也转换为CNTK格式)将用于测试目的。
构造回归模型 (Constructing Regression model)
First, we need to process the data files in CNTK format and for that, we are going to use the helper function named create_reader as follows −
首先,我们需要以CNTK格式处理数据文件,为此,我们将使用名为create_reader的帮助器函数,如下所示-
def create_reader(path, input_dim, output_dim, rnd_order, sweeps):
x_strm = C.io.StreamDef(field='predictors', shape=input_dim, is_sparse=False)
y_strm = C.io.StreamDef(field='medval', shape=output_dim, is_sparse=False)
streams = C.io.StreamDefs(x_src=x_strm, y_src=y_strm)
deserial = C.io.CTFDeserializer(path, streams)
mb_src = C.io.MinibatchSource(deserial, randomize=rnd_order, max_sweeps=sweeps)
return mb_src
Next, we need to create a helper function that accepts a CNTK mini-batch object and computes a custom accuracy metric.
接下来,我们需要创建一个辅助函数,该函数接受CNTK迷你批处理对象并计算自定义精度度量。
def mb_accuracy(mb, x_var, y_var, model, delta):
num_correct = 0
num_wrong = 0
x_mat = mb[x_var].asarray()
y_mat = mb[y_var].asarray()
for i in range(mb[x_var].shape[0]):
v = model.eval(x_mat[i])
y = y_mat[i]
if np.abs(v[0,0] – y[0,0]) < delta:
num_correct += 1
else:
num_wrong += 1
return (num_correct * 100.0)/(num_correct + num_wrong)
Now, we need to set the architecture arguments for our NN and also provide the location of the data files. It can be done with the help of following python code −
现在,我们需要为NN设置架构参数,并提供数据文件的位置。 可以在以下python代码的帮助下完成-
def main():
print("Using CNTK version = " + str(C.__version__) + "\n")
input_dim = 5
hidden_dim = 20
output_dim = 1
train_file = ".\\...\\" #provide the name of the training file(80 data items)
test_file = ".\\...\\" #provide the name of the test file(20 data items)
Now, with the help of following code line our program will create the untrained NN −
现在,在以下代码行的帮助下,我们的程序将创建未经训练的NN-
X = C.ops.input_variable(input_dim, np.float32)
Y = C.ops.input_variable(output_dim, np.float32)
with C.layers.default_options(init=C.initializer.uniform(scale=0.01, seed=1)):
hLayer = C.layers.Dense(hidden_dim, activation=C.ops.tanh, name='hidLayer')(X)
oLayer = C.layers.Dense(output_dim, activation=None, name='outLayer')(hLayer)
model = C.ops.alias(oLayer)
Now, once we have created the dual untrained model, we need to set up a Learner algorithm object. We are going to use SGD learner and squared_error loss function −
现在,一旦我们创建了双重未经训练的模型,就需要建立一个Learner算法对象。 我们将使用SGD学习器和squared_error损失函数-
tr_loss = C.squared_error(model, Y)
max_iter = 3000
batch_size = 5
base_learn_rate = 0.02
sch=C.learning_parameter_schedule([base_learn_rate, base_learn_rate/2], minibatch_size=batch_size, epoch_size=int((max_iter*batch_size)/2))
learner = C.sgd(model.parameters, sch)
trainer = C.Trainer(model, (tr_loss), [learner])
Now, once we finish with Learning algorithm object, we need to create a reader function to read the training data −
现在,完成学习算法对象后,我们需要创建一个读取器函数以读取训练数据-
rdr = create_reader(train_file, input_dim, output_dim, rnd_order=True, sweeps=C.io.INFINITELY_REPEAT)
boston_input_map = { X : rdr.streams.x_src, Y : rdr.streams.y_src }
Now, it’s time to train our NN model −
现在是时候训练我们的NN模型了-
for i in range(0, max_iter):
curr_batch = rdr.next_minibatch(batch_size, input_map=boston_input_map) trainer.train_minibatch(curr_batch)
if i % int(max_iter/10) == 0:
mcee = trainer.previous_minibatch_loss_average
acc = mb_accuracy(curr_batch, X, Y, model, delta=3.00)
print("batch %4d: mean squared error = %8.4f, accuracy = %5.2f%% " \ % (i, mcee, acc))
Once we have done with training, let’s evaluate the model using test data items −
训练完成后,让我们使用测试数据项评估模型-
print("\nEvaluating test data \n")
rdr = create_reader(test_file, input_dim, output_dim, rnd_order=False, sweeps=1)
boston_input_map = { X : rdr.streams.x_src, Y : rdr.streams.y_src }
num_test = 20
all_test = rdr.next_minibatch(num_test, input_map=boston_input_map)
acc = mb_accuracy(all_test, X, Y, model, delta=3.00)
print("Prediction accuracy = %0.2f%%" % acc)
After evaluating the accuracy of our trained NN model, we will be using it for making a prediction on unseen data −
在评估了训练有素的NN模型的准确性之后,我们将使用它来对看不见的数据进行预测-
np.set_printoptions(precision = 2, suppress=True)
unknown = np.array([[0.09, 50.00, 4.5, 17.00, 350.00], dtype=np.float32)
print("\nPredicting median home value for feature/predictor values: ")
print(unknown[0])
pred_prob = model.eval({X: unknown)
print("\nPredicted value is: ")
print(“$%0.2f (x1000)” %pred_value[0,0])
完全回归模型 (Complete Regression Model)
import numpy as np
import cntk as C
def create_reader(path, input_dim, output_dim, rnd_order, sweeps):
x_strm = C.io.StreamDef(field='predictors', shape=input_dim, is_sparse=False)
y_strm = C.io.StreamDef(field='medval', shape=output_dim, is_sparse=False)
streams = C.io.StreamDefs(x_src=x_strm, y_src=y_strm)
deserial = C.io.CTFDeserializer(path, streams)
mb_src = C.io.MinibatchSource(deserial, randomize=rnd_order, max_sweeps=sweeps)
return mb_src
def mb_accuracy(mb, x_var, y_var, model, delta):
num_correct = 0
num_wrong = 0
x_mat = mb[x_var].asarray()
y_mat = mb[y_var].asarray()
for i in range(mb[x_var].shape[0]):
v = model.eval(x_mat[i])
y = y_mat[i]
if np.abs(v[0,0] – y[0,0]) < delta:
num_correct += 1
else:
num_wrong += 1
return (num_correct * 100.0)/(num_correct + num_wrong)
def main():
print("Using CNTK version = " + str(C.__version__) + "\n")
input_dim = 5
hidden_dim = 20
output_dim = 1
train_file = ".\\...\\" #provide the name of the training file(80 data items)
test_file = ".\\...\\" #provide the name of the test file(20 data items)
X = C.ops.input_variable(input_dim, np.float32)
Y = C.ops.input_variable(output_dim, np.float32)
with C.layers.default_options(init=C.initializer.uniform(scale=0.01, seed=1)):
hLayer = C.layers.Dense(hidden_dim, activation=C.ops.tanh, name='hidLayer')(X)
oLayer = C.layers.Dense(output_dim, activation=None, name='outLayer')(hLayer)
model = C.ops.alias(oLayer)
tr_loss = C.squared_error(model, Y)
max_iter = 3000
batch_size = 5
base_learn_rate = 0.02
sch = C.learning_parameter_schedule([base_learn_rate, base_learn_rate/2], minibatch_size=batch_size, epoch_size=int((max_iter*batch_size)/2))
learner = C.sgd(model.parameters, sch)
trainer = C.Trainer(model, (tr_loss), [learner])
rdr = create_reader(train_file, input_dim, output_dim, rnd_order=True, sweeps=C.io.INFINITELY_REPEAT)
boston_input_map = { X : rdr.streams.x_src, Y : rdr.streams.y_src }
for i in range(0, max_iter):
curr_batch = rdr.next_minibatch(batch_size, input_map=boston_input_map) trainer.train_minibatch(curr_batch)
if i % int(max_iter/10) == 0:
mcee = trainer.previous_minibatch_loss_average
acc = mb_accuracy(curr_batch, X, Y, model, delta=3.00)
print("batch %4d: mean squared error = %8.4f, accuracy = %5.2f%% " \ % (i, mcee, acc))
print("\nEvaluating test data \n")
rdr = create_reader(test_file, input_dim, output_dim, rnd_order=False, sweeps=1)
boston_input_map = { X : rdr.streams.x_src, Y : rdr.streams.y_src }
num_test = 20
all_test = rdr.next_minibatch(num_test, input_map=boston_input_map)
acc = mb_accuracy(all_test, X, Y, model, delta=3.00)
print("Prediction accuracy = %0.2f%%" % acc)
np.set_printoptions(precision = 2, suppress=True)
unknown = np.array([[0.09, 50.00, 4.5, 17.00, 350.00], dtype=np.float32)
print("\nPredicting median home value for feature/predictor values: ")
print(unknown[0])
pred_prob = model.eval({X: unknown)
print("\nPredicted value is: ")
print(“$%0.2f (x1000)” %pred_value[0,0])
if __name__== ”__main__”:
main()
输出量 (Output)
Using CNTK version = 2.7
batch 0: mean squared error = 385.6727, accuracy = 0.00%
batch 300: mean squared error = 41.6229, accuracy = 20.00%
batch 600: mean squared error = 28.7667, accuracy = 40.00%
batch 900: mean squared error = 48.6435, accuracy = 40.00%
batch 1200: mean squared error = 77.9562, accuracy = 80.00%
batch 1500: mean squared error = 7.8342, accuracy = 60.00%
batch 1800: mean squared error = 47.7062, accuracy = 60.00%
batch 2100: mean squared error = 40.5068, accuracy = 40.00%
batch 2400: mean squared error = 46.5023, accuracy = 40.00%
batch 2700: mean squared error = 15.6235, accuracy = 60.00%
Evaluating test data
Prediction accuracy = 64.00%
Predicting median home value for feature/predictor values:
[0.09 50. 4.5 17. 350.]
Predicted value is:
$21.02(x1000)
保存训练后的模型 (Saving the trained model)
This Boston Home value dataset has only 506 data items (among which we sued only 100). Hence, it would take only a few seconds to train the NN regressor model, but training on a large dataset having hundred or thousand data items can take hours or even days.
这个波士顿房屋价值数据集只有506个数据项(其中我们仅起诉了100个)。 因此,训练NN回归模型仅需几秒钟,但是对具有数百或数千个数据项的大型数据集进行训练可能要花费数小时甚至数天。
We can save our model, so that we won’t have to retain it from scratch. With the help of following Python code, we can save our trained NN −
我们可以保存我们的模型,这样就不必从头开始保留它。 借助以下Python代码,我们可以保存训练有素的NN-
nn_regressor = “.\\neuralregressor.model” #provide the name of the file
model.save(nn_regressor, format=C.ModelFormat.CNTKv2)
Following are the arguments of save() function used above −
以下是上面使用的save()函数的参数-
File name is the first argument of save() function. It can also be written along with the path of file.
文件名是save()函数的第一个参数。 也可以将其与文件路径一起写入。
Another parameter is the format parameter which has a default value C.ModelFormat.CNTKv2.
另一个参数是格式参数,其默认值为C.ModelFormat.CNTKv2 。
加载训练好的模型 (Loading the trained model)
Once you saved the trained model, it’s very easy to load that model. We only need to use the load () function. Let’s check this in following example −
保存训练好的模型后,很容易加载该模型。 我们只需要使用load()函数。 让我们在以下示例中进行检查-
import numpy as np
import cntk as C
model = C.ops.functions.Function.load(“.\\neuralregressor.model”)
np.set_printoptions(precision = 2, suppress=True)
unknown = np.array([[0.09, 50.00, 4.5, 17.00, 350.00], dtype=np.float32)
print("\nPredicting area median home value for feature/predictor values: ")
print(unknown[0])
pred_prob = model.eval({X: unknown)
print("\nPredicted value is: ")
print(“$%0.2f (x1000)” %pred_value[0,0])
The benefit of saved model is that once you load a saved model, it can be used exactly as if the model had just been trained.
保存的模型的好处是,一旦加载了保存的模型,就可以像使用模型一样对它进行精确的使用。
卸载cntk-gpu