1.创建Springboot工程
2.创建数据库及表
创建库表语句可以在idea中完成,也可以在Navicat 15 for MySQL数据库连接工具中实现
这里以在idea创建数据库为例:
打开Navicat软件,找到我们创建的数据库名并打开
3.在我们新创建的springboot工程中找到pom.xml配置文件,在里面添加MP依赖
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.16</version>
</dependency>
注意:依赖要放在<dependencies></dependencies>中
添加完依赖别忘了刷新 Maven
4.添加MP的相关配置信息
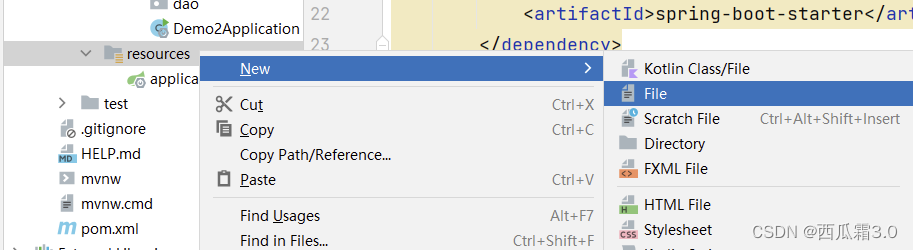
在application.yml中添加以下信息:
spring:
datasource:
type: com.alibaba.druid.pool.DruidDataSource
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/mybatisplus_test?characterEncoding=utf-8&serverTimezone=GMT%2B8
username: root
password: root
语句格式看清楚,避免产生错误
spring.datasource.type :配置使用什么数据源
driver-class-name:根据url自动识别
url:jdbc指我们使用jdbc的驱动方式连接数据库;mysql表示连接的数据库类型是mysql;localhost是数据库的具体地址;3306是数据库的端口号;mybatisplus_test是数据库名;characterEncoding设置编码格式;serverTimezone设置时区,GMT%2B8表示的是东8时区也就是北京时间。
username:数据库账号
password:数据库密码
5.根据数据库表创建实体类
package com.it.demo2.domain;
public class User {
private Long id;
private String name;
private String password;
private Integer age;
private String tel;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", password='" + password + '\'' +
", age=" + age +
", tel='" + tel + '\'' +
'}';
}
}
这样看set and get方法和tostring方法有点臃肿,可以在实体类上方添加@Data注解,@Data注解包含上面注解的功能,不过使用@Data注解需要安装lombok插件,新版idea内置lombok,自需要添加注解即可
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
package com.it.demo2.domain;
import lombok.Data;
@Data
public class User {
private Long id;
private String name;
private String password;
private Integer age;
private String tel;
}
添加完注解后就清爽多了
6.创建Dao接口
package com.it.demo2.dao;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.it.demo2.domain.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserDao extends BaseMapper<User> {
}
7.编写引导类
其实我们创建springboot工程时,引导类自动创建了
但是如果Dao接口要被容器扫描到有两种方法
1.在引导类上添加@MapperScan注解,其属性为所要扫描的Dao所在包。
package com.it.demo2;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.it.demo2.dao")
public class Demo2Application {
public static void main(String[] args) {
SpringApplication.run(Demo2Application.class, args);
}
}
好处为只需要在引导类上写一次就可以扫描到指定报下的所有Dao接口,Dao接口上的@Mapper就可以不用写了。
2.在Dao接口上添加@Mapper注解,并且确保Dao处在引导类所在包或其子包中
package com.it.demo2.dao;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.it.demo2.domain.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserDao extends BaseMapper<User> {
}
缺点是每个Dao接口上都需要写上@Mapper注解
8.编写测试类
package com.it.demo2;
import com.it.demo2.dao.UserDao;
import com.it.demo2.domain.User;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.List;
@SpringBootTest
class Demo2ApplicationTests {
@Autowired
private UserDao userDao;
@Test
void testgeuAll() {
List<User> userList = userDao.selectList(null);
System.out.println(userList);
}
}
运行结果为
可以发现结果和我们数据库中的信息是一致的
查询成功
完整的项目结构为