DoublyLinkedList.h
#pragma once
#include <iostream>
using namespace std;
template<class T>
struct Node
{
public:
T value;
Node *prev;
Node *next;
Node() {}
Node(T t, Node *prev, Node *next)
{
this->value = t;
this->prev = prev;
this->next = next;
}
};
template<class T>
class DoublyLink
{
public:
DoublyLink();
~DoublyLink();
int Size();
int IsEmpty();
T Get(int index);
T GetFirst();
T GetLast();
int Insert(int index, T t);
int InsertFirst(T t);
int InsertLast(T t);
int Del(int index);
int DeleteFirst();
int DeleteLast();
private:
int count;
Node<T> *phead;
Node<T>* GetNode(int index);
};
template<class T>
DoublyLink<T>::DoublyLink() : count(0)
{
phead = new Node<T>();
phead->prev = phead->next = phead;
}
template<class T>
DoublyLink<T>::~DoublyLink()
{
Node *ptmp;
Node *pnode = phead->next;
while (pnode != phead)
{
ptmp = pnode;
pnode = pnode->next;
delete ptmp;
}
delete phead;
phead = NULL;
}
template<class T>
int DoublyLink<T>::Size()
{
return count;
}
template<class T>
int DoublyLink<T>::IsEmpty()
{
return count == 0;
}
template<class T>
Node<T>* DoublyLink<T>::GetNode(int index)
{
if (index < 0 || index >= count)
{
cout << "GetNode failed! Index is out of range." << endl;
return NULL;
}
if (index < count / 2)
{
int i = 0;
Node<T> *pnode = phead->next;
while ((i++) < index)
{
pnode = pnode->next;
}
return pnode;
}
int i = count - 1;
Node<T> *pnode = phead->prev;
while ((i--) > index)
{
pnode = pnode->prev;
}
return pnode;
}
template<class T>
T DoublyLink<T>::Get(int index)
{
return GetNode(index)->value;
}
template<class T>
T DoublyLink<T>::GetFirst()
{
return GetNode(0)->value;
}
template<class T>
T DoublyLink<T>::GetLast()
{
return GetNode(count - 1)->value;
}
template<class T>
int DoublyLink<T>::Insert(int index, T t)
{
if (index == 0)
return InsertFirst(t);
Node<T> *pindex = GetNode(index);
Node<T> *pnode = new Node<T>(t, pindex->prev, pindex);
pindex->prev->next = pnode;
pindex->prev = pnode;
count++;
return 0;
}
template<class T>
int DoublyLink<T>::InsertFirst(T t)
{
Node<T> *pnode = new Node<T>(t, phead, phead->next);
phead->next->prev = pnode;
phead->next = pnode;
count++;
return 0;
}
template<class T>
int DoublyLink<T>::InsertLast(T t)
{
Node<T> *pnode = new Node<T>(t, phead->prev, phead);
phead->prev->next = pnode;
phead->prev = pnode;
count++;
return 0;
}
template<class T>
int DoublyLink<T>::Del(int index)
{
Node<T> *pnode = GetNode(index);
pnode->next->prev = pnode->prev;
pnode->prev->next = pnode->next;
delete pnode;
count--;
return 0;
}
template<class T>
int DoublyLink<T>::DeleteFirst()
{
return Del(0);
}
template<class T>
int DoublyLink<T>::DeleteLast()
{
return Del(count - 1);
}
DoublyLinkedList.cpp
#include <iostream>
#include <DoublyLinkedList.h>
#include <sstream>
using namespace std;
void int_test()
{
int iarr[4] = { 10, 20, 30, 40 };
cout << "\n----int_test----" << endl;
DoublyLink<int> *link = new DoublyLink<int>();
link->InsertFirst(iarr[0]);
link->InsertLast(iarr[1]);
link->Insert(1, iarr[2]);
link->Insert(2, iarr[3]);
cout << "IsEmpty:" << link->IsEmpty() << endl;
cout << "Size:" << link->Size() << endl;
int size = link->Size();
for (int i = 0; i < size; i++)
{
cout << "第" << i << "个元素为:" << link->Get(i) << endl;
}
}
void string_test()
{
string sarr[4] = { "one", "two", "three", "four" };
cout << "\n----string_test----" << endl;
DoublyLink<string> *link = new DoublyLink<string>();
link->InsertFirst(sarr[0]);
link->InsertLast(sarr[1]);
link->Insert(1, sarr[2]);
link->Insert(2, sarr[3]);
cout << "IsEmpty:" << link->IsEmpty() << endl;
cout << "Size:" << link->Size() << endl;
int size = link->Size();
for (int i = 0; i < size; i++)
{
cout << "第" << i << "个元素为:" << link->Get(i) << endl;
}
}
struct stu
{
int id;
char name[20];
};
static stu arr_stu[] =
{
{ 10,"andy" },
{ 20,"baby" },
{ 30,"city" },
{ 40,"dog" },
};
#define ARR_STU_SIZE ((sizeof(arrstu))/(sizeof(arrstu[0])))
void object_test()
{
cout << "\n----object_test----" << endl;
DoublyLink<stu> *link = new DoublyLink<stu>();
link->InsertFirst(arr_stu[0]);
link->InsertLast(arr_stu[1]);
link->Insert(1, arr_stu[2]);
link->Insert(2, arr_stu[3]);
cout << "IsEmpty:" << link->IsEmpty() << endl;
cout << "Size:" << link->Size() << endl;
int size = link->Size();
for (int i = 0; i < size; i++)
{
cout << "第" << i << "个元素为:" << link->Get(i).id << " " << link->Get(i).name << endl;
}
}
int main()
{
int_test();
string_test();
object_test();
cout << endl;
system("pause");
return 0;
}
测试结果
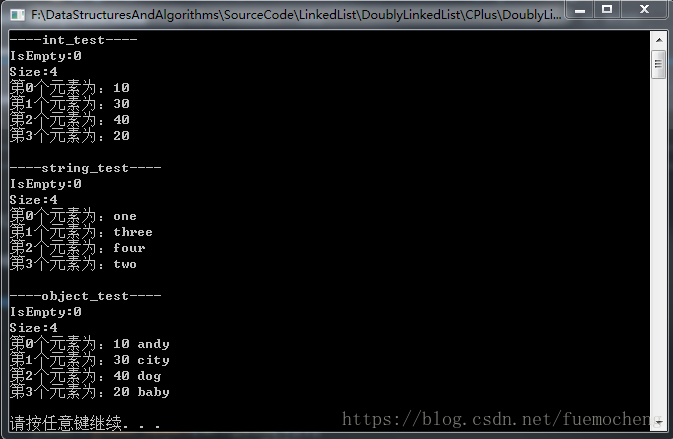
参考:http://wangkuiwu.github.io/2013/01/01/dlink/