a = 10
type(a)
a = 'I said :"stupid! '
type(a)
>> str
print('Use \n to print a new line')
s = 'Hello World'
# Grab everything but the last letter
s[:-1]
# Concatenate strings!
s + ' concatenate me!'
#We can use the multiplication symbol to create repetition!
letter = 'z'
letter*10
>> 'zzzzzzzzzz'
# Upper Case a string
s.upper()
>>'HELLO WORLD CONCATENATE ME!'
# Lower case
s.lower()
# Split a string by blank space (this is the default)
s.split()
>> ['Hello', 'World', 'concatenate', 'me!']
# Split by a specific element (doesn't include the element that was split on)
s.split('W')
>> ['Hello ', 'orld concatenate me!']
'Insert another string with curly brackets: {}'.format('The inserted string')
print("I'm going to inject %s here." %'something')
print("I'm going to inject %s text here, and %s text here." %('some','more'))
print("I'm going to inject %s text here, and %s text %d here." %('some','more',1))
#You can also pass variable names:
x, y = 'some', 'more'
print("I'm going to inject %s text here, and %s text here."%(x,y))
print('He said his name was %s.' %'Fred')
print('He said his name was %r.' %'Fred')
>>He said his name was Fred.
He said his name was 'Fred'.
print('I once caught a fish %s.' %'this \tbig')
print('I once caught a fish %r.' %'this \tbig')
>>I once caught a fish this big.
I once caught a fish 'this \tbig'.
print('First: %s, Second: %5.2f, Third: %r' %('hi!',3.1415,'bye!'))
print('The {2} {1} {0}'.format('fox','brown','quick'))
>>The quick brown fox
# Inserted objects can be assigned keywords
print('First Object: {a}, Second Object: {b}, Third Object: {c}'.format(a=1,b='Two',c=12.3))
>> First Object: 1, Second Object: Two, Third Object: 12.3
print('A {p} saved is a {p} earned.'.format(p='penny'))
## Alignment, padding and precision with .format()
## Within the curly braces you can assign field lengths, left/right alignments, rounding parameters and more
print('{0:8} | {1:10}'.format('Fruit', 'Quantity'))
print('{0:8} | {1:10}'.format('Apples', 3.))
print('{0:8} | {1:10}'.format('Oranges', 10))
>> Fruit | Quantity
>> Apples | 3.0
>> Oranges | 10
print('{0:<8} | {1:^8} | {2:>8}'.format('Left','Center','Right'))
print('{0:<8} | {1:^8} | {2:>8}'.format(11,22,33))
>> Left | Center | Right
>> 11 | 22 | 33
#You can precede the aligment operator with a padding character
print('{0:=<8} | {1:-^8} | {2:.>8}'.format('Left','Center','Right'))
print('{0:=<8} | {1:-^8} | {2:.>8}'.format(11,22,33))
>> Left==== | -Center- | ...Right
>> 11====== | ---22--- | ......33
#The following two print statements are equivalent:
print('This is my ten-character, two-decimal number:%10.2f' %13.579)
print('This is my ten-character, two-decimal number:{0:10.2f}'.format(13.579))
## Formatted String Literals (f-strings) offer several benefits over the older .format() string method described above. For one, you can bring outside variables immediately into to the string rather than pass them as arguments through .format(var).
name = 'Fred'
print(f"He said his name is {name}.")
#Pass !r to get the string representation:
print(f"He said his name is {name!r}")
>> He said his name is 'Fred'
#Float formatting follows "result: {value:{width}.{precision}}"
num = 23.45678
print("My 10 character, four decimal number is:{0:10.4f}".format(num))
print(f"My 10 character, four decimal number is:{num:{10}.{6}}")
num = 23.45
print("My 10 character, four decimal number is:{0:10.4f}".format(num))
print(f"My 10 character, four decimal number is:{num:10.4f}")
## We can also use + to concatenate lists, just like we did for strings.
my_list + ['new item']
# We can also use the * for a duplication method similar to strings:
# Make the list double
my_list * 2
list1 = [1, 2, 3, 'append me!']
#Use pop to "pop off" an item from the list. By default pop takes off the last index, but you can also specify which index to pop off. Let's see an example:
# Pop off the 0 indexed item
list1.pop(0)
>> 1
# Show
list1
>> [2, 3, 'append me!']
# Assign the popped element, remember default popped index is -1
popped_item = list1.pop()
popped_item
>> 'append me!'
new_list = ['a','e','x','b','c']
# Use reverse to reverse order (this is permanent!)
new_list.reverse()
# Use sort to sort the list (in this case alphabetical order, but for numbers it will go ascending)
new_list.sort()
## Python has an advanced feature called list comprehensions. They allow for quick construction of lists.
# Build a list comprehension by deconstructing a for loop within a []
first_col = [row[0] for row in matrix]
## A Python dictionary consists of a key and then an associated value. That value can be almost any Python object.
my_dict = {'key1':123,'key2':[12,23,33],'key3':['item0','item1','item2']}
# We can also create keys by assignment. For instance if we started off with an empty dictionary, we could continually add to it:
# Create a new dictionary
d = {}
# Create a new key through assignment
d['animal'] = 'Dog'
d['answer'] = 42
d
# Dictionary nested inside a dictionary nested inside a dictionary
d = {'key1':{'nestkey':{'subnestkey':'value'}}}
# Keep calling the keys
d['key1']['nestkey']['subnestkey']
#A few Dictionary Methods
d.keys()
d.values()
# the above two will return dict_keys and dict_values, then we do list() to turn this into list
p = np.array(list(d.values()))
# Method to return tuples of all items
d.items()
##--------------dictionary to numpy -----------------------
import numpy as np
result = {0: 1.1181753789488595, 1: 0.5566080288678394, 2: 0.4718269778030734, 3: 0.48716683119447185, 4: 1.0, 5: 0.1395076201641266, 6: 0.20941558441558442}
names = ['id','data']
formats = ['f8','f8']
dtype = dict(names = names, formats=formats)
array = np.array(list(result.items()), dtype=dtype)
print(repr(array))
>> array([(0., 1.11817538), (1., 0.55660803), (2., 0.47182698),
(3., 0.48716683), (4., 1. ), (5., 0.13950762),
(6., 0.20941558)], dtype=[('id', '<f8'), ('data', '<f8')])
## Tuples built-in methods:
# Use .index to enter a value and return the index
t.index('one')
# Use .count to count the number of times a value appears
t.count('one')
# Immutability :It can't be stressed enough that tuples are immutable. To drive that point home!
t[0]= 'change'
>> TypeError: 'tuple' object does not support item assignment
# Because of this immutability, tuples can't grow. Once a tuple is made we can not add to it.
t.append('nope')
>>'tuple' object has no attribute 'append'
## Sets are an unordered collection of unique elements. We can construct them by using the set() function.
x = set()
# We add to sets with the add() method
x.add(1)
#Show
x
>> {1}
#Note the curly brackets. This does not indicate a dictionary! Although you can draw analogies as a set being a dictionary with only keys.
# Try to add the same element
x.add(1)
#Show
x
>> {1}
# That's because a set is only concerned with unique elements! We can cast a list with multiple repeat elements to a set to get the unique elements.
# Create a list with repeats
list1 = [1,1,2,2,3,4,5,6,1,1]
list(set(list1))
Python3 basics
最新推荐文章于 2022-11-25 17:37:46 发布
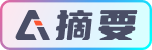