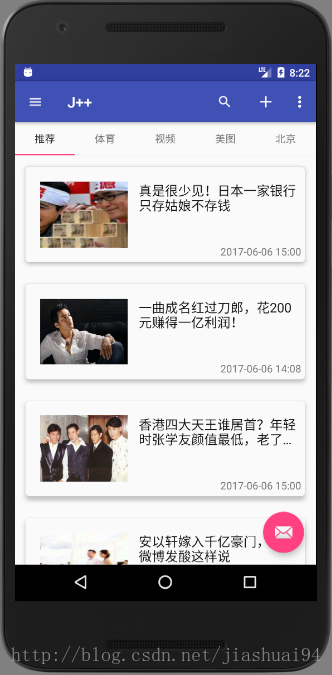
简介
如今,Android中也有自带这种指示器的控件TabLayout。TabLayout存在于android design库中,它提供了一个水平的布局来展示Tabs。
使用
1、添加依赖
当然了,第一步还是添依赖,好无聊
compile 'com.android.support:design:26.0.0-alpha1'
2、布局中使用
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
>
<android.support.design.widget.TabLayout
android:id="@+id/tab"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.design.widget.TabItem
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="推荐"/>
<android.support.design.widget.TabItem
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="娱乐"/>
<android.support.design.widget.TabItem
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="体育"/>
</android.support.design.widget.TabLayout>
</LinearLayout>
类似于RadioGroup一样,使用TabLayout作为父布局,其内部添加TabItem控件。其中text就是指示器的文字提示。
当然了,不仅可以在不居中直接添加TabItem子标签,这样可变性不好,我们可以在代码中动态添加,节后会介绍到!
3、动态添加TabItem标签
TabLayout tabLayout = (TabLayout) findViewById(R.id.tab);
for (int i = 0; i < titles.length; i++) {
TabLayout.Tab tab = tabLayout.newTab();
tab.setText(titles[i]);
tabLayout.addTab(tab);
}
这只是一种简单的动态添加TabItem标签的方法。
4、添加TabItem标签的点击事件
tabLayout.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
Toast.makeText(TabLayoutActivity.this, tab.getText(), Toast.LENGTH_SHORT).show();
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
});
5、为Tab添加图标
for (int i = 0; i < titles.length; i++) {
TabLayout.Tab tab = tabLayout.newTab()
tab.setText(titles[i])
tab.setIcon(R.mipmap.ic_launcher)
tabLayout.addTab(tab)
}
结合第三步,创建Tab对象,对其设置文字和图片
6、修改TabLayout的样式
Tablayout支持定制化修改,提供了不少自定义属性供开发者进行设置。有以下属性支持修改:
- tabIndicatorColor:指示器颜色
- tabBackground:tablayout的背景颜色
- tabIndicatorHeight:指示器高度
- tabGravity:指示器位置
- tabMode:显示模式
- tabSelectedTextColor:文字选中颜色
- tabTextColor:文字未选中颜色
- tabTextAppearance:字体
- tabMaxWidth:tab最大宽度
- tabMinWidth:tab最小宽度
关键的来了!
·a 创建布局
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context=".activity.MainActivity"
tools:showIn="@layout/activity_main">
<android.support.design.widget.TabLayout
android:id="@+id/tab"
android:layout_width="match_parent"
android:layout_height="45dp"
app:tabGravity="fill"
app:tabMode="fixed" />
<View
android:id="@+id/fenge"
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_below="@id/tab"
android:background="#f2f2f2" />
<android.support.v4.view.ViewPager
android:id="@+id/vp_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/fenge" />
</RelativeLayout>
vp_content.setAdapter(new FragmentPagerAdapter(getSupportFragmentManager()) {
private String[] titles={"推荐","体育","视频","美图","北京"};
@Override
public Fragment getItem(int position) {
if(position==0){
return new TuijianFragment();
}else if(position==1){
return new SportsFragment();
}else if(position==2){
return new VideoFragment();
}else if(position==3){
return new PictureFragment();
}else {
return new BeijingFragment();
}
}
@Override
public int getCount() {
return 5;
}
@Override
public CharSequence getPageTitle(int position) {
return titles[position];
}
});
tab.setupWithViewPager(vp_content);