# 8-1 消息
def display_message(thing):
print(f"本章学习{thing}")
display_message('函数')
display_message('元组')
# 8-2 喜欢的图书
def favorite_book(title):
print(f"One of my favorite books is {title}")
favorite_book('Alice in Wonderland')
# 8-3 T恤
def make_shirt(size, title):
print(f"这件T恤的尺码是:{size}号,印有'{title}'字样")
make_shirt(size='大', title = 'I love Python')
make_shirt('大', 'I love Python')
# 8-4 大号T恤
def make_shirt(size, title):
print(f"制作一件印有字样'{title}'的{size}号T恤。")
make_shirt('大', 'I love Python')
make_shirt('中', 'I love Python')
make_shirt('小', 'I love Python')
# 8-5 城市
def describe_city(city, nation='China'):
print(f"{city} is in {nation}")
describe_city(city = 'Shanghai')
describe_city('Taiwan')
describe_city('Reykjavik', 'Iceland')
# 8-6 城市名
def city_country(city, country):
str1 = city + ', ' + country
return str1
c_c1 = city_country('Taibei', 'China')
c_c2 = city_country('Reykjavik', 'Iceland')
c_c3 = city_country('santiago', 'Chile')
print(c_c1)
print(c_c2)
print(c_c3)
# 8-7 专辑
def make_album(singer, name, num = ''):
album = {'singer':singer, 'name':name}
if num:
album['num'] = num
return album
ma1 = make_album('Lijian', 'efaf')
print(ma1)
ma2 = make_album('DengZiqi', 'drms', 10)
print(ma2)
ma3 = make_album('LiWen', 'forever', '8')
print(ma3)
# 8-8 用户的专辑
def make_album(singer, name, num = ''):
album = {'singer': singer_name, 'name': album_name, 'num':album_num}
return album
while True:
print("Please tell singer_name,album_name,album_num.")
print("enter 'q' at any time to quit")
singer_name = input("singer_name:")
if singer_name == 'q':
break
album_name = input("album_name:")
if album_name == 'q':
break
album_num = input("album_num:")
if album_num == 'q':
break
a = make_album(singer_name, album_name, album_num)
print(a)
# 8-9 魔术师
def show_magicians(names):
for name in names:
print(name)
magicians_1 = ['ming', 'tian', 'geng', 'mei', 'hao']
show_magicians(magicians_1)
# 8-10 了不起的魔术师
def make_great(bf_names, af_names):
while bf_names:
current_name = bf_names.pop()
current_name += 'the Great'
af_names.append(current_name)
def show_magicians(bf_names, af_names):
print("bf_names:")
for bf_name in bf_names:
print(bf_name)
print("\naf_names:")
for af_name in af_names:
print(af_name)
bf_names = ['ming', 'tian', 'geng', 'mei', 'hao']
af_names = []
make_great(bf_names, af_names)
show_magicians(bf_names, af_names)
# 8-11 不变的魔术师
def make_great(bf_names, af_names):
while bf_names:
current_name = bf_names.pop()
current_name += 'the Great'
af_names.append(current_name)
def show_magicians(bf_names, af_names):
print("bf_names:")
for bf_name in bf_names:
print(bf_name)
print("\naf_names:")
for af_name in af_names:
print(af_name)
bf_names = ['ming', 'tian', 'geng', 'mei', 'hao']
af_names = []
make_great(bf_names[:], af_names) # 添加一个副本
show_magicians(bf_names, af_names)
# 8-12 三明治
def make_sandwich(*requests):
print("\nMaking a sandwich with the following requests: ")
for request in requests:
print("- " + request)
make_sandwich('pepperoni')
make_sandwich('mushrooms', 'extra cheese')
make_sandwich('mushrooms', 'green peppers', 'extra cheese')
# 8-13 用户简介
def build_profile(first, last, **user_info):
profile = {}
profile['first_name'] = first
profile['last_name'] = last
for key, value in user_info.items():
profile[key] = value
return profile
user_profile = build_profile('Dashen', 'Sun',
location = 'Hefei' ,
university ='USTC',
field = 'quantum')
print(user_profile)
# 8-14 汽车
def make_car(brand, model, **info):
profile = {}
profile['brand_name'] = brand
profile['model_name'] = model
for k,v in info.items():
profile[k] = v
return profile
info = make_car('BYD', 'SUV',
location = 'Shenzhen',
nation = 'China',
color= 'yellow')
print(info)
# 8-15 打印模型
from printing_functions import print_models as pm
from printing_functions import show_completed_models as scm
# from printing_functions import *
unprinted_designs = ['iphone case', 'robot pendant', 'dodecahedron']
completed_models = []
pm(unprinted_designs,completed_models) # 调用函数
scm(completed_models)
print("\nunprinted_designs: ")
print(unprinted_designs)
print("\ncompleted_models:")
print(completed_models)
# 8-16 导入
# 1.导入整个模块
import build_profile123
user_profile = build_profile123.build_profile('albert', 'einstein',
location = 'princeton',
field = 'physics')
print(user_profile)
# 2.导入特定的函数
from build_profile123 import build_profile
user_profile = build_profile('albert', 'einstein',
location = 'princeton',
field = 'physics')
print(user_profile)
# 3.使用as给函数指定别名
from build_profile123 import build_profile as bp
user_profile = bp('albert', 'einstein',
location = 'princeton',
field = 'physics')
print(user_profile)
# 4.使用as给模块指定别名
import build_profile123 as bp123
user_profile = bp123.build_profile('albert', 'einstein',
location = 'princeton',
field = 'physics')
print(user_profile)
# 5.导入模块中的所有函数
from build_profile123 import *
user_profile = build_profile('albert', 'einstein',
location = 'princeton',
field = 'physics')
print(user_profile)
《Python编程:从入门到实践》习题答案——第8章 函数
最新推荐文章于 2024-07-30 17:23:09 发布
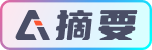