In order to avoid midair collisions, most commercial flights are monitored by ground-based air traffic control centers that track their position using radar. For this problem, you will be given information on a set of airplanes and a set of control centers, and you must compute how monitoring of the airplanes is distributed among the control centers. The position of each airplane is represented by a unique (x, y)coordinate pair. For the purpose of this problem, the height (altitude) of the airplanes can be ignored.
The number of airplanes that can be monitored by a given control center varies from time to time due to changes in staff and equipment. At any given time, each control center monitors as many planes as it can, choosing the airplanes to be monitored according to the following priorities:
-
(1)
- it will prefer to monitor planes that are closer to the control center rather than ones that are farther away; (2)
- if two airplanes are equally distant from the center and the center can monitor only one of them, it will choose the one that is farther to the north (positive y-axis); (3)
- if two airplanes are equally distant and have the same y-coordinate, the center will give preference to the airplane that is farther to the east (positive x-axis).
At any given moment, each control center has a circular ``span of control" whose radius is the distance to the farthest airplane being monitored by the control center. All airplanes inside the span of control are monitored by the control center. Airplanes on the boundary of the span of control may or may not be monitored by the control center, depending on its capacity and on the priorities listed above.
You will not be given the positions of the control centers. Instead, for each control center, you will be given the number of airplanes that it is currently monitoring, and two points that are on the boundary of its current span of control. With this information, you can compute the position of the control center and decide which airplanes it is monitoring. If the data is consistent with more than one possible span of control, you should choose the span that includes the airplane that is farthest to the north, breaking ties by choosing the airplane that is farthest to the north then to the east.
The figure below, which shows four airplanes and two control centers, illustrates the problem. Each control center is represented by a circular span of control and by two points on the boundary of this span, labeled A and B. P1, P2, P3, and P4 label the four airplanes. In this example, airplanes P1 and P4 are each being monitored by a single control center, airplane P3 is being monitored by two control centers, and airplane P2 is not being monitored by either control center.
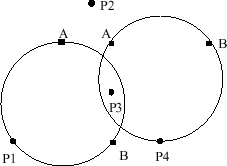
Input
The input consists of several trial data sets. The first line of input in each trial data set contains two integers NP ( 0 < NP < 100 ) and NC ( 0 < NC < 10 ), which represent the number of airplanes and the number of control centers, respectively. Each of the next NP lines contains two floating-point numbers that represent the (x, y) coordinates of one airplane. Each of the next NC lines describes one control center. Each contains an integer between 0 and NP (inclusive) indicating the number of airplanes monitored by the control center, followed by two pairs of floating point numbers that represent the (x, y) coordinates of two points on the boundary of its span of control (neither of which is the position of an airplane). If two distances differ by less than 0.00001, you should treat them as the same distance.The last data set is followed by a line containing two zeros.
Output
For each trial, compute the number of airplanes that are monitored by zero control centers, the number of airplanes that are monitored by one control center, and so on up to the number of airplanes that are monitored by NC control centers. Print the trial number followed by a sequence of NC + 1 integers, where the i -th integer in the sequence represents the number of airplanes that are monitored by i - 1 control centers. If data for one of the control centers is inconsistent, print ` Impossible ' instead of the sequence of integers for that trial. Use the format shown in the example output, and print a blank line after each trial.Sample Input
4 2 3.0 0.0 0.0 0.0 1.6 2.8 2.0 1.0 2 1.0 2.0 2.0 0.0 2 2.0 2.0 4.0 2.0 2 1 0.0 0.5 0.0 -0.5 0 -1.0 0.0 1.0 0.0 0 0
Sample Output
Trial 1: 1 2 1 Trial 2: Impossible
#include<stdio.h>
#include<algorithm>
#include<string.h>
#include<stdlib.h>
#include<math.h>
using namespace std;
const double eps=1e-5;
struct coor
{
double x,y;
};
double r;
int C,NP,NC,m,i,j,k,ok;
int covers[100],ans[10],cov[100],Tc[100];
coor A,B;
coor P[100];
coor operator -(coor a,coor b)
{
a.x-=b.x;
a.y-=b.y;
return a;
}
bool cmp(coor a,coor b)
{
return a.y>b.y+eps || (a.y>b.y-eps && a.x>b.x);
}
double len(coor a)
{
return sqrt(a.x*a.x + a.y*a.y);
}
bool cross(coor A,coor B,coor C,coor &O)
{
double A1,B1,C1,A2,B2,C2,tmp;
A1=B.x-A.x;
B1=B.y-A.y;
C1=(A.y*A.y-B.y*B.y+A.x*A.x-B.x*B.x)/2.0;
A2=C.x-A.x;
B2=C.y-A.y;
C2=(A.y*A.y-C.y*C.y+A.x*A.x-C.x*C.x)/2.0;
tmp=B1*A2-B2*A1;
if(fabs(tmp)<eps)
return false;
O.y=(C2*A1-C1*A2)/tmp;
O.x=(C1*B2-C2*B1)/tmp;
return true;
}
bool check()
{
int i;
for(i=0;i<NP;i++)
{
if(cov[i]&&!Tc[i])
return false;
if(!cov[i]&&Tc[i])
return true;
}
return true;
}
void work(coor A,coor B,coor C)
{
coor O;
double Tr;
int i,in,on;
if(!cross(A,B,C,O))
return ;
Tr=len(A-O);
in=0;on=0;
for(i=0;i<NP;i++)
if(len(O-P[i])<Tr-eps)
in++;
else if(len(O-P[i])<Tr+eps)
on++;
if(in>m || in+on<m)
return;
on=m-in;
for(i=0;i<NP;i++)
if(len(O-P[i])<Tr-eps)
Tc[i]=1;
else if(len(O-P[i])<Tr+eps && on)
{
Tc[i]=1;on--;
}
else
Tc[i]=0;
if(r<0||Tr<r-eps||(Tr<r+eps && check()))
{
r=Tr;
for(i=0;i<NP;i++)
cov[i]=Tc[i];
}
}
int main()
{
while(scanf("%d%d",&NP,&NC)&&NP)
{
for(i=0;i<NP;i++)
scanf("%lf %lf",&P[i].x,&P[i].y);
sort(P,P+NP,cmp);
memset(covers,0,sizeof(covers));
ok=1;
for(i=0;i<NC;i++)
{
scanf("%d %lf %lf %lf %lf",&m,&A.x,&A.y,&B.x,&B.y);
r=-1;
for(j=0;j<NP;j++)
work(A,B,P[j]);
for(j=0;j<NP;j++)
covers[j]+=cov[j];
if(r<0)
ok=0;
}
memset(ans,0,sizeof(ans));
for(i=0;i<NP;i++)
ans[covers[i]]++;
printf("Trial %d: ",++C);
if(ok)
for(i=0;i<=NC;i++)
printf("%d ",ans[i]);
else
printf("Impossible");
printf("\n\n");
}
return 0;
}