顺序栈的操作和数组差不多,代码比较简洁,完整代码在最下方。
在这个笔记里,只是顺序栈的最基本操作。
栈:栈是一种只能在
一端进行插入或删除操作的
线性表。
栈是一种在操作上稍加限制的线性表,即
栈本质上是线性表,所以相应地也有顺序表和链式表,在栈里就是
顺序栈和
链式栈。
其中,允许进行插入或删除的一端称为栈顶(Top)。栈顶由一个称为栈顶指针的位置指示器(其实就是一个变量,对于顺序栈,就是记录栈顶元素所在数组位置标号的一个整型变量;对于链式栈,就是记录栈顶元素所在结点地址的指针)来指示,它是动态变化的。表的另一端称为栈底,栈底是固定不变的。栈的插入和删除操作一般称为入栈和出栈。
栈的主要特点是
先进后出(FILO)
当n 个元素以某种顺序进栈,并且可以在任意时刻出栈(在满足先进后出的前提下)时,所获得的元素排列的数目N恰好满足函数Catalan()的计算,即
N=1/(n+1) * Cn 2n
----------------------------------------------------------------------------------------------------------------------------------
(1)顺序栈定义
typedef struct
{
int data[maxSize]; //存放栈中元素
int top; //栈顶指针
}SqStack;
(2)入栈
进栈必须是先移动指针,再进入元素
int push() { st.top = -1; //初始化栈,只需将栈顶指针设置为-1 if (st.top ==maxSize - 1) return 0; printf("请输入入栈数据!按回车键停止输入!"); printf("\n请输入数据:"); while (1) { ++(st.top); scanf_s("%d", &st.data[st.top]); char c = getchar(); if (c == '\n') break; } icount = st.top + 1; printf("\n栈内数据为:"); for (int i = 0; i <icount; i++) printf("%d ", st.data[i]);
return 1; }
(4)出栈
出栈必须先取出元素,再变动指针
int pop()
{
int a[maxSize] = { 0 };
if (st.top == -1)
return 0;
for (int i = 0; i <icount; i++)
{
a[i] = st.data[st.top];
(st.top)--;
}
printf("\n出栈数据为:");
for(int j=0;j<icount;j++)
printf("%d ", a[j]);
}
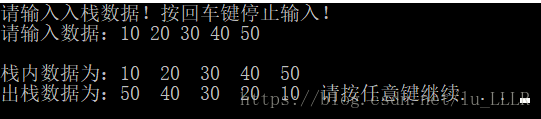
---------------------------------------------------------------------------------------------------------------------------------
完整代码:
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include <string.h>
#define maxSize 100
/*****************顺序栈定义********************/
typedef struct
{
int data[maxSize]; //存放栈中元素
int top; //栈顶指针
}SqStack;
SqStack st;
int icount;
/*********************进栈*********************/
int push()
{
st.top = -1; //初始化栈,只需将栈顶指针设置为-1
if (st.top ==maxSize - 1)
return 0;
printf("请输入入栈数据!按回车键停止输入!");
printf("\n请输入数据:");
while (1)
{
++(st.top);
scanf_s("%d", &st.data[st.top]);
char c = getchar();
if (c == '\n')
break;
}
icount = st.top + 1;
printf("\n栈内数据为:");
for (int i = 0; i <icount; i++)
printf("%d ", st.data[i]);
return 1;
}
/****************************出栈*************************/
int pop()
{
int a[maxSize] = { 0 };
if (st.top == -1)
return 0;
for (int i = 0; i <icount; i++)
{
a[i] = st.data[st.top];
(st.top)--;
}
printf("\n出栈数据为:");
for(int j=0;j<icount;j++)
printf("%d ", a[j]);
}
void main()
{
push();
pop();
system("pause");
}