二值图
二值图:只有 0 和 1 两种取值
灰度图
灰度图:对8位灰度取值,有256种取值, 0表示黑色,1表示白色。
彩色图像转灰度图像公式:
g
r
a
y
(
x
,
y
)
=
0.299
r
(
x
,
y
)
+
0.587
g
(
x
,
y
)
+
0.114
b
(
x
,
y
)
gray(x,y) = 0.299r(x,y) + 0.587g(x,y) + 0.114b(x,y)
gray(x,y)=0.299r(x,y)+0.587g(x,y)+0.114b(x,y)
import numpy as np
import matplotlib.pyplot as plt
import cv2 as cv
def show(img):
if img.ndim == 2:
plt.imshow(img, cmap='gray')
else:
plt.imshow(cv.cvtColor(img, cv.COLOR_BGR2RGB))
plt.show()
A = np.random.randint(0, 256, (2, 4), dtype=np.uint8)
show(A)
RGB彩色图
RGB彩色图:
- 真彩色:R、G、B通道各有8位取值
- 假彩色:8位表示256种颜色。暂不讨论。
B = np.random.randint(0, 256, (2, 4, 3), dtype=np.uint8)
show(B)
8位整型图像
C = np.uint8([-100, 0, 100, 255, 355, 27]).reshape((2, -1))
print(C) # 8位整型图像,自动转换非uint8为uint8
show(C)
# [[156 0 100]
# [255 99 27]]
浮点数图像
A2 = np.float32(A) # 浮点数图像
print(A2)
show(A2)
A2 /= 255
print(A2)
show(A2)
通道的分离与合并
显示原图像
img = cv.imread('dog.jpg')
show(img)
分离通道
b,g,r = cv.split(img)
show(b)
show(g)
show(r)
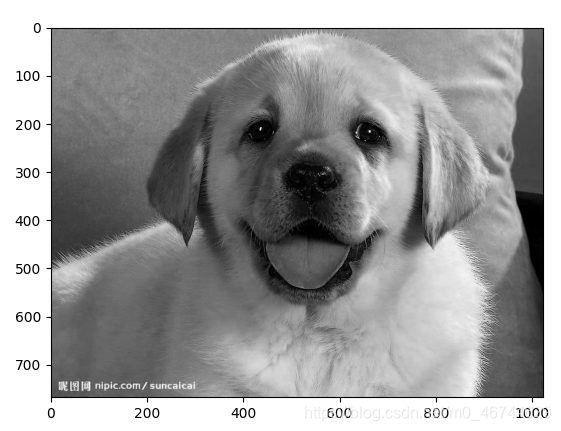
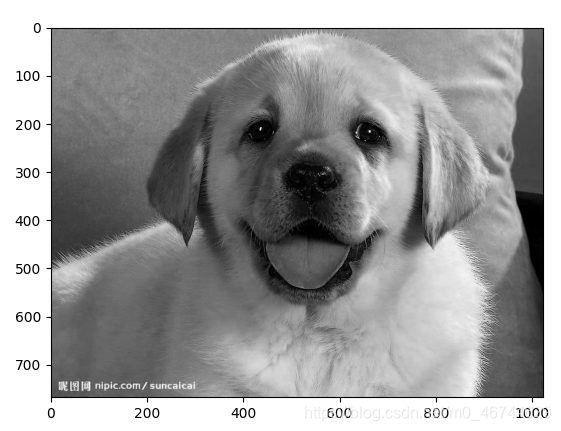
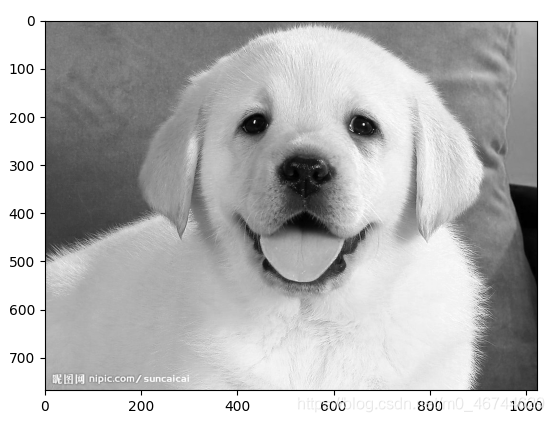
通道合并
img2 = cv.merge([b,g,r])
show(img2)
彩色图像转为灰度图
# 彩色图像转换为灰度图
gray1 = 1/3*b + 1/3*g + 1/3 * r
show(gray1)
gray2 = np.uint8(gray1) # 类型转换方式一
gray3 = gray1.astype(np.uint8) # 类型转换方式二
gray4 = cv.cvtColor(img, cv.COLOR_BGR2GRAY) # 彩色转灰度,上面的转换方式只为介绍原理,主要使用这个api
show(gray4)
图像二值化
# 图像二值化,需先将图像转为灰度图像后,才能二值化
thresh = 125
gray4[gray4 > thresh] = 255 # True部分设置位255
print(gray4)
show(gray4)
# [[255 255 255 ... 255 255 255]
# [255 255 255 ... 255 255 255]
# [255 255 255 ... 255 255 255]
# ...
# [255 255 255 ... 62 61 61]
# [255 255 255 ... 63 63 62]
# [255 255 255 ... 64 64 64]]
gray4[gray4 < thresh] = 0 # True部分设置位0
print(gray4)
show(gray4)
# [[255 255 255 ... 255 255 255]
# [255 255 255 ... 255 255 255]
# [255 255 255 ... 255 255 255]
# ...
# [255 255 255 ... 0 0 0]
# [255 255 255 ... 0 0 0]
# [255 255 255 ... 0 0 0]]
_, img_bin = cv.threshold(gray2, 125, 255, cv.THRESH_BINARY) # _ 表示二值化的阈值 125是阈值,小于125默认为0,大于125自行设定,当前为255, ,上面的转换方式只为介绍原理,主要使用这个api
# _, img_bin = cv.threshold(gray2, 125, 255, 0) # 与上一行功能一致
show(img_bin)
print(_)
# 125.0
完整代码
"""
二值图:只有0、1两种取值
灰度图:对8位灰度取值,有256种取值, 0:黑色|1:白色
彩色图像转灰度图像:gray(x,y) = 0.299r(x,y) + 0.587g(x,y) + 0.114b(x,y)
RGB彩色图:
真彩色:R、G、B通道各有8位取值
假彩色:8位表示256种颜色
"""
import numpy as np
import matplotlib.pyplot as plt
import cv2 as cv
def show(img):
if img.ndim == 2:
plt.imshow(img, cmap='gray')
else:
plt.imshow(cv.cvtColor(img, cv.COLOR_BGR2RGB))
plt.show()
A = np.random.randint(0, 256, (2, 4), dtype=np.uint8)
show(A)
B = np.random.randint(0, 256, (2, 4, 3), dtype=np.uint8)
show(B)
C = np.uint8([-100, 0, 100, 255, 355, 27]).reshape((2, -1))
print(C) # 8位整型图像,自动转换非uint8为uint8
show(C)
# [[156 0 100]
# [255 99 27]]
A2 = np.float32(A) # 浮点数图像
print(A2)
show(A2)
A2 /= 255
print(A2)
show(A2)
# 通道的分离和合并
img = cv.imread('dog.jpg')
show(img)
b,g,r = cv.split(img)
show(b)
show(g)
show(r)
img2 = cv.merge([b,g,r])
show(img2)
# 彩色图像转换为灰度图
gray1 = 1/3*b + 1/3*g + 1/3 * r
show(gray1)
gray2 = np.uint8(gray1) # 类型转换方式一
gray3 = gray1.astype(np.uint8) # 类型转换方式二
gray4 = cv.cvtColor(img, cv.COLOR_BGR2GRAY) # 彩色转灰度,上面的转换方式只为介绍原理,主要使用这个api
# 图像二值化
thresh = 125
gray4[gray4 > thresh] = 255 # True部分设置位255
print(gray4)
show(gray4)
gray4[gray4 < thresh] = 0 # True部分设置位0
print(gray4)
show(gray4)
_, img_bin = cv.threshold(gray2, 125, 255, cv.THRESH_BINARY) # _ 表示二值化的阈值 125是阈值,小于125默认为0,大于125自行设定,当前为255,上面的转换方式只为介绍原理,主要使用这个api
# _, img_bin = cv.threshold(gray2, 125, 255, 0) # 与上一行功能一致
show(img_bin)
print(_)